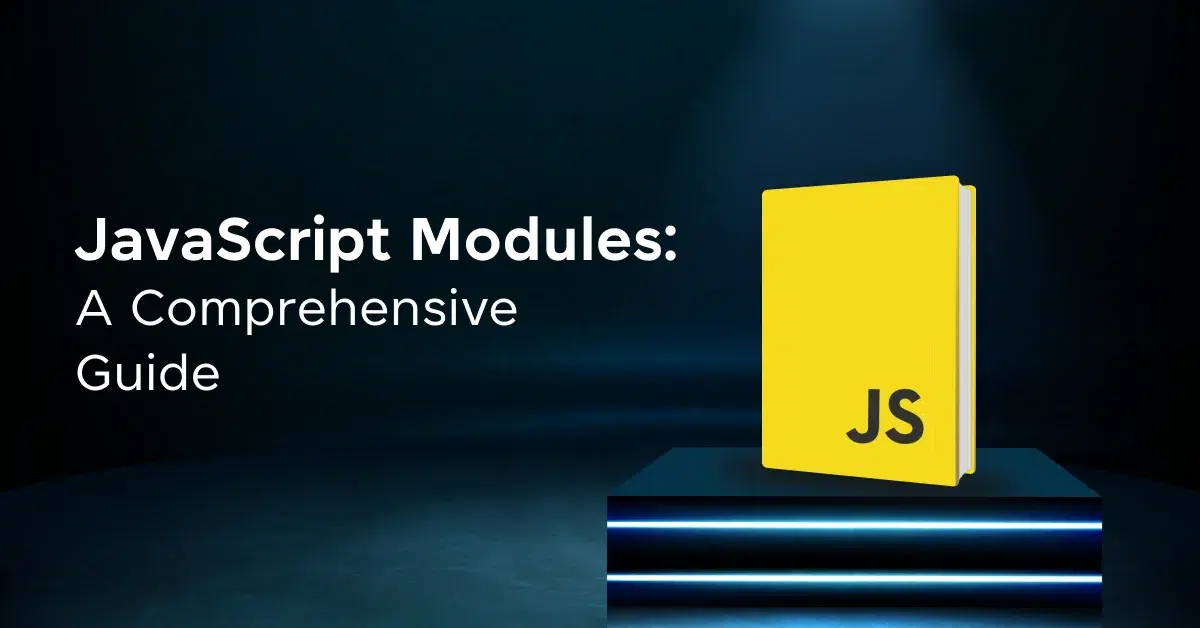
JavaScript Modules: A Comprehensive Guide [2024]
Mar 25, 2024 7 Min Read 1111 Views
(Last Updated)
Writing code for a website in JavaScript is a tough task in itself but more than that, even if you finish coding it, re-visiting it to make minor changes is one of the hardest tasks as you have to search thousands of lines of code to find that one minor change.
So, is there any way to simplify that process? Yes. There is a concept that will make you JavaScript experts feel at ease and that is JavaScript Modules. You’ll see more about that later but honestly, it will save you from all that manual searching work.
In this article, you are going to learn more about JavaScript modules that will help you sort codes whilst coding so that you can revisit it anytime to make changes.
Without any delay, let’s get started.
Table of contents
- What are JavaScript Modules?
- Benefits of Using JavaScript Modules
- Keep your Code Clean and Organized
- Makes Your Code Reusable
- Easier to Maintain
- Improves Performance
- How to Use JavaScript Modules?
- Step 1: Create Your Modules
- Step 2: Use Your Modules
- Common Types of JavaScript Modules
- CommonJS (CJS)
- ES Modules (ESM)
- Best Practices for Using JavaScript Modules
- Keep Your Modules Focused
- Use Clear and Descriptive Names
- Prefer Named Exports Over Default Exports
- Import Only What You Need
- Organize Imports and Exports Clearly
- Be Mindful of Module Side Effects
- Conclusion
- FAQs
- What are named exports and how are they useful?
- Is it possible to mix different module systems in one project?
- What is a default export and when should it be used?
- Are dynamic imports supported in all browsers?
- How do you handle module dependencies in JavaScript?
What are JavaScript Modules?
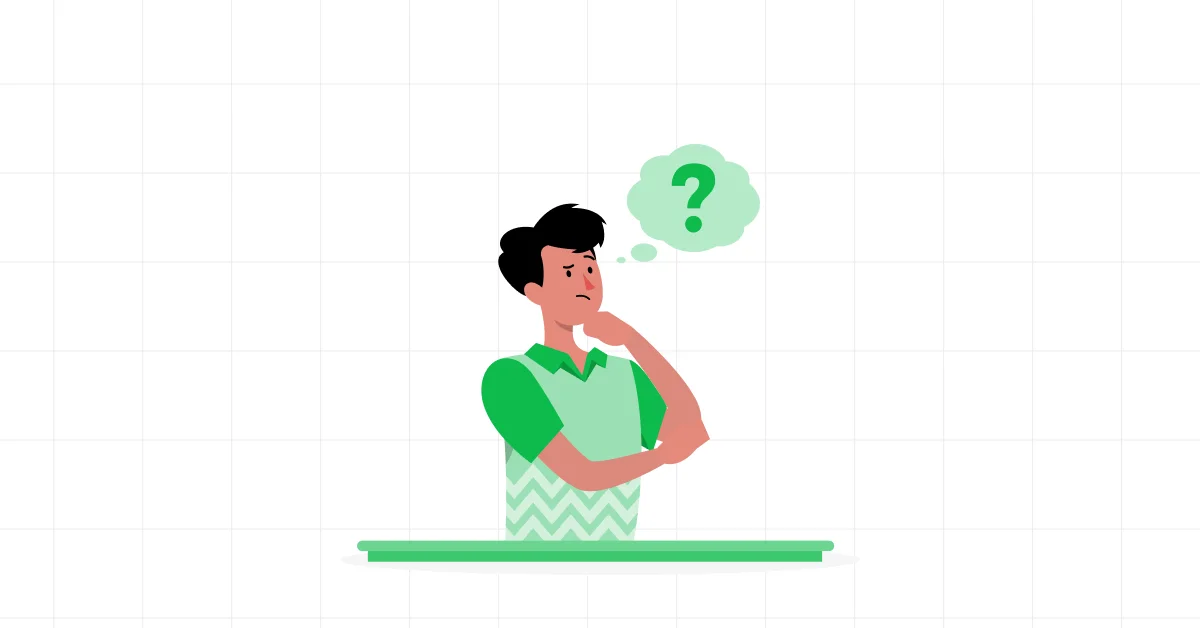
Let us start by understanding JavaScript Modules with a fun analogy instead of a boring textbook definition. Imagine you’re building a giant LEGO set. Instead of dumping all the LEGO pieces into a single giant pile, you sort them into smaller, manageable boxes.
Each box contains pieces that are meant to build a specific part of the set—a car, a house, a tree, and so on. This sorting makes it easier to find what you need and understand how each piece fits into the bigger picture. JavaScript modules work in a very similar way.
In full-stack development, JavaScript modules are like those smaller, sorted boxes of LEGO pieces. A JavaScript module is essentially a file. But it’s not just any file—it’s a special one that contains specific bits of code.
This could be anything from a collection of functions that deal with dates, to a set of variables that hold colors for your website’s theme, or even a single function that pops up a message on the screen.
In simple terms, JavaScript modules help you organize your code by breaking it down into smaller, more manageable pieces. This organization not only makes your code cleaner and easier to understand but also improves the way different parts of your code interact with each other.
Know More: Best JavaScript Roadmap Beginners Should Follow 2024
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
Benefits of Using JavaScript Modules
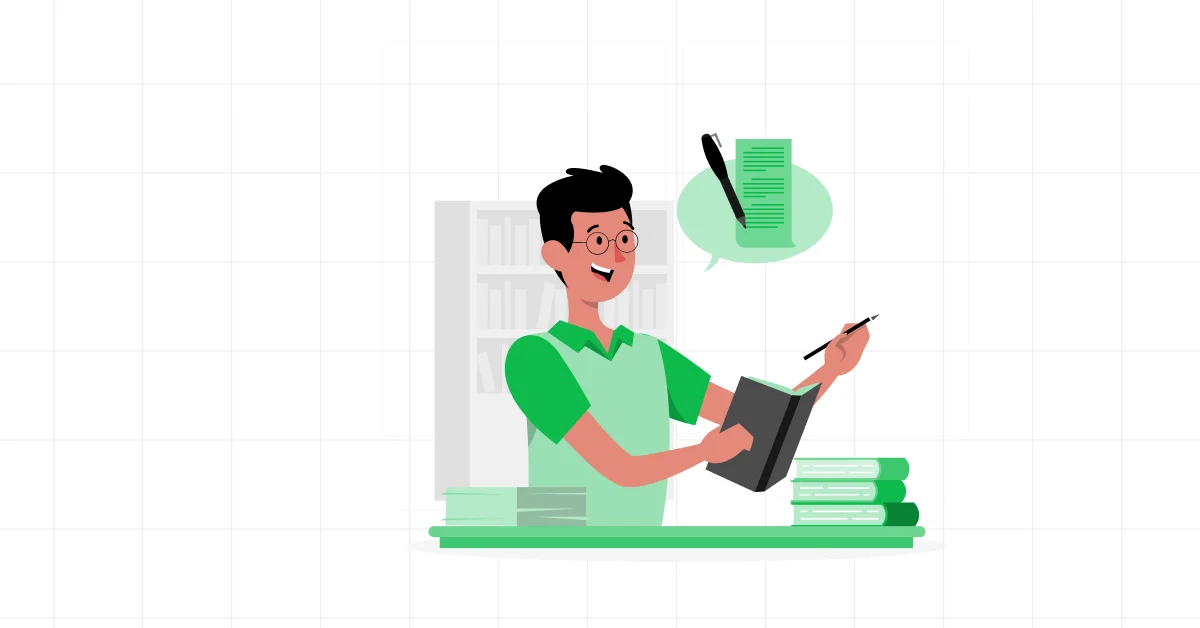
When we talked about JavaScript modules, we used an analogy of organizing LEGO pieces into boxes and that was easy and fun to understand, right? Similarly, we will be comparing the benefits of JavaScript modules with organizing a kitchen.
Now, let’s explore why this organization is not just neat but beneficial, especially when building websites or web applications.
1. Keep your Code Clean and Organized
Think of your kitchen. You have drawers for utensils, shelves for dishes, and cabinets for pots and pans. This organization makes it easy to find what you need and keeps your kitchen tidy.
JavaScript modules do the same for your code. By dividing your code into modules, you create a clean environment where everything has its place, making it simpler for you or anyone else to understand and navigate through your project.
2. Makes Your Code Reusable
Have you ever made a meal and had leftovers that you could use for another meal? That’s efficiency. Modules allow you to write code once and then reuse it in different parts of your project, or even in entirely different projects.
It saves time and effort because you don’t have to start from scratch every time.
Also Explore: Arrays in JavaScript: A Comprehensive Guide
3. Easier to Maintain
Imagine if something gets dirty in your kitchen. If the kitchen is neatly organized, you can clean on just that part without having to worry about the entire kitchen.
Similarly, if there’s an issue in your code or you need to update a part of it, JavaScript modules make it much easier to do so. You can simply go to the specific module where the issue lies, fix it, and you’re done.
This compartmentalization of code saves time and reduces the risk of accidentally messing up other parts of your code.
4. Improves Performance
Coming back to the kitchen analogy, imagine if you had to unload your entire kitchen every time you cooked a meal. That would be inefficient and time-consuming, right?
To prevent that, JavaScript modules allow developers to load only the parts of the code they need, when they need them. This is like having all your ingredients prepped and ready to go.
It makes your website faster because it doesn’t have to load unnecessary code, leading to a better experience for your users.
Explore: Best Techniques for Creating Seamless Animations with CSS and JavaScript [2024]
How to Use JavaScript Modules?
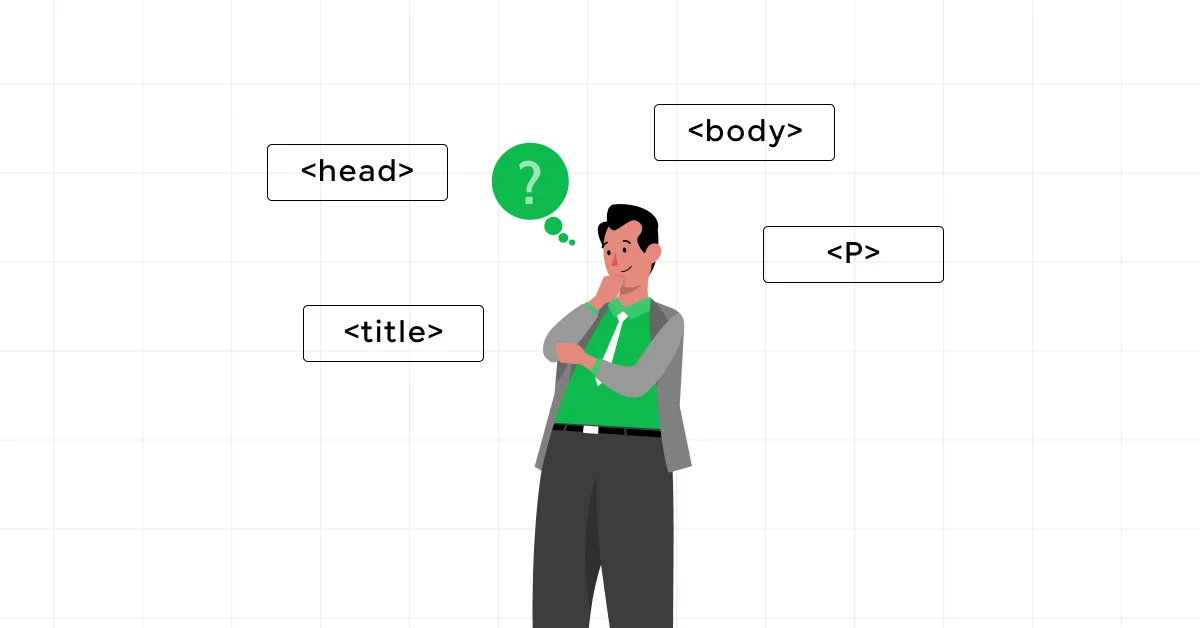
We talked a lot about JavaScript modules and its benefits. But, what’s more interesting than learning how to use those? That’s what you are going to see in this section.
Using JavaScript modules is like putting together a puzzle. Each piece (module) has its unique spot, and when you find where it fits, you start seeing the whole picture (your web app) come together.
It sounds technical, but it’s quite straightforward once you get the hang of it. Let’s break it down into simple steps, and learn how to use JavaScript modules with a basic example.
Step 1: Create Your Modules
First, you need to create your JavaScript modules. Think of a module as a container for a group of related functions or items. For our example, let’s make a simple math module that can add and subtract numbers.
Create a file named 'mathModule.js'
. This is your module file. Inside this file, you’re going to define some functions. Here’s how:
// mathModule.js
// This function adds two numbers
function add(a, b) {
return a + b;
}
// This function subtracts one number from another
function subtract(a, b) {
return a - b;
}
// Here we make our functions available to other files that want to use this module
export { add, subtract };
Also Read: Constructors in JavaScript: 6 Uses Every Top Programmer Must Know
Step 2: Use Your Modules
Now, let’s use the functions from your module in a different file. Suppose you have another file called 'app.js'
where you want to use the 'add'
and 'subtract'
functions.
In 'app.js'
, you’ll “import” the functions you need. Like this:
// app.js
// Import the add and subtract functions from the mathModule.js file
import { add, subtract } from './mathModule.js';
// Now you can use them just like any other function
console.log(add(2, 3)); // This should print 5 to the console
console.log(subtract(5, 3)); // This should print 2 to the console
What Just Happened?
- You created a module called
'mathModule.js'
that contains two functions:'add'
and'subtract'
. - You then used those functions in another file by importing them. This means you can write a function once, in one file, and use it wherever you need it by importing.
This modular approach has several benefits:
- Organization: Your code is cleaner and easier to manage when it’s broken down into focused modules.
- Reusability: Write your code once and use it in multiple places.
- Maintainability: When you need to update a function, you do it in one place, and the update takes effect wherever the module is used.
And there you have it! That’s how you use JavaScript modules. By breaking your code into smaller, reusable pieces, you make your life as a developer much easier and your code is now much cleaner and more efficient.
Learn More: Most Popular JavaScript Front-End Tools in 2024
Common Types of JavaScript Modules
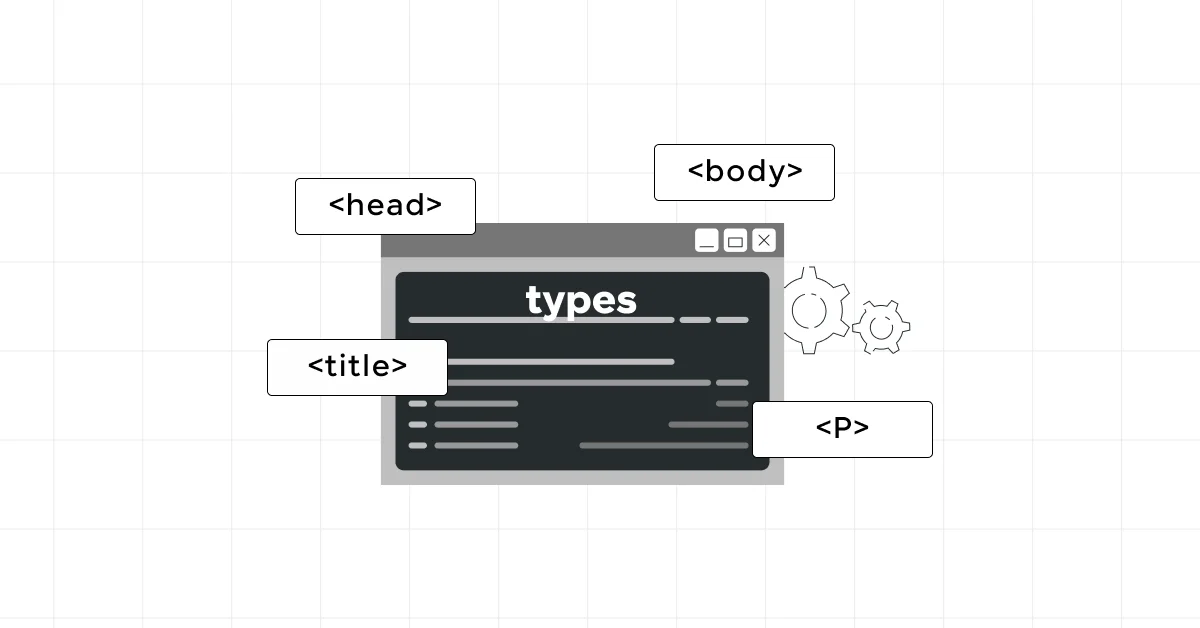
When you’re getting into JavaScript development, you’ll quickly encounter terms like ES Modules and CommonJS. These are different ways (or formats) to use modules in JavaScript.
Think of these systems as different types of containers for your code, each with its own way of packing and unpacking. Let’s look at the two most common types: CommonJS and ES Modules.
1. CommonJS (CJS)
Imagine you’re packing your stuff to move. You decide to use sturdy, reliable boxes that you can easily stack and unstack. In the JavaScript world, CommonJS modules are like these boxes.
Originally developed for use in Node.js (JavaScript’s way of running outside a web browser), CommonJS modules are straightforward and synchronous. This means when you’re using them, you’re essentially saying, “Load this piece of code, then wait until it’s fully loaded before moving on.”
Before ES Modules were introduced, CommonJS was the go-to way to create and use modules in Node.js applications. In CommonJS, you use 'require'
to import modules and 'module.exports'
to export them.
Here’s how you might see CommonJS in action:
// Creating a module in CommonJS
// math.js
module.exports = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
};
// Using a module in CommonJS
// app.js
const math = require('./math');
console.log(math.add(2, 3)); // The output will be 5
In this example, 'module.exports'
is used to pack up the code in 'math.js'
, and 'require()'
is used to unpack it from that.
Learn All About Loops in JavaScript: A Comprehensive Guide
2. ES Modules (ESM)
Now, imagine instead of those sturdy boxes that you used in the previous case, now you have sleek, modern suitcases with compartments and organizers.
These suitcases are designed to be both efficient and easy to handle, perfect for air travel. ES Modules are the sleek suitcases of the JavaScript module world.
ES Modules, or ECMAScript Modules, are the official standard for working with modules in JavaScript, introduced in ES6 (ECMAScript 2015).
They’re designed for the static structure of the web and support asynchronous loading. This means you can load modules in parallel, making your web applications faster and more efficient.
They work in most modern web browsers and with tools like Webpack for bundling your code.
In ES Modules, you use 'export'
to share code from one module and 'import'
to use that code in another module.
Here’s a simple example of using ES Modules:
// Creating a module in ESM
// math.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
// Using a module in ESM
// app.js
import { add, subtract } from './math.js';
console.log(add(5, 3)); // The output will be 8
In this setup, 'export'
is used to specify which parts of the module you want to share, and 'import'
allows you to choose which parts you want to use.
The Key Difference
- CommonJS is like those reliable moving boxes—great for server-side when you’re loading one thing at a time.
- ES Modules are like those efficient suitcases—perfect for the web, where loading things quickly and in parallel is key.
Both types of modules have their place in JavaScript modules, and understanding when and how to use them will make you a more versatile and effective developer.
Understand all about Backend Development: Roles, Responsibilities, Skills, and Salary
Best Practices for Using JavaScript Modules
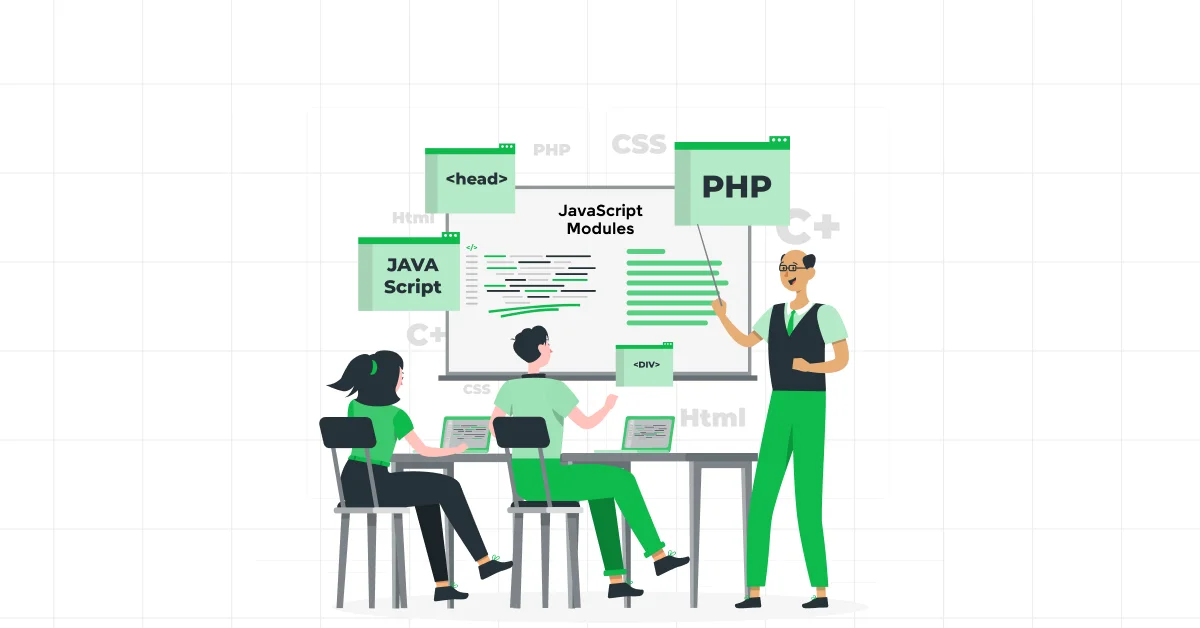
Using JavaScript modules is like organizing a big family dinner. Just as you want everything from dishes to seating arranged perfectly for smooth interactions and a memorable evening, organizing your code with JavaScript modules requires attention to detail and adherence to certain best practices.
Let’s look at some of these practices in simple terms, ensuring your code is not just functional but a pleasure to work with.
1. Keep Your Modules Focused
Imagine each module as a dish on the table. Just as you wouldn’t mix dessert with the main course in one dish, keep your modules focused on a single responsibility.
This makes it easier to find, update, and reuse your code. If a module is about handling user input, keep it that way and don’t mix in unrelated functionality like sending emails.
2. Use Clear and Descriptive Names
Naming your modules is like labeling dishes at a buffet. You want everyone to know exactly what they’re getting without having to guess. Use names that clearly describe what the module does or contains.
For instance, a module that handles user authentication might be named 'authManager'
rather than something vague like 'utils'
.
Must Know About Top 10 Full-Stack Developer Frameworks in 2024
3. Prefer Named Exports Over Default Exports
When you’re sharing dishes at the table, it’s better to have a variety of clearly identified options rather than one big mystery dish.
Similarly, using named exports makes it clear what functionality you’re offering from a module. It helps avoid confusion and makes your code more predictable. For example, instead of exporting a single default object, export each function or variable with its own name.
// Instead of this
export default {
fetchData,
parseData,
};
// Do this
export { fetchData, parseData };
4. Import Only What You Need
Just as you wouldn’t fill your plate with every dish on the table if you’re only going to eat a few, import only the functions or variables you need from a module.
This keeps your code cleaner and can improve performance by reducing the amount of unused code.
// Instead of importing everything
import * as Utils from './utils';
// Just import what you need
import { formatDate } from './utils';
5. Organize Imports and Exports Clearly
Imagine organizing the dinner table so that everyone knows where to sit and can easily reach the dishes they want. Similarly, organize your imports and exports at the top of your module files. This makes it easy to see dependencies at a glance and keeps your code tidy.
6. Be Mindful of Module Side Effects
If a dish is too spicy, it can affect everyone’s meal experience. A module with side effects (like modifying global variables) can have unexpected impacts on other parts of your application. Be cautious with JavaScript modules that might introduce side effects and isolate them when possible.
To know what side effects may occur with JavaScript Modules, you need to extensively practice those and to help you with that, GUVI’s Webkata provides you with a series of tests that let you get hands-on experience with JavaScript.
Also Read: Master Backend Development With JavaScript | Become a Pro
If you want to learn more about JavaScript Modules and their implementation, then you must sign up for GUVI’s Certified Full Stack Development Course, which gives you in-depth knowledge of the practical implementation of Javascript through various real-life FSD projects.
Conclusion
In conclusion, JavaScript modules are essential for managing and maintaining the vast expanse of code in modern full-stack development. From encapsulating code to enhance reusability and maintainability, understanding the different types, to following best practices for a clean and efficient codebase, modules are fundamental.
They not only streamline development processes but also ensure that your project remains scalable and organized. Embracing JavaScript modules in projects paves the way for a more structured, understandable, and collaborative coding experience.
Also Read: Top Full Stack Development Trends for 2024: What to Expect
FAQs
1. What are named exports and how are they useful?
Named exports allow individual functions, variables, or classes to be exported from a module. They are useful for clarity and specificity, enabling the import of only what’s necessary.
2. Is it possible to mix different module systems in one project?
While technically possible, mixing module systems (like CommonJS and ES Modules) can complicate build processes and runtime behavior. It’s generally recommended to stick with one system for consistency.
3. What is a default export and when should it be used?
A default export allows a module to export a single value as its default export. It’s useful when a module is designed to offer one main functionality or when it’s expected that one function or object will be primarily used.
4. Are dynamic imports supported in all browsers?
Dynamic imports are widely supported in modern browsers, allowing for code splitting and lazy loading. However, older browsers may require polyfills for full compatibility.
5. How do you handle module dependencies in JavaScript?
Dependencies are managed through the import
statement in ES Modules and the require
function in CommonJS, allowing modules to declare which other modules they depend on.
Did you enjoy this article?