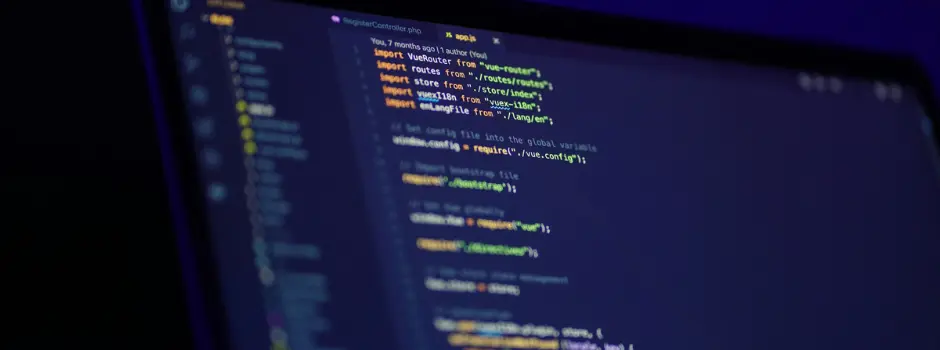
Constructors in JavaScript: 6 Uses Every Top Programmer Must Know
Mar 19, 2024 4 Min Read 627 Views
(Last Updated)
In the world of JavaScript programming, constructors play a crucial role. They are special functions that are used to create and initialize objects.
When an object is created using the new keyword, the constructor gets called, allowing you to set values for the object’s properties and perform any necessary initialization tasks.
In this comprehensive guide, we will explore the concept of constructors in JavaScript, understand how they work, and examine various examples and use cases.
Table of contents
- What is a Constructor in JavaScript?
- How Constructors in JavaScript Work?
- How to use Constructors in JavaScript:
- 1) Using the "this" Keyword in Constructors
- 2) Creating Multiple Objects with Constructors
- 3) Constructor with Parameters
- 4) Constructor vs Object Literal
- 5) Understanding Object Prototypes
- 6) Built-in Constructors in JavaScript
- Takeaways...
- FAQs
- What are constructors in JavaScript?
- What is the difference between a constructor and a class in JavaScript?
- What are constructors in JavaScript used for?
- Can we call multiple constructors?
What is a Constructor in JavaScript?
A constructor is a special function in JavaScript that is used to create and initialize objects. It is typically defined within a class or an object blueprint. When a new object is created using the new
keyword and a constructor function, the constructor gets invoked, and a new instance of the object is created.
The purpose of a constructor is to set the initial state of the object and define its properties and methods. It allows you to specify the values of the object’s properties when it is created, making it easier to work with and manipulate the object later on.
Must Read: Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
How Constructors in JavaScript Work?
When a constructor is called in JavaScript, several operations take place:
- A new empty object is created.
- The
this
keyword starts referring to the newly created object, making it the current instance object. - The properties and methods defined within the constructor are attached to the object.
- The new object is returned as the result of the constructor call.
This sequence of operations ensures that a new object with the desired properties and behaviors is created and ready to be used.
Also Read: All About Loops in JavaScript: A Comprehensive Guide
Given below is the code to initialize two user instances as shown in the image above:
function UserG(start, end) {
this.firstName = start
this.lastName = end
}
var user1 = new UserG("GUVI", "GEEK")
console.log(user1)
var user2 = new UserG("Jaishree", "Tomar")
console.log(user2)
How to use Constructors in JavaScript:
Let’s explore some examples of constructors in JavaScript to understand how they are used.
1) Using the “this” Keyword in Constructors
In a constructor, the this
keyword refers to the newly created object. You can use the this
keyword to define and assign values to the object’s properties. Here’s an example:
// Constructor
function Carss(making, model, year) {
this.make = making;
this.model = model;
this.year = year;
}
// Create a new car object
var myCar = new Carss('Tesla', 'CyberTruck', 2024);
console.log(myCar.make); // Output: Tesla
console.log(myCar.model); // Output: CyberTruck
console.log(myCar.year); // Output: 2024
In the example above, the Car
constructor takes three parameters: make
, model
, and year
. The this
keyword is used to assign the parameter values to the corresponding properties of the newly created myCar
object.
By using the this
keyword, you ensure that the values are specific to each instance of the Car
object. If you were to create multiple car objects using the same constructor, each object would have its own unique set of property values.
Must Read: 4 Key Differences Between == and === Operators in JavaScript
2) Creating Multiple Objects with Constructors
Constructors are not limited to creating just a single object. You can create multiple objects using the same constructor. Each object will have its own set of properties and values. Here’s an example:
// Constructor
function CircleGUVI(radius) {
this.radius = radius;
this.getArea = function() {
return Math.PI * Math.pow(this.radius, 2);
};
}
// Create two circle objects
var circle1 = new CircleGUVI(10);
var circle2 = new CircleGUVI(15);
console.log(circle1.getArea()); // Output: 314.1592653589793
console.log(circle2.getArea()); // Output: 706.8583470577034
In the above example, the Circle
constructor takes a radius
parameter and defines a getArea
method. The getArea
method calculates and returns the area of the circle based on its radius.
By creating multiple circle objects using the new
keyword and the Circle
constructor, we can compute and output the areas of different circles. Each object has its own radius
property and getArea
method, ensuring that the calculations are specific to each circle.
Also Explore: Arrays in JavaScript: A Comprehensive Guide
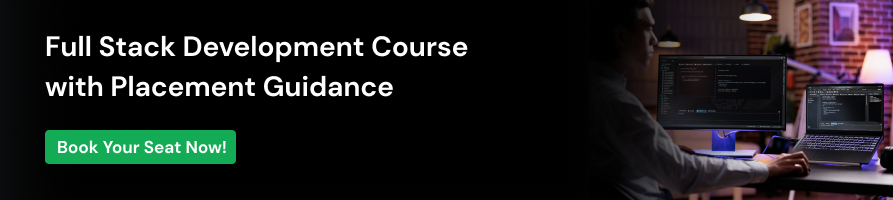
3) Constructor with Parameters
Constructors in JavaScript can accept parameters, allowing you to pass values during object creation. This enables you to set different property values for each object based on the provided arguments.
Let’s consider an example of a Rectangle
constructor:
// Constructor
function RectangleGUVI(width, height) {
this.width = width;
this.height = height;
this.getArea = function() {
return this.width * this.height;
};
}
// Create two rectangle objects
var rectangle1 = new RectangleGUVI(20, 10);
var rectangle2 = new RectangleGUVI(27, 12);
console.log(rectangle1.getArea()); // Output: 200
console.log(rectangle2.getArea()); // Output: 324
In the above example, the Rectangle
constructor takes two parameters: width
and height
. The constructor assigns the respective parameter values to the width
and height
properties of the newly created rectangle objects.
By creating multiple rectangles using the new
keyword and the Rectangle
constructor, we can calculate and output the areas of different rectangles. Each object has its own width
and height
properties, ensuring that the calculations are specific to each rectangle.
Must Explore: What is jQuery? A Key Concept You Should Know
4) Constructor vs Object Literal
In JavaScript, you have multiple ways to create objects. While constructors are useful for creating multiple objects with similar properties and behaviors, object literals are handy for creating single objects with specific properties.
Let’s compare the two approaches using an example:
// Using a Constructor
function PersonatGUVI(name, age) {
this.name = name;
this.age = age;
}
var person1 = new PersonatGUVI('GUVI', 10);
var person2 = new PersonatGUVI('Jaishree', 22);
// Using an Object Literal
var person3 = {
name: 'Saakshi',
age: 22
};
In the example above, we have created three objects: person1
and person2
using the Person
constructor, and person3
using an object literal.
The objects created with the constructor have similar properties and behaviors, allowing us to create multiple instances easily. On the other hand, the object created with the object literal approach is a single, standalone object with specific properties.
When deciding whether to use a constructor or an object literal, consider the specific needs of your program. Constructors are ideal when you need to create multiple objects with shared properties and behaviors. Object literals are suitable for creating single, independent objects with unique properties.
Also Explore: HTML Tutorial: A Comprehensive Guide for Web Development
5) Understanding Object Prototypes
In JavaScript, object prototypes play a significant role in defining shared properties and methods for objects created with a constructor. Prototypes allow objects to inherit properties and methods from a common prototype object.
Let’s consider an example of a Person
constructor with a shared method defined in the prototype:
// Constructor
function PersonGUVI(name, age) {
this.name = name;
this.age = age;
}
// Shared method defined in the prototype
Person.prototype.greet = function() {
console.log('Hello, my name is ' + this.name);
};
// Create a new person object
var person = new PersonGUVI('GUVI', 10);
person.greet(); // Output: Hello, my name is GUVI
In the above example, the Person
constructor creates a person
object with name
and age
properties. The shared greet
method is defined in the Person.prototype
, allowing all instances of Person
objects to access and invoke the method.
By defining methods in the prototype, you ensure that they are shared across all instances of the objects created with the constructor. This improves memory efficiency and allows you to add or modify shared behaviors easily.
6) Built-in Constructors in JavaScript
JavaScript provides several built-in constructors that you can use to create objects with specific types and functionalities. However, it is generally recommended to use primitive data types where possible for better performance. Here are some examples of built-in constructors:
var stringObject = new String('Hello');
var numberObject = new Number(24);
var booleanObject = new Boolean(true);
var arrayObject = new Array(1, 2, 3);
var dateObject = new Date();
In the above examples, we create objects using the built-in constructors String
, Number
, Boolean
, Array
, and Date
. These constructors allow us to work with string, number, boolean, array, and date objects, respectively.
While using these constructors is possible, it is generally recommended to use primitive data types directly, as they offer better performance and are simpler to work with.
If you want to learn more about programming with JavaScript and make a successful career out of it, then you must sign up for the Certified Full Stack Development Course, offered by GUVI, which gives you in-depth knowledge of the practical implementation of all frontend as well as backend development through various real-life FSD projects.
Takeaways…
Constructors in JavaScript are essential for creating and initializing objects. They provide a way to define object blueprints and set initial property values.
By using constructors, you can create multiple objects with shared properties and behaviors, pass parameters during object creation, and leverage prototypes for shared methods.
Understanding constructors enables you to build more robust and flexible applications. Whether you’re creating simple objects or complex data structures, constructors play a vital role in JavaScript programming.
Remember to use constructors wisely, considering the specific needs of your program.
Must Read: Variables and Data Types in JavaScript: A Complete Guide
FAQs
What are constructors in JavaScript?
Constructors in JavaScript are special functions used for initializing objects created with a class or constructor function.
What is the difference between a constructor and a class in JavaScript?
The main difference between a constructor and a class in JavaScript is that a constructor is a function used to create and initialize objects, while a class is a blueprint for creating objects.
What are constructors in JavaScript used for?
Constructors in JavaScript are used to set initial values, assign properties, and perform other setup tasks when creating objects.
Did you enjoy this article?