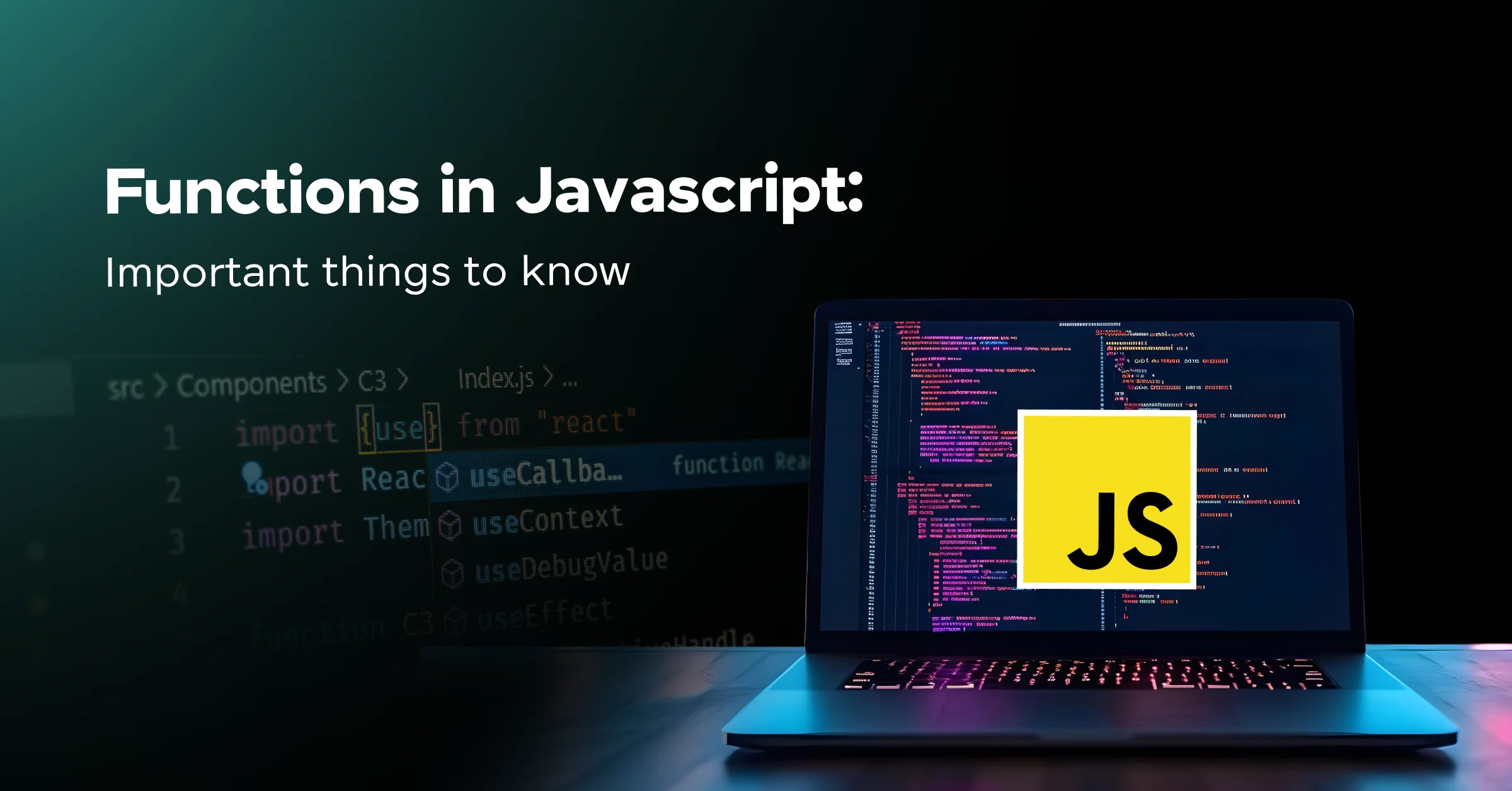
Functions in JavaScript: Important Things To Know [2024]
Mar 19, 2024 6 Min Read 1343 Views
(Last Updated)
Remember the popular saying “Get the fundamentals down and the level of everything you do will rise”, this applies to literally anything that you do. So, if you want to ace the field of JavaScript, the first step you need to do is to master the basics.
A key concept that many tend to ignore or not master is ‘functions’ in JavaScript. This is mainly because functions in JavaScript may seem to be easy on the outside but it leads you to confusion one too many times. It is important to understand it better.
In this article, you are going to learn about functions in JavaScript. By the end, you will have an updated sense of knowledge of JavaScript that boosts your morale towards full-stack development.
So, without further ado, let’s get started on JavaScript!
Table of contents
- What is a Function in JavaScript?
- Types of Functions in JavaScript
- Function Declarations
- Function Expressions
- Arrow Functions
- IIFE (Immediately Invoked Function Expressions)
- Async Functions
- Understanding Parameters and Arguments in JavaScript
- Parameters
- Arguments
- Why It Matters?
- Return Values in JavaScript Functions
- Understanding Return Values
- Why Return Values Matter
- Exploring the World of Higher-Order Functions in JavaScript
- What Are Higher-Order Functions?
- Why Use Higher-Order Functions?
- Examples of Higher-Order Functions in JavaScript
- Conclusion
- FAQs
- What are function expressions in JavaScript?
- What are anonymous functions in JavaScript?
- Can JavaScript functions be nested?
- What role does JavaScript play in Full Stack Development?
- Why is understanding functions important for JavaScript developers?
What is a Function in JavaScript?
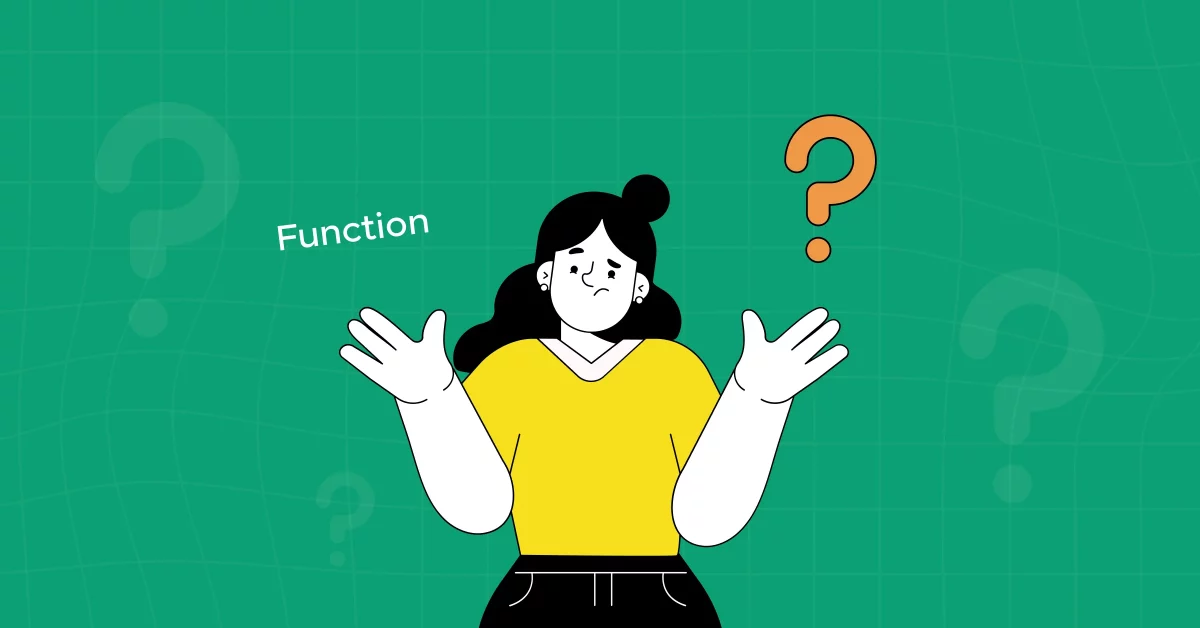
A function is a reusable piece of code that performs a specific task. Think of it as a small machine within your larger application; you feed it some input (though input is optional), and it processes that input to produce some output or perform an action.
Here’s a simple analogy to help you better understand functions in JavaScript: Imagine you have a recipe for a cake. Every time you want to bake this cake, you don’t need to look up the recipe again; you just follow the steps you’ve memorized.
In programming terms, the recipe is like a function, and baking the cake is like calling the function. You can “bake” as many cakes as you like once you have the “recipe”.
So, when you’re writing JavaScript, remember that functions help you structure your code in logical blocks, making it easier to read, maintain, and debug. Whether you’re just starting or you’re an experienced developer, mastering functions is key to becoming proficient in JavaScript.
Read More: 7 Best JavaScript Practices Every Developer Must Follow
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
Types of Functions in JavaScript
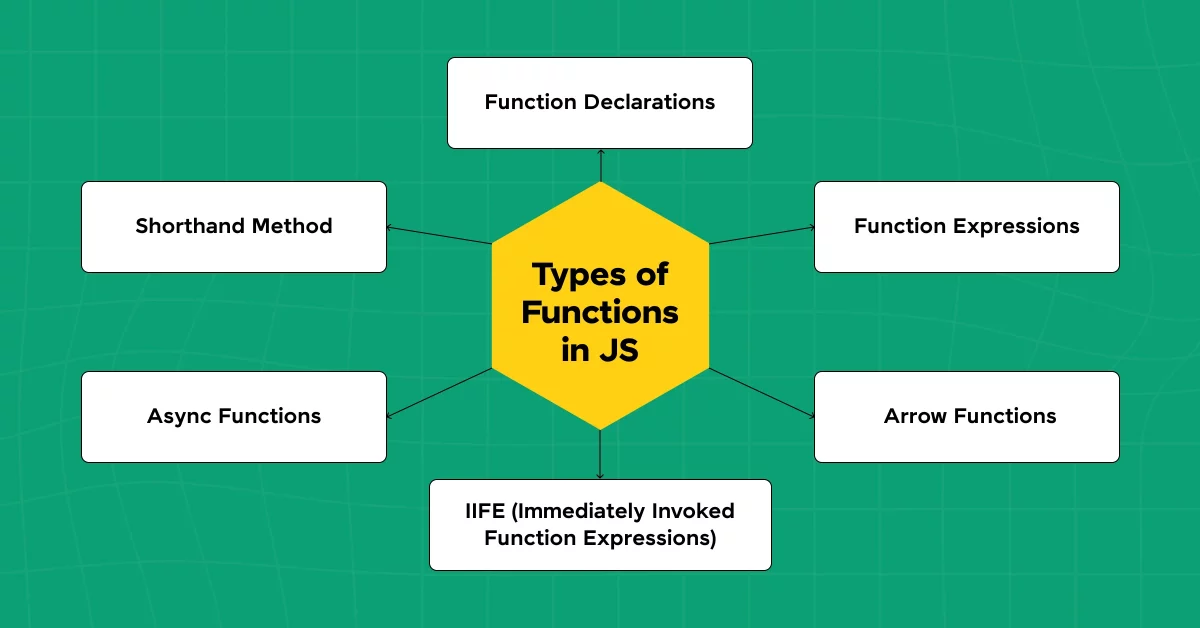
JavaScript, with its versatile nature, offers a variety of ways to create functions in JavaScript. Each type has its unique flavor and use case.
Let us see some basic types of functions in JavaScript:
1. Function Declarations
Think of function declarations as the standard way to define a function. They’re like telling a story from start to finish in one go.
You use the 'function'
keyword to define a function, followed by the name of the function, then parentheses '()'
that can enclose parameters, and finally, curly braces '{}'
that contains the function’s body.
Example:
function greet() {
console.log("Hello, world!");
} greet();
Output: “Hello, world!”
This is a straightforward, no-surprises way to create a function where you declare it straight away and you will get the required output. It’s hoisted, meaning you can call it before it’s defined in your code.
Also Explore: How to Create a Simple “Hello World” Page Using HTML
2. Function Expressions
Function expressions are more like assigning a task to a variable. You create an anonymous function (a function without a name) and assign it to a variable. These are not hoisted, so you need to define them before you can call them.
Example:
const sayGoodbye = function() {
console.log("Goodbye, world!");
};
sayGoodbye();
Output: “Goodbye, world!”
Here, you defined 'sayGoodbye'
as a function before using it in the code. This is the major difference between Function Declaration and Function Expressions.
Function expressions are flexible and can be used as values, including being passed as arguments to other functions.
3. Arrow Functions
Introduced in JavaScript ES6, arrow functions provide a more concise syntax for writing functions in JavaScript.
They are especially handy for one-liners and can omit the 'function'
keyword and 'return'
statement. Arrow functions have a lexical 'this'
value, meaning they inherit 'this'
from the parent scope at the time they are defined, not when they are called.
Example:
const add = (a, b) => a + b;
console.log(add(5, 3));
Output: 8
Arrow functions are great for short, simple functions, particularly as callbacks or function arguments.
4. IIFE (Immediately Invoked Function Expressions)
IIFEs are function expressions that run as soon as they are defined. They are enclosed in parentheses to avoid syntax errors and immediately invoked with another set of parentheses.
Example:
(function() {
console.log("IIFE is running!");
})();
Output: “IIFE is running!”
IIFEs are useful for creating private scopes and executing code immediately without polluting the global namespace.
5. Async Functions
An async function is a special type of function that makes dealing with asynchronous operations more comfortable and more readable.
The word “asynchronous” might sound complicated, but it simply means that something doesn’t happen immediately or in the direct sequence of commands.
When you declare a function as 'async'
, you’re telling JavaScript, “Hey, I know this task might take some time, so feel free to move on and do other things while waiting for this to finish.” This is incredibly useful in full-stack development, where waiting for data to load is a common task.
Example:
async function fetchUserData() {
let response = await fetch('https://api.example.com/user');
let data = await response.json();
console.log(data); // This will log the user data to the console.
}
fetchUserData();
In this example, 'fetchUserData'
is an async function. The 'await'
keyword is used to wait for the fetch operation to complete before moving on to the next line. This makes your code look and behave a bit more like synchronous code, even though it’s handling asynchronous operations.
Learn More: Best JavaScript Course Online with Certification
Understanding Parameters and Arguments in JavaScript
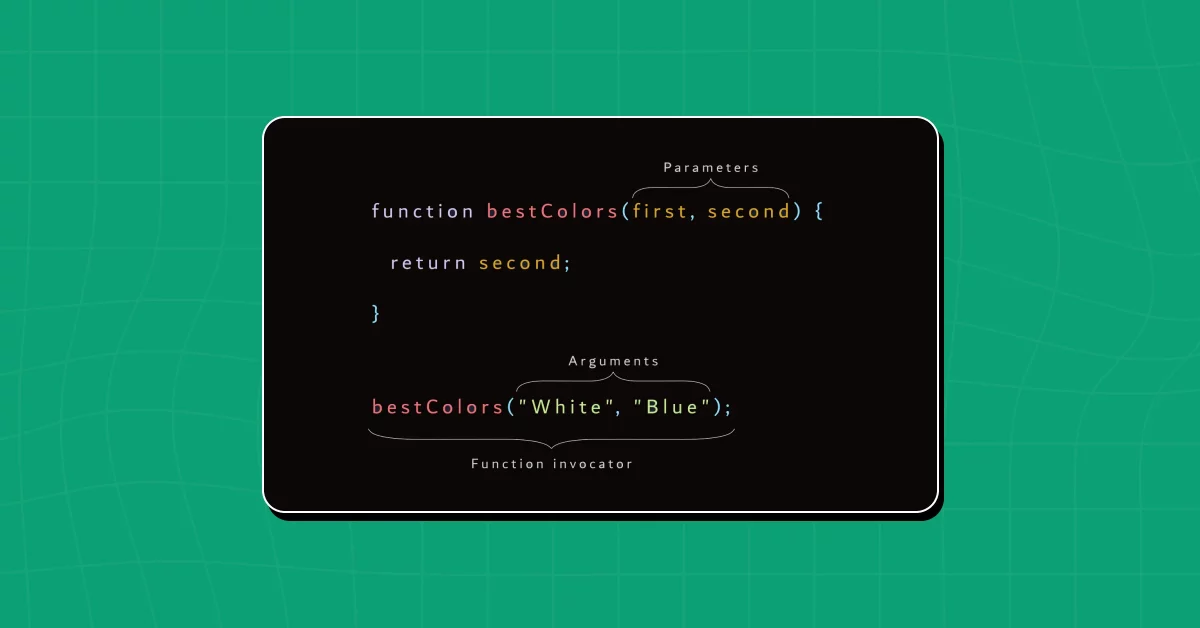
Let’s talk about another concept in functions in JavaScript that’s as fundamental as it is powerful—parameters and arguments.
If you’re just starting, these terms might seem a bit daunting, but fear not! This section will prove to be helpful for your better understanding.
Imagine you’ve got a magic box (a function in JavaScript) that can transform anything you put inside it. However, for the magic to work, you need to tell the box what to transform. This is where parameters and arguments come into play in functions in JavaScript.
1. Parameters
Parameters are like placeholders or variables that you define when you create your function. They specify what kind of information the function needs to work its magic.
When you create a function, you’re essentially telling it, “Hey, prepare to receive some information when you’re called, and here’s what I’ll call that information.”
Here’s a simple example:
function multiply(x, y) {
return x * y;
}
In this 'multiply'
function, x
and y
are parameters. They tell the function, “Expect two pieces of information when you’re called, and use these to perform the multiplication.”
2. Arguments
Arguments in functions in JavaScript, on the other hand, are the actual values you provide to the function when you call it.
They’re what you put in the magic box to transform. Using the same ‘multiply'
function, when you call it with specific numbers, those numbers are the arguments.
let result = multiply(5, 3);
Here, 5
and 3
are arguments. They’re the real data passed into the function, replacing the placeholders x
and y
, and allowing the function to execute its task and return 15
, the product of 5
and 3
.
Must Read: 4 Key Differences Between == and === Operators in JavaScript
Why It Matters?
Understanding the distinction between parameters and arguments is crucial because it helps you grasp how functions in JavaScript work and how data flows through them.
Parameters define the blueprint of the interaction, setting up what kind of data a function can accept. Arguments are the actual data, making the function do something specific with that data.
This concept is at the heart of writing dynamic functions in JavaScript that can perform a wide range of tasks with different inputs.
If you want to gain mastery in JavaScript, then hands-on practice is a must. Consider trying
GUVI’s Webkata, a front-end practice platform that provides you with an innovative way to learn full-stack development by a series of tasks.
Explore Master Backend Development With JavaScript | Become a Pro
Return Values in JavaScript Functions
Till now you’ve learned how to declare functions, call functions, and even learned about parameters and arguments in this article on functions in JavaScript.
Still, there’s one more crucial concept that turns these functions into JavaScript, a powerful tool for coding: Return Values.
Understanding Return Values
Imagine you’ve sent a letter to a friend asking for a recipe. When your friend replies, the letter you receive back is the “return value” of your request.
Functions in JavaScript work similarly. A return value is what a function gives back to you after it completes its task. It’s the outcome of all the operations the function has performed with the inputs (arguments) you provided.
Here’s an example:
function addNumbers(a, b) {
return a + b;
}
In this simple 'addNumbers'
function, a
and b
are added together, and the result is sent back using the 'return'
statement. When you call this function with two numbers, it “returns” their sum.
let sum = addNumbers(5, 7);
console.log(sum); // This will log '12' to the console.
Here, 12
is the return value of addNumbers(5, 7)
.
Why Return Values Matter
Return values are a fundamental part of functions in JavaScript because they let you capture the results of function operations.
Without a return value, a function might do something internally, but you won’t be able to access the outcome of its work.
By using return values, you can:
- Store the result of a function in a variable, just like we stored the sum in the
'sum'
variable. - Use that result as an input for another function, chaining functions together to perform more complex operations.
- Make decisions in your code based on what a function returns.
Explore: Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
Exploring the World of Higher-Order Functions in JavaScript
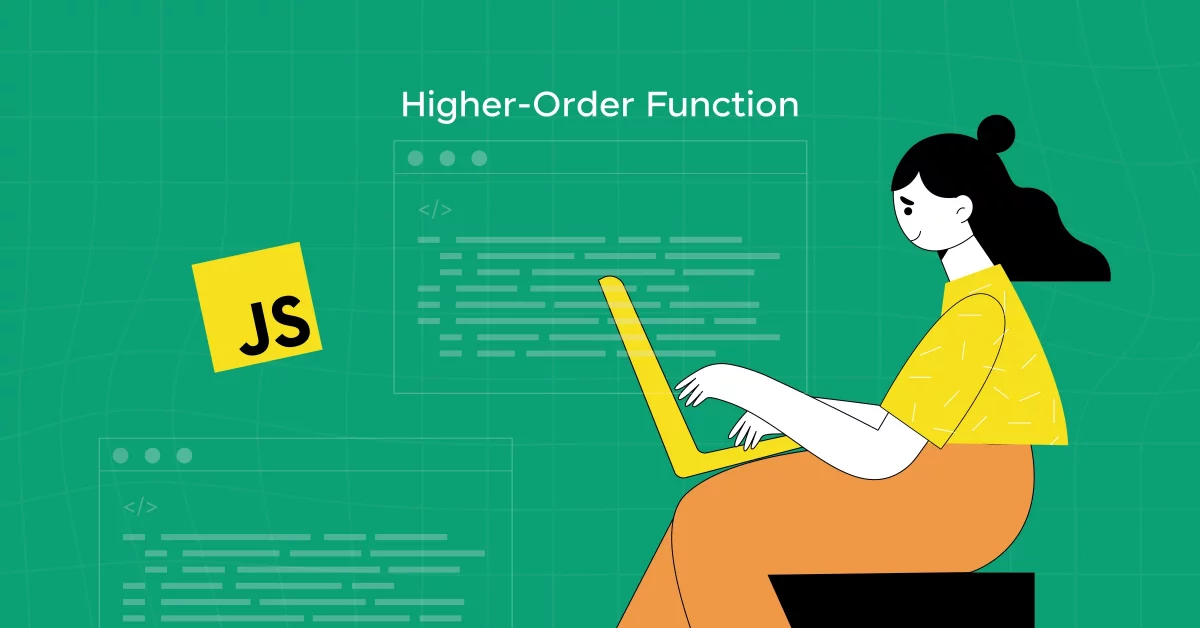
As a bonus section, we introduce you to the concept of higher-order functions in JavaScript that lets you do wonders, and knowing this will put you one step ahead of other fellow JavaScript enthusiasts.
What Are Higher-Order Functions?
To explain the concept of higher-order functions in JavaScript simply, let us imagine that you have a robot that can perform tasks for you.
Now, imagine you can give this robot another smaller robot that does a specific task, and the first robot uses this second robot to complete a bigger job. In programming, particularly in JavaScript, higher-order functions work somewhat like this first robot.
A higher-order function is a function that can take another function as an argument, or return a function as its result, or even both!
It’s like a function that deals with other functions, either by taking them in to process data or spitting out new functions ready to be used later.
Also Read: Arrays in JavaScript: A Comprehensive Guide
Why Use Higher-Order Functions?
You may ask a question, why use higher-order functions in JavaScript when we already have plenty of functions?
The beauty of higher-order functions lies in their ability to make your code more modular, more readable, and easier to debug and maintain.
They allow you to write cleaner code by abstracting actions, meaning you can create a function that performs a specific task and then pass it around to be used by other functions.
Examples of Higher-Order Functions in JavaScript
A classic example is the 'Array.prototype.map()'
function in JavaScript. This is a higher-order function because it takes another function as its argument.
The function you pass to 'map()'
is applied to every element in an array, transforming the array into something new:
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(number => number * 2);
console.log(doubled); // Output: [2, 4, 6, 8]
In this example, 'map()'
takes a simple function (number => number * 2
) that doubles a number. It then applies this function to every item in the 'numbers'
array, producing a new array with each number doubled.
Another great example is the 'Array.prototype.filter()'
function, which filters an array based on the condition defined in the function passed to it:
const numbers = [1, 2, 3, 4];
const even = numbers.filter(number => number % 2 === 0);
console.log(even); // Output: [2, 4]
Here, 'filter()'
takes a function that checks if a number is even and then applies this function to filter out the odd numbers from the numbers
array.
This is how higher-order functions in JavaScript work making sure the code is more modular and easy to understand.
Know More: 42 JavaScript Questions Towards Better Interviews
If you want to learn more about functions in JavaScript and their implementation, then you must sign up for GUVI’s Certified Full Stack Development Course, which gives you in-depth knowledge of the practical implementation of Javascript through various real-life FSD projects.
Conclusion
In conclusion, throughout this article on functions in JavaScript, we’ve covered everything from the basics, like defining and calling functions, to more complex concepts such as function expressions, parameters, arguments, and return values.
We’ve also dived into advanced topics like higher-order functions, async functions, highlighting the flexibility and power of JavaScript for handling a wide range of tasks.
Through this journey, you’ve seen how functions are the building blocks of JavaScript, crucial for writing clean, efficient, and maintainable code. Understanding functions in depth helps you to unlock the full potential of JavaScript, making your coding journey both more productive and enjoyable.
Must Explore: 7 Best Reasons to Learn JavaScript | 1 Bonus Point
FAQs
1. What are function expressions in JavaScript?
Function expressions involve creating a function and assigning it to a variable. Unlike function declarations, expressions are not hoisted.
2. What are anonymous functions in JavaScript?
Anonymous functions are functions without a name. They are often used as arguments to other functions or as immediately invoked function expressions (IIFE).
3. Can JavaScript functions be nested?
Yes, functions can be nested within other functions, and the inner function can access the variables of the outer function (creating a closure).
4. What role does JavaScript play in Full Stack Development?
JavaScript is crucial in FSD as it enables developers to build interactive and dynamic web applications. It’s used both on the client-side (frontend) and server-side (backend), making it a versatile language for full stack developers.
5. Why is understanding functions important for JavaScript developers?
Functions are the primary means of executing code and organizing a JavaScript application, making them fundamental for tasks ranging from UI interactions to server-side logic.
Did you enjoy this article?