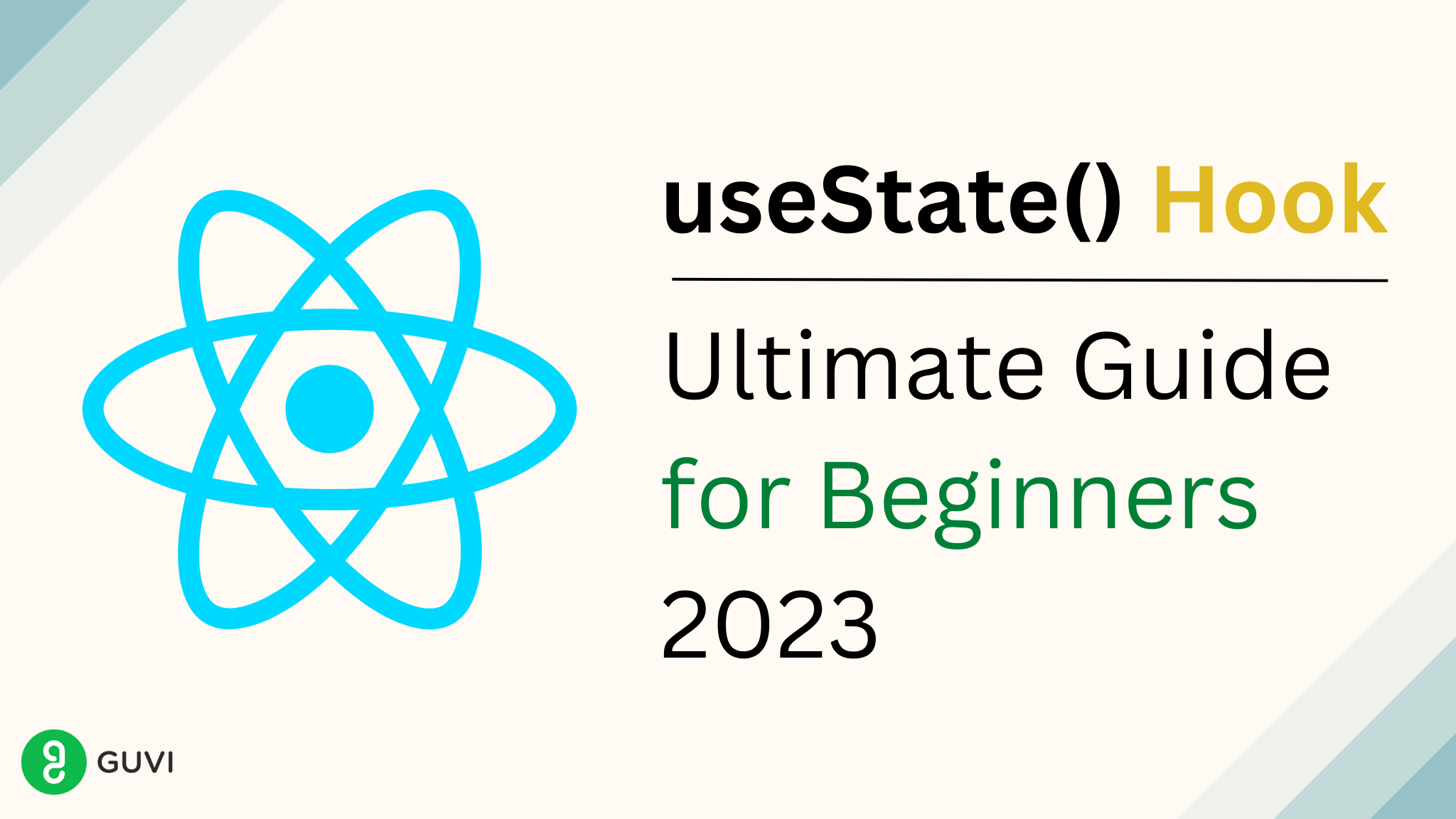
useState() Hook in React for Beginners | React Hooks 2024
Mar 25, 2024 4 Min Read 2407 Views
(Last Updated)
State is a way to store and manipulate data that controls the behavior and rendering of a component in react. In this blog, we will be focusing on the useState() Hook, which is a built-in way to add state to functional components.
If you’re new to React, you might be wondering why we’re talking about functional components instead of class-based components. This is because functional components are easy and simpler to understand than class-based components, and React’s Hooks API is designed to make it easier to manage state and disadvantages in these types of components.
A React Hook is a JavaScript function that allows you to use state and other React features in functional components, instead of having to use class-based components. Hooks allow you to reuse stateful logic across your components without having to re-write the same code or change the component hierarchy.
Hooks are of 2 types: built-in Hooks and custom Hooks. Built-in Hooks, such as useState, useEffect, are provided by React and are used to manage state, side effects, and context in functional components whereas custom hooks are user-defined Hooks that allow you to reuse stateful logic across multiple components
Table of contents
- What is a useState() Hook
- Syntax
- Explanation:
- Usage
- Import the useState hook
- Adding state to a component
- Updating state based on the previous state
- Using Multiple State Variables
- Last words
What is a useState() Hook
useState() hook in react allows you to add state to functional components. It returns an array consisting of two elements: the current state and a function to update it. The first time the component is rendered, the initial state is passed as the argument to useState.
Syntax
import React, { useState } from ‘react’
const [count, setCount] = useState(initialState);
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. React is a major part of the FSD curriculum.
If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Career Program with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced certification course.
Explanation:
You might have thought, what has been done above in the syntax of useState hook. Don’t worry! Let’s understand the syntax in detail.
Import
To use the useState hook, we first import it into our component as seen above.
Parameters
- initialState: This is the value that you want the state to be initially. It can be a value of any type, but there is a special behavior for functions.
- Now, when we pass a function as initialState, it will be called as an initializer function. This must be pure, must not take any arguments, and must return a value of any type. React will call your initializer function when initializing the component, and store its return value as the initial state.
Returns
Here, useState is a Hook that is imported from the React library. It is called with the initial value of the state that you want to add to the component. useState returns an array with two elements: the current state and a function to update it.
- The first element of the array is the current state, which is stored in a variable. This variable can be named anything, but it’s common to use the same name as the state you’re storing, such as count.
- The second element of the array is the function that updates the state, which is stored in another variable. This variable is commonly named with the same name as the state and “set” as a prefix, such as setCount.
Usage
Let’s understand that how we can use the useState hook in a detailed manner.
Import the useState hook
For using the useState hook, we’ll first need to import it. To import the hook, just write the following code at start of your react app:
import React, { useState } from "react";
Or
import { useState } from "react";
Note: Above in the second statement, we are destructuring useState from react as it is a named export.
Adding state to a component
Now we have imported our hook from react and let’s see how it is added & initialized to a component. For initialization a state we call it in our functional component. As discussed in the syntax above, it accepts an initial state and return two values:
- A current state
- A function to update it
Initialize state at the top of the function component as show below:
import { useState } from "react";
function App() {
const [ count, setCount ] = useState(0);
}
Here, we have count as our initial state variable and setCount as a function to update the state. Finally, we have set our initial state as 0. ( useState(0) )
Updating state based on the previous state
To update state based on the previous state in React, you can use a function that takes in the previous state as an argument, instead of directly passing the new state.
Here’s an example of how you might update state based on the previous state using useState() hook:
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(prCount => prCount + 1)}>
click
</button>
</div>
);
}
In this example, we are using an updater function that takes in the previous state (prCount) as an argument, and returns the updated state (prCount + 1). With this pattern, you can ensure that the state updates correctly, even if multiple updates are made at one time because the function is called with the current value of the state, ensuring that it always has access to the current state when finding the new value.
Using Multiple State Variables
To use multiple state variables in a functional component using the useState Hook, we simply need to call the hook multiple times, once for each piece of state that we want to add. Each call to useState will return a separate array containing the current state and a function to update it.
function manyStateDeclare() {
const [ name, setName ] = useState(‘GUVI’);
const [ age, setAge ] = useState(23);
const [ count, setCount ] = useState(0);
…
}
In the above component, we have name, age, and count as different state variables, and we can update them individually with different functions. We don’t have to use many state variables as they can hold objects and arrays.
Last words
Concluding the blog, the useState Hook is a powerful tool in React that allows you to add state to components. It provides a simple and consistent way to manage state, and it also allows you to reuse stateful logic across your components. By understanding the basics of how useState() hook works, we can now start building more dynamic and interactive UI with React.
It’s important to remember that the state updates are asynchronous and batched and we can use multiple state variables in a component by calling useState hook multiple times. By keeping these things in mind, you’ll be well on your way to mastering state management in React with the useState() Hook.
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Career Program with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced course.
So, what are you waiting for? Hurry up!
Did you enjoy this article?