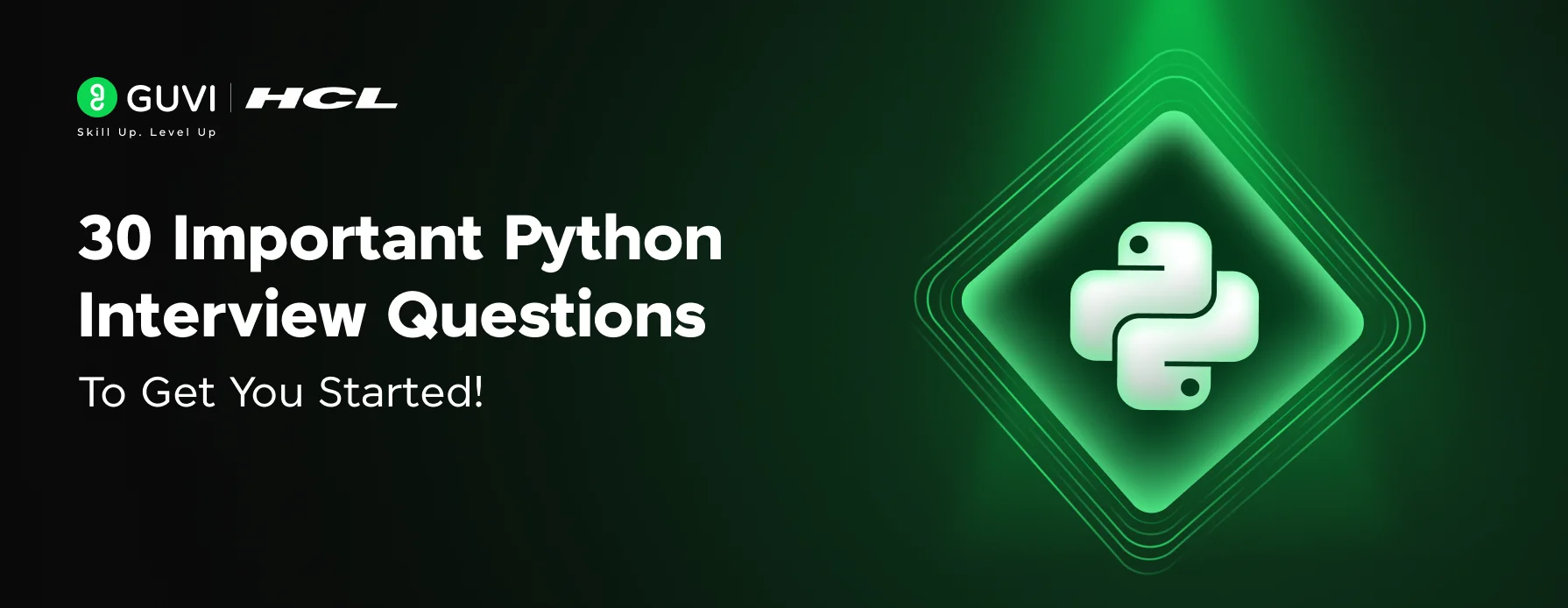
30 Important Python Interview Questions To Get You Started!
Jul 08, 2025 7 Min Read 16799 Views
(Last Updated)
Are you preparing for a Python interview and wondering which questions actually matter?
Python is a versatile language used in data science, web development, AI, and more. Interviewers often test not just your syntax knowledge but also your problem-solving approach.
Most of the top applications use the Python programming language for a majority of their tasks. For Data Science, Full Stack Web Development, Cyber Security, Automation Testing, UI/UX Development, or any field that you choose today, Python is a language that you can not skip.
In this article, we’ve handpicked the Top 30 Python Interview Questions, segmented into Beginner, Intermediate, and Advanced levels. From simple definitions to coding exercises and real-world logic, this guide is designed to help you build confidence and clarity, one question at a time.
Table of contents
- Beginner-Level Python Interview Questions
- What is Python?
- What are the key features of Python?
- What is the difference between a list and a tuple?
- How do you write a function in Python?
- What are Python's built-in data types?
- How do you check if an element exists in a list?
- What is the difference between is and ==?
- Write a program to find the largest of three numbers.
- How do you handle user input in Python?
- What is indentation in Python, and why is it important?
- Intermediate-Level Python Interview Questions
- What are *args and kwargs?
- Explain list comprehension with an example.
- How do you read and write files in Python?
- Write a program to count vowels in a string.
- How do you handle exceptions in Python?
- What is a lambda function?
- Write a function to reverse a string.
- What are Python sets, and how are they different from lists?
- Explain the difference between append() and extend() in lists.
- Write a function to check if a number is prime.
- Advanced-Level Python Interview Questions
- What are generators, and how do they differ from regular functions?
- What is the Global Interpreter Lock (GIL) in Python?
- Explain Python’s memory management.
- Write a function to flatten a nested list.
- What is the difference between deepcopy() and copy()?
- Write a function to find the Fibonacci number at position n.
- How do you implement a stack using classes?
- How to sort a dictionary by values?
- Write a program to check if two strings are anagrams.
- What are Python’s magic methods?
- Conclusion
Beginner-Level Python Interview Questions
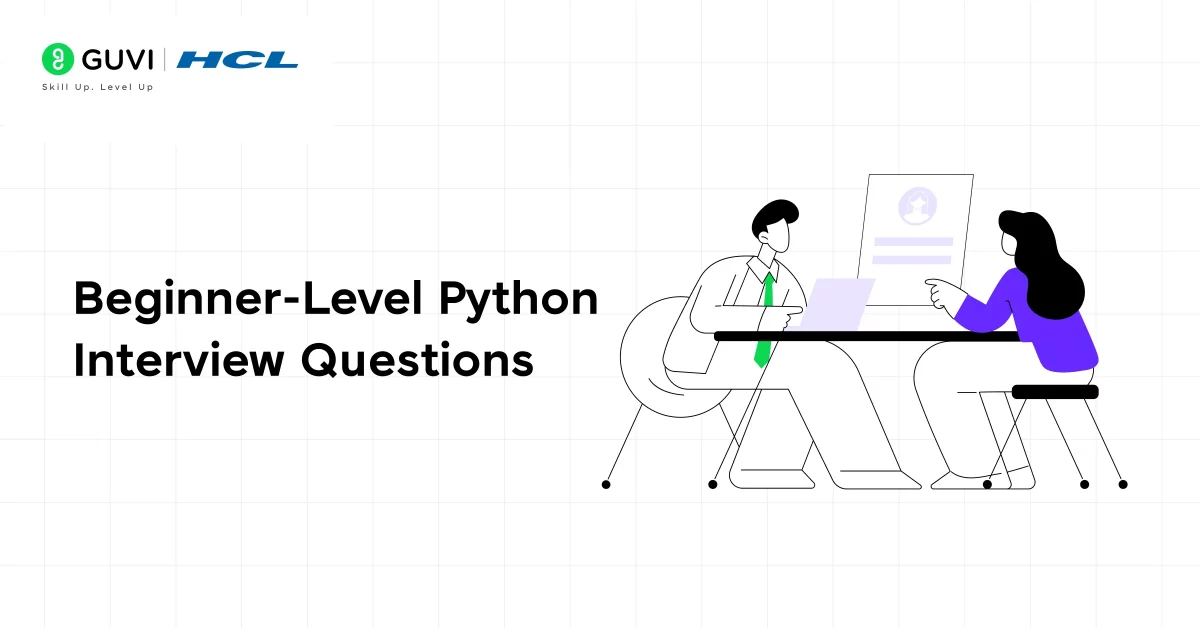
If you’re just starting your Python journey or preparing for your first tech interview, this section is for you. These questions cover the basics, from data types and syntax to simple coding exercises, and are often asked to gauge how well you understand Python’s core concepts. Mastering these is your first step to building a solid foundation.
1. What is Python?
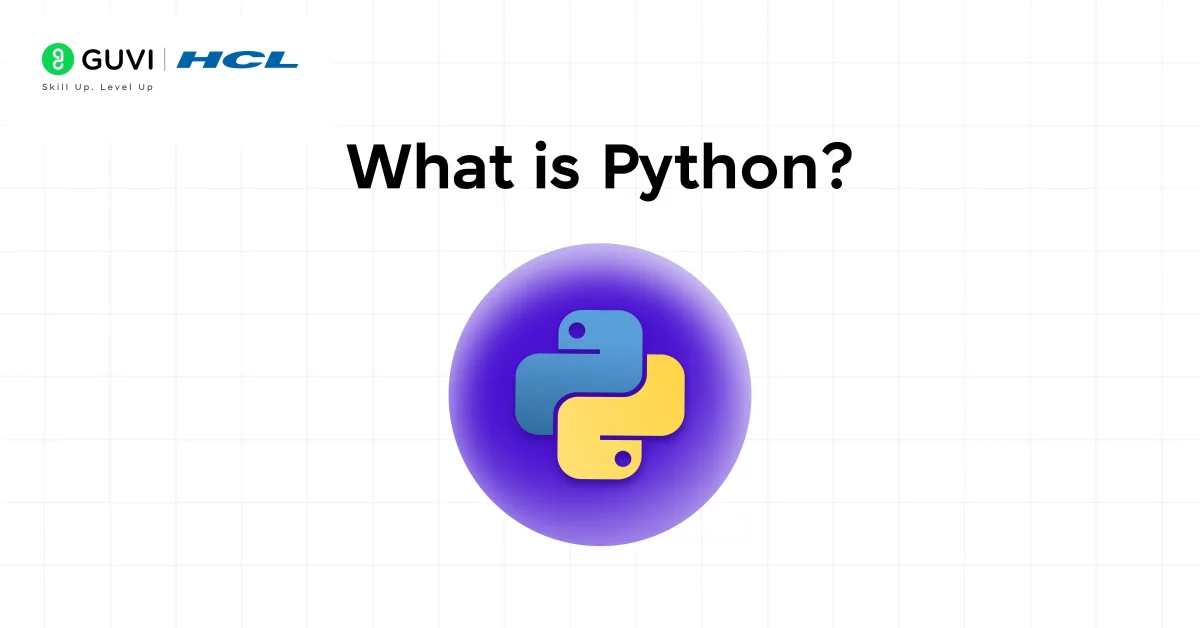
Python is an interpreted, object-oriented, high-level, general-purpose programming language with dynamic semantics. The implementation of Python was started in December 1989 by Guido Van Rossum at CWI in the Netherlands.
In February 1991, he published the code to alt. sources. Furthermore, in 1994, Python 1.0 was released with new features like lambda, map, filter, and reduce.
2. What are the key features of Python?
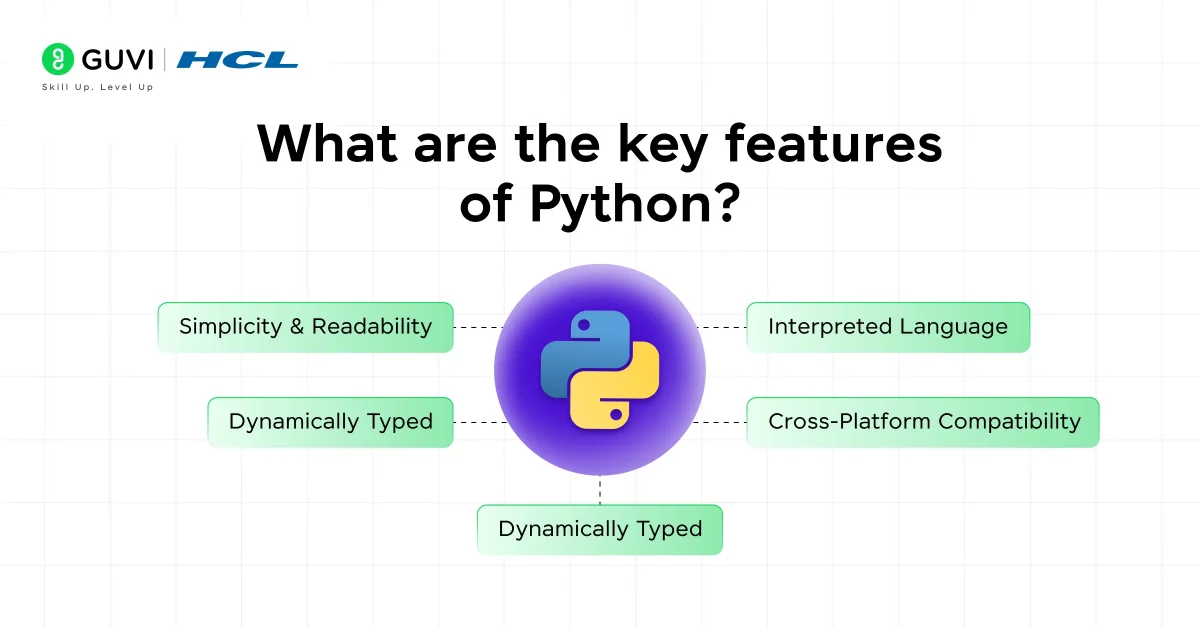
Developers love Python for a few solid reasons:
- Simplicity & Readability: The syntax is clear and resembles English, making it beginner-friendly.
- Interpreted Language: Python executes lines one by one, which simplifies debugging.
- Dynamically Typed: You don’t need to declare variable types; Python figures it out.
- Cross-Platform Compatibility: Write once, run anywhere (Windows, Linux, Mac).
- Large Standard Library: You get modules for math, file I/O, JSON, OS-level operations, and more out of the box.
If you want to understand why learning Python is important, read the blog – Top 12 Key Benefits of Learning Python
3. What is the difference between a list and a tuple?
Both are used to store collections, but they have key differences:
Feature | List | Tuple |
Mutability | Mutable (can change) | Immutable (can’t change) |
Syntax | [] (square brackets) | () (parentheses) |
Use Case | General-purpose collections | Fixed collections (like days of the week) |
Performance | Slightly slower | Faster (due to immutability) |
Example:
my_list = [1, 2, 3]
my_tuple = (1, 2, 3)
Explore: Learn Python in 2025: 10 Interesting Reasons to Do So!
4. How do you write a function in Python?
Functions allow you to encapsulate logic that can be reused.
Syntax:
def function_name(parameters):
# block of code
return result
Example:
def add(a, b):
return a + b
print(add(3, 5)) # Output: 8
Functions are first-class objects in Python — they can be passed around like variables!
5. What are Python’s built-in data types?
Python comes with several built-in data types:
- Numeric: int, float, complex
- Text: str
- Boolean: bool
- Sequence: list, tuple, range
- Mapping: dict
- Set Types: set, frozenset
- Binary: bytes, bytearray
Each data type is an object with built-in methods.
6. How do you check if an element exists in a list?
Use the in keyword to check for membership.
Example:
fruits = ["apple", "banana", "cherry"]
if "banana" in fruits:
print("Yes, it's in the list!")
This works for strings, sets, tuples, and dictionaries too.
Explore: 7 Best Websites to Learn Python for Free
7. What is the difference between is and ==?
This is a common interview trick question.
Operator | Checks for | Example |
== | Value equality | 2 == 2.0 → True |
is | Object identity (memory) | [] is [] → False |
a = [1, 2]
b = [1, 2]
print(a == b) # True → same content
print(a is b) # False → different objects
8. Write a program to find the largest of three numbers.
This is a typical coding warm-up.
Method 1: Using built-in max()
def largest(a, b, c):
return max(a, b, c)
Method 2: Using logic
def largest(a, b, c):
if a >= b and a >= c:
return a
elif b >= a and b >= c:
return b
else:
return c
9. How do you handle user input in Python?
Use the input() function – it always returns a string.
Example:
name = input("Enter your name: ")
print("Hi", name)
You can cast it to other types:
age = int(input("Enter your age: "))
Explore: Top 12 Key Benefits of Learning Python in 2025
10. What is indentation in Python, and why is it important?
Python uses indentation to define code blocks (unlike C, Java, etc., which use {}).
Correct:
if age > 18:
print("Adult")
Incorrect (throws IndentationError):
if age > 18:
print("Adult") # ❌ no indentation
Standard practice is to use 4 spaces for each indentation level.
Intermediate-Level Python Interview Questions

Now that you’ve got the basics down, it’s time to level up. In this section, you’ll find questions that test your ability to write cleaner, more efficient code, work with data structures, and handle real-world logic.
11. What are *args and kwargs?
When you’re unsure how many arguments will be passed to a function, you can use:
- *args for non-keyworded variable-length arguments
- **kwargs for keyworded variable-length arguments
Example with *args:
def sum_all(*args):
return sum(args)
print(sum_all(1, 2, 3)) # Output: 6
Example with **kwargs:
def greet_user(**kwargs):
print(f"Hello {kwargs.get('first_name')} {kwargs.get('last_name')}!")
greet_user(first_name="John", last_name="Doe")
This makes your function flexible and reusable across different input cases.
Discover: Become a Top Python Backend Developer
12. Explain list comprehension with an example.
List comprehension provides a compact syntax for generating new lists from iterables.
Basic Syntax:
[expression for item in iterable if condition]
Example:
squares = [x**2 for x in range(5)]
print(squares) # [0, 1, 4, 9, 16]
With condition:
even_squares = [x**2 for x in range(10) if x % 2 == 0]
It’s more readable and Pythonic than using loops for list creation.
13. How do you read and write files in Python?
Python provides the built-in open() function for file operations.
Reading a file:
with open("sample.txt", "r") as file:
content = file.read()
Writing to a file:
with open("output.txt", "w") as file:
file.write("Hello, Python!")
Using with ensures the file is automatically closed after the block is executed.
14. Write a program to count vowels in a string.
Here’s how to do it using a loop + list comprehension:
def count_vowels(text):
return sum(1 for char in text.lower() if char in 'aeiou')
print(count_vowels("Python Interview")) # Output: 5
The function uses generator expressions for performance and brevity.
15. How do you handle exceptions in Python?
Exception handling helps you gracefully catch errors and avoid program crashes.
Syntax:
try:
# risky code
except SomeError:
# handle error
finally:
# always executes
Example:
try:
x = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed")
finally:
print("Execution finished")
Use try-except to isolate errors and keep your code robust.
16. What is a lambda function?
A lambda function is a short, anonymous function created with the lambda keyword.
Syntax:
lambda arguments: expression
Example:
double = lambda x: x * 2
print(double(5)) # Output: 10
Lambdas are often used with map(), filter(), or sorted() when a quick, inline function is needed.
17. Write a function to reverse a string.
There are many ways, but the most Pythonic is using slicing:
def reverse_string(s):
return s[::-1]
print(reverse_string("Python")) # Output: nohtyP
The slice [::-1] walks through the string in reverse.
18. What are Python sets, and how are they different from lists?
A set is an unordered collection of unique elements.
Set vs List:
Feature | List | Set |
Ordered | Yes | No |
Duplicates | Allowed | Not allowed |
Mutable | Yes | Yes |
Example:
s = set([1, 2, 2, 3])
print(s) # Output: {1, 2, 3}
Sets are useful for membership tests and removing duplicates.
Discover: Best Upskilling Courses in Tech [2025]
19. Explain the difference between append() and extend() in lists.
Both are used to add elements to a list, but they behave differently.
append() adds a single element:
a = [1, 2]
a.append([3, 4])
print(a) # [1, 2, [3, 4]]
extend() adds each element from an iterable:
a = [1, 2]
a.extend([3, 4])
print(a) # [1, 2, 3, 4]
Use extend() when you want to flatten another iterable into your list.
20. Write a function to check if a number is prime.
Here’s a simple yet efficient version:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
print(is_prime(11)) # True
print(is_prime(12)) # False
This uses square root optimization to reduce the number of checks.
In case you want to read more about Python programming from scratch and become well-equipped with the domain, consider reading GUVI’s Python eBook: A Beginner’s Guide to Coding & Beyond, which covers the key concepts of OOPs, File Handling, and Data Connectivity.
Advanced-Level Python Interview Questions
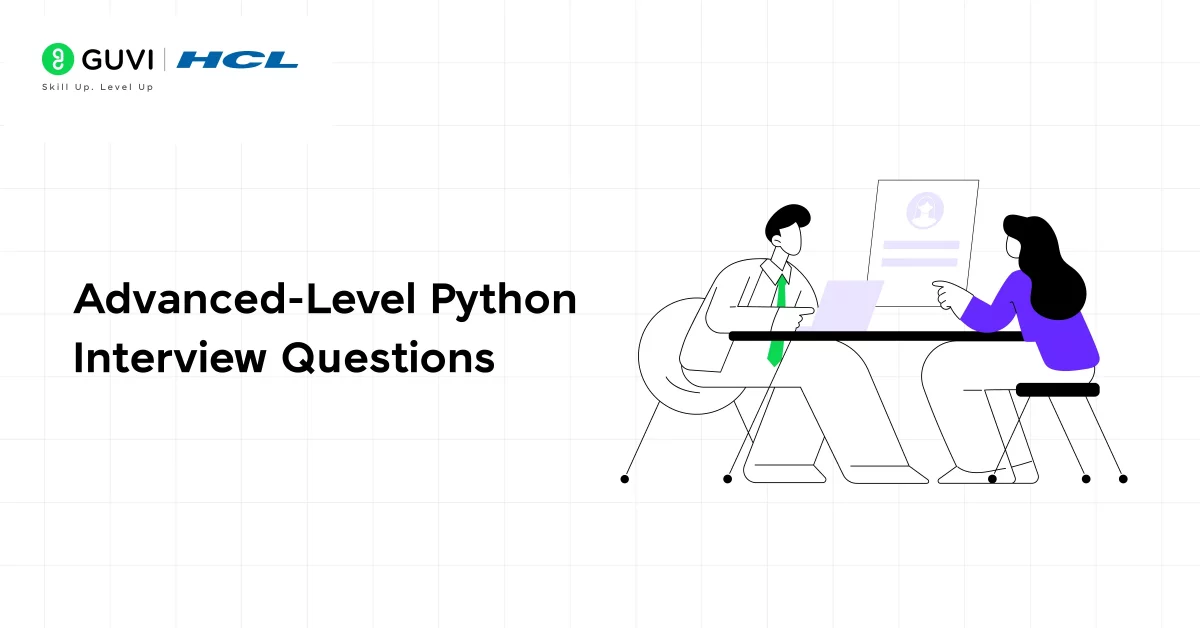
This section is for those who want to stand out. Whether you’re going for mid-level or senior roles, these questions dive into advanced Python concepts like memory management, generators, decorators, and object-oriented design patterns.
21. What are generators, and how do they differ from regular functions?
A generator is a special kind of function that returns an iterator and yields one value at a time, instead of returning all values at once.
- Uses the yield keyword instead of the return
- Maintains its state between successive calls
- More memory efficient for large datasets
Example:
def countdown(n):
while n > 0:
yield n
n -= 1
for number in countdown(5):
print(number)
Compared to returning a full list, generators are ideal when working with large streams of data or infinite sequences.
22. What is the Global Interpreter Lock (GIL) in Python?
The Global Interpreter Lock (GIL) is a mechanism that restricts the execution of multiple threads in the CPython interpreter. Even if you write multi-threaded code, only one thread can execute Python bytecode at a time.
- This limits true parallelism in CPU-bound operations.
- It doesn’t affect I/O-bound tasks (like file reading or network calls).
To fully utilize multiple cores, you can use the multiprocessing module instead of threading.
23. Explain Python’s memory management.
Python handles memory management automatically using:
- Reference Counting – Each object has a count of references pointing to it. When it reaches zero, it’s deallocated.
- Garbage Collection – A cyclic garbage collector (gc module) handles objects involved in reference cycles.
You don’t need to manually free memory like in C/C++, but you should avoid creating reference cycles or leaky containers that hold unnecessary data.
24. Write a function to flatten a nested list.
Here’s a recursive approach to flattening a list of lists:
def flatten(lst):
result = []
for item in lst:
if isinstance(item, list):
result.extend(flatten(item))
else:
result.append(item)
return result
print(flatten([1, [2, [3, 4]], 5])) # Output: [1, 2, 3, 4, 5]
This function works regardless of how deeply nested the list is. You could also explore itertools.chain() for non-recursive flat lists.
25. What is the difference between deepcopy() and copy()?
- copy() creates a shallow copy — it copies references of nested objects.
- deepcopy() creates a deep copy — it recursively copies all nested objects.
Example:
import copy
a = [[1, 2], [3, 4]]
b = copy.copy(a)
c = copy.deepcopy(a)
a[0][0] = 100
print(b) # [[100, 2], [3, 4]] → changed!
print(c) # [[1, 2], [3, 4]] → unaffected!
Use deepcopy() when you want complete isolation from the original object.
26. Write a function to find the Fibonacci number at position n.
The recursive approach is intuitive but inefficient:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
But for performance, use memoization:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
Or go iterative for optimal speed:
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
a, b = b, a + b
return a
27. How do you implement a stack using classes?
A stack follows LIFO (Last In, First Out). Let’s implement it with a class:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop() if not self.is_empty() else None
def peek(self):
return self.items[-1] if not self.is_empty() else None
def is_empty(self):
return len(self.items) == 0
You can also implement a queue using collections.deque for better performance.
28. How to sort a dictionary by values?
A dictionary in Python is an important data structure. By using a Dictionary, we can store data in the form of key-value pairs.
Each key in a dictionary can store at most one value. While declaring a key, it must be noted that the keys in the dictionary must be immutable, and the supported data types for declaring a key are: integers, strings, and tuples, as these are immutable.
sorted() function in Python
The sorted function is used to sort an iterable object in a particular order. And then it returns the result in the form of a list.
Syntax
sorted(iterable, key, reverse)
29. Write a program to check if two strings are anagrams.
Two strings are anagrams if their sorted characters match.
def is_anagram(s1, s2):
return sorted(s1) == sorted(s2)
print(is_anagram("listen", "silent")) # True
For large-scale checks, consider using collections. Counter for better performance.
30. What are Python’s magic methods?
Magic methods (also called dunder methods, short for “double underscore”) let you override built-in behavior in Python classes.
Examples:
- __init__() – Constructor
- __str__() – String representation
- __len__() – Length of object
- __eq__() – Equality comparison
- __add__() – + operator
By implementing magic methods, you make your classes more intuitive and expressive.
If you want to learn more about Python through a structured course material, consider enrolling in GUVI’s Free Self-Paced IITM Pravartak Certified Python Course that lets you start from scratch and gradually move towards the level where you can write programs to gather, clean, analyze, and visualize data.
Conclusion
In conclusion, interviews are not just about syntax recall; they’re about how you think, structure your code, and solve problems under pressure. The key is not to memorize answers but to practice implementing them, tweaking them, and understanding the “why” behind each one.
Bookmark this guide, revisit the tough ones, and keep coding. You’re closer to cracking that dream Python role than you think!
Did you enjoy this article?