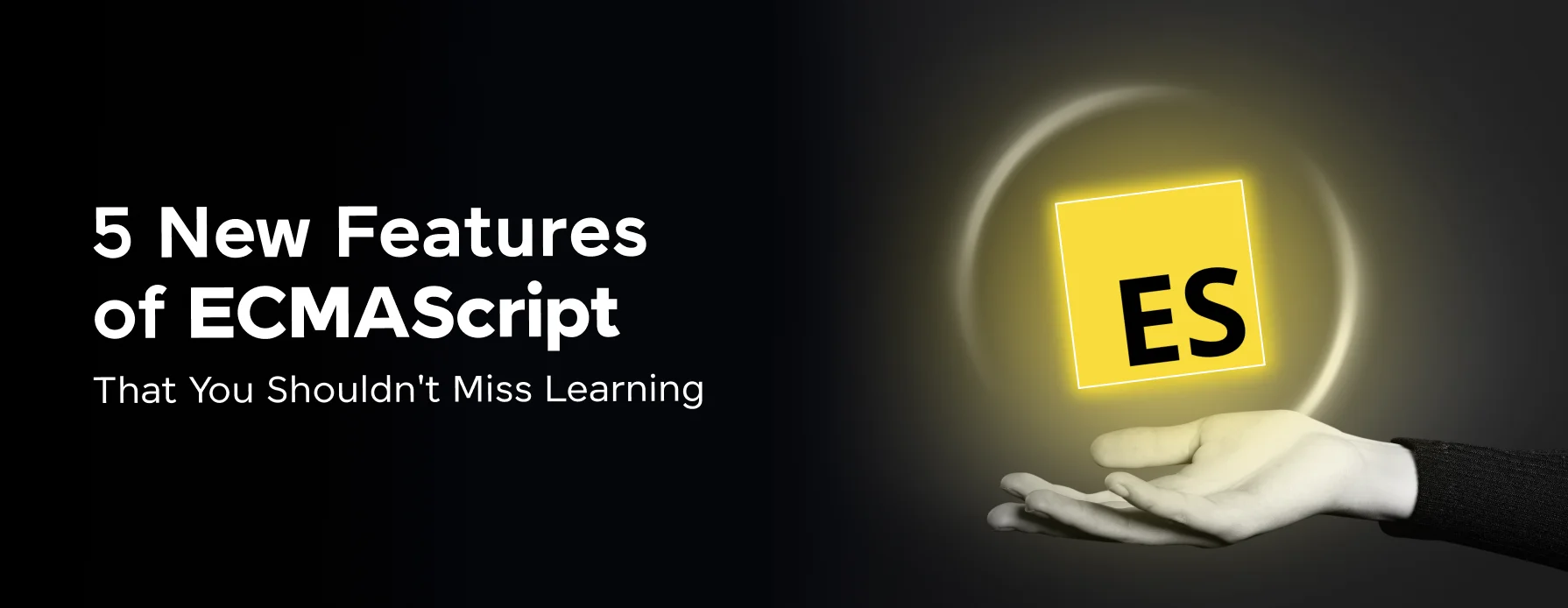
5 New Features of ECMAScript That You Shouldn’t Miss Learning
Apr 30, 2024 6 Min Read 229 Views
(Last Updated)
Technology is evolving rapidly and we can see a lot of updates regularly. As a developer, you need to know about all these features and keep yourself updated.
One of those important technology for full-stack development is ECMAScript, a version of JavaScript. Compared to its earlier version, there have been a lot of new features of ECMAScript.
If you are not aware of those, no need to worry as this article covers all the new features of ECMAScript and their benefits and use cases.
So, sit back, relax, and read it till the end!
Table of contents
- What is ECMAScript?
- Features of ECMAScript: What's New about it?
- Atomics and Synchronization: Simplifying Complex Concepts
- Numeric Separators in JavaScript
- Promise.any(): Simplifying Multiple Asynchronous Operations
- String.prototype.replaceAll(): Making Text Manipulation Easier
- Logical Assignment Operators in JavaScript
- Conclusion
- FAQs
- How does Atomics.waitAsync() improve JavaScript concurrency?
- What are some practical uses of Promise.any() in web development?
- Can Atomics.waitAsync() be used with any type of shared memory operation?
- Are there any performance considerations with using String.prototype.replaceAll() for large texts?
What is ECMAScript?
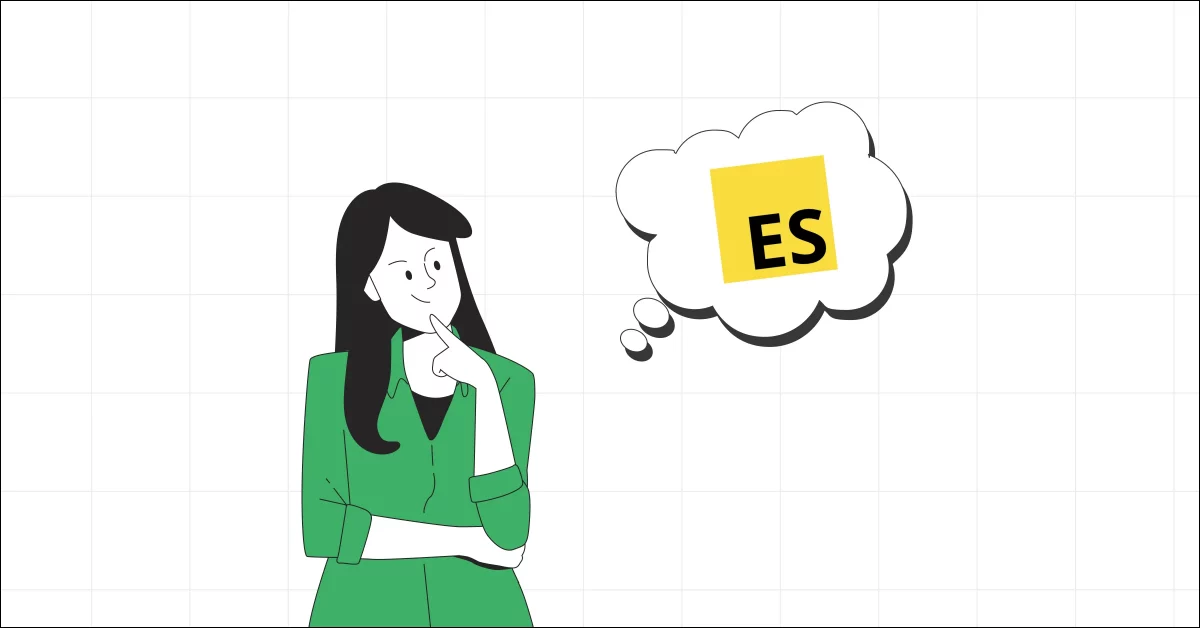
Before we rush into the features of ECMAScript, you need to understand what it is. ECMAScript is essentially a standardized version of JavaScript, which is one of the most widely used programming languages in the world, particularly for creating interactive websites.
Think of ECMAScript as the rulebook that defines how JavaScript should work, ensuring that it behaves the same way across different web browsers and platforms.
Developers and browser manufacturers refer to this standard to make sure that their scripts and browsers are compatible with each other.
Over the years, updates to ECMAScript have been released to introduce new features of ECMAScript and improve the language, making it more powerful and easier to use for developers around the globe.
Let us now understand about these features of ECMAScript.
Learn More: 7 Best JavaScript Practices Every Developer Must Follow
Features of ECMAScript: What’s New about it?
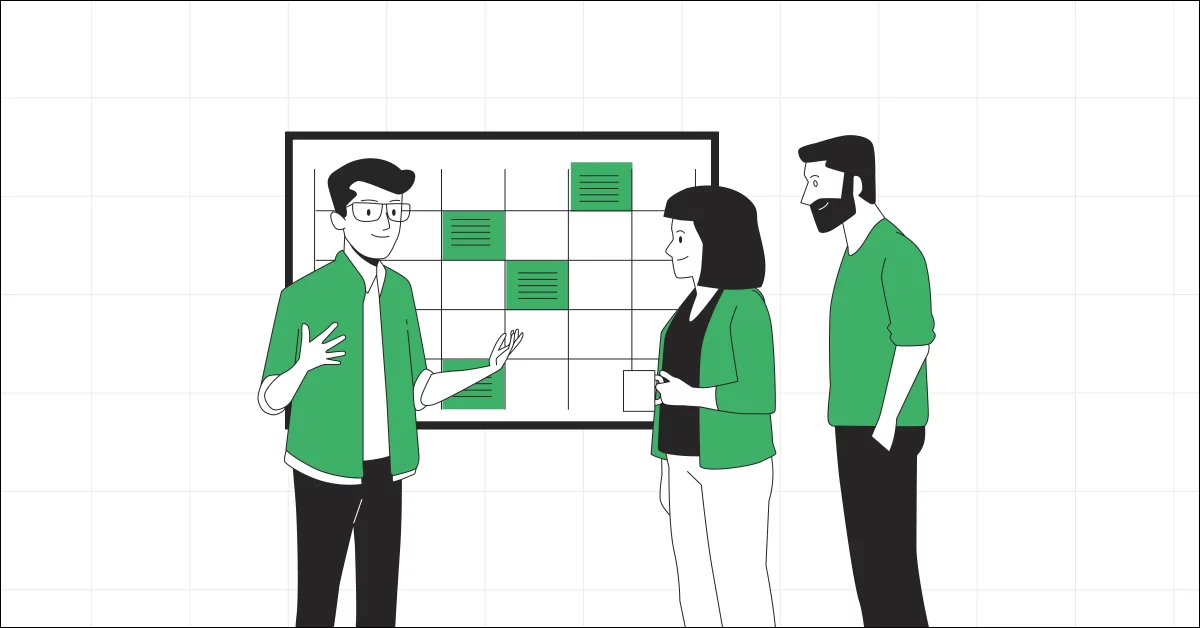
Now you must have a basic understanding of what ECMAScript is, but that alone is insufficient. You also need to know the fundamentals of full-stack development and JavaScript.
If you do not know, consider enrolling in a certified online full-stack development course offered by a recognized institution that lets you gain strong fundamental knowledge of full-stack development.
You can also learn JavaScript in a self-paced manner online. So, do refer to those and know the basics before moving further on the features of ECMAScript.
Let us now see some of the new features of ECMAScript:
1. Atomics and Synchronization: Simplifying Complex Concepts
Introduction to Atomics
The first of the many features of ECMAScript that we are going to see is Atomics. When you’re dealing with JavaScript, especially in web applications that perform multiple tasks simultaneously, ensuring that these tasks don’t step on each other‘s toes is crucial.
This is where “atomics” comes into play. Atomics provides a way to manage memory access across different threads, which is just a fancy way of saying they help coordinate the work being done by different parts of your program so that they don’t conflict with each other.
Why Do We Need Atomics?
Imagine you and your friend are both editing a shared document. If you’re not careful, one could overwrite the other’s changes.
In programming, especially in environments where tasks run concurrently (at the same time), similar issues can occur. Here, atomics acts as the necessary communication and synchronization tool to manage these operations safely.
Atomics ensures that certain operations with shared memory—like reading, writing, or updating values—are done predictably and safely. This helps prevent various programming bugs that could arise when threads interact in uncontrolled ways.
Also Read: Best Programming Languages For The Future!
How Atomics Work
At the core, atomics is one of the primary features of ECMAScript that provides several key functionalities:
- Lock-free synchronization: Unlike traditional locking mechanisms which can cause other threads to wait and thus slow down execution, atomics provides ways to manage memory without these delays. They ensure that when one thread updates a shared variable, no other thread can simultaneously modify it, preventing conflicts and ensuring data integrity.
- Atomic operations: These are operations that are completely performed without interference from other threads. For example, incrementing a shared counter that tracks website visitors. With atomics, you can increment the counter safely, knowing that no visitor count is missed or duplicated, even if many people visit the site at the exact same time.
- Waiting and waking: Threads can sometimes need to wait for a certain condition before they continue their task. Atomics provides methods like Atomics.wait() and Atomics.notify() which helps in these situations. For example, a thread can wait for a signal that new data is available before continuing its processing.
The Addition of Atomics.waitAsync()
A recent exciting development in the features of ECMAScript is Atomics.waitAsync()
. This function is an enhancement over Atomics.wait()
because it allows a thread to wait without stopping its execution entirely.
You can think of it like setting a timer when cooking, you can continue with other tasks around the kitchen rather than standing and watching the oven.
This non-blocking feature allows for more efficient management of tasks, particularly in web environments where stopping or delaying operations can affect user experience.
Also Explore: Top Technologies to Learn for a JavaScript Backend Developer
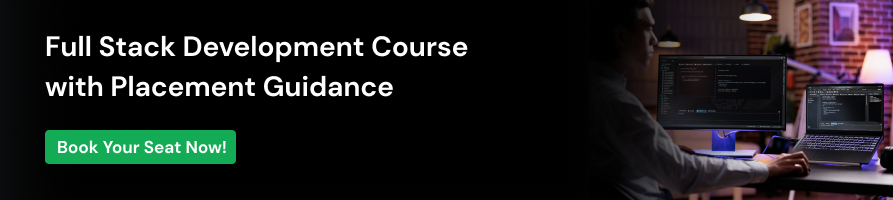
2. Numeric Separators in JavaScript
What Are Numeric Separators?
When you’re dealing with numbers, especially large ones, it can be really easy to lose track of how big the number is just by looking at it. To solve this, in the features of ECMAScript, we have Numeric Separators
Numeric separators help you, the programmer, visually separate numbers to make them easier to read and understand.
Why Use Numeric Separators?
Imagine you’re looking at a bank statement, and you see a number like 100000000. It might take you a few seconds to figure out if that’s ten million or a hundred million. Now, if the number were written as 100,000,000, you’d recognize it much faster.
Numeric separators in the features of ECMAScript serve the same purpose—they make numbers easier to read by letting you add underscores as thousands of separators.
Also Read: All About Loops in JavaScript: A Comprehensive Guide
How Do Numeric Separators Work?
In the features of ECMAScript, as in many other programming languages, you can now write numbers with underscores to separate digits into groups. This doesn’t change the value of the number; it just makes it easier to read. For example:
- A million can be written as
1_000_000
. - A billion as
1_000_000_000
. - Even more complex numbers, like a credit card number, can be broken down into their standard groupings, like
1234_5678_9012_3456
.
This is one prominent feature of ECMAScript that is particularly useful in finance and data analysis, where you often work with large numbers and precision is key. By using numeric separators, you can reduce the chance of misreading a number and making costly mistakes.
Explore: Best JavaScript Roadmap Beginners Should Follow
3. Promise.any()
: Simplifying Multiple Asynchronous Operations
What is Promise.any()
?
When you’re working with the features of ECMAScript, particularly in web development, you often need to deal with operations that don’t complete instantly—these are called asynchronous operations.
For example, fetching data from a server or reading files. Promises are tools in JavaScript that help manage these asynchronous operations. Promise.any()
is a function that works with these promises in a very specific and useful way.
Why Use Promise.any()
?
Imagine you’re trying to get tickets for a concert online. You open several tabs to increase your chances because the site might crash due to high traffic. The moment you secure a ticket in one tab, you don’t care about the other tabs anymore.
Promise.any()
, in the features of ECMAScript works similarly in programming. It takes multiple promises and resolves as soon as the first one succeeds. This is very useful when you want a result quickly and from multiple sources of data.
Also Read: 4 Key Differences Between == and === Operators in JavaScript
How Does Promise.any()
Work?
Promise.any()
accepts an array (a list) of promises and gives you back a single promise that is fulfilled as soon as one of the promises in the array is fulfilled.
It doesn’t matter which one—it will return the value from the first promise that succeeds. If all the promises fail, then Promise.any()
will reject, providing a list of errors from all the failed promises, which can be useful for understanding why things didn’t work out.
Benefits of Promise.any()
In the features of ECMAScript, Promise.any()
provides a lot of benefits:
- Efficiency: It helps your applications run more efficiently as it reduces waiting time, using the quickest response available.
- Fallbacks: It’s perfect for situations where you have multiple sources or backups for data or services. You can attempt several options and settle on the first one that works, which is great for reliability.
- Simplicity: It simplifies error handling in scenarios where multiple tasks are involved, focusing only on the failure scenario if every single option fails.
Also Know JavaScript Modules: A Comprehensive Guide [2024]
4. String.prototype.replaceAll()
: Making Text Manipulation Easier
What is String.prototype.replaceAll()
?
Have you ever had a moment when you needed to change a word or a phrase in a long piece of text or in several places within a document? Maybe you spotted a typo in an email or wanted to update a common phrase in a report.
In JavaScript, dealing with such text manipulations can be tricky, especially if you need to replace all instances of a word or phrase. This is why, in the arsenal of the features of ECMAScript, we have String.prototype.replaceAll()
, which makes it much easier to handle these changes.
Why Use replaceAll()
?
Before replaceAll()
, JavaScript offered replace()
, which could also change the text but had a limitation: it only replaced the first occurrence of the text you were targeting unless you used a regular expression with a global flag (a bit more complex to set up).
replaceAll()
simplifies this by replacing every instance of the specified text without needing any complex syntax.
How Does replaceAll()
Work?
replaceAll()
is straightforward: you tell it what text to find and what text to replace it with, and it takes care of the rest across the entire string. Here’s how you can use it:
let text = "Hello World! World is beautiful.";
let newText = text.replaceAll("World", "Earth");
console.log(newText); // Output: "Hello Earth! Earth is beautiful."
In this example, every instance of “World” is replaced with “Earth”, making the changes consistent throughout the string. This is why, replaceAll()
is considered to be one of the important time-reducing features of ECMAScript.
Explore More: JavaScript Tools Every Developer Should Know
5. Logical Assignment Operators in JavaScript
Introduction to Logical Assignment Operators
When you’re working with programming languages like JavaScript, you often find yourself needing to update the value of a variable based on certain conditions.
Typically, this involves writing a few lines of code to check a condition and then assign a value. For this, the features of ECMAScript have introduced a set of tools called logical assignment operators that simplify this process.
These operators allow you to perform an assignment and a logical operation (like checking if a value exists) at the same time, making your code more concise and easier to read.
What Are Logical Assignment Operators?
Logical assignment operators combine the traditional assignment operators (=
) with logical operators. The main logical operators used in this context are:
&&
(Logical AND)||
(Logical OR)??
(Nullish coalescing)
These operators help you assign values to variables based on some logical condition directly within the assignment itself.
Also Read: Arrays in JavaScript: A Comprehensive Guide
How Do Logical Assignment Operators Work?
Let’s break down each operator with an example to see how you can use them:
Logical AND Assignment (&&=
):
let x = 5;
let condition = false;
x &&= 10;
console.log(x); // Output will be 5 because condition is false
In this case, x
will only be updated to 10
if x
is true(a value that is not false
, 0
, ""
, null
, undefined
, or NaN
). Since x
is initially 5
(which is true), but the condition is false, it does not update x
.
Logical OR Assignment (||=
):
let message = '';
message ||= 'Default message';
console.log(message); // Output will be 'Default message'
Here, message
will be assigned a ‘Default message’ if message
is false (a value that is false
, 0
, ""
, null
, undefined
, or NaN
). Since message
is an empty string (false), it gets updated.
Nullish Coalescing Assignment (??=
):
let username = null;
username ??= 'Guest';
console.log(username); // Output will be 'Guest'
This operator is similar to the logical OR but only considers null
or undefined
as reasons to assign the new value. Since username
is null
, it gets updated to ‘Guest’.
Must Explore: Constructors in JavaScript: 6 Uses Every Top Programmer Must Know
Practical Applications
One of the prominent features of ECMAScript is Logical assignment operators and it can be incredibly useful in various programming scenarios:
- Setting default values: As shown above, they are great for assigning default values to variables that might not be initialized.
- Conditional updates: Useful in situations where a variable should only be updated based on a condition without writing additional if statements.
- Simplifying code: They help reduce the amount of boilerplate code, making your scripts shorter and more readable.
These are some of the new and trending features of ECMAScript that you as a developer need to keep an eye on and with these features of ECMAScript, you can code easily and effectively.
If you want to learn more about the features of ECMAScript, then consider enrolling in
GUVI’s Certified Full-stack Development Career Program not only gives you theoretical knowledge but also practical knowledge with the help of real-world projects.
Also Read: Functions in JavaScript: Important Things To Know
Conclusion
In conclusion, the latest features of ECMAScript continue to enhance JavaScript’s capabilities, making coding more efficient and developer-friendly.
Features like Atomics and Synchronization improve how code executes in multi-threaded environments, while Numeric Separators enhance code readability. Promise.any()
offers efficient handling of multiple asynchronous operations, and String.prototype.replaceAll()
simplifies text manipulation. Logical Assignment Operators further streamline code complexity, allowing for more readable and maintainable scripts.
These features of ECMAScript collectively contribute to a robust development experience, ensuring JavaScript remains a top choice for programmers worldwide.
Must Explore: 42 JavaScript Questions Towards Better Interviews
FAQs
1. How does Atomics.waitAsync() improve JavaScript concurrency?
Atomics.waitAsync()
provides a way to wait for changes in shared memory without blocking the thread, thus improving the performance of web applications using concurrency.
2. What are some practical uses of Promise.any() in web development?
It is useful for fetching resources from multiple sources where the fastest response is needed, such as loading resources from CDN fallbacks.
3. Can Atomics.waitAsync() be used with any type of shared memory operation?
It is specifically designed for use with shared memory operations that can benefit from non-blocking synchronization mechanisms.
Did you enjoy this article?