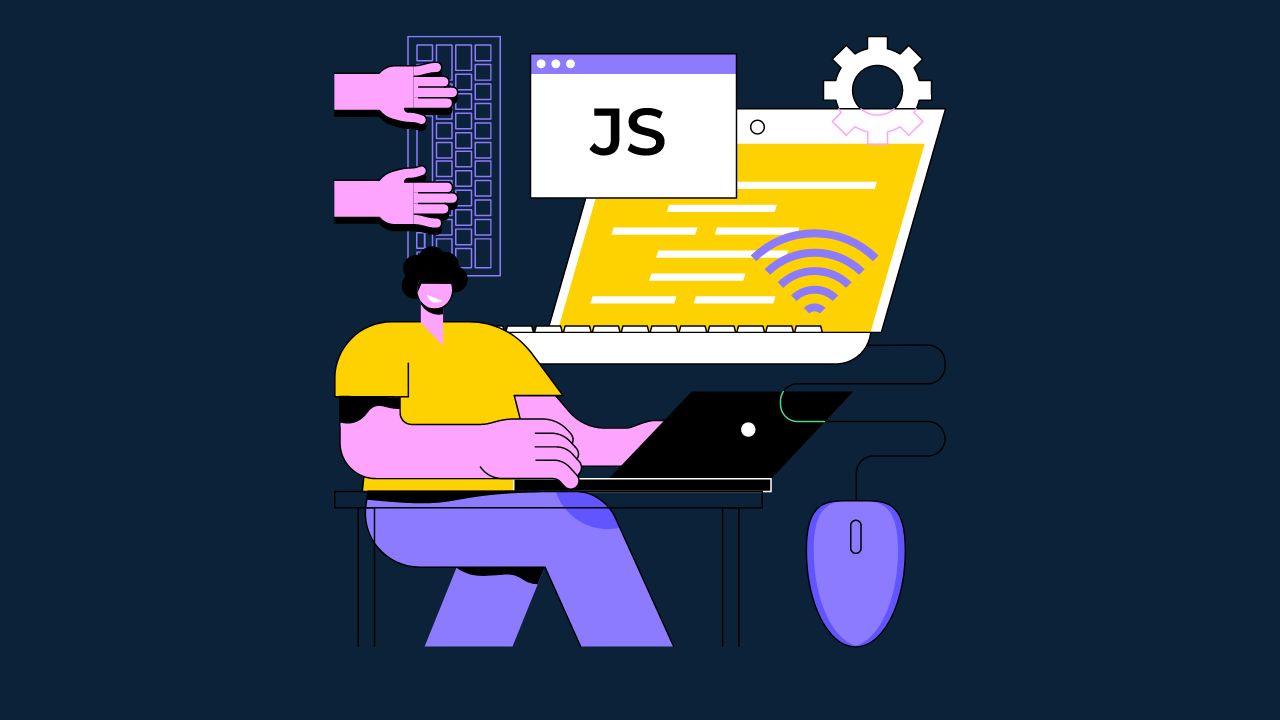
Master Backend Development With JavaScript | Become a Pro
Sep 02, 2024 6 Min Read 2117 Views
(Last Updated)
Backend development refers to the development of the server side of web applications. It involves building the infrastructure, logic, and databases that enable the front-end or client-side of the application to interact with the server and provide data or functionality to users.
Backend development plays a critical role in web development, as it provides the foundation for web applications’ overall functionality, performance, and security. A well-designed and optimized backend can help ensure that web applications are fast, reliable, and scalable.
This roadmap will provide an overview of the key concepts and technologies involved in backend development using JavaScript. From setting up a development environment to deploying a production-ready application, we will cover everything you need to know to become a skilled backend developer in JavaScript.
So, whether you are a beginner who is looking to learn basics or an experienced developer seeking to enhance your skills, this roadmap will provide a clear path to mastering backend development with JavaScript.
Table of contents
- Fundamentals of Backend Development in JavaScript
- Building Web Servers with Node.js
- Working with Databases in Node.js
- Authentication and Authorization
- Testing and Debugging Node.js Applications
- Deploying Node.js Applications
- Choose a hosting provider
- Set up the server environment
- Build and deploy your application
- Manage dependencies and environment variables
- Monitor your application
- Conclusion
Fundamentals of Backend Development in JavaScript
Node.js is a JavaScript runtime environment that allows developers to build server-side applications using JavaScript. It uses an event-driven, non-blocking I/O model that is lightweight and efficient.
Server-side JavaScript refers to using JavaScript on the server side of web applications. It allows developers to write both the front-end and back-end of web applications in the same language, providing a more streamlined and efficient development process.
To start with Node.js, you must install it on your local machine. You can download the latest version of Node.js from the official website and follow the installation instructions for your operating system.
Node Package Manager is package manager for Node.js that allows developers to easily install, manage, and share reusable code packages. It provides access to a vast ecosystem of open-source packages that can be used to speed up the development process and add functionality to your applications. You can use NPM to install packages locally or globally and manage package dependencies for your applications.
If you’re interested in upgrading from backend development, then go beyond for full-stack development essentials like front-end frameworks, back-end technologies, and database management. Join GUVI’s Full Stack Development Career Program with Placement Assistance. With this program, you will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Building Web Servers with Node.js
Express.js is a very popular web application framework for the Node.js that makes it easy to build web servers and APIs. It provides a simple and flexible interface for handling HTTP requests and responses and a powerful set of features for building complex web applications.
To build a simple HTTP server with Express.js, you can start by creating a new Node.js project and installing the Express.js package using NPM. Then, you can create a new file for your server code and import the Express.js module:
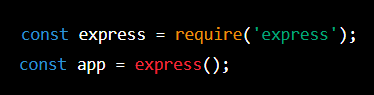
Next, you can define a route for handling HTTP requests to the root URL of your server:
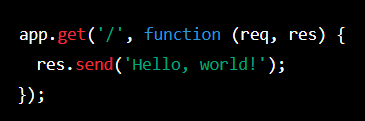
This route uses the get method of the app object to define a handler function that sends a response with the text “Hello, world!”.
To create a RESTful API with Express.js, you can define routes for handling different HTTP requests and responses. For example, you might define a route for retrieving a list of items from a database:
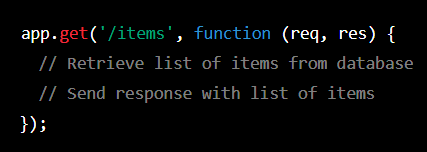
This route uses the get method of the app object to define a handler function that retrieves a list of items from a database and sends a response with the list of items.
Express.js provides a powerful and flexible framework for building web servers and APIs with Node.js. By mastering its features and capabilities, you can easily create fast, efficient, and scalable backend applications.
Working with Databases in Node.js
Databases are essential to backend development, as they allow developers to store, retrieve, and manage data for web applications. There are two main types of databases: SQL and NoSQL databases.
SQL databases, such as MySQL and PostgreSQL, are relational databases that use tables to store and organize data. NoSQL databases, such as MongoDB and CouchDB, are non-relational databases that use collections to store data in a more flexible and scalable way.
To connect to a database with Node.js, you can use a database driver or an Object-Relational Mapping (ORM) library such as Mongoose for MongoDB. The driver or ORM library will provide methods and APIs for interacting with the database, allowing you to perform CRUD (Create, Read, Update, Delete) operations on the data.
For example, to connect to a MongoDB database with Mongoose, you can create a new connection using the connect method and define a schema for your data:
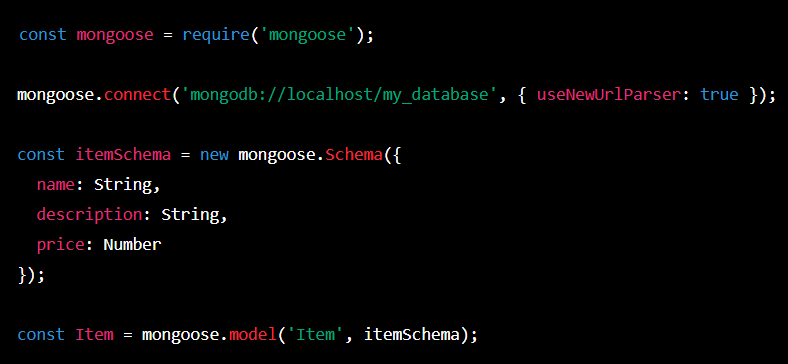
This code creates a new connection to a MongoDB database running on the local machine, defines a schema for an item with a name, description, and price field, and creates a new model for the schema.
To create a simple API that interacts with the database, you can define routes for handling HTTP requests and responses that use the Mongoose model to perform CRUD operations on the data. For example, you might define a route for creating a new item:
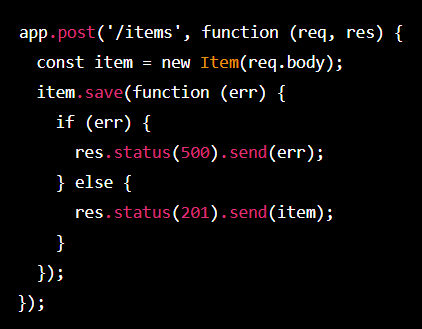
This route uses the post method of the app object to define a handler function that creates a new item from the request body, saves it to the database using the save method of the Mongoose model, and sends a response with the created item.
Overall, working with databases in Node.js requires a solid understanding of database concepts and technologies and the ability to use drivers or ORM libraries to interact with the data. By mastering these skills, you can easily create robust and scalable backend applications that can handle large amounts of data.
Authentication and Authorization
Authentication and authorization are essential concepts in web development that control resource access and protect sensitive data. Authentication refers to the process of verifying the identity of a user. In contrast, authorization refers to granting or denying access to resources based on the user’s identity and permissions.
To implement user authentication in Node.js, you can use a library such as Passport.js, which provides a flexible and modular framework for authenticating users with various authentication strategies, such as local authentication with a username and password or authentication with social media accounts.
For example, to implement local authentication with Passport.js, you can create a new strategy and define a handler function that checks the user’s credentials:
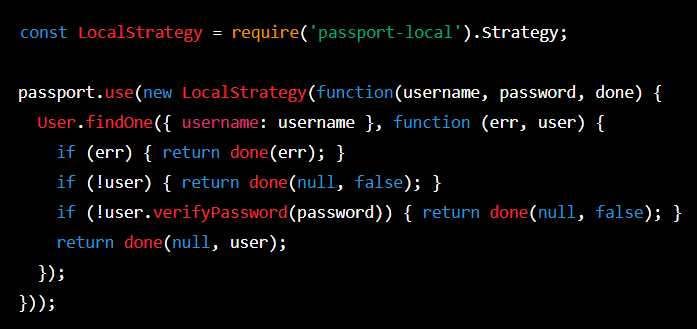
This code creates a new local strategy with Passport.js. It defines a handler function that uses the Mongoose findOne method to find a user with the given username and password and returns an error if the user is not found or the password is incorrect.
To use JSON Web Tokens (JWT) for token-based authentication, you can use a library such as jsonwebtoken to create and verify tokens that contain user information and expiration dates. For example, you might create a token when a user logs in and store it in a cookie or local storage:

This code creates a new token with the user’s ID and a secret key and sets an expiration time of one hour.
To handle user permissions and roles, you can use a middleware function that checks the user’s role or permissions before allowing access to certain resources. For example, you might define a middleware function that checks if the user is an administrator before allowing access to certain routes:
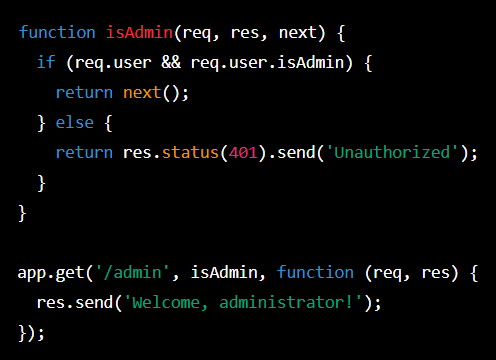
This code defines a middleware function called isAdmin that checks if the user is an administrator and a route that requires the user to be an administrator to access.
Overall, authentication and authorization are essential aspects of web development that require a solid understanding of security concepts and the ability to implement secure and scalable solutions with libraries and frameworks such as Passport.js and JWT. By mastering these skills, you can create secure and reliable backend applications that protect user data and resources from unauthorized access.
Testing and Debugging Node.js Applications
Testing and debugging are essential aspects of backend development that ensure the quality and reliability of your Node.js applications. Unit testing and integration testing are two common types of testing used to check the functionality and integration of individual components of your application.
To write unit tests in Node.js, you can use a testing framework such as Mocha, which provides a simple and flexible interface for defining and running tests, and an assertion library such as Chai, which provides a range of assertion styles and utilities for testing values and objects. For example, you might define a simple test case that checks if a function returns the correct output:
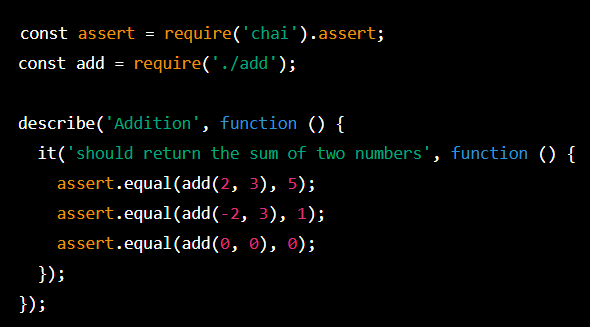
This code defines a test suite with Mocha and a test case that checks if the add function returns the correct sum for different input values.
To debug Node.js applications, you can use the built-in Node Debugger, which provides a command-line interface for setting breakpoints, stepping through code, and inspecting variables and objects at runtime. For example, you might start a Node.js application in debug mode and attach a debugger to it using the –inspect flag:
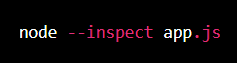
This code starts the app.js file in debug mode and listens for debugger connections on a specific port.
Overall, testing and debugging are essential skills for backend developers that help ensure the reliability and security of your applications. You can write and debug tests and applications more efficiently and effectively by using tools and libraries such as Mocha, Chai, and the Node Debugger.
Deploying Node.js Applications
Deploying a Node.js application to production involves several steps, including configuring the server environment, setting up a deployment pipeline, and managing dependencies and environment variables. Here are some key steps and best practices for deploying Node.js applications:
1. Choose a hosting provider
Several hosting providers support Node.js applications, including Heroku, AWS, Google Cloud, and Digital Ocean. Choose a provider based on your specific needs, such as scalability, cost, and ease of use.
2. Set up the server environment
Configure your server environment with the necessary dependencies and runtime environment for your application. This might include installing Node.js, setting up a web server, and installing required packages or libraries.
3. Build and deploy your application
Create a deployment pipeline that builds and deploys the application automatically. This might involve using a tool like Jenkins or Travis CI to automate the build process and using a deployment tool like Capistrano or Git to automate the deployment process.
4. Manage dependencies and environment variables
Make sure your application dependencies are up-to-date and properly configured. Use environment variables to manage sensitive information such as API keys and database credentials.
5. Monitor your application
Use tools like New Relic or AppDynamics to monitor your application’s performance and detect any issues or errors.
When deploying Node.js applications, it’s essential to follow best practices to ensure the security and reliability of your application. These best practices include using HTTPS for secure communication, a load balancer for scalability, and implementing security measures like firewalls and various intrusion detection systems.
Additionally, it’s essential to keep your application up-to-date with security patches and to regularly back up your data to prevent data loss in case of an outage or security breach.
Heroku is a popular hosting platform for Node.js applications that offers a range of tools and services for deployment and management. Heroku provides a simple and easy-to-use interface for deploying Node.js applications and offers features such as auto-scaling, logging, and monitoring features. To deploy a Node.js application to Heroku, you can use the Heroku CLI or integrate your deployment pipeline with Heroku’s API.
Conclusion
In this roadmap, we covered the fundamentals of backend development in JavaScript, including Node.js, server-side JavaScript, NPM, Express.js, databases, authentication and authorization, testing and debugging, and deploying Node.js applications.
We started with an introduction to backend development and the importance of backend development in web development. Then, we covered the core concepts and tools used in backend development, including Node.js, Express.js, MongoDB, Mongoose, Passport.js, JWT, Mocha, Chai, and Heroku. We also discussed best practices for deploying Node.js applications to production and monitoring application performance.
Many resources are available for those interested in learning more about backend development in JavaScript, including online courses, tutorials, and documentation. Here are a few recommended resources:
- Node.js documentation
- MongoDB documentation
- Express.js documentation
- Passport.js documentation
- JWT documentation
- Mocha documentation
- Chai documentation
- Heroku documentation
Additionally, GUVI offers JavaScript Course. You can also join online communities like Stack Overflow, Reddit, or GitHub to connect with other developers and ask questions.
Dream big & kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Career Program with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Did you enjoy this article?