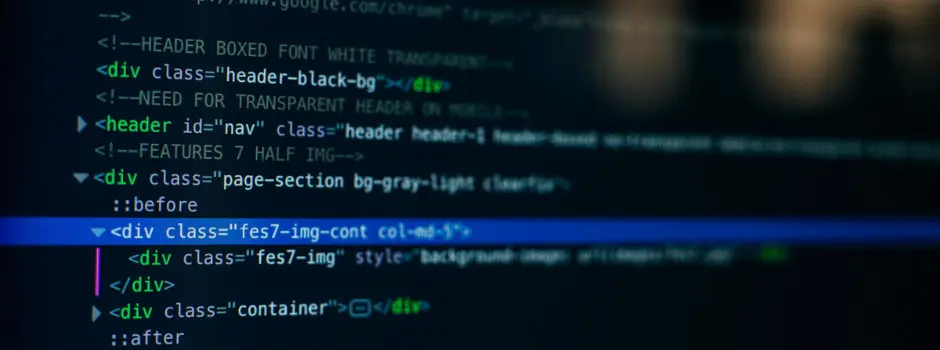
Mastering CSS Selectors: A Comprehensive Guide
Mar 05, 2025 10 Min Read 3806 Views
(Last Updated)
In web development, CSS selectors are indispensable tools that enable developers to select and manipulate HTML elements. They provide a powerful way to apply unique styles to elements based on their attributes, hierarchy, state, and more.
This article aims to offer an in-depth understanding of CSS selectors, their types, and use cases.
Table of contents
- Introduction to CSS Selectors
- Types of CSS Selectors
- 1) Simple Selectors
- 2) Universal Selectors
- 3) Combinator Selectors
- 4) Pseudo-Class Selectors
- 5) Pseudo-Elements Selectors
- 6) Attribute Selectors
- Concluding Thoughts...
- FAQs
- What are CSS type selectors used for?
- What is a CSS rule?
- What is a class in CSS?
- What are the main parts of CSS?
Introduction to CSS Selectors
CSS Selectors are the building blocks of Cascading Style Sheets (CSS). They are used to “select” the HTML elements that you want to style.
Without selectors, applying styles to specific elements on a webpage would be nearly impossible. For example:
p {
color: red;
}
In the code snippet above:
- Selector (
p
): This is the selector part of the CSS rule. In this example, it’s targeting all<p>
elements in the HTML document. - Declaration Block (
{ ... }
): This block contains one or more declarations. In this example, it contains a single declaration. - Property (
color
): This is the property being styled. It specifies which aspect of the element’s presentation is being changed. In this case, it’s the text color. - Value (
red
): This is the value assigned to the property. It specifies how the property should be styled. In this case, it sets the text color of the<p>
elements to red.
So, the CSS rule p { color: red; }
selects all <p>
elements and sets their text color to red.
Also Find Out Types of CSS: A Comprehensive Guide to Styling Web Pages
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with placement assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Instead, if you would like to explore HTML and CSS through a Self-paced course, try GUVI’s Modern HTML and CSS Self-Paced certification course.
Types of CSS Selectors
CSS selectors are broadly classified into five categories: Simple selectors, Universal selectors, Combinator selectors, Pseudo-class selectors, Pseudo-elements selectors, and Attribute selectors. Let us understand them through a simple table:
CSS Selectors | Description |
---|---|
1. Simple Selectors | Simple selectors are utilized to choose HTML elements based on various criteria, including their element name, ID, attributes, and more. |
2. Universal Selector | This selector targets all elements present on the page. |
3. Combinator Selectors | These selectors combine other selectors to indicate relationships between elements, such as descendants ( ) or child (>). |
4. Pseudo-Class Selectors | This selector chooses elements based on their state or position, like :hover for creating hover effects. |
5. Pseudo-Element Selectors | This selector is employed to select specific parts of an element, such as ::before or ::after. |
6. Attribute Selectors | It selects elements based on their attribute values. |
Also Read: 10 Best HTML and CSS Project Ideas for Beginners
Now that we know their types, we will move on to learning and understanding all of these selectors in-depth separately in the section that follows.
1) Simple Selectors
Simple selectors are the most basic type of CSS selectors. They include the following:
- Element/Type selectors: These selectors select elements based on the element type. For example, ‘h1’ selects all the h1 elements. You will understand better with an example, such as the one below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Element Type Selector Example</title>
<style>
/* Element Type Selector */
p {
color: blue;
}
</style>
</head>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph with blue text color.</p>
<p>This is another paragraph with blue text color.</p>
</body>
</html>
In this example, we’re using an element type selector to target all <p>
elements in the HTML document and set their text color to blue.
- Element Type Selector (
p
): Thep
in CSS targets all<p>
elements in the HTML document. - CSS Property (
color: blue;
): This sets the text color of the targeted<p>
elements to blue.
When you open this HTML document in a web browser, you’ll see that both paragraphs have blue text color applied to them.
Also Read: HTML vs CSS: Critical Differences Developers Can’t Ignore
- Class selectors: Class selectors select elements based on their class attribute. They are denoted by a ‘.’ followed by the class name.
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Class Selector Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1 class="highlight">Welcome to Class Selector Example</h1>
<p>This is a paragraph without any special styling.</p>
<p class="highlight">This is a paragraph with the 'highlight' class applied.</p>
</body>
</html>
CSS:
.highlight {
background-color: yellow;
color: blue;
padding: 10px;
}
In the above code:
- HTML Structure:
- We have a simple HTML document with three elements: two
<p>
elements and one<h1>
element. - One of the
<p>
elements have a class attribute set to “highlight”.
- We have a simple HTML document with three elements: two
- CSS Styling:
- In the linked stylesheet (styles.css), we define a class selector
.highlight
. - This selector applies styles to any element with the class “highlight”.
- The styles specified within the selector include yellow background color, blue text color, and additional padding.
- In the linked stylesheet (styles.css), we define a class selector
- Class Selector:
- The class selector
.highlight
selects all elements with the class “highlight” and applies the defined styles to them. - In this example, the
<h1>
and one of the<p>
elements have the “highlight” class applied to them. - As a result, the
<h1>
element and the<p>
element with the “highlight” class will have a yellow background, blue text, and extra padding.
- The class selector
The class selector allows us to apply the same styles to multiple elements across the HTML document by simply adding the class attribute to those elements. This promotes code reusability and helps maintain consistency in styling.
Must Read: A Comprehensive Guide to HTML and CSS Roadmap
- ID selectors: ID selectors select an element based on its id attribute. They are denoted by a ‘#’ followed by the id name.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ID Selector Example</title>
<style>
/* ID Selector */
#main-heading {
color: blue;
font-size: 24px;
}
</style>
</head>
<body>
<h1 id="main-heading">Welcome to My Website</h1>
<p>This is some content on the page.</p>
</body>
</html>
In this example:
- We have an HTML
<h1>
element with theid
attribute set to"main-heading"
. - In the CSS section, we use the ID selector
#main-heading
to target this specific element. - We then apply styles to the
#main-heading
selector, setting its text color to blue and font size to 24 pixels.
So basically:
- The
#main-heading
selector targets the HTML element with the ID"main-heading"
. - It applies the specified styles (in this case, color and font size) to that specific element only.
- IDs are unique within an HTML document, meaning that only one element should have a specific ID value. This uniqueness makes ID selectors suitable for targeting specific elements when you need to apply styles or perform other operations on them.
In summary, an ID selector in CSS is used to target and style a specific HTML element based on its unique identifier, which is defined using the id
attribute in HTML. It provides a powerful way to apply styles to individual elements on a webpage.
Also Read: Style Matters: Exploring Essential CSS Properties You Must Know
2) Universal Selectors
A universal selector in CSS is denoted by an asterisk *
. It matches any element in the HTML document, allowing you to apply styles to all elements simultaneously. It’s commonly used to reset default styles or apply global styles across an entire document.
Here’s a simple example demonstrating the universal selector:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Universal Selector Example</title>
<style>
/* Apply a border to all elements */
* {
border: 1px solid black;
}
/* Apply specific styles to headings */
h1, h2, h3 {
color: blue;
}
</style>
</head>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
<div>This is a div.</div>
</body>
</html>
In this example:
- The universal selector
*
is used to apply a border of 1-pixel solid black to all elements in the document. - Additionally, specific styles are applied to
<h1>
,<h2>
, and<h3>
elements to set their text color to blue.
When you open this HTML document in a browser, you’ll see that all elements on the page have a 1-pixel solid black border around them. This is because the universal selector *
matches all elements in the document, applying the specified border style to each one.
Also Read: CSS Transitions: Important Things To Know About
3) Combinator Selectors
Combinator selectors are used to select elements based on a specific relationship between them. They include:
- Descendant selector (space): This selector selects all elements that are descendants of a specified element. Let us understand through an example:
HTML:
<div class="container">
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<p>Paragraph inside container</p>
</div>
Now, let’s say you want to style the <li>
elements that are descendants of the <ul>
element inside the .container
div. You would use a descendant selector to achieve this. Here’s how you would do it:
CSS:
.container ul li {
/* Styles for <li> elements inside <ul> within .container */
color: blue;
font-weight: bold;
}
What happened:
.container ul li
: This CSS selector targets<li>
elements that are descendants (children, grandchildren, etc.) of a<ul>
element, which in turn is a descendant of an element with the class.container
.- In this case, it selects both
<li>
elements inside the<ul>
element within the.container
div. - The styles specified within the rule will be applied to these selected
<li>
elements.
So, in summary, a descendant selector allows you to apply styles to elements that are nested within other elements in the HTML document, based on their hierarchical relationship. It’s denoted by a space between the selectors, indicating the parent-child relationship.
Must Explore: Top 11 CSS Frameworks for Front-End Developers: A Comprehensive Guide
- Child selector (>): This selector selects all elements that are direct children of a specified element. See the example below:
<div class="parent">
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<p>Paragraph inside parent</p>
<div>
<span>Span inside nested div</span>
</div>
</div>
And the corresponding CSS using the child selector:
.parent > ul {
background-color: lightblue;
}
In the code above:
.parent > ul
: This selector targets<ul>
elements that are direct children of an element with the classparent
. It applies the specified styles (in this case, setting the background color to light blue) only to the<ul>
element that is an immediate child of the.parent
element.
In this example, the <ul>
element that contains “Item 1” and “Item 2” is directly nested inside the .parent
div. Therefore, it matches the criteria specified by the child selector (>
), and the background color of this <ul>
element will be changed to light blue according to the CSS rule.
However, the <ul>
element is the only immediate child of .parent
that matches the criteria of being a direct child. Other elements like the <p>
and the nested <div>
with the <span>
inside are not affected by this CSS rule because they are not direct children of .parent
.
So, the child selector (>
) allows for very specific targeting of elements that are direct children of a particular parent element in the HTML hierarchy.
Also Find Out Best Techniques for Creating Seamless Animations with CSS and JavaScript
- Adjacent sibling selector (+): This selector selects all elements that are the next siblings of a specified element. Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Adjacent Sibling Selector Example</title>
<style>
/* Adjacent Sibling Selector */
h2 + p {
color: blue;
}
</style>
</head>
<body>
<h2>Title</h2>
<p>This paragraph will be styled because it's an adjacent sibling of the h2 element.</p>
<p>This paragraph will NOT be styled because it's not an adjacent sibling of the h2 element.</p>
<h2>Another Title</h2>
<p>This paragraph will be styled because it's an adjacent sibling of the second h2 element.</p>
</body>
</html>
In this example, we have an adjacent sibling selector targeting <p>
elements that are immediately preceded by an <h2>
element.
- The selector
h2 + p
selects any<p>
element that comes immediately after an<h2>
element. - We apply the style
color: blue;
to the selected<p>
elements, which changes their text color to blue. - Only the
<p>
elements directly following an<h2>
element will be affected by this CSS rule. - Other
<p>
elements that are not adjacent siblings of an<h2>
will not be styled.
- General sibling selector (~): This selector selects all elements that are siblings of a specified element. Example:
HTML:
<div>
<p>This is the first paragraph.</p>
<p>This is the second paragraph.</p>
<p>This is the third paragraph.</p>
<p>This is the fourth paragraph.</p>
</div>
Now, let’s say we want to apply some styles to all <p>
elements that come after the first <p>
element. We can achieve this using the general sibling selector (~
). Here’s how it looks in CSS:
CSS:
p:first-child ~ p {
color: blue;
}
In the code above:
p:first-child
: This selector targets the first<p>
element among its siblings.~
: This is the general sibling combinator. It selects all sibling elements that come after the specified element.p
: This is the type selector for<p>
elements.
So, the CSS rule p:first-child ~ p
selects all <p>
elements that are siblings of the first <p>
element and applies the specified styles to them. In this case, we’re setting the text color of those paragraphs to blue.
Applied to our example HTML structure, this CSS rule will select the second, third, and fourth <p>
elements, but not the first one, as it comes before the first <p>
element.
Also Read: A Complete Guide to HTML and CSS for Beginners
4) Pseudo-Class Selectors
Pseudo-class selectors are used to select elements that are in a specific state. They include:
- :hover: Selects an element when you mouse over it.
- :active: Selects an element during the activation of the element.
- :focus: Selects an element when it has focus.
- :visited: Selects all links that have been visited by the user.
Let us understand all four with one example to keep it simple and precise:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Pseudo-class Selectors Example</title>
<style>
/* Common styles */
button {
padding: 10px 20px;
border: none;
cursor: pointer;
margin-right: 10px;
}
/* :hover */
button:hover {
background-color: lightblue;
}
/* :active */
button:active {
transform: translateY(2px);
}
/* :focus */
input:focus {
outline: 2px solid green;
}
/* :visited */
a:visited {
color: purple;
}
</style>
</head>
<body>
<!-- :hover -->
<button>Hover me</button>
<!-- :active -->
<button>Click me</button>
<!-- :focus -->
<input type="text" placeholder="Type something and click outside to focus">
<!-- :visited -->
<a href="https://www.example.com">Visited Link</a>
</body>
</html>
In the code above:
:hover
: In the example, when you hover over the button, its background color changes to light blue.:active
: In the code above, when you click on the button, it moves slightly downwards due to thetransform
property.:focus
: Above, when you click inside the input field, a green outline appears around it.:visited
: In the example, the color of the link changes to purple after it has been visited.
These pseudo-classes provide a way to enhance the user experience by styling elements dynamically based on user interaction or state.
Must Read | Complete CSS Tutorial: Essential Guide to Understand CSS
5) Pseudo-Elements Selectors
Pseudo-elements selectors are used to apply styles to a specific part of an element. They include:
- ::before: Inserts content before an element.
- ::after: Inserts content after an element.
- ::first-line: Selects the first line of an element.
- ::first-letter: Selects the first letter of an element.
Let us understand all four with one example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Pseudo-elements Example</title>
<style>
.box::before {
content: "Before ";
font-weight: bold;
}
.box::after {
content: " After";
font-style: italic;
}
.box::first-line {
color: blue;
}
.box::first-letter {
font-size: 150%;
color: red;
}
</style>
</head>
<body>
<div class="box">
This is a simple example text.
</div>
</body>
</html>
In the code above:
::before
Pseudo-element: In this example, “Before ” is inserted before the content of the.box
element with bold font-weight.::after
Pseudo-element: In the code above, ” After” is inserted after the content of the.box
element with italic font-style.::first-line
Pseudo-element: Here, the color of the first line of text within the.box
element is set to blue.::first-letter
Pseudo-element: In the above example, the font size of the first letter of text within the.box
element is increased to 150% and its color is set to red.
These examples illustrate the usage of each pseudo-element to style specific parts of an element’s content, enhancing the presentation of text on a webpage.
Also Read: What does a Front-End Developer do? A Complete Guide
6) Attribute Selectors
Attribute selectors are used to select elements based on their attribute or attribute values. They include:
- [attribute]: Selects elements with a specified attribute.
- [attribute=value]: Selects elements with a specified attribute and value.
- [attribute~=value]: Selects elements with an attribute containing a specified word.
- [attribute^=”value”]: Selects elements that have the specified attribute with a value beginning exactly with a given string.
Let’s illustrate each with a simple HTML example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Attribute Selector Example</title>
<style>
/* [attribute] selector */
[target] {
color: blue;
}
/* [attribute="value"] selector */
[href="https://example.com"] {
font-weight: bold;
}
/* [attribute~="value"] selector */
[class~="highlight"] {
background-color: yellow;
}
/* [attribute^="value"] selector */
[src^="https://"] {
border: 1px solid black;
}
</style>
</head>
<body>
<!-- [attribute] selector -->
<a href="https://example.com" target="_blank">Link 1</a>
<a target="_self">Link 2</a>
<br><br>
<!-- [attribute="value"] selector -->
<a href="https://example.com">Link 3</a>
<a href="https://another-site.com">Link 4</a>
<br><br>
<!-- [attribute~="value"] selector -->
<div class="highlight">Div 1</div>
<div class="highlight other">Div 2</div>
<br><br>
<!-- [attribute^="value"] selector -->
<img src="https://example.com/image.jpg" alt="Image 1">
<img src="http://another-site.com/image.jpg" alt="Image 2">
</body>
</html>
What happened:
- [attribute] selector: In this example, it selects
<a>
elements with thetarget
attribute. - [attribute=”value”] selector: In the code above, it selects
<a>
elements with thehref
attribute set to"https://example.com"
. - [attribute~=”value”] selector: In this example, it selects
<div>
elements with the classhighlight
. - [attribute^=”value”] selector: With the example above, it selects
<img>
elements with thesrc
attribute starting with"https://"
.
These selectors provide powerful ways to target specific elements in HTML based on their attributes and attribute values, allowing for fine-grained control over styling and behavior.
Also Explore: Top 20 HTML & CSS Interview Questions With Answers
Concluding Thoughts…
CSS selectors are powerful tools that can greatly increase your efficiency when styling your web pages.
Understanding how to use them effectively can save you time and frustration, and can lead to cleaner, more manageable stylesheets.
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with placement assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Instead, if you would like to explore HTML and CSS through a Self-paced course, try GUVI’s Modern HTML and CSS Self-Paced certification course.
Must Know About Top Full Stack Development Trends: What to Expect
FAQs
CSS type selectors are used to target HTML elements based on their tag name, applying styles to all elements of that type.
A CSS rule consists of a selector and a declaration block. The selector specifies which elements the styles should apply to, while the declaration block contains the styles to be applied.
In CSS, a class is a reusable identifier that allows you to apply styles to multiple elements in an HTML document.
The main parts of CSS include selectors, properties, and values. Selectors target specific elements, properties define the styles to be applied, and values specify the settings for those styles.
Did you enjoy this article?