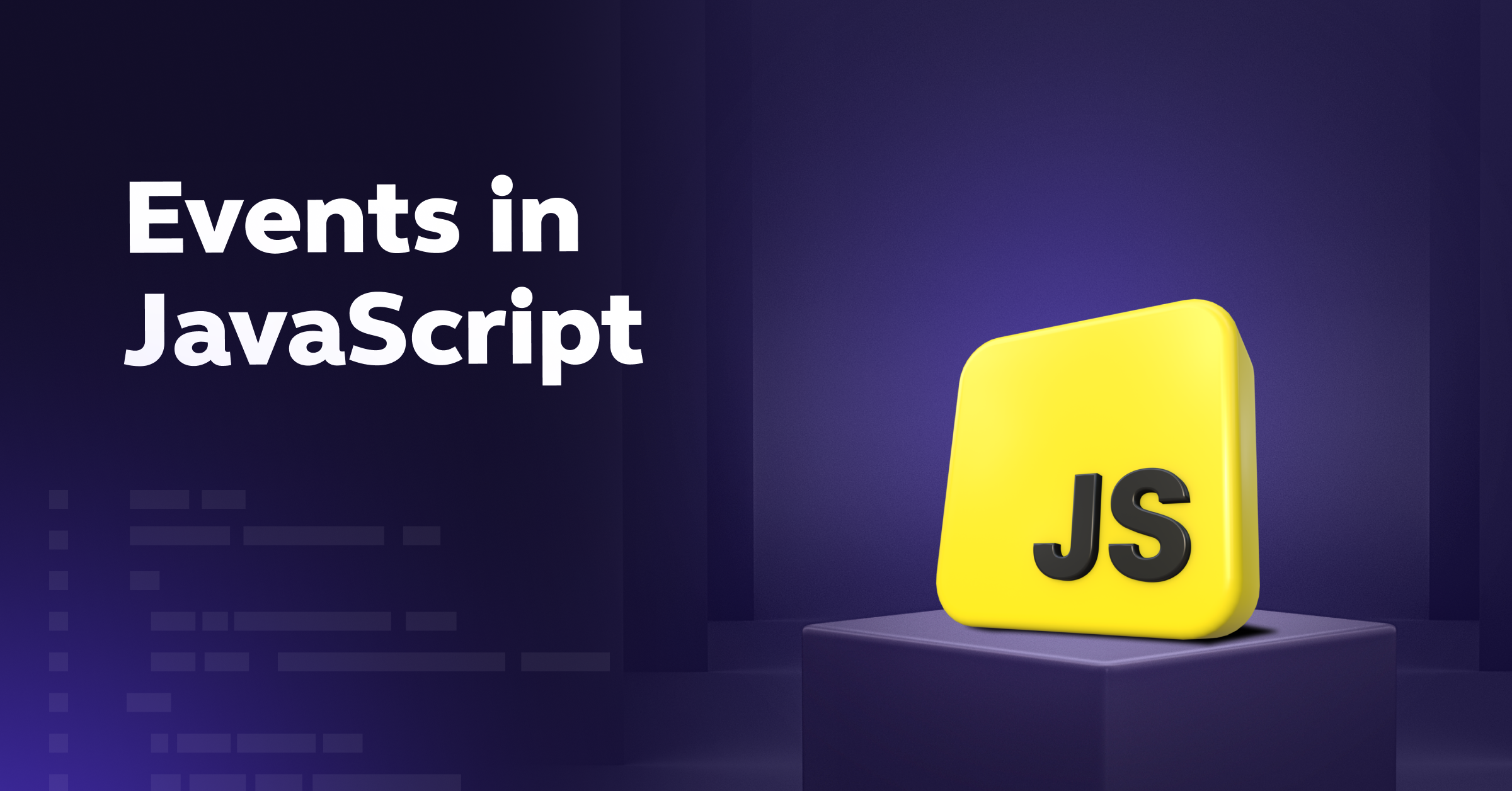
What are Events in JavaScript? A Complete Guide
Mar 28, 2025 5 Min Read 11456 Views
(Last Updated)
When you interact with a website—like clicking a button, moving your mouse over an image, or typing on your keyboard—things happen on the screen. These actions are called events. In JavaScript, events are what make websites respond to what you do. Events tell the website when to do something based on what you’re doing. Understanding events is very important if you want to create websites that feel alive and respond to users.
In this blog, we’re going to break down events in JavaScript into easy-to-understand pieces. Let’s see how events work in JavaScript!
Table of contents
- What is a JavaScript Event?
- How to Create an Event in JavaScript?
- Types of Events in JavaScript
- A. Onclick Event
- B. Onekeyup Event
- C. Onmouseover Event
- D. Onload Event
- E. Onfocus Event
- JavaScript Event Phases
- Event Capturing
- Event Targeting
- Event Bubbling
- Conclusion
- FAQs
- What are JavaScript events, and how do they work?
- What are some common types of JavaScript events and their use cases?
- What is event propagation, and how does it work in JavaScript?
What is a JavaScript Event?
A JavaScript event is a specific action that occurs within a web page or application, such as clicking on an element, moving the mouse, pressing a key, or loading a page. Essentially, events are like signals that tell the browser to execute certain code or perform specific actions in response to user interactions or other occurrences.
Also, Find Out the Best JavaScript Roadmap Beginners Should Follow
Events in JavaScript are triggered by various interactions or system-generated actions. When an event occurs, it is handled by event listeners or event handlers, which are functions specifically written to respond to particular events.
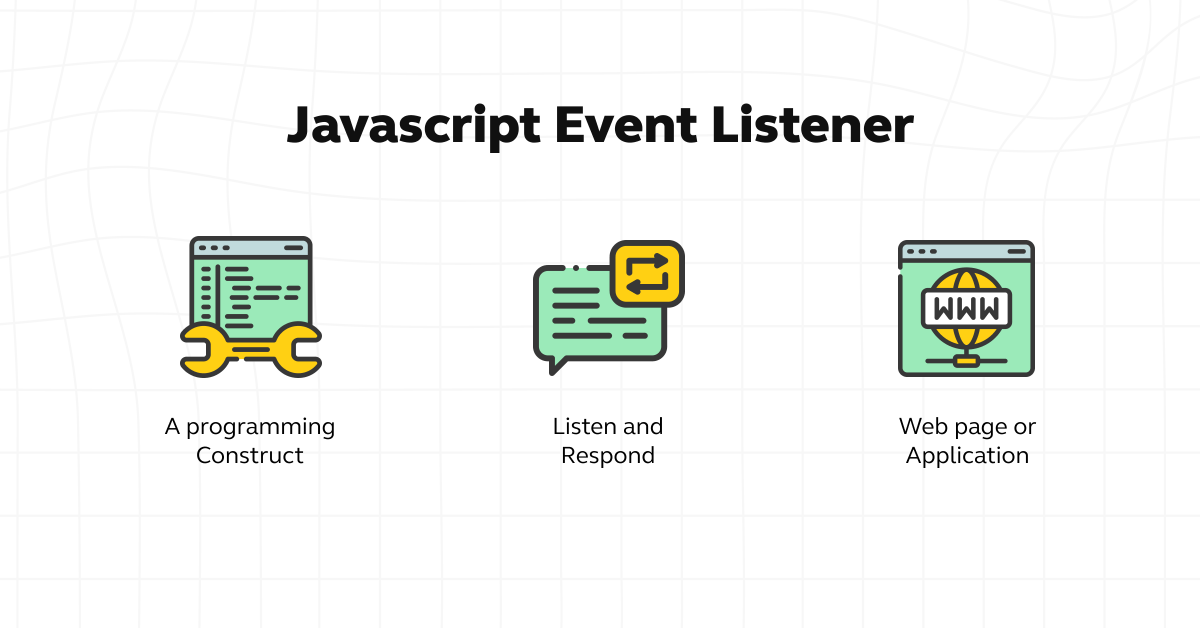
Here’s a simplified breakdown of how events work in JavaScript:
- Event Triggering: Events can be triggered by user interactions (like clicking, hovering, typing) or by the browser itself (like when a page finishes loading).
- Event Handling: To respond to these events, developers write event handler functions. These functions contain the instructions or code that should run when the event occurs.
- Event Binding: Event handlers are then bound or attached to specific elements or objects on the webpage using JavaScript methods like addEventListener() or by directly assigning functions to event attributes in HTML.
- Event Execution: When the specified event occurs, the associated event handler function is executed, allowing the webpage to respond dynamically to user actions or other events.
Understanding how events are triggered and handled in JavaScript is fundamental to building interactive and responsive web applications. It enables developers to create engaging user experiences by customizing how their websites respond to user inputs and other events.
Also Read: 42 JavaScript Questions Towards Better Interviews
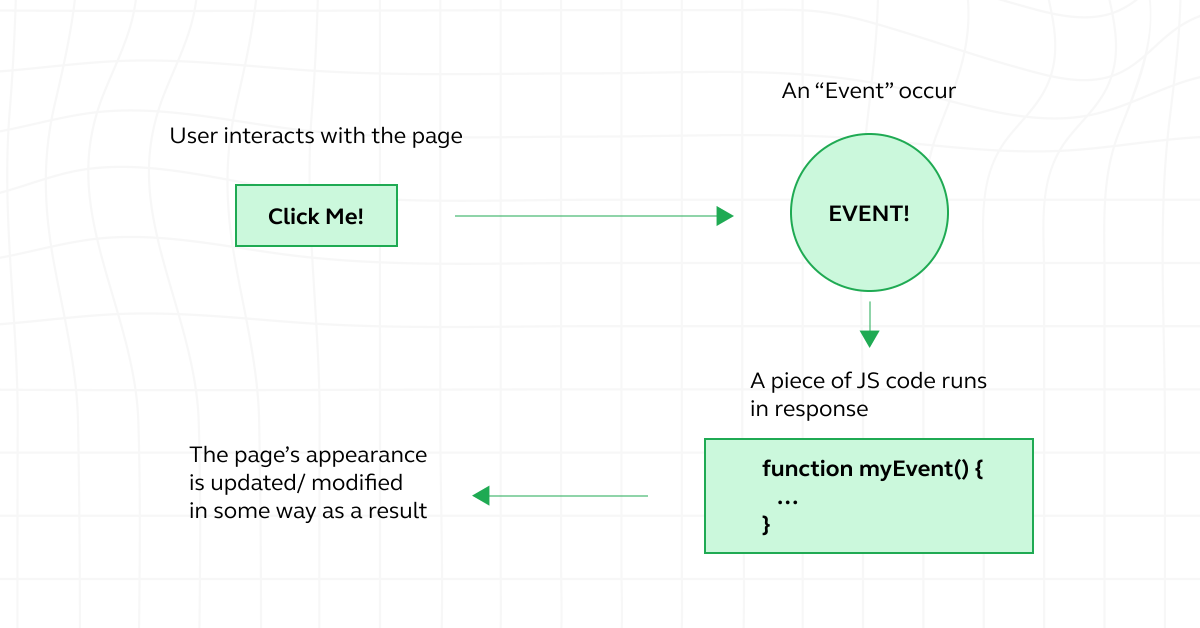
Now that we’ve talked about what a JavaScript event is and why it’s important for making websites interactive, let’s move on to creating your events in JavaScript.
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
How to Create an Event in JavaScript?
The following is a step-by-step guide to creating custom events:
- Event Initialization: Begin by initializing a new event object using the Event() constructor. This object represents the custom event you want to create.
- Event Configuration: Configure the event object by specifying its type. The event type is a descriptive string that identifies the custom event you’re creating. You can also provide additional options, such as whether the event bubbles up through the DOM hierarchy or can be canceled.
- Dispatching the Event: Once the event object is configured, you can dispatch it to trigger the custom event. Dispatching an event involves specifying the target element or object where the event should originate from and calling the dispatchEvent() method on that target.
Also Read: JavaScript Tools Every Developer Should Know
Demonstrative Code Examples for Clarity
// Step 1: Event Initialization
var customEvent = new Event(‘customEvent’);
// Step 2: Event Configuration
// You can also pass additional options as the second argument, such as { bubbles: true, cancelable: true }
customEvent.initEvent(‘customEventType’, true, true);
// Step 3: Dispatching the Event
var targetElement = document.getElementById(‘targetElement’);
targetElement.dispatchEvent(customEvent);
In this example:
- We first initialize a new event object named customEvent using the Event() constructor.
- Then, we configure the event object by calling the initEvent() method, specifying the event type (‘customEventType’) and additional options (e.g., whether the event bubbles and is cancelable).
- Finally, we dispatch the custom event on a target element with the ID ‘targetElement’ using the dispatchEvent() method.
By following these steps, you can create custom events in JavaScript and trigger them as needed within your web applications. Custom events are particularly useful for creating modular and extensible code, allowing different parts of your application to communicate with each other through event-driven architecture.
Also Read: The Beginner’s Guide To Closures In JavaScript
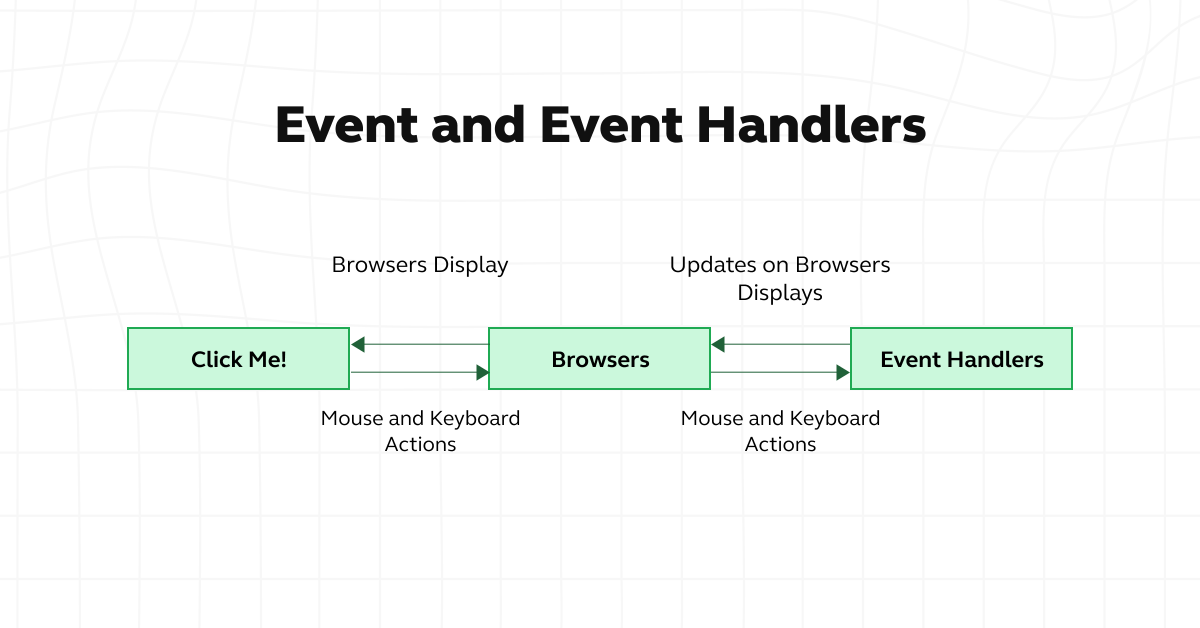
Now that we’ve covered how to create events in JavaScript, let’s shift our focus to the various types of events you can use to enhance your web applications.
Types of Events in JavaScript
The various types of events in JavaScript are as follows:
A. Onclick Event
- The onclick event occurs when a user clicks on an HTML element. It’s one of the most commonly used events in JavaScript.
- Use onclick to trigger actions like submitting a form, displaying a pop-up, navigating to a new page, or toggling visibility of elements.
- Example:
<button onclick=”alert(‘Button clicked!’)”>Click me</button>
B. Onekeyup Event
- The onekeyup event triggers when a user releases a key after pressing it down. It’s useful for capturing user input from keyboard interactions.
- Use onekeyup to perform live search filtering, validate input fields, or trigger actions based on specific key combinations.
- Example:
document.getElementById(‘searchInput’).addEventListener(‘keyup’, function(event) {
console.log(‘Key released:’, event.key);
// Perform search operation or other actions based on user input
});
C. Onmouseover Event
- The onmouseover event occurs when the mouse pointer moves onto an element, triggering an action.
- Use onmouseover to create interactive elements like tooltips, image galleries with hover effects, or menu dropdowns.
- Example:
<img src=”image.jpg” onmouseover=”showTooltip(‘Hover over me!’)” onmouseout=”hideTooltip()”>
Also Read: 7 Best Reasons to Learn JavaScript | 1 Bonus Point
D. Onload Event
- The onload event is fired when a web page or an element has finished loading completely.
- Use onload to initialize scripts, fetch dynamic content, or execute actions that depend on the page’s full rendering.
- Example:
window.onload = function() {
console.log(‘Page loaded’);
// Initialize page content or perform other actions
};
E. Onfocus Event
- The onfocus event is triggered when an element gains focus, typically through user interaction like tabbing or clicking.
- Use onfocus to enhance usability by providing visual feedback, validating input fields, or dynamically updating content.
- Example:
<input type=”text” onfocus=”this.style.backgroundColor=’lightblue'” onblur=”this.style.backgroundColor=’white'”>
Understanding these types of events in JavaScript empowers developers to create dynamic and interactive web applications that respond seamlessly to user actions and inputs. Events play an important role in shaping user experiences on the web.
Also Read: 4 Key Differences Between == and === Operators in JavaScript
Having explored the various types of events in JavaScript, which range from user-initiated actions like clicks and keypresses to system-generated events such as load and resize, let’s learn about an equally important aspect of JavaScript event handling: the event phases.
JavaScript Event Phases
Let’s explore JavaScript event phases:
1. Event Capturing
Event capturing is the first phase in the event flow process where the event is captured or detected at the highest level of the DOM hierarchy and then propagates down to the target element. Event capturing allows developers to intercept events before they reach their target, enabling global event handling and delegation. Practical use cases include implementing event delegation, validating user input, and applying consistent event handling across multiple elements.
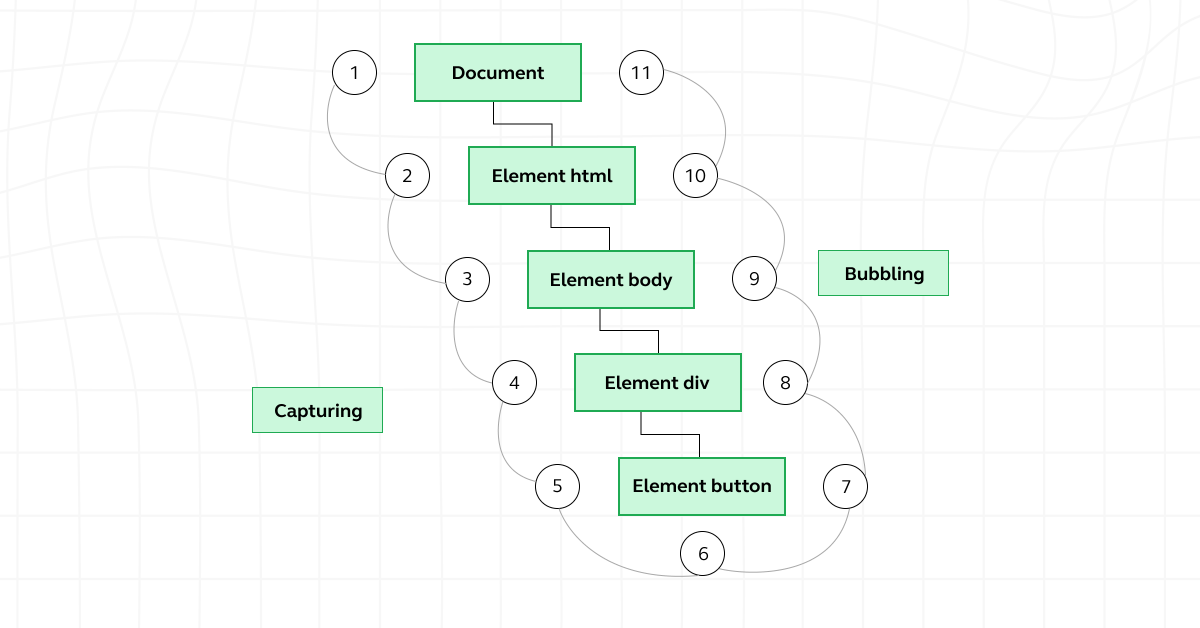
2. Event Targeting
Event targeting, also known as the “at target” phase, occurs when the event reaches the target element that triggered it. During the targeting phase, event handlers attached directly to the target element are executed, allowing for specific actions or behaviors to be applied based on the event.
Also Read: 7 Best JavaScript Practices Every Developer Must Follow
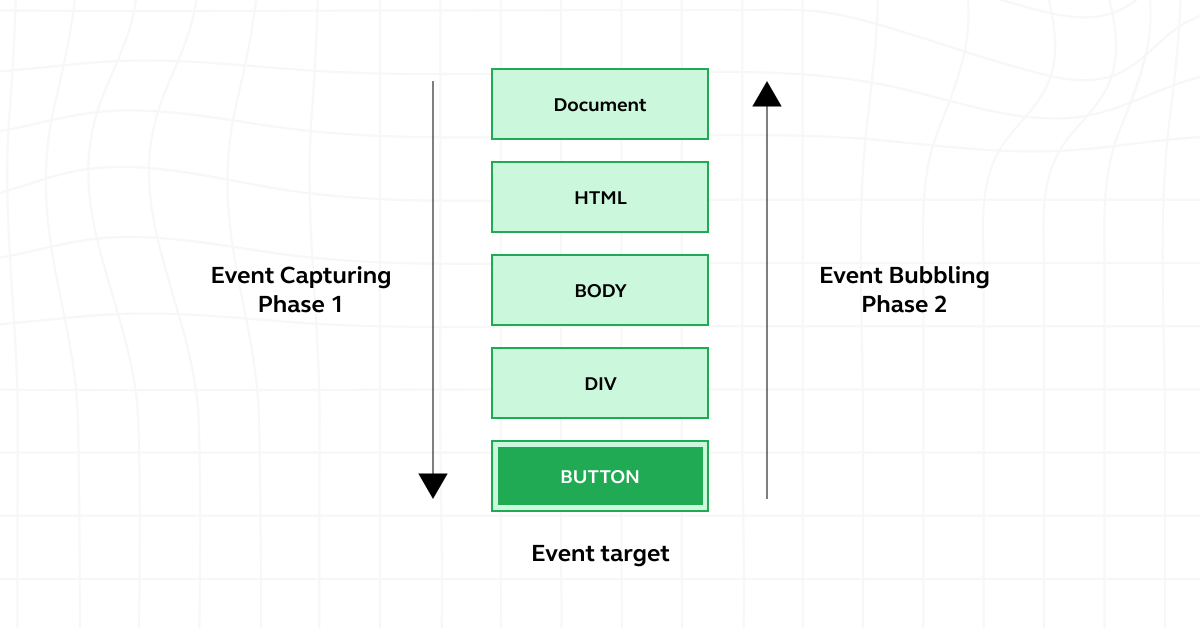
3. Event Bubbling
Event bubbling is the final phase in the event flow process where the event, having been processed at the target element, propagates back up through the DOM hierarchy to the root element. Unlike event capturing, which starts from the top of the DOM tree and moves downward, event bubbling starts at the target element and moves upward. Practical implications include simplifying event handling by allowing for more concise code, enabling event delegation, and facilitating the creation of interactive components.
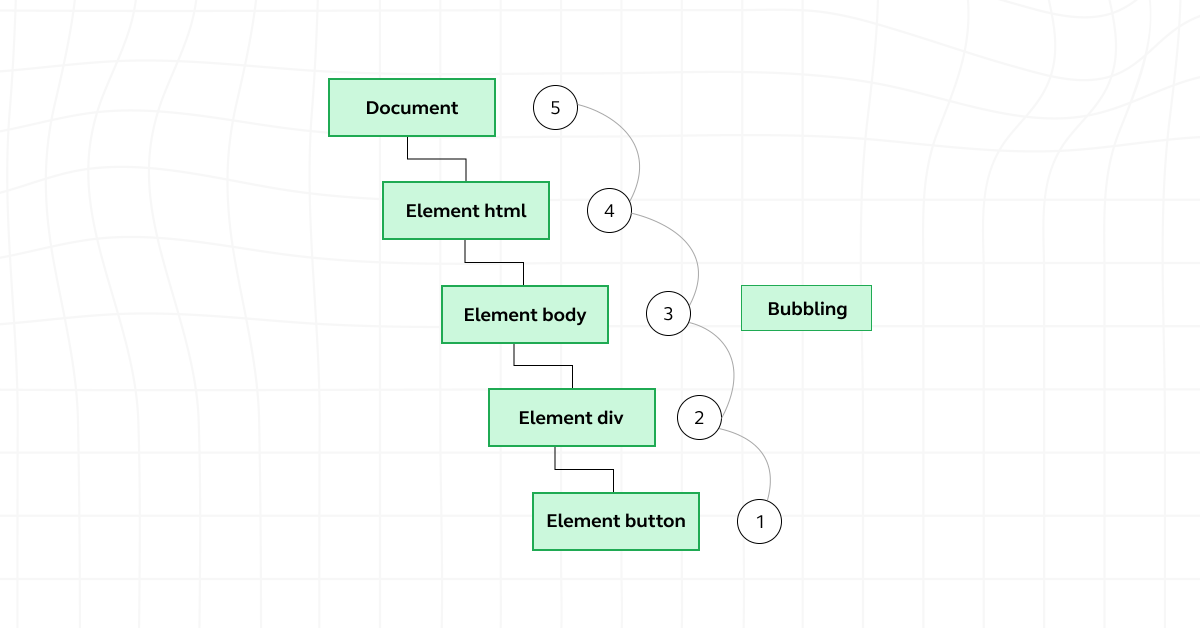
Understanding these event phases in JavaScript is important for effective event handling and manipulation. Mastering event capturing, targeting, and bubbling provides you with powerful tools to create dynamic and responsive web applications.
Ready to boost your web development career? Join GUVI’s Full Stack Development Course! Learn both front-end and back-end skills through easy-to-follow projects and expert tips. This course covers everything from basics to advanced web apps. Enroll today!
Conclusion
JavaScript events play an important role in modern web development, and mastering them is essential for creating dynamic, interactive, and user-friendly applications. So, keep learning, experimenting, and practicing, and you’ll continue to grow as a proficient web developer. All the best!
Also Read: 30 Best JavaScript Project Ideas For You [3 Bonus Portfolio Projects]
FAQs
JavaScript events are actions or occurrences that happen in the browser, such as a user clicking a button, hovering over an element, or a page finishing loading.
These events trigger JavaScript code to execute, allowing developers to create interactive and dynamic web applications. Events are handled using event listeners or event handlers, which are functions that respond to specific events when they occur.
Common types of JavaScript events include onclick, onmouseover, onkeydown, onload, and many more. Each event type corresponds to a specific user action or system-generated event.
Event propagation refers to the process by which events propagate or travel through the DOM hierarchy.
There are two main phases of event propagation: event capturing and event bubbling.
1) During event capturing, the event travels from the outermost ancestor element down to the target element. After reaching the target element, the event enters the event targeting phase, where event handlers attached directly to the target element are executed.
2) Finally, during event bubbling, the event travels back up the DOM hierarchy from the target element to the outermost ancestor element. Understanding event propagation is essential for managing event handling efficiently and avoiding unexpected behavior in complex web applications.
Did you enjoy this article?