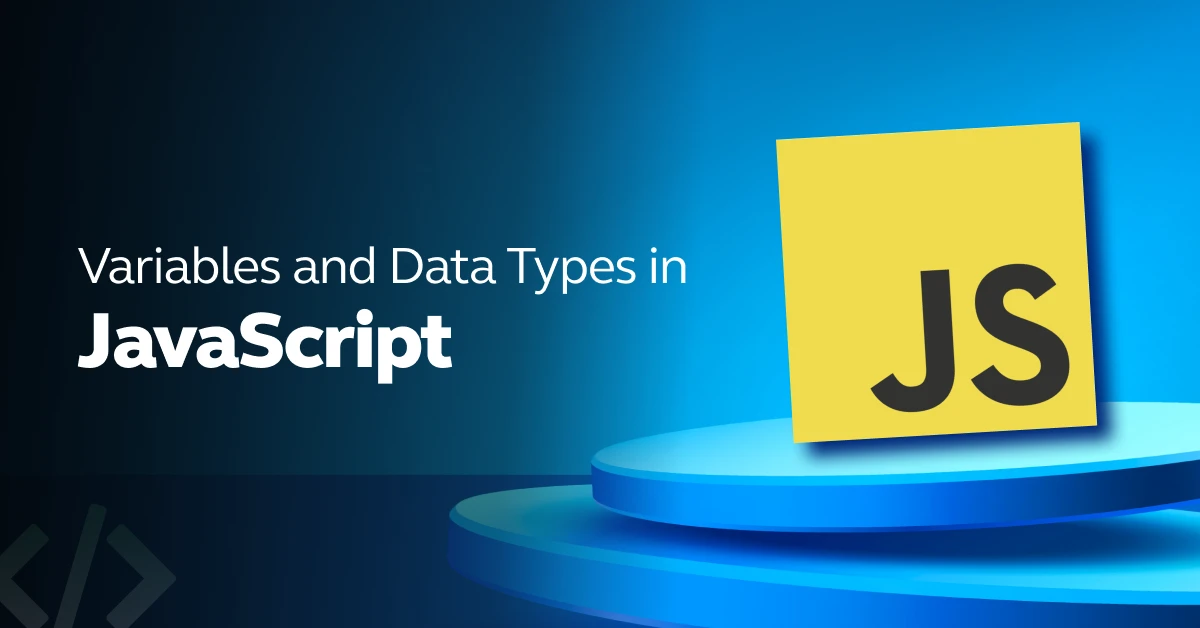
Variables and Data Types in JavaScript: A Complete Guide
Mar 19, 2024 3 Min Read 694 Views
(Last Updated)
JavaScript programming introduces you to the two fundamental concepts for developing excellent web experiences: variables and data types. Variables act as identifiers for storing, retrieving, and manipulating data throughout your code, offering a way to dynamically interact with and track information.
Data types, in parallel, define the kind of data you’re working with, from simple numbers and text to more complex structures, influencing how you can operate on the data stored in variables.
Grasping these concepts is important, laying the foundation for JavaScript development. This blog aims to clarify variables and data types simply, ensuring a solid foundation for your programming journey.
Table of contents
- What is JavaScript?
- JavaScript Data Types
- JavaScript Primitive Data Types
- JavaScript Non-primitive Data Types
- JavaScript Variables
- var
- let
- const
- When to Use Each
- Scope and Hoisting
- Conclusion
- FAQs
- How do let, var and const differ?
- Why are data types important in JavaScript?
- What's the difference between undefined and null?
What is JavaScript?
JavaScript is a powerful, versatile programming language primarily used for creating interactive and dynamic content on the web. It runs on the client’s browser, enabling web developers to implement complex features on web pages, such as real-time updates, interactive maps, animated 2D/3D graphics, scrolling video jukeboxes, and much more.
Also Read: The 5 Most User-Friendly Programming Languages
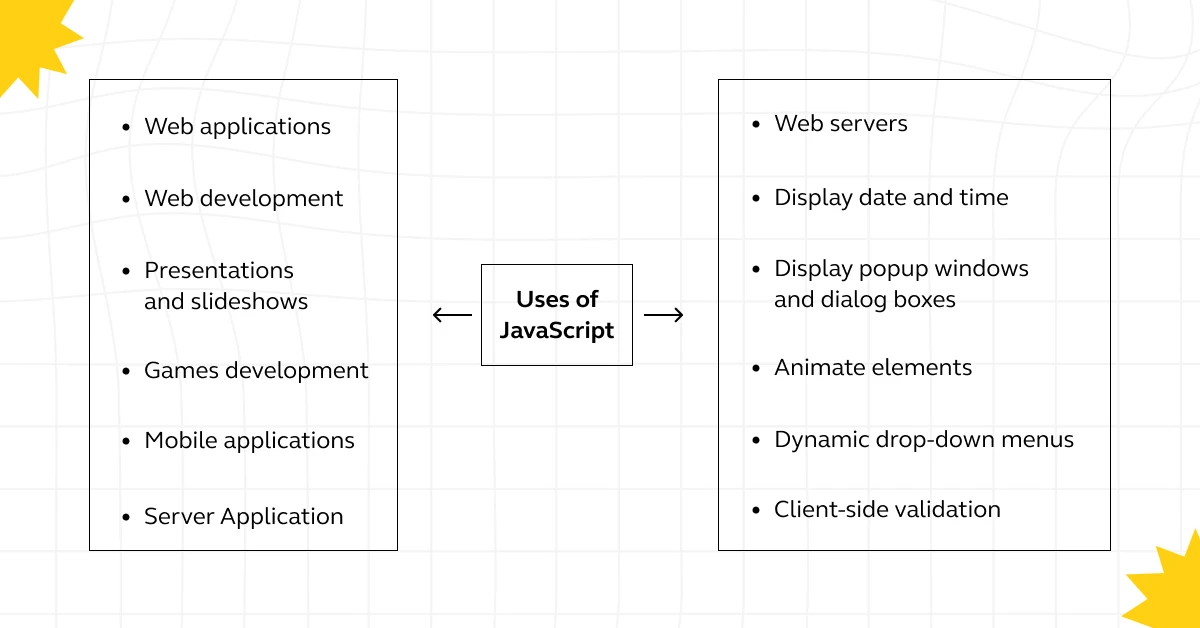
Having established a foundational understanding of what JavaScript is, let’s now learn the various data types that JavaScript supports, which serve as the backbone for data manipulation and functionality within the language.
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
JavaScript Data Types
Understanding data types in JavaScript is important for effective programming. Data types specify the kind of data you can work with and how you can operate on that data. They are foundational in helping programmers understand what operations are possible on a given piece of data.
Data types are important because they help enforce the correctness of your code. They define the operations you can perform with your data, the storage space it occupies, and how the computer interprets it. In JavaScript, knowing your data types means you can write more predictable and bug-free code.
Also Read: JavaScript Tools Every Developer Should Know
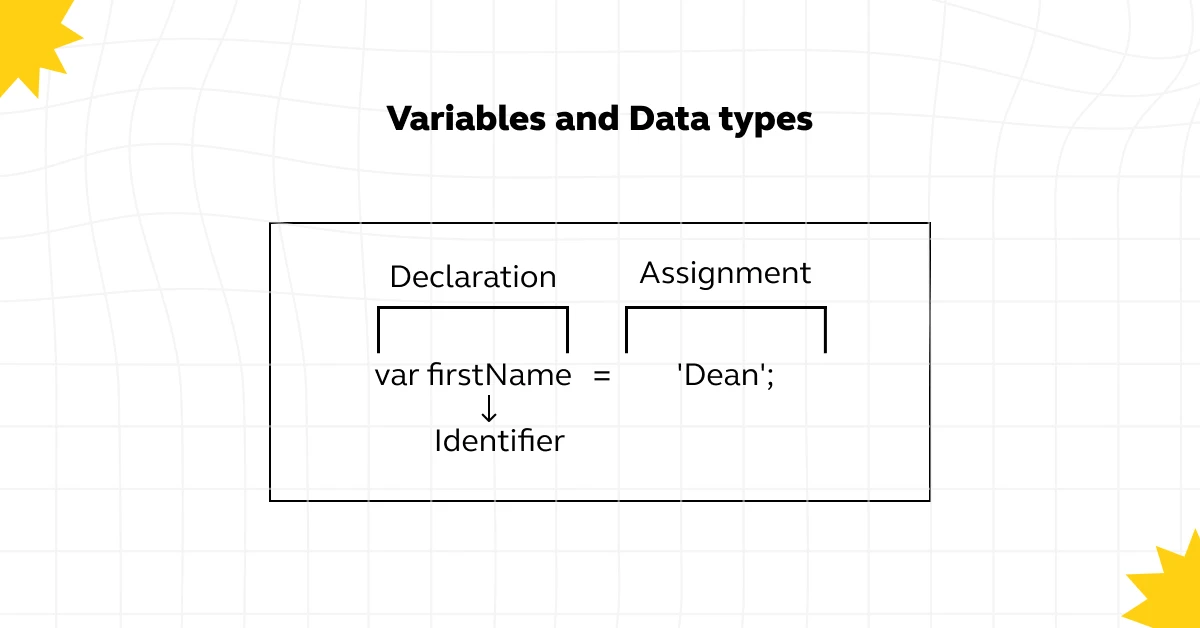
JavaScript Primitive Data Types
1. String: A string represents textual data. It’s a sequence of characters used to store and manipulate text.
Example:
let greeting = “Hello, World!”;
2. Number: The number data type handles both integers and floating-point numbers. It’s used for any numeric value.
Example:
let integerExample = 10;
let floatingPointExample = 10.5;
3. Boolean: A boolean represents a logical entity and can have two values: true and false. It’s often used in conditional statements.
Example:
let isJavaScriptFun = true;
4. Undefined: The undefined data type represents a variable that has been declared but not assigned a value.
Example:
let thisIsUndefined;
5. Null: Null is an assignment value that represents the intentional absence of any object value. Undefined means a variable has been declared but not defined.
Example:
let thisIsNull = null;
6. Symbol: Introduced in ECMAScript 2015, Symbol is a primitive data type that is unique and immutable. It’s used as the key for object properties.
Also Read: 42 JavaScript Questions Towards Better Interviews
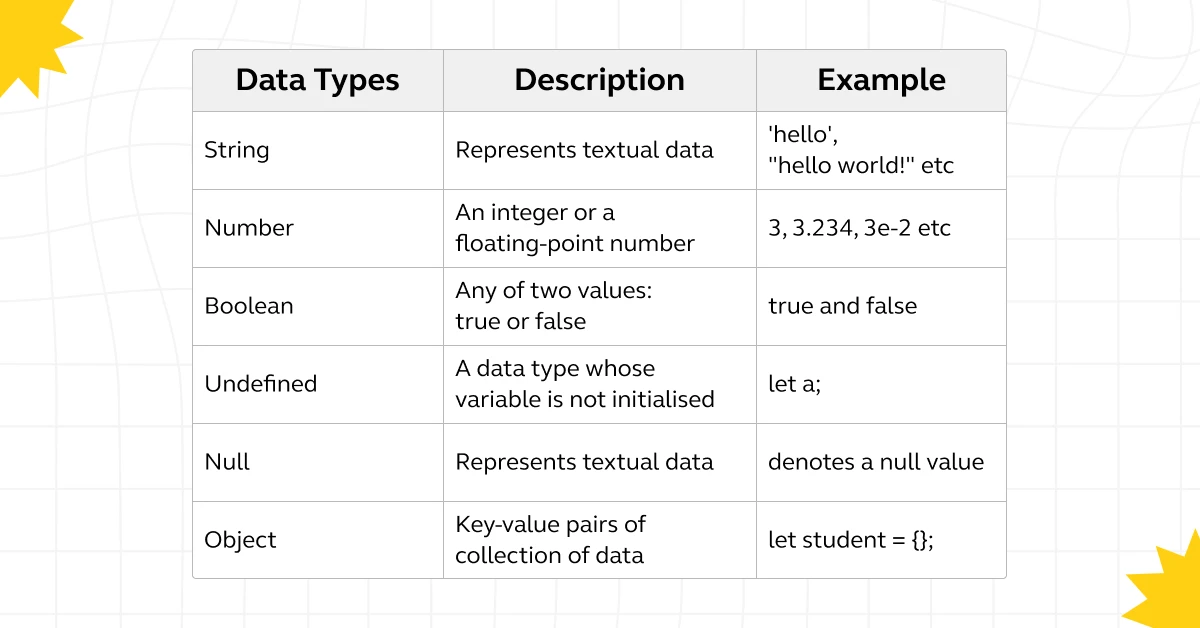
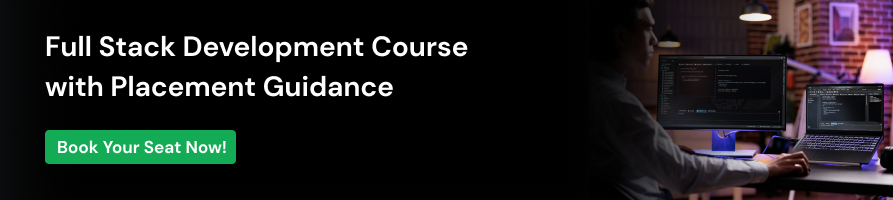
JavaScript Non-primitive Data Types
1. Object: Objects in JavaScript are collections of properties. They are significant for storing, manipulating, and representing data in a structured way.
Example
let person = {
firstName: “John”,
lastName: “Doe”,
age: 30
};
2. Array: Arrays are used to store multiple values in a single variable. They are objects that represent a list of items.
Example
let fruits = [“Apple”, “Banana”, “Cherry”];
Also Read: How to Render an Array of Objects in React? [in 3 easy steps]
3. Function: In JavaScript, functions are first-class objects, meaning they can be stored in variables, passed as arguments to other functions, and returned from functions.
Example
function greet(name) {
return `Hello, ${name}!`;
}
greet(“Alice”);
Also Read: useState() Hook in React for Beginners | React Hooks 2024
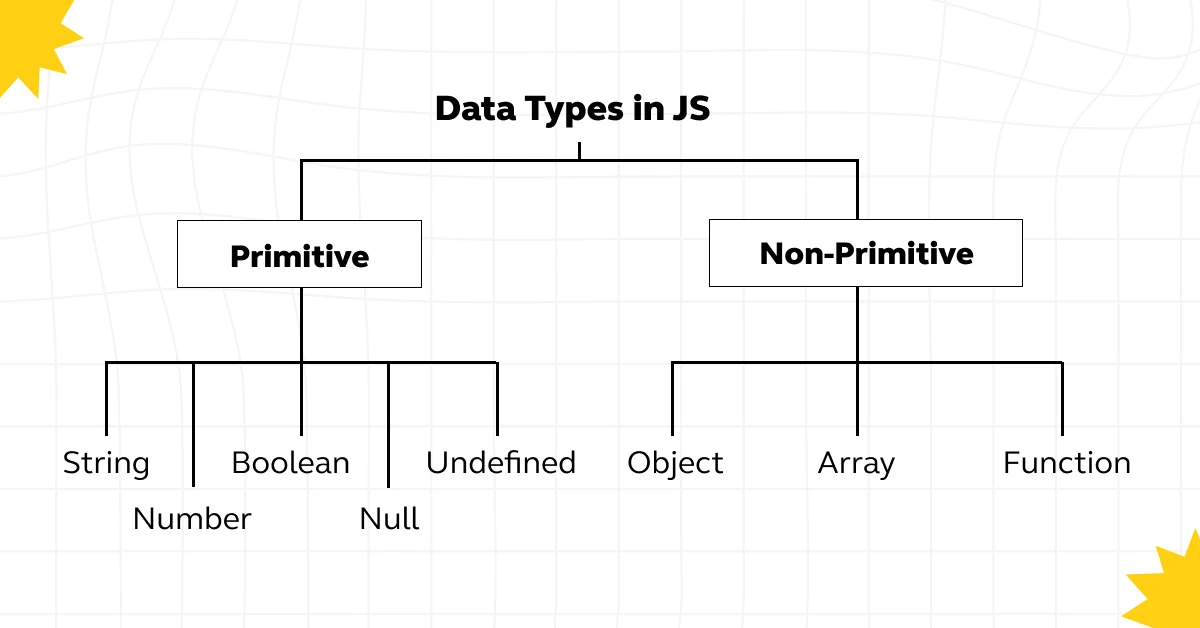
Each data type in JavaScript has its unique features and use cases. Understanding these will help you make the most of JavaScript’s flexibility and expressiveness in your programming projects.
Also Read: Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
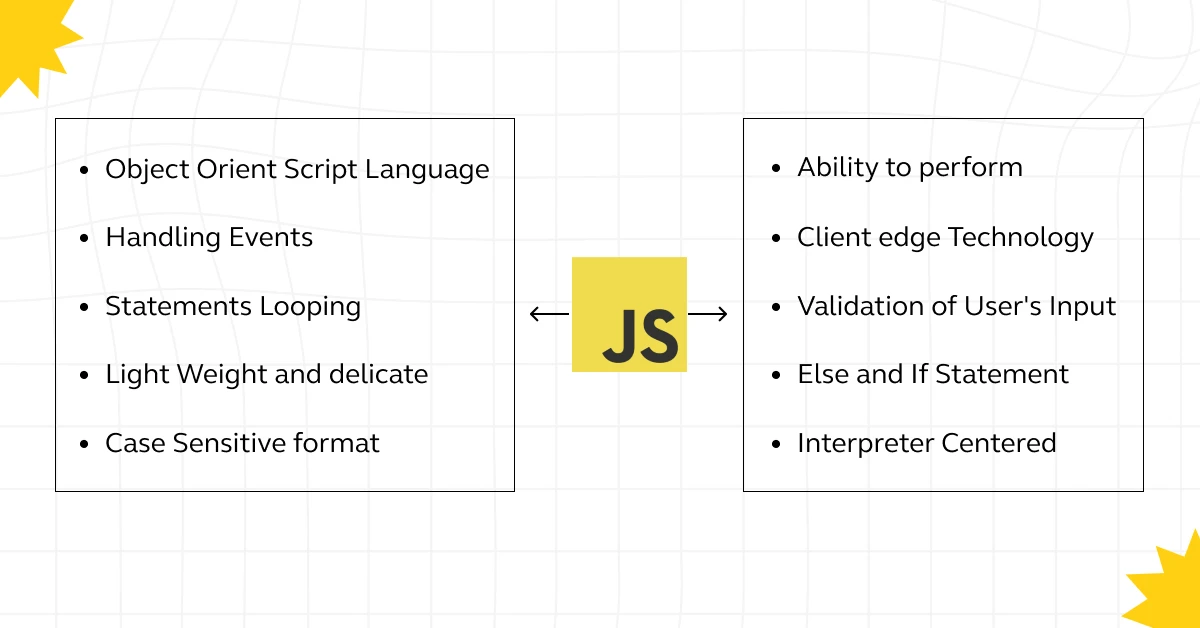
Having explored the foundational JavaScript data types, it’s time to turn our attention to another fundamental concept: JavaScript variables. These are the containers that store data values, allowing us to label and manipulate data efficiently in our programs. Let’s learn how variables work in JavaScript and how they can be used to hold the data types we’ve just discussed.
JavaScript Variables
In JavaScript, variables are the containers for storing data values. They let us label data with a descriptive name, so our programs can be understood more clearly by humans and manipulated more easily by the computer.
1. var
Usage: Historically used for declaring variables in JavaScript.
Scope: Function scope, meaning if it’s declared inside a function, it’s only available within that function.
Hoisting: Variables declared with var are hoisted to the top of their scope, initialized with undefined.
Example:
var greeting = “Hello, world!”;
console.log(greeting);
Output: “Hello, world!”
Also Read: “var functionName = function” VS “function functionName”
2. let
Usage: Introduced in ES6 (ECMAScript 2015) for declaring variables that can change later.
Scope: Block scope, which means it’s only available within the block (like loops or conditionals) it was declared in.
Hoisting: Variables declared with let are hoisted to the top of their block scope but are not initialized, leading to a “Temporal Dead Zone” until the declaration is reached.
Example:
let age = 25;
age = 26; // reassigning the value
console.log(age);
Output: 26
3. const
Usage: Also introduced in ES6 for declaring variables that are not meant to change after their initial assignment.
Scope: Block scope, similar to let.
Hoisting: Like let, const declarations are hoisted to the top of their block but are not initialized.
Example:
const PI = 3.14;
console.log(PI); // 3.14
// PI = 3.14159; // This will throw an error because const values cannot be reassigned
When to Use Each
- Use var if you need a variable with function scope or if you’re working in an environment that doesn’t support ES6.
- Use let when you need a block-scoped variable that you plan to reassign.
- Use const for block-scoped variables that should not be reassigned after their initial declaration.
Scope and Hoisting
- Scope determines where variables and functions are accessible within your code. Variables declared with var have function scope, while let and const have block scope.
- Hoisting is JavaScript’s default behavior of moving declarations to the top of their scope before code execution. While var variables are hoisted and initialized with undefined, let and const are hoisted but not initialized, meaning they cannot be accessed until their declaration is evaluated at runtime.
Are you ready to transform your career and become a tech industry pro? Join GUVI’s Full Stack Development Course and master the skills needed to excel as a developer. Enroll now!
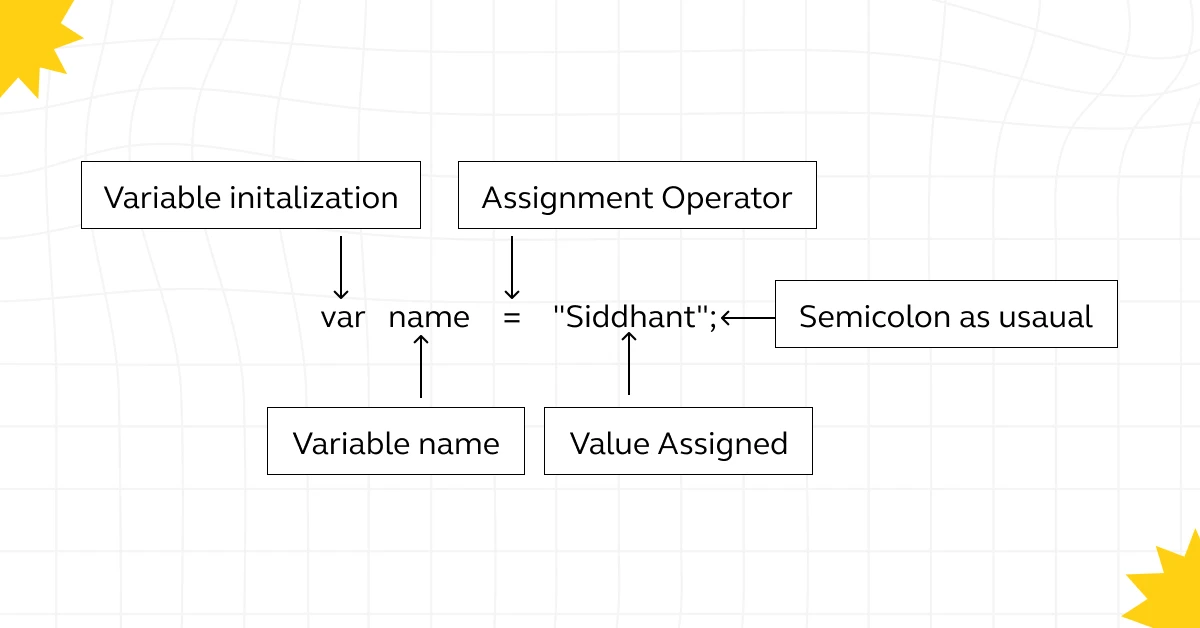
Conclusion
Remember, every great developer started somewhere, and the willingness to experiment, make mistakes, and learn from them is what leads to mastery. So, start coding, and watch as the concepts of variables and data types become second nature in your JavaScript adventures.
Must Read: 7 Best Reasons to Learn JavaScript | 1 Bonus Point
FAQs
How do let, var and const differ?
var: Function scope, can be redeclared and updated.
let: Block scope, can’t be redeclared in the same scope, can be updated.
const: Block scope, can’t be redeclared or updated, must be initialized.
Why are data types important in JavaScript?
They determine how data can be used and manipulated, affecting operations and preventing errors. Understanding them helps in organizing and managing data effectively.
Did you enjoy this article?