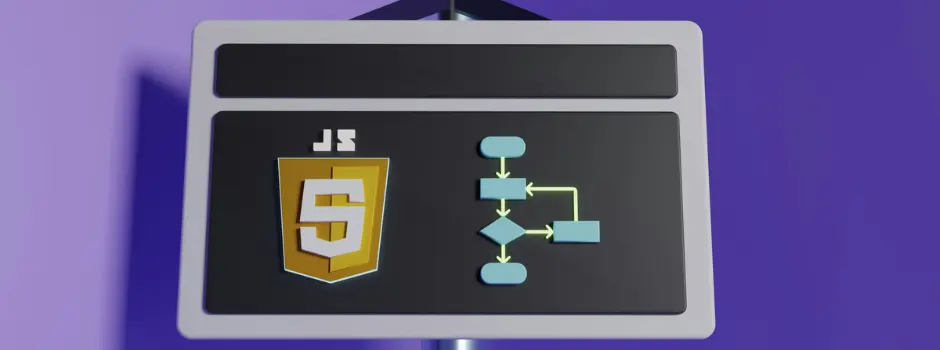
All About Loops in JavaScript: A Comprehensive Guide
Jun 23, 2025 5 Min Read 7022 Views
(Last Updated)
Loops are an essential part of any programming language, and JavaScript is no exception. They allow us to execute a set of instructions repeatedly, making our code more efficient and reducing redundancy.
In this comprehensive guide, we will explore the different types of loops in JavaScript, their syntax, and how to effectively use them in your code.
Table of contents
- Introduction to Loops in JavaScript
- 1) The for Loop
- 2) The do...while Loop
- 3) The while Loop
- 4) The Labeled Statement
- 5) The break Statement
- 6) The continue Statement
- 7) The for...in Loop
- 8) The for...of Loop
- Best Practices for Using Loops
- Common Mistakes to Avoid
- Concluding Thoughts...
- FAQs
- What are JavaScript loops?
- How many loops are there in JavaScript?
- What is the use of for of loop in JavaScript?
- What is an array in JavaScript?
Introduction to Loops in JavaScript
Loops are control structures that allow us to repeatedly execute a block of code until a certain condition is met.
They are used when we want to operate multiple times without having to write the same code over and over again. In JavaScript, there are several types of loops, each with its own syntax and use cases.
1) The for
Loop
The for
Loop is one of the most commonly used loops in JavaScript. It allows us to execute a block of code a specified number of times. The syntax of the for loop is as follows:
for (initialization; condition; increment) {
// code to be executed
}
- The
initialization
The step is executed only once before the loop starts. It is where we declare and initialize any variables used in the loop. - The
condition
is evaluated before each iteration, and if it evaluates totrue
The loop continues. - The
increment
Step is executed after each iteration, and is typically used to update the loop control variable.
Let’s see an example to illustrate the usage of the for
loop:
for (let i = 1; i < 8; i++) {
console.log(i);
}
In this example, the loop will iterate five times, printing the values from 1 to 7 to the console.
Must Read: Master JavaScript Frontend Roadmap: From Novice to Expert
2) The do...while
Loop
The do...while
Loop is similar to the while
loop, but with one key difference: the condition is evaluated after the code block has been executed.
This means that the code block will always execute at least once, regardless of the condition. The syntax of the do...while
The loop is as follows:
do {
// code to be executed
} while (condition);
Let’s look at an example to understand how the do...while
loop works:
let i = 0;
do {
console.log(i);
i++;
} while (i < 10);
In this example, the code block will execute once, printing the value i
to the console. Then, the condition i <
10 is checked.
If it evaluates to true
The loop continues, and the code block is executed again. This process repeats until the condition becomes false
. It is one of the most versatile loops in JavaScript.
3) The while
Loop
The while
A loop is used to execute a block of code as long as a specified condition is true
. It is similar to the do...while
loop, but the condition is evaluated before the code block is executed.
The syntax of the while
The loop is as follows:
while (condition) {
// code to be executed
}
Let’s see an example to understand how the while
loop works:
let i = 0;
while (i < 17) {
console.log(i);
i++;
}
In this example, the code block will execute as long as the condition i <
17 is true
. The value of i
will be printed to the console and then i
will be incremented by 1.
This process will repeat until the condition becomes false
. It is one of the most used loops in JavaScript.
Also Read: JavaScript Tools Every Developer Should Know
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
4) The Labeled Statement
In JavaScript, you can use labels to identify a loop or a block of code. Labels are often used with the break
and continue
statements to control the flow of the program. The syntax of a labeled statement is as follows:
label: statement
Let’s look at an example of using labels with the for
loop and the break
statement:
outerLoop: for (let i = 0; i < 9; i++) {
innerLoop: for (let j = 0; j < 9; j++) {
if (i === 1 && j === 1) {
break outerLoop;
}
console.log(`i = ${i}, j = ${j}`);
}
}
In this example, we have an outer loop labeled as outerLoop
and an inner loop labeled as innerLoop
. When the condition i === 1 && j === 1
is met, the break
The statement is executed, causing the program to break out of the outer loop. This allows us to selectively break out of multiple nested loops.
5) The break
Statement
The break
The statement is used to exit a loop or switch statement prematurely. When the break
statement is encountered, the program flow immediately moves to the next statement outside of the loop or switch.
This is useful when we want to terminate a loop early based on a certain condition. Let’s see an example to understand how the break
statement works:
for (let i = 0; i < 7; i++) {
if (i === 5) {
break;
}
console.log(i);
}
In this example, the loop will iterate four times, printing the values from 0 to 4 to the console. When i
it becomes 5, the break
statement is executed, and the loop is terminated.
Develop your first project with us: 10 Best HTML and CSS Project Ideas for Beginners
6) The continue
Statement
The continue
The statement is used to skip the current iteration of a loop and move on to the next iteration. Unlike the break
statement, which terminates the loop, the continue
statement only affects the current iteration.
This is useful when we want to skip certain iterations based on a condition. Let’s look at an example to understand how the continue
statement works:
for (let i = 0; i < 10; i++) {
if (i === 9) {
continue;
}
console.log(i);
}
In this example, the loop will iterate five times, but when i
is equal to 9, the continue
The statement is executed, and the code block is skipped for that iteration. As a result, the value 9 is not printed to the console.
7) The for...in
Loop
The for...in
A statement is used to iterate over the properties of an object. It allows us to access each property of an object and perform a specific action. The syntax of the for…in The loop is as follows:
for (variable in object) {
// code to be executed
}
Let’s see an example to understand how the for...in
loop works:
const person = {
name: "GUVI",
age: 10,
city: "Chennai, Tamil Nadu"
};
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
In this example, the loop will iterate over each property of the person
object and print the key-value pairs to the console. The output will be:
name: GUVI
age: 10
city: Chennai, Tamil Nadu
Must Read: 4 Key Differences Between == and === Operators in JavaScript
8) The for...of
Loop
The for...of
statement is used to iterate over iterable objects, such as arrays, strings, and other iterable built-in objects. It provides a simpler and more concise syntax compared to the for
loop or the for...in
loop.
The syntax of the for...of
The loop is as follows:
for (variable of iterable) {
// code to be executed
}
Let’s look at an example to understand how the for...of
loop works:
const languages = ["javascript", "python", "html"];
for (let lang of languages) {
console.log(lang);
}
In this example, the loop will iterate over each element in the fruits
array and print the value to the console. The output will be:
javascript
python
html
Best Practices for Using Loops
When using loops in JavaScript, it’s important to follow best practices to ensure clean and efficient code. Here are some tips for using loops effectively:
- Use meaningful variable names: Choose variable names that accurately describe the purpose of the loop control variable. This makes the code more readable and understandable.
- Initialize variables outside the loop: If possible, initialize loop control variables outside the loop to avoid unnecessary reinitialization.
- Use the most appropriate loop for the task: Choose the loop that best fits the requirements of your code. For example, use a
for
loop when you know the number of iterations in advance, and use awhile
loop when the number of iterations is unknown. - Avoid infinite loops: Make sure your loops have a proper exit condition to prevent them from running indefinitely. Infinite loops can cause your program to crash or become unresponsive.
- Minimize code inside loops: Try to keep the code inside loops as concise as possible. Move any complex calculations or resource-intensive operations outside the loop if they don’t need to be repeated.
- Use
break
andcontinue
judiciously: Whilebreak
andcontinue
Statements can be useful, but excessive use can make the code harder to understand and maintain. Use them sparingly and only when necessary.
Also Read: 30 Best JavaScript Project Ideas For You [3 Bonus Portfolio Projects]
Common Mistakes to Avoid
When working with loops in JavaScript, it’s easy to make mistakes that can lead to bugs or inefficient code. Here are some common mistakes to avoid:
- Forgetting to update the loop control variable: Make sure to update the loop control variable inside the loop to avoid infinite loops or incorrect results.
- Using the wrong loop type: Choose the appropriate loop type for the task at hand. Using the wrong loop type can lead to unnecessary complexity or inefficiency.
- Modifying the iterable object inside a
for...of
loop: If you modify the iterable object inside afor...of
loop, it can cause unexpected behavior or errors. Consider creating a copy of the iterable object if you need to modify it. - Not using curly braces for single-line blocks: While it’s possible to omit curly braces for single-line blocks in loops, it can lead to ambiguity and make the code harder to read. Always use curly braces, even for single-line blocks.
- Not initializing loop control variables: Always initialize loop control variables before using them in a loop. Failure to do so can result in undefined behavior or errors.
Must Read: 7 Best Reasons to Learn JavaScript | 1 Bonus Point
If you want to learn more about JavaScript as well as web development and make a successful career out of it, then you must sign up for the Certified Full Stack Development Course, offered by GUVI, which gives you in-depth knowledge of the practical implementation of all frontend as well as backend development through various real-life FSD projects.
Concluding Thoughts…
Loops are a fundamental tool in JavaScript programming, allowing us to execute a block of code repeatedly.
In this comprehensive guide, we have explored the different types of loops in JavaScript, including the for
, do...while
, while
, for...in
, and for...of
loops. We have also discussed best practices for using loops and common mistakes to avoid.
By mastering all the loops in JavaScript and the art of looping, you can write more efficient and powerful JavaScript code.
Must Explore: Variables and Data Types in JavaScript: A Complete Guide
FAQs
JavaScript loops are control structures that allow for repetitive execution of code.
There are four types of loops in JavaScript: for loop, while loop, do-while loop, and for…of loop.
The for…of loop in JavaScript is used to iterate over iterable objects such as arrays, strings, maps, sets, etc. Dive into the article above to gain a better understanding of all loops in JavaScript.
An array in JavaScript is a special variable that can hold more than one value at a time.
Did you enjoy this article?