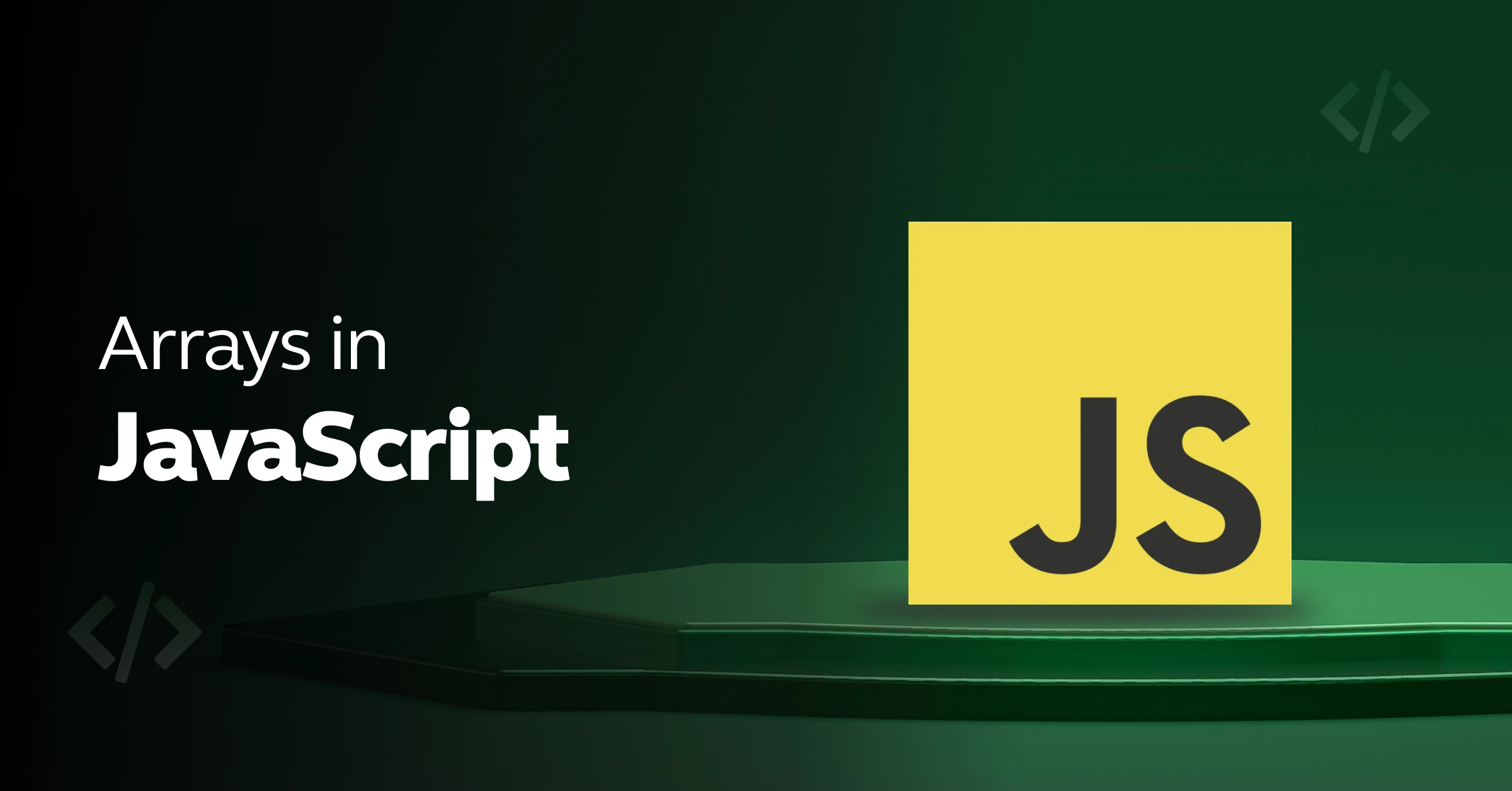
Arrays in JavaScript: A Comprehensive Guide
Apr 08, 2025 5 Min Read 3774 Views
(Last Updated)
Ever heard of a toolbox that can hold many tools at once? Well, in JavaScript, arrays are like that toolbox! They help us keep lots of information organized neatly in one place. Understanding arrays is very important in JavaScript.
In this blog series, we’re going to explore arrays in JavaScript step by step. We’ll start with the basics and gradually move on to more interesting concepts. By the time we’re done, you’ll be able to use arrays to make your code more awesome and solve all sorts of problems.
Let’s go on this fun adventure together and learn how to make our JavaScript code even better!
Table of contents
- What is an Array in JavaScript?
- Creating and Initializing Arrays
- Syntax for Array Declaration
- Initializing Arrays with Values
- Creating Empty Arrays
- Basic Operations on JavaScript Arrays
- Accessing Elements of an Array
- Accessing the First Element of an Array
- Accessing the Last Element of an Array
- Modifying the Array Elements
- Adding Elements to the Array
- Removing Elements from an Array
- Array Length
- Increase and Decrease the Array Length
- Iterating Through Array Elements
- Array Concatenation
- Conversion of an Array to String
- Check the Type of Arrays
- JavaScript Array Methods
- Conclusion
- FAQs
- What are the advantages of using arrays in JavaScript?
- How do you access elements within a multidimensional array in JavaScript?
- What is the difference between the push() and unshift() methods in JavaScript arrays?
What is an Array in JavaScript?
An array in JavaScript is a special container that allows you to store multiple pieces of data under one variable name. Each piece of data in an array is called an element, and you can access these elements using their position or index in the array.
For example, you can use an array to store a list of numbers, words, or even other arrays. Arrays in JavaScript are flexible and can dynamically adjust their size, meaning you can add or remove elements as needed. This makes them incredibly versatile for organizing and manipulating data in your JavaScript code.
Also Read: How to Render an Array of Objects in React? [in 3 easy steps]
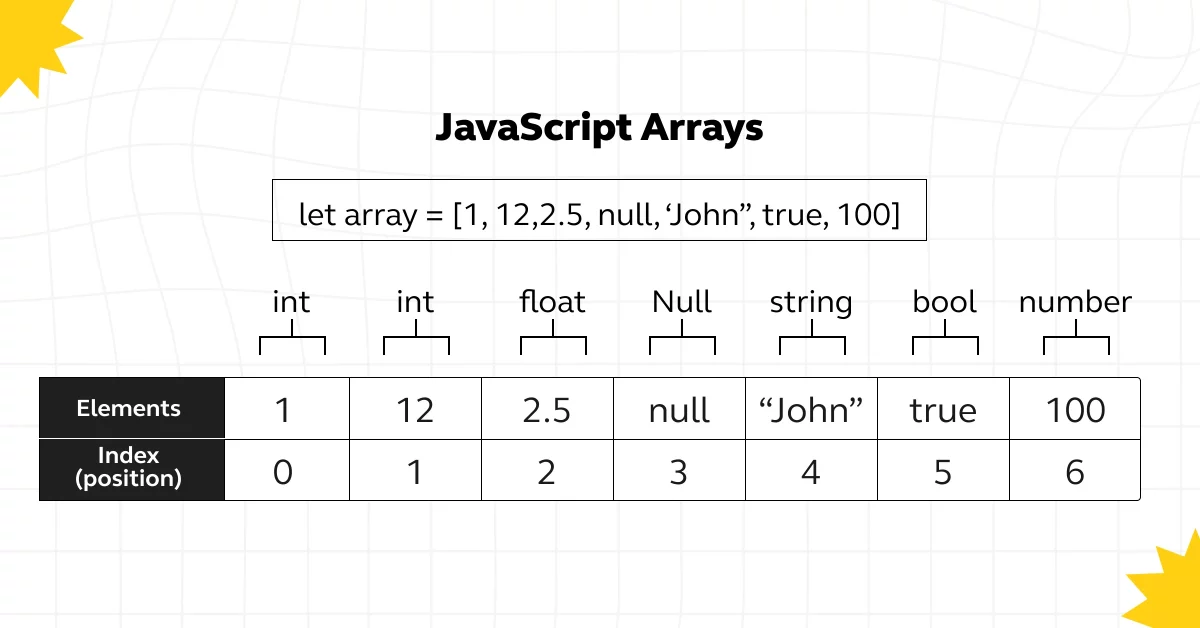
Now that we’ve explored what an array in JavaScript is and the flexibility it offers in managing collections of data, let’s learn how we can create and initialize arrays to put this powerful tool to work in our coding projects.
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
Creating and Initializing Arrays
Arrays in JavaScript are incredibly versatile and easy to work with. Let’s learn how you can create and initialize arrays in your code.
1. Syntax for Array Declaration
To declare an array in JavaScript, you use square brackets []. Here’s the basic syntax:
let myArray = [];
This line of code creates an empty array named myArray. You can then add elements to it or initialize it with values.
Also Read: JavaScript Tools Every Developer Should Know
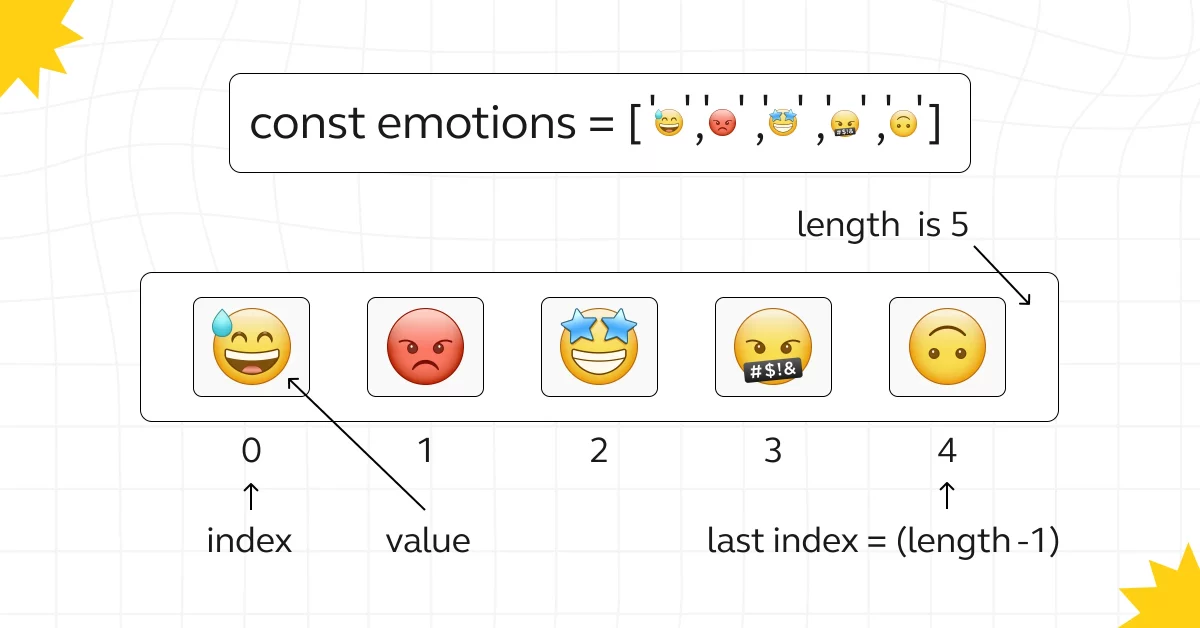
2. Initializing Arrays with Values
You can initialize an array with values when you create it. Here’s how you do it:
let fruits = [‘apple’, ‘banana’, ‘orange’, ‘kiwi’];
In this example, fruits is an array containing four strings: ‘apple’, ‘banana’, ‘orange’, and ‘kiwi’.
3. Creating Empty Arrays
If you want to create an empty array without any initial values, you can do so by simply declaring an array without any elements:
let emptyArray = [];
This creates an empty array named emptyArray that you can later populate with values as needed.
Arrays are incredibly flexible, allowing you to store different types of data, including numbers, strings, objects, and even other arrays. Understanding how to create and initialize arrays is fundamental to working effectively with JavaScript’s data structures.
Also Read: Best JavaScript Roadmap Beginners Should Follow
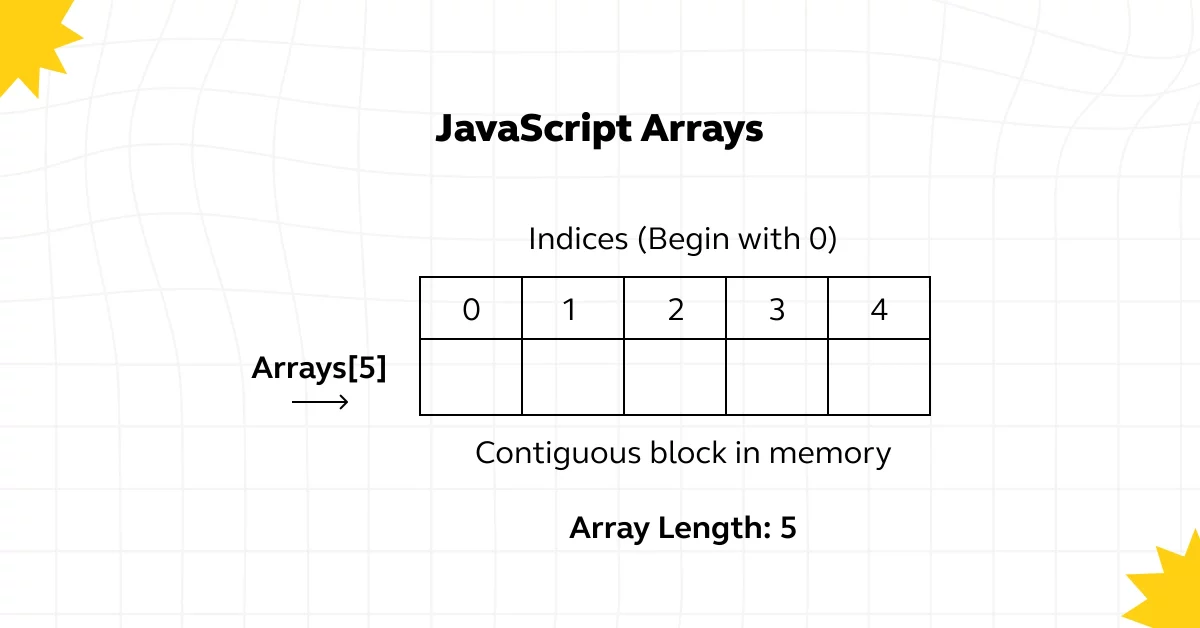
Having explored the essentials of creating and initializing arrays in JavaScript, let’s shift our focus to the fundamental operations that can be performed on these arrays.
Basic Operations on JavaScript Arrays
JavaScript arrays offer a plethora of operations for managing and manipulating data efficiently. Let’s explore some fundamental operations:
1. Accessing Elements of an Array
To access an element at a specific index in an array, you can use square brackets [] with the index number.
let fruits = [‘apple’, ‘banana’, ‘orange’];
console.log(fruits[1]);
Output: ‘banana’
2. Accessing the First Element of an Array
You can access the first element of an array by using index 0.
console.log(fruits[0]);
Output: ‘apple’
3. Accessing the Last Element of an Array
To access the last element of an array, you can use the index length – 1.
console.log(fruits[fruits.length – 1]);
Output: ‘orange’
Find Out 4 Key Differences Between == and === Operators in JavaScript
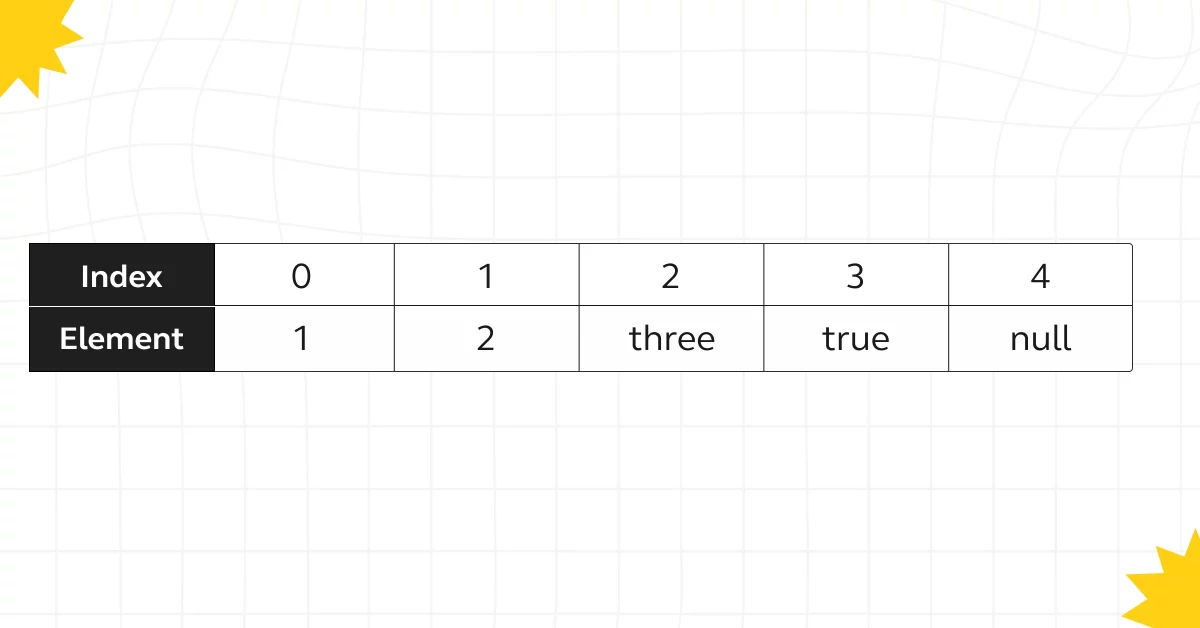
4. Modifying the Array Elements
You can modify elements in an array by assigning a new value to a specific index.
fruits[1] = ‘grape’;
console.log(fruits);
Output: [‘apple’, ‘grape’, ‘orange’]
5. Adding Elements to the Array
You can add elements to the end of an array using the push() method.
fruits.push(‘kiwi’);
console.log(fruits);
Output: [‘apple’, ‘grape’, ‘orange’, ‘kiwi’]
6. Removing Elements from an Array
To remove elements from an array, you can use methods like pop(), shift(), or splice().
fruits.pop(); // Removes the last element
console.log(fruits);
Output: [‘apple’, ‘grape’, ‘orange’]
fruits.shift(); // Removes the first element
console.log(fruits);
Output: [‘grape’, ‘orange’]
Also Read: Master JavaScript Frontend Roadmap: From Novice to Expert
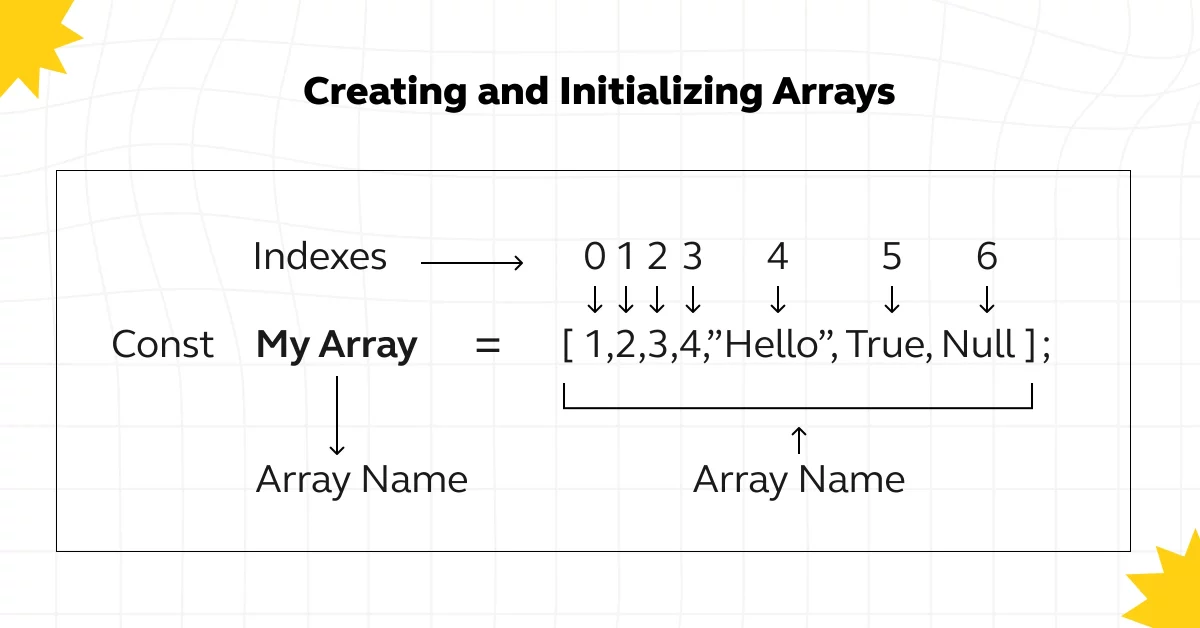
7. Array Length
You can get the length of an array using the length property.
console.log(fruits.length);
Output: 2
8. Increase and Decrease the Array Length
You can increase or decrease the length of an array using the length property. Be cautious as it can truncate or add undefined values.
fruits.length = 5; // Increase length
console.log(fruits);
Output: [‘grape’, ‘orange’, undefined, undefined, undefined]
fruits.length = 2; // Decrease length
console.log(fruits);
Output: [‘grape’, ‘orange’]
9. Iterating Through Array Elements
You can iterate through all elements of an array using loops like for loop or array methods like forEach().
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
Output:
‘grape’
‘orange’
Also Read: Build a Search Component in React [just in 3 simple steps]
10. Array Concatenation
You can concatenate two arrays using the concat() method.
javascript let moreFruits = [‘apple’, ‘banana’]; let allFruits = fruits.concat(moreFruits); console.log(allFruits);
Output: [‘grape’, ‘orange’, ‘apple’, ‘banana’]
11. Conversion of an Array to String
You can convert an array to a string using the join() method.
javascript console.log(fruits.join(‘, ‘));
Output: ‘grape, orange’
12. Check the Type of Arrays
To check if a variable is an array, you can use the Array.isArray() method.
javascript console.log(Array.isArray(fruits));
Output: true
These basic operations form the foundation for working with arrays in JavaScript. Understanding them will enable you to manipulate arrays effectively in your code.
Also Read: 30 Best JavaScript Project Ideas For You [3 Bonus Portfolio Projects]
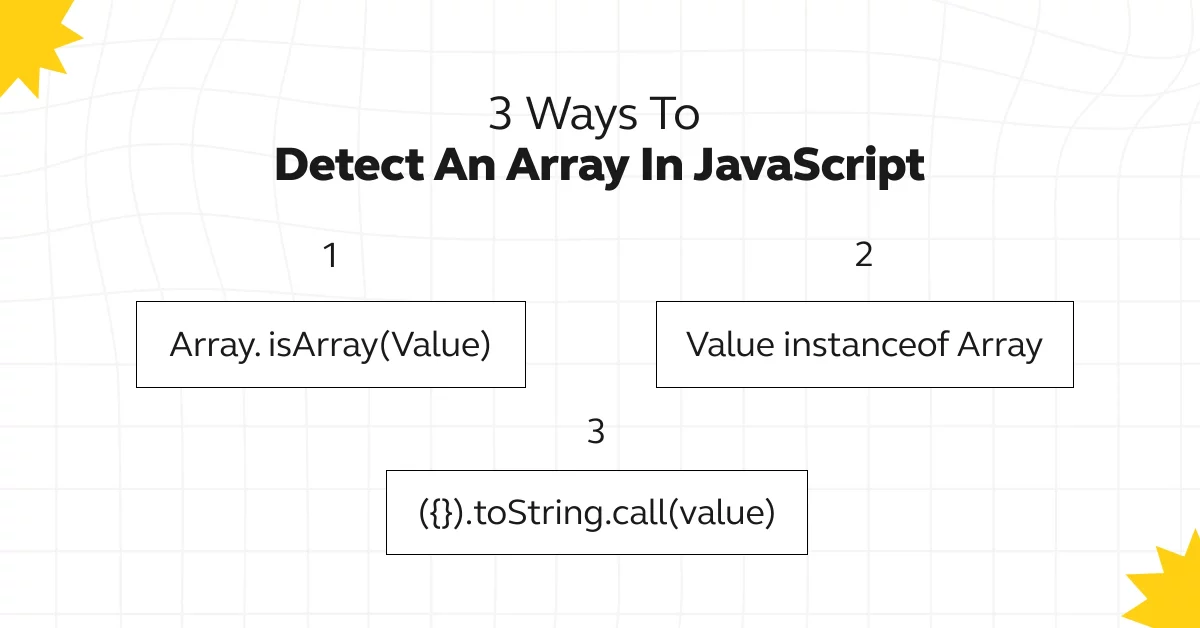
Building on our understanding of basic operations on JavaScript arrays, let’s now learn the powerful array methods that JavaScript offers. These methods provide a vast array of functionalities, from manipulating elements to iterating over arrays, enhancing our ability to handle and transform data efficiently.
JavaScript Array Methods
JavaScript provides a rich set of methods for working with arrays efficiently. Let’s explore some of the most commonly used array methods:
1. map(): Creates a new array by applying a function to each element of the original array.
2. filter(): Creates a new array with elements that pass a certain condition specified by a callback function.
3. sort(): Sorts the elements of an array in place, either alphabetically or numerically, based on a provided function.
4. forEach(): Executes a provided function once for each array element.
5. concat(): Returns a new array by combining the elements of the original array with other arrays or values.
6. every(): Checks if all elements in an array pass a specified condition provided by a callback function.
7. some(): Checks if at least one element in an array passes a specified condition provided by a callback function.
8. includes(): Checks if an array includes a certain element, returning true or false.
9. join(): Joins all elements of an array into a string, optionally separated by a specified separator.
10. reduce(): Executes a reducer function on each element of the array, resulting in a single output value.
Also Read: 42 JavaScript Questions Towards Better Interviews
11. find(): Returns the value of the first element in the array that satisfies a provided testing function.
12. findIndex(): Returns the index of the first element in the array that satisfies a provided testing function.
13. indexOf(): Returns the index of the first occurrence of a specified element in the array, or -1 if not found.
14. fill(): Fills all the elements of an array from a start index to an end index with a static value.
15. slice(): Returns a shallow copy of a portion of an array into a new array object selected from start to end.
16. reverse(): Reverses the order of the elements in an array in place.
17. push(): Adds one or more elements to the end of an array and returns the new length of the array.
18. pop(): Removes the last element from an array and returns that element.
19. shift(): Removes the first element from an array and returns that element, shifting all other elements to a lower index.
20. unshift(): Adds one or more elements to the beginning of an array and returns the new length of the array.
These array methods offer powerful functionalities for manipulating, transforming, and querying arrays in JavaScript, enabling developers to write cleaner and more efficient code.
Also Read: Variables and Data Types in JavaScript: A Complete Guide
Ready to elevate your coding skills and learn web development? Join GUVI’s Full Stack Development Course and start your journey from mastering JavaScript arrays to building comprehensive web applications. Enroll today!
Conclusion
Arrays are the building blocks of JavaScript programming, empowering developers to create dynamic and interactive applications. By understanding the power of arrays, you’ll unlock endless possibilities for innovation and creativity in your JavaScript projects. Keep coding, exploring, and pushing the boundaries of what you can achieve with arrays in JavaScript!
Also Explore: All About Loops in JavaScript: A Comprehensive Guide
FAQs
Arrays provide a convenient way to store and access multiple elements of data under a single variable name. They offer flexibility in managing collections of data, enabling easy iteration, manipulation, and organization.
With built-in methods and functionalities, arrays streamline code, making it more readable and efficient.
In JavaScript, a multidimensional array is an array of arrays, creating a grid-like structure. To access elements within a multidimensional array, you use multiple sets of square brackets, with each bracket representing the index of the nested array.
For example, myArray[0][1] would access the element at the second position of the first nested array in myArray.
Both push() and unshift() methods add elements to an array, but they do so at different ends. push() adds elements to the end of the array, increasing its length.
unshift() adds elements to the beginning of the array, shifting existing elements to higher indices.
For example, myArray.push(‘newElement’) adds ‘newElement’ to the end of myArray, while myArray.unshift(‘newElement’) adds it to the beginning.
Did you enjoy this article?