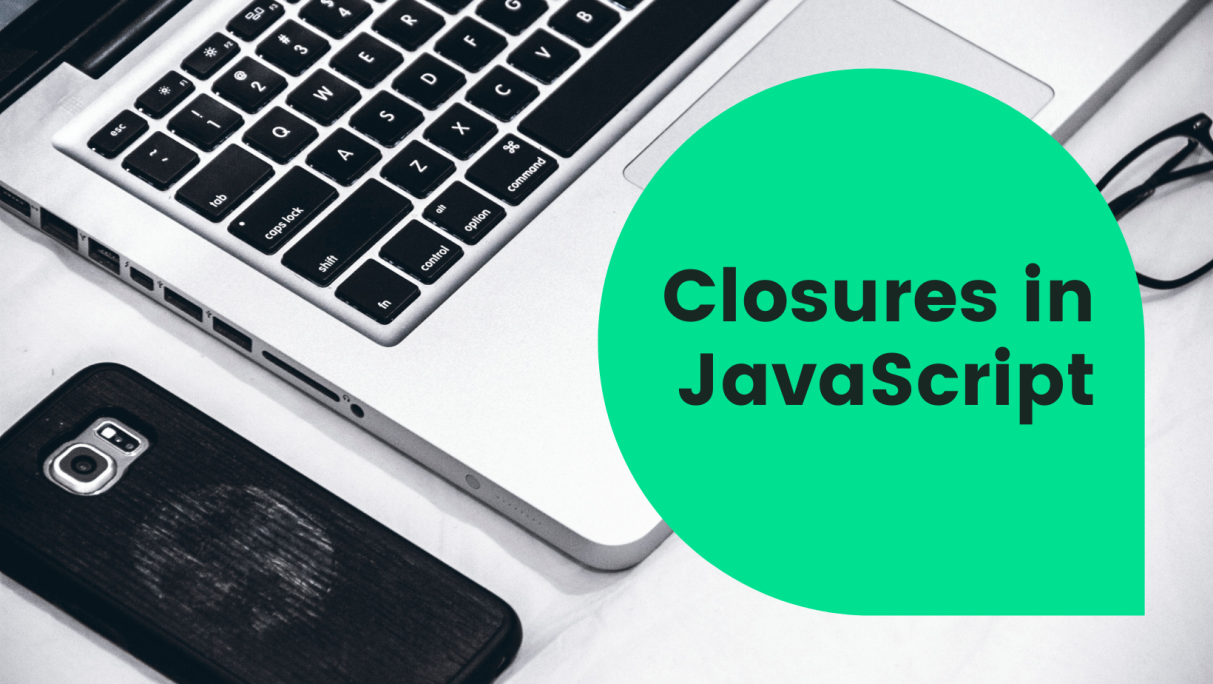
The Beginner’s Guide To Closures In JavaScript
Mar 21, 2024 4 Min Read 889 Views
(Last Updated)
If you’re a beginner and wondering what are closures in JavaScript, you’ve come to the right place. In this blog, we’ll be talking about closures in JavaScript. Let’s understand more about its history, advantages, and some examples to understand its functioning. They are crucial in functional programming, and hence they’re something that is frequently asked about during the JavaScript coding interview.
Without much ado, let’s read about it:
Table of contents
- What are Closures?
- What is Lexical Scope?
- Closures in JavaScript
- Closures in JavaScript Simplified For You
- Running the Code
- When to Use Closures in JavaScript?
- Emulating Private Methods
- Maintaining States Between Function Calls
- Functional Programming
- Create Iterators
- Conclusion
- FAQs on Closures of JavaScript
- Q1. Closures can be used for performing which of the following operations?
- Q2. JavaScript uses which type of scoping?
- Q3. Which of the following is mandatory to implement lexical scoping?
- Q4. JavaScript is a/an ______ language?
- Q5. What will be the output of the following JavaScript code?
What are Closures?
Closures are one of the very basic building blocks in JavaScript. Undoubtedly, every JavaScript developer must have come across closures! And would have used Closures knowingly or unknowingly. The callbacks, higher-order, and event handler functions can access variables from the outer scope, thanks to closures. To understand closures, one first needs to understand Lexical Environment (or Lexical Scoping).
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Career Program with placement assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you would like to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced certification course.
What is Lexical Scope?
A Lexical scope in JavaScript means that variables defined outside of a function can be accessed inside of another function that was defined after the declaration of the variable. However, variables defined inside a function will not be accessible outside that function. Every function in JavaScript maintains a link to its outer lexical environment that maps all the names (E.g. variables, parameters) within a scope, along with their values.
In the above-written code, JavaScript searches for values a and b. It begins to look locally and goes outside until it finds what it is looking for. However, if we remove the variable declaration of ‘a’ in line no. 1, JavaScript will not go inside the multiply function and will throw an Undefined Error.
This is known as a Lexical scope. We can determine the scope of a component by the location of this component within the code.
Closures in JavaScript
Now, coming to the main question, What are closures and how do they work?
According to the definition given on MDN (Mozilla Developer Network) Web Docs,
“Closures are the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment).”
However, this is not the simplest definition and hence, hard to understand by beginners so let’s break this down in simpler words:
A closures function gives access to an outer function’s scope from an inner function. It is the combination of a function and the surrounding state (lexical environment) within which that specific function was declared. Now, the lexical environment consists of all the local variables that were inside scope at the time the closures were made. We can create a closures function by defining a function inside another one. And then exposing it by returning or passing it to another function.
The function declared inside will have access to all the variables in the function from the outer scope, even after the outer function is done executing.
Closures in JavaScript Simplified For You
In the example of the closures above, we have defined a function multiply(a) that returns an anonymous function. The function it returns takes a single argument b and returns the multiplication of a and b. Let’s consider that multiply is a function factory that makes functions that can multiply a certain value to the argument passed. In the above example, the function factory creates two new functions – one that multiplies 10 to its argument, and one that multiplies 20.
When the code is given in line no. 7 and line no. 8 get executed multiple functions are called and stored in their respective variables. As you might be expecting after the execution, arguments passed would no longer be accessible but this is not the case. Variables multiplyBy10 and multiplyBy20 hold a reference to the anonymous function which was returned when function multiply was called with arguments 10 and 20, respectively. The instance of the returned anonymous function maintains a reference to its lexical environment, within which the passed arguments exist.
multiplyBy10 and multiplyBy20 are both closures. They have the same function definition but store different lexical environments and variable values. In multiplyBy10 the lexical environment of a is 10, while in the lexical environment for multiplyBy20, a is 20.
Running the Code
Let us run the above code in the console of a browser with the console.dir( ) method and see what information we get:
Now, here we can see that the console.dir( ) method is7 used to get information about the variables that hold the reference of the function, we can see that both are Closures with different lexical environments, i.e., 10 and 20.
Let’s look into another example to get a better understanding of closures in JavaScript:
In the above example, the outer function Counter returns the reference of the inner function increase counter (). Here, the function increase counter increases the outer variable counter to 1. The Counter( ) function updates its closures every time it is called. So calling the inner function multiple times will increase the counter by one every time. So, we can say that closures are valid in multiple levels of inner functions as well.
When to Use Closures in JavaScript?
1. Emulating Private Methods
We generally use closures as access modifiers to give objects’ data a kind of private state. Data privacy is an essential property that helps us to program an interface, not an implementation. Any exposed method defined within the scope of the closure is privileged (accessed by only certain entities).
2. Maintaining States Between Function Calls
Let’s suppose there is a function that multiplies different values together. One way of doing that is with the help of a global variable because they are available throughout the code. But, there is a drawback! Yes, a variable that is globally accessible can also be modified from anywhere in the code. We can achieve this using closures. Closures help in maintaining as well as capturing the state between different function calls without using a global variable.
3. Functional Programming
Currying, which is an important function of functional programming, is also possible due to closures. It occurs when a function returns another function until we completely supply the arguments.
4. Create Iterators
Last but not least, creating an iterator. It is fairly easy to create iterators. This is because using closures we can well-preserve the data from the outer scope. Every call creates a new iterator (using closures) with a fixed starting index. Then, at every successive call of the iterator, the next value is returned. However, we should be cautious while using it with jQuery (or other JavaScript events).
Apart from the above-mentioned, you can think of several other ways to use closures according to your needs. You just need to have a good knowledge of scopes and lexical scopes to get the best out of closures.
Kickstart your Full Stack Development journey by enrolling in GUVI’s Full Stack Development Career Program with placement assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced certification course.
Conclusion
The closures are a very useful concept. Beginner JavaScript Programmers often find the concept of closures too overwhelming and skip it altogether. The scope rules, lexical scoping, and nested scopes are the accessibility of variables. The lexical scope allows a function scope to statically access the variables from the outside of the scopes. There can be a function or a block scope. Most of the time people are already using them without actually realizing it.
Closures matter in a code because they allow us to retain the values even after execution, which is a powerful and unique feature in JavaScript that only functions can have. Sometimes, we need to hold onto some values and likely keep them separate from others. So what do you use in that case? simple, A function. Why, though? To keep track of the data over time, with a closure.
FAQs on Closures of JavaScript
Q1. Closures can be used for performing which of the following operations?
Ans. The options are –
Maintaining states between function calls
Emulating private methods
Creating iterators
All of the above
Correct Answer: (d) All of the above
Q2. JavaScript uses which type of scoping?
Ans. The options are –
Segmental
Sequential
Lexical
Literal
Correct Answer: (c) Lexical scoping
Q3. Which of the following is mandatory to implement lexical scoping?
Ans.
Reference the current scope chain
Dereference the current scope chain
Return the value
None of the above
Correct Answer: (a) to implement the lexical scoping, the internal state of JavaScript must include a reference to the current scope chain apart from the code of the function.
Q4. JavaScript is a/an ______ language?
Ans.
High-level
Assembly
Object-oriented
Object-based
Correct Answer: (d) JavaScript is an object-based language. It is not a completely object-oriented language because it does not follow three basic properties of OOPs such as polymorphism, Encapsulation, and Inheritance.
Q5. What will be the output of the following JavaScript code?
Ans.
var temp=50;
if (temp>100)
{
document.write(100);
}
else
{
document.write(temp);
}
100
0
50
Undefined
Correct Answer: (c) 5050 is not greater than 100, therefore if the condition fails then else condition will execute. Hence, 50 will be our output.
So, that was all about Closures in JavaScript. Go ahead and practice umpteen questions and coding challenges around the topic in our specially curated Practice platform CODEKATA.
Wish to learn every bit of JavaScript? Have the most simplified glance of all the concepts in JavaScript in GUVI Classes. You can join us with a click.
Master Tech Courses with 100% Placement Support in ZEN Class From GUVI!
Find more such informational blogs around the corner and keep reading!
Did you enjoy this article?