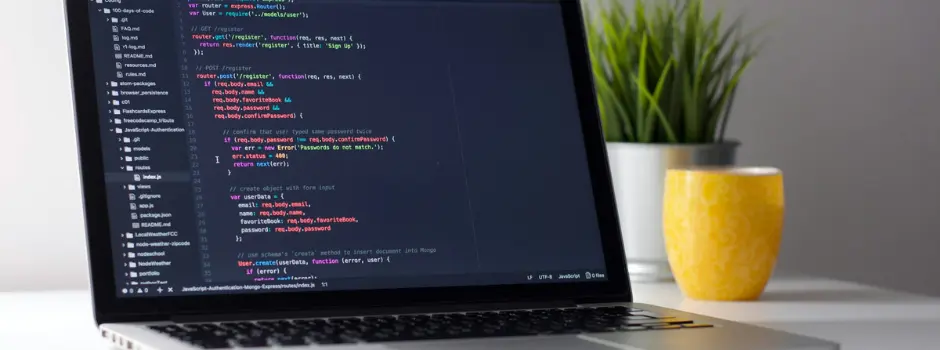
A Comprehensive Guide On Objects, Methods, and Classes In JavaScript
Mar 18, 2024 7 Min Read 400 Views
(Last Updated)
Object-oriented programming (OOP) is a fundamental concept in JavaScript, and it revolves around three fundamental aspects: objects, classes, and methods.
Understanding these three concepts and how they interact is crucial for mastering JavaScript programming language.
In this article, we’ll explore these concepts in depth and demonstrate their practical applications.
Table of contents
- Deciphering JavaScript Objects
- 1) Creating JavaScript Objects
- 2) Adding Properties to JavaScript Objects
- 3) Deleting Object Properties
- 4) Dealing with Object Keys
- 5) Accessing Object Properties
- 6) Dynamically Setting Properties
- 7) Object Method Shorthand
- 8) Advantages of Using Object Short Methods
- 9) The Object Spread Operator
- 10) Object Destructuring
- 11) The this Keyword in JavaScript
- Getting on with Classes in JavaScript
- 1) Understanding Prerequisites
- 2) The Advent of Classes in JavaScript
- 3) Advantages of Using JavaScript Classes
- 4) Creating a Class in JavaScript
- 5) Instantiating a Class
- 6) Class Inheritance
- 7) Advanced Concepts in JavaScript Classes
- Introduction to JavaScript Methods
- 1) Types of JavaScript Methods
- 2) Understanding this in JavaScript Methods
- 3) Arrow Functions and Methods
- 4) Prototype Methods in JavaScript
- 5) Static Methods in JavaScript
- 6) Private and Protected Methods
- 7) Getters and Setters
- 8) Class Fields
- 9) Inheritance in JavaScript Methods
- 10) Polymorphism in JavaScript Methods
- Concluding Thoughts...
- FAQs
- What is an object type in JavaScript?
- What is the difference between method and class in JavaScript?
- What is a property in JavaScript?
- What is an event in JavaScript?
Deciphering JavaScript Objects
Picture a real-world object, such as a car. It has properties like color and model, and it can perform actions such as driving and honking. Similarly, JavaScript objects have properties and methods (actions). Properties represent values associated with an object, while methods represent tasks that can be performed by objects.
Also Read: Best JavaScript Roadmap Beginners Should Follow 2024
JavaScript objects are mutable—they are addressed by reference, not by value. If the person is an object, then changing the name property of the person changes the name for the “person” object, because the person and the person are the same object.
const person = {
name:'John',
age:30,
hobbies:[
'reading','playing','sleeping'
],
greet:function(){
console.log('Hello World')
}
}
1) Creating JavaScript Objects
JavaScript provides several ways to create objects:
- Using an Object initializer is the simplest way to create a JavaScript Object. This is also called creating an object using a literal notation.
- Using a constructor function or a class to define an object type, then create an instance of the object with new.
- Using the Object.create method to create a new object, using an existing object as the prototype of the newly created object.
Here’s an example of creating an object using literal notation:
const person = {
name:'John',
age:30,
hobbies:[
'reading','playing','sleeping'
],
greet:function(){
console.log('Hello World')
}
}
In the code above, person
is an object with properties name
, age
, hobbies
, and a method greet
.
Also Read: How to Render an Array of Objects in React? [in 3 easy steps]
2) Adding Properties to JavaScript Objects
JavaScript objects are dynamic, meaning properties can be added, changed, or deleted after an object is created.
Properties can be added to an object by simply giving it a value. Let’s add a gender
property to our person
object:
person.gender = 'male';
The name
property of the person
object now has the value ‘David’.
3) Deleting Object Properties
The delete
operator in JavaScript removes a property from an object. If the object inherits a property from a prototype, and you delete the property from the object, the object will continue to use the inherited property.
delete person.gender;
The gender
property is now removed from the person
object.
Also Find 4 Key Differences Between == and === Operators in JavaScript
4) Dealing with Object Keys
In JavaScript, object keys are always strings. Whether you use an integer, a string, a symbol, or any other type as key, they are converted into strings (except for symbols).
let person = {
'first name':'John',
age:30,
hobbies:[
'reading','playing','sleeping'
]
}
In the example above, the key first name
is a special key because it contains a space. It’s enclosed in quotation marks to avoid a syntax error.
5) Accessing Object Properties
There are two main ways to access or get the values of properties within an object:
- Dot notation:
objectName.propertyName
- Bracket notation:
objectName['propertyName']
If the property name is a number or a special key, you have to use bracket notation:
console.log(person['first name']); // Outputs: 'John'
Must Read: Variables and Data Types in JavaScript: A Complete Guide
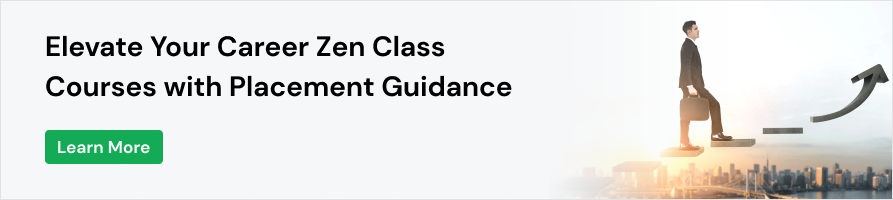
6) Dynamically Setting Properties
JavaScript allows you to set properties dynamically using bracket notation. This is useful when you need to create properties based on certain conditions.
const userInput ='level';
let person = {
'first name':'John',
age:30,
[userInput]: 'see',
}
console.log(person); // Outputs: { 'first name': 'John', age: 30, level: 'see' }
7) Object Method Shorthand
In ES6, there is a shorthand for defining methods in an object. You can remove the function keyword and the colon.
let person = {
name:'John',
age:30,
greet(){
console.log('Hello World')
}
}
8) Advantages of Using Object Short Methods
Object short methods have several advantages over regular methods:
- Conciseness: They allow for more compact and readable code.
- Performance: The shorter syntax makes it easier to write and maintain code.
- Reusability: You can easily reuse object short methods in other objects.
- Organization: With object-short methods, you can group related methods within an object and keep your code organized.
Also Explore: Constructors in JavaScript: 6 Uses Every Top Programmer Must Know
9) The Object Spread Operator
The object spread operator (...
) is used to take the properties and values of one object and copy them (spread them) into another object.
let person = {
name:'John',
age:30,
hobbies:[
'reading','playing','sleeping'
]
}
const person2 ={...person};
console.log(person2.age); // Outputs: 30
10) Object Destructuring
Object destructuring is a JavaScript feature that allows you to extract properties from objects and bind them to variables.
const person = { name: 'David', age: 39 };
const { name, age } = person;
console.log(name); // Outputs: 'David'
console.log(age); // Outputs: 39
11) The this
Keyword in JavaScript
this
is a special keyword in JavaScript that refers to the context in which a function is called. In the context of an object method, this
refers to the object the method is called on.
let person = {
name:'John',
age:30,
greet:function(){
return `My name is ${this.name}, and my age is ${this.age} years old`;
},
}
console.log(person.greet()); // Outputs: 'My name is John, and my age is 30 years old.'
Also Read: Most Popular JavaScript Front-End Tools in 2024
Getting on with Classes in JavaScript
Classes in JavaScript are a blueprint for creating objects. They encapsulate data with code to manipulate that data.
They are primarily syntactic sugar over JavaScript’s existing prototype-based inheritance. The class syntax does not introduce a new object-oriented inheritance model to JavaScript.
1) Understanding Prerequisites
Before we dive headfirst into our exploration of classes in JavaScript, there are a few prerequisites you should be familiar with:
- Class Diagrams: These will be employed to illustrate our examples.
- OOP Concepts: A basic understanding of the principles of Object-Oriented Programming is crucial.
- Prototypal Inheritance: This is a fundamental aspect of JavaScript and forms the basis for classes.
- Constructor Functions: Familiarity with constructor functions in JavaScript is beneficial.
2) The Advent of Classes in JavaScript
The advent of ECMAScript 2015 (ES6) marked a significant milestone in JavaScript’s evolution, introducing the concept of classes.
This was a game-changer for developers, providing a cleaner, more streamlined approach to implementing object-oriented programming patterns.
While JavaScript continues to operate on a prototype-based inheritance model, classes offer a layer of syntactic sugar over this model, making it more digestible and user-friendly.
They brought JavaScript closer to other OOP-based programming languages such as C++ and Java, thereby broadening its appeal.
Also Read: All About Loops in JavaScript: A Comprehensive Guide
2.1) The Essence of JavaScript Classes
At their core, classes in JavaScript are essential functions. Before classes made their debut, developers used constructor functions to achieve object-oriented programming in JavaScript.
The advent of classes simply provided a cleaner, more intuitive syntax for doing so.
2.2) The Role of Constructors in Classes
Within a JavaScript class, the constructor method plays a crucial role. This special method is used for creating and initializing objects created with that class.
There can only be one special method with the name “constructor” in a class. Having more than one occurrence of a constructor method in a class will throw a SyntaxError error.
3) Advantages of Using JavaScript Classes
The introduction of classes in JavaScript has made it significantly easier for developers to build software around OOP concepts. Some of the advantages include:
- Enhanced readability and organization: Classes help keep code organized and easier to read, making it simpler to work with.
- Reusability: Classes allow for code reusability, which is a key principle of OOP.
- Encapsulation: Classes also allow for the encapsulation of data, which is another fundamental principle of OOP.
Also Read: Best JavaScript Frameworks in 2024
4) Creating a Class in JavaScript
The syntax for creating a class involves the class
keyword followed by the name of the class. The methods and properties are defined inside the class using the constructor
function. For example:
class Car {
constructor(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
drive() {
console.log(this.make + ' ' + this.model + ' is driving...');
}
}
In the Car
class, make
, model
, and year
are properties, and drive
is a method. The constructor
function is automatically called when a new object is created from the class.
5) Instantiating a Class
To create an object from a class, you use the new
keyword followed by the class name and any necessary arguments. For example:
let myCar = new Car('Toyota', 'Camry', 2005);
The myCar
object is an instance of the Car
class.
Also Read: Functions in JavaScript: Important Things To Know [2024]
6) Class Inheritance
Inheritance is a key feature of OOP. It allows you to create a new class that inherits the properties and methods of an existing class. To create a class that inherits from another class, you use the extends
keyword.
For example, suppose we want a Truck
class that has all the properties and methods of the Car
class, plus a tow
method.
class Truck extends Car {
constructor(make, model, year) {
super(make, model, year);
}
tow() {
console.log(this.make + ' ' + this.model + ' is towing...');
}
}
In the Truck
class, the super
keyword is used to call the constructor of the parent class. This ensures that the Truck
class has all the properties of the Car
class.
7) Advanced Concepts in JavaScript Classes
Now that we have a basic understanding of JavaScript classes, let’s delve into some more advanced concepts.
7.1) Abstract Functions and Inheritance
In JavaScript, a class can inherit features from another class, a process known as inheritance. This can be achieved using the extends
keyword.
Abstract functions are functions that exist in the parent class but do not have a body, meaning that they don’t perform any action themselves. The responsibility to provide a specific implementation of the function lies with the class that extends the parent class.
7.2) Static Keywords in JavaScript
The static
keyword in JavaScript defines a static method for a class. Static methods aren’t called on instances of the class. Instead, they’re called on the class itself.
7.3) Private Members in JavaScript
JavaScript classes also support private members — properties and methods that can only be accessed from within the class that defines them. They are created by prefixing a hash #
to their names.
Also Read: What are Events in JavaScript? A Complete Guide
Introduction to JavaScript Methods
In JavaScript, methods are essentially functions that are stored as object properties. They operate on the data contained within the object and can be invoked using the dot notation.
JavaScript methods allow developers to perform actions on objects and manipulate object data.
let person = {
firstName: "John",
lastName : "Doe",
fullName : function() {
return this.firstName + " " + this.lastName;
}
};
In the above example, fullName
is a method of the person
object, and it returns the full name of the person.
Must Read: Java vs JavaScript: Top 3 Comparisons
1) Types of JavaScript Methods
JavaScript offers a plethora of built-in methods that can be used on different data types. These methods can be broadly classified into:
- Array Methods: JavaScript arrays come with a host of methods like
push()
,pop()
,shift()
,unshift()
,splice()
,slice()
,sort()
,filter()
,map()
, etc. These methods allow developers to manipulate array elements effectively. - String Methods: String methods in JavaScript, such as
charAt()
,concat()
,indexOf()
,slice()
,split()
,toLowerCase()
,toUpperCase()
, etc., provide a way to manipulate and work with strings. - Number Methods: JavaScript number methods like
toString()
,toExponential()
,toFixed()
,toPrecision()
,valueOf()
, etc., are used to manipulate numbers. - Date Methods: Date methods help in managing and manipulating dates in JavaScript. Key methods include
getDate()
,getDay()
,getFullYear()
,getHours()
,getMinutes()
, etc.
Explore: JavaScript Modules: A Comprehensive Guide [2024]
2) Understanding this
in JavaScript Methods
The this
keyword is a significant aspect of JavaScript methods. It refers to the object from which the method was invoked. Essentially, this
gives methods access to the object’s properties and other methods.
Consider the following example:
let car = {
make: "Toyota",
model: "Corolla",
displayCar: function() {
return this.make + " " + this.model;
}
};
In this case, this
within the displayCar
method refers to the car
object.
3) Arrow Functions and Methods
JavaScript ES6 introduced arrow functions, a new way to define functions. However, arrow functions behave differently when used as methods. Unlike regular functions, arrow functions do not have their own this
context; they inherit it from the surrounding code.
let car = {
make: "Toyota",
model: "Corolla",
displayCar: () => {
return this.make + " " + this.model; // this here is not bound to the car object
}
};
In the above example, this
inside the arrow function does not refer to the car
object, which can lead to unexpected results.
4) Prototype Methods in JavaScript
Prototype methods are another important concept in JavaScript. Each object has a prototype
property that allows you to add methods and properties that can be shared across instances of an object.
function Car(make, model) {
this.make = make;
this.model = model;
}
Car.prototype.displayCar = function() {
return this.make + " " + this.model;
};
let myCar = new Car("Toyota", "Corolla");
myCar.displayCar(); // "Toyota Corolla"
In the above example, displayCar
is a method added to the prototype of Car
, and it can be used in all instances of Car
.
Also Read: Best Techniques for Creating Seamless Animations with CSS and JavaScript [2024]
5) Static Methods in JavaScript
Static methods in JavaScript are defined on the class itself, not on instances of the class. They’re often used for utility functions that don’t rely on any object data.
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
static compareCar(car1, car2) {
return car1.make === car2.make && car1.model === car2.model;
}
}
In this example, compareCar
is a static method. It can be invoked directly from the Car
class, not from an instance of the class.
Also Read: Arrays in JavaScript: A Comprehensive Guide
6) Private and Protected Methods
JavaScript supports private and protected methods, which limit the accessibility of certain methods to the class itself or its subclasses.
- Private Methods: Private methods are declared using a
#
character and are only accessible within the class they are defined. - Protected Methods: Protected methods, while not explicitly available in JavaScript, can be mimicked by using certain conventions, such as prefixing method names with an underscore (
_
).
7) Getters and Setters
Getters and setters are special types of methods in JavaScript that allow you to define the ways to get and set the values of your object properties.
class Car {
constructor(make, model) {
this._make = make;
this._model = model;
}
get make() {
return this._make;
}
set make(value) {
this._make = value;
}
}
In the above example, make
is a property with a getter and a setter. The getter returns the value of _make
, and the setter sets the value of _make
.
8) Class Fields
Class fields are a relatively new addition to JavaScript. They allow you to add instance and static properties directly to classes. Class fields can be public or private, with private fields being denoted by a #
character.
class Car {
make = 'Toyota'; // public field
#model = 'Corolla'; // private field
}
In this example, make
is a public field, and #model
is a private field.
Must Read: The Beginner’s Guide To Closures In JavaScript
9) Inheritance in JavaScript Methods
Inheritance allows you to create new classes that inherit the properties and methods of existing classes. In JavaScript, you achieve inheritance using the extends
keyword.
class Vehicle {
constructor(make, model) {
this.make = make;
this.model = model;
}
displayVehicle() {
return this.make + ' ' + this.model;
}
}
class Car extends Vehicle {
// Car class inherits from Vehicle class
}
In this example, the Car
class inherits from the Vehicle
class, gaining access to its constructor and displayVehicle
method.
10) Polymorphism in JavaScript Methods
Polymorphism is a concept that allows a method to behave differently based on the object that invokes it. In JavaScript, polymorphism is implemented through method overriding, where a subclass provides a different implementation of a method already provided by its parent class.
class Vehicle {
displayVehicle() {
return 'This is a vehicle';
}
}
class Car extends Vehicle {
displayVehicle() {
return 'This is a car';
}
}
In this example, the displayVehicle
method in the Car
class overrides the displayVehicle
method in the Vehicle
class.
Must Explore: Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
Concluding Thoughts…
In conclusion, objects, classes, and methods in JavaScript are the pillars of object-oriented programming in the language.
Understanding these concepts is key to mastering JavaScript and creating effective, efficient code. The best way to do so is by learning Full Stack Development, where you’ll gain a deeper understanding of all these programming concepts and more making it a stellar career choice.
These fundamentals set the foundation for more complex concepts like inheritance, encapsulation, and polymorphism, enabling developers to write clean, modular, and reusable code.
FAQs
What is an object type in JavaScript?
In JavaScript, an object type refers to a data structure that stores key-value pairs, allowing for complex data representation and manipulation.
What is the difference between method and class in JavaScript?
In JavaScript, a method is a function associated with an object, while a class is a blueprint for creating objects with shared properties and methods.
What is a property in JavaScript?
In JavaScript, a property is a characteristic of an object, defined as a key-value pair that describes the object’s attributes or features.
Did you enjoy this article?