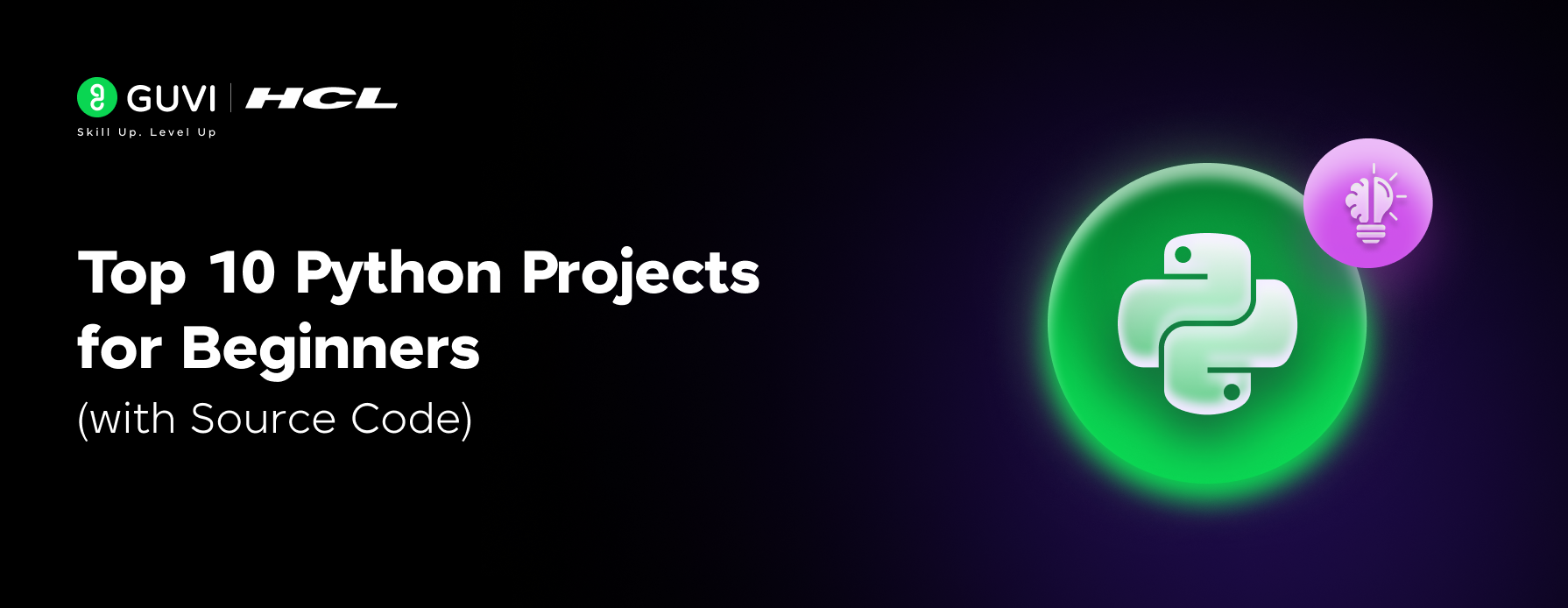
Top 10 Python Projects for Beginners with Source Code
Jul 07, 2025 4 Min Read 19330 Views
(Last Updated)
Python’s popularity as a programming language is unmatched, securing its place as the most sought-after skill amongst developers, as highlighted by a 2020 Stack Overflow survey.
Its simplicity, coupled with an extensive library and compatibility across platforms, makes Python the go-to for beginners and seasoned programmers alike, embarking on Python projects like calculators, games, and web applications.
This article sets the stage for newcomers to dive into programming, offering 15 Python project ideas ranging from creating a simple calculator and tic-tac-toe game to more advanced ventures in web scrapping and AI with Python and Pandas.
These Python projects not only solidify the fundamentals but also inspire creativity and problem-solving, essential skills in the developers’ toolkit.
Table of contents
- Why Python Projects Matter for Beginners
- Top 10 Python Projects for Beginners
- Todo List App
- Rock, Paper, and Scissors Game
- Movie Recommendation System
- Web Scraper
- Iris Flower Classifier
- Stock Price Prediction
- Auto Email Sender
- Instagram Bot
- Smart Door Lock System
- Temperature & Humidity Logger
- Concluding Thoughts...
- FAQs
- How to create a simple Python project?
- What kind of projects can be created using Python?
- How can I practice Python as a beginner?
- What is == in Python?
Why Python Projects Matter for Beginners
Engaging in Python projects is crucial for beginners, providing a hands-on approach that complements theoretical learning. Here’s why starting with Python projects is beneficial:
- Practical Application of Knowledge: Beginners can apply what they’ve learned about Python syntax and structures in real-world scenarios, enhancing understanding and retention.
- Skill Development: Python projects like building calculators or games allow novices to practice defining functions, using conditional statements, and managing data flow.
- Community Engagement: The vast Python community offers support and resources, making learning interactive and less daunting for new programmers.
- Portfolio Building: Completing Python projects gives beginners tangible outcomes to showcase their skills, crucial for career advancement.
- Versatility of Python: Python’s use in diverse fields, from web development to data science, means learners can explore various interests and career paths.
- Cross-Platform Compatibility: Python’s ability to run on different operating systems without modification broadens learning and application opportunities.
- Automation and Efficiency: Python can automate mundane tasks, allowing beginners to focus on more complex and creative aspects of programming.
Are you interested in starting a career in Python? Kickstart your Python journey using Guvi’s FREE E-book on Python: A Beginner’s Guide to Coding and Beyond. It covers all the necessary concepts you need to know starting from the installation of Python on your machine.
Top 10 Python Projects for Beginners
Now, let’s dive into the top 10 Python projects for beginners. This section lists Python projects from various domains such as web development, data analysis, data science, machine learning and AI, and Internet of Things (IoT). By only focusing on these 10 projects, you will gain experience in different domains. Let’s get started!
1. Todo List App
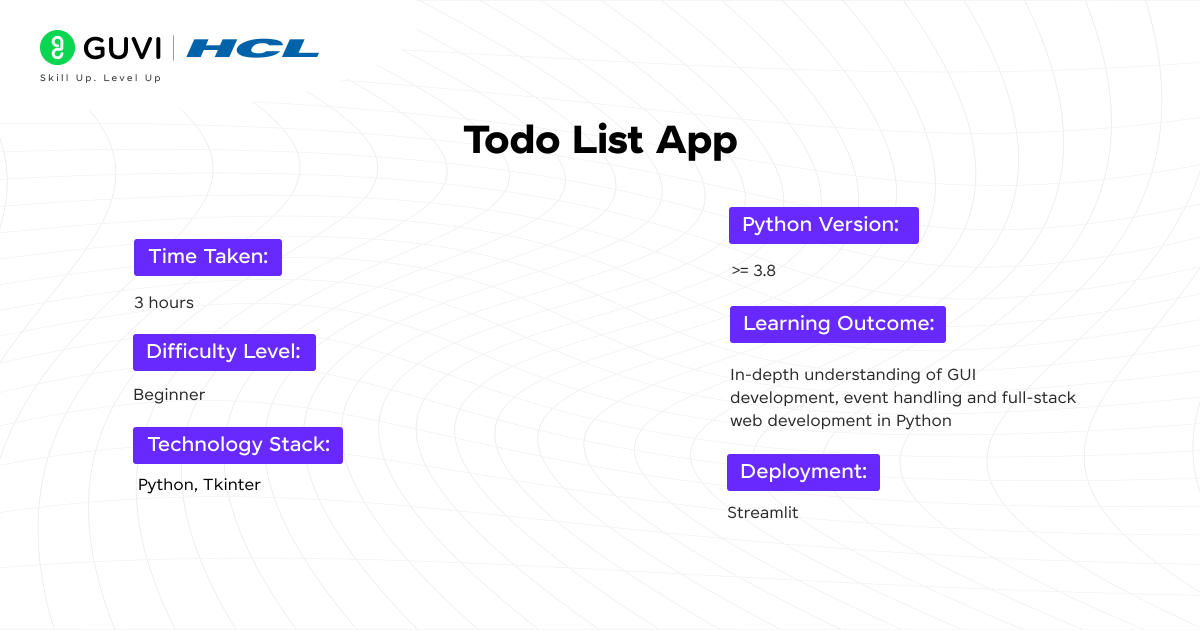
This is a very basic application built using Python and Tkinter. It offers a GUI where users can add, delete, and mark tasks as completed. It is a great beginner-friendly project for learning how GUI applications work in Python.
Time Taken: 3 hours
Difficulty Level: Beginner
Tech Stack: Python, Tkinter
Python Version: >= 3.8
Learning Outcome: In-depth understanding of GUI development, event handling, and full-stack web development in Python
Deployment: Streamlit
Source Code: Todo List
2. Rock, Paper, and Scissors Game
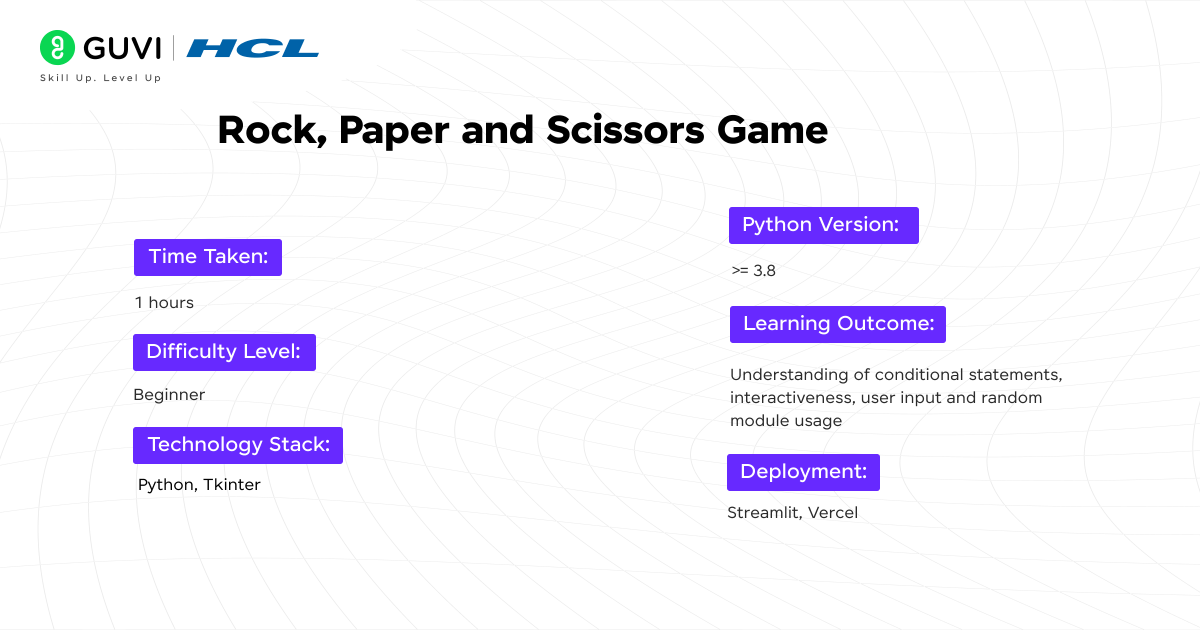
This project is a simple, fun, and interactive game developed using the Python Tkinter package. This game lets the user play against the computer by choosing one of the three options. It uses basic conditional logic to determine the winner after every round.
Time Taken: 1 hour
Difficulty Level: Beginner
Tech Stack: Python, Tkinter
Python Version: >= 3.8
Learning Outcome: Understanding of conditional statements, interactiveness, user input and random module usage
Deployment: Streamlit, Vercel
Source Code: Rock, Paper, and Scissors
3. Movie Recommendation System
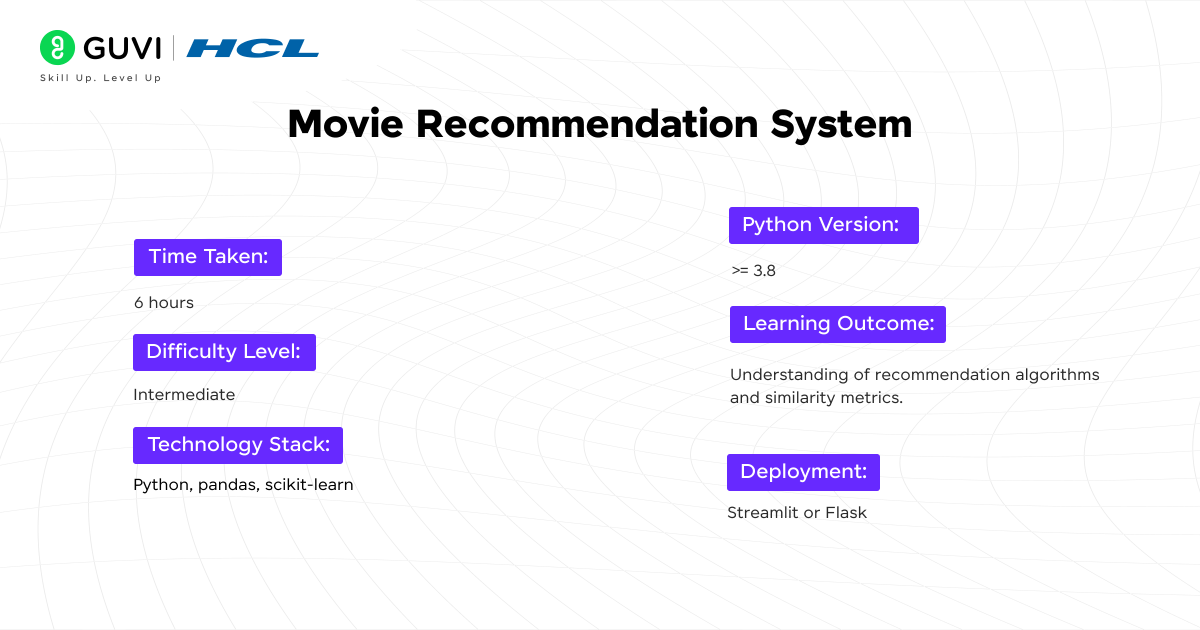
This is a recommendation system built using Python that leverages the power of the pandas library. It suggests movies based on user preferences by analyzing similarities between films. It is a great project for understanding data-driven solutions.
Time Taken: 6 hours
Difficulty Level: Intermediate
Tech Stack: Python, pandas, scikit-learn
Python Version: >= 3.8
Learning Outcome: Understanding of recommendation algorithms and similarity metrics
Deployment: Streamlit or Flask
Source Code: Movie Recommendation System
4. Web Scraper
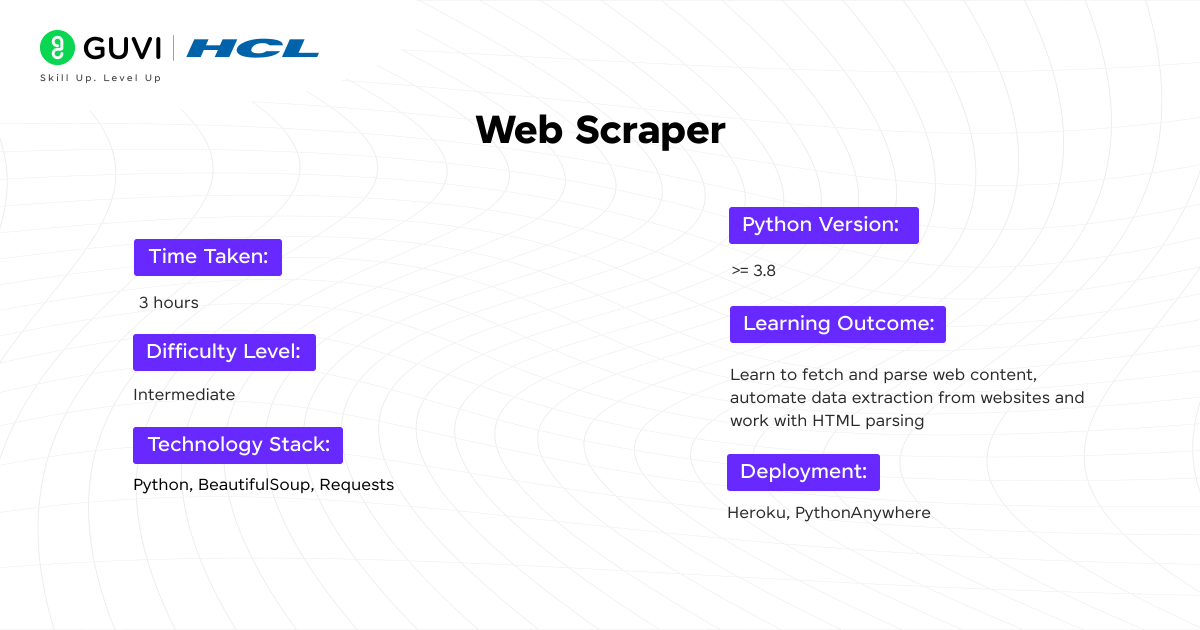
It is a real-time web scraper built using Python and the BeautifulSoup
library. It extracts information from websites and presents the data in a structured format. It parses the HTML and handles requests. The collected data is then stored in a structured database for further use.
Time Taken: 3 hours
Difficulty Level: Intermediate
Tech Stack: Python, BeautifulSoup, Requests
Python Version: >= 3.8
Learning Outcome: Learn to fetch and parse web content, automate data extraction from websites and work with HTML parsing
Deployment: Heroku, PythonAnywhere
Source Code: Web Scraper
5. Iris Flower Classifier
This is a machine learning classification that predicts the species of Iris flowers based on petals and sepal dimensions. It uses the popular Iris dataset and applies supervised learning algorithms to train and predict.
Time Taken: 4 hours
Difficulty Level: Intermediate
Tech Stack: Python, scikit-learn, Pandas
Python Version: >= 3.8
Learning Outcome: Understanding of supervised learning, classification algorithms, and model evaluation techniques
Deployment: Streamlit, Heroku
Source Code: Iris Flow Classifier
6. Stock Price Prediction
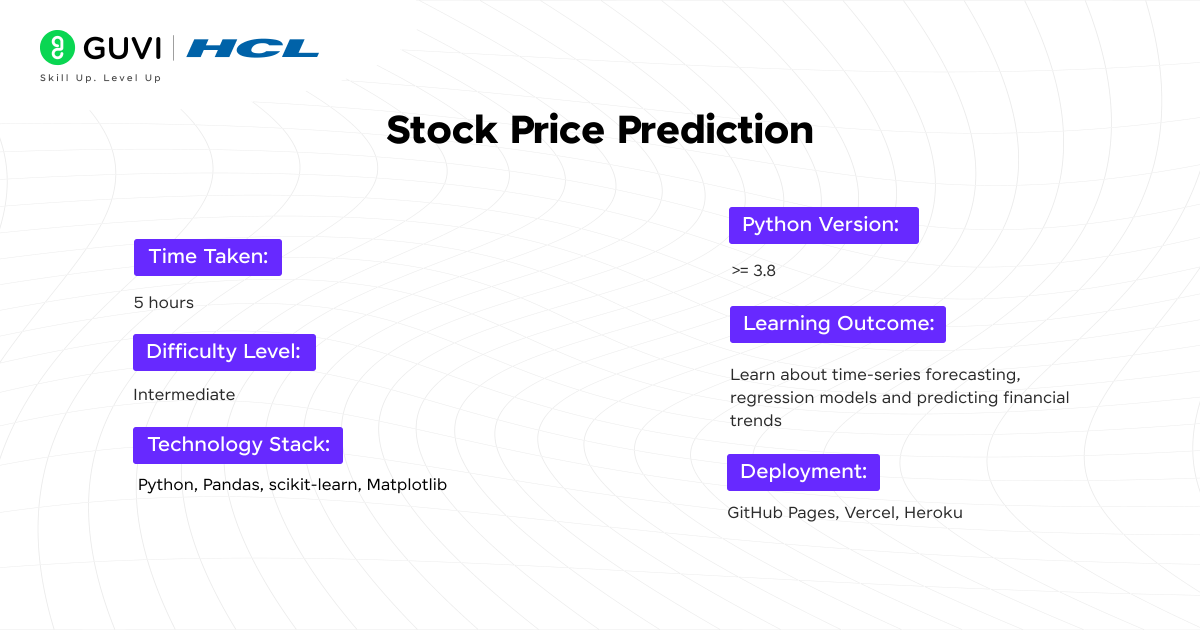
This focuses on predicting stock prices using machine learning techniques. This project utilizes historical data and applies models such as Linear Regression to forecast future trends. It is a great way to understand time series prediction.
Time Taken: 5 hours
Difficulty Level: Intermediate
Tech Stack: Python, Pandas, scikit-learn, Matplotlib
Python Version: >= 3.8
Learning Outcome: Learn about time-series forecasting, regression models, and predicting financial trends
Deployment: GitHub Pages, Vercel, Heroku
Source Code: Stock Price Prediction
If you want to learn the necessary skills required for data science starting from scratch to advance in a single course from India’s top Industry Instructors, consider enrolling in GUVI’s Zen class course “Become a Data Science Course Professional with IIT-M Pravartak” that not only teaches you everything about data science, but also provides you with hands-on project experience and industry-grade certificate!
7. Auto Email Sender
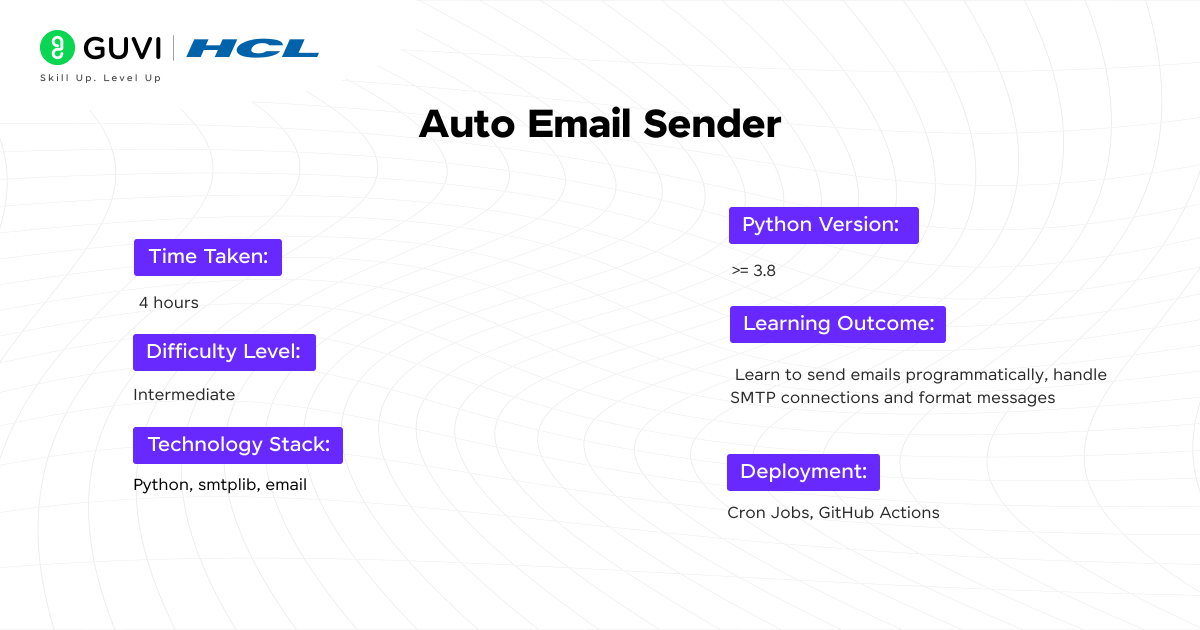
Auto Email sender built using Python’s smtplib
and email libraries. It allows users to send emails directly from a script by configuring the sender’s credentials. This can be customized for bulk or scheduled sending.
Time Taken: 4 hours
Difficulty Level: Intermediate
Tech Stack: Python, smtplib, email
Python Version: >= 3.8
Learning Outcome: Learn to send emails programmatically, handle SMTP connections and format messages
Deployment: Cron Jobs, GitHub Actions
Source Code: Auto Email Sender
8. Instagram Bot
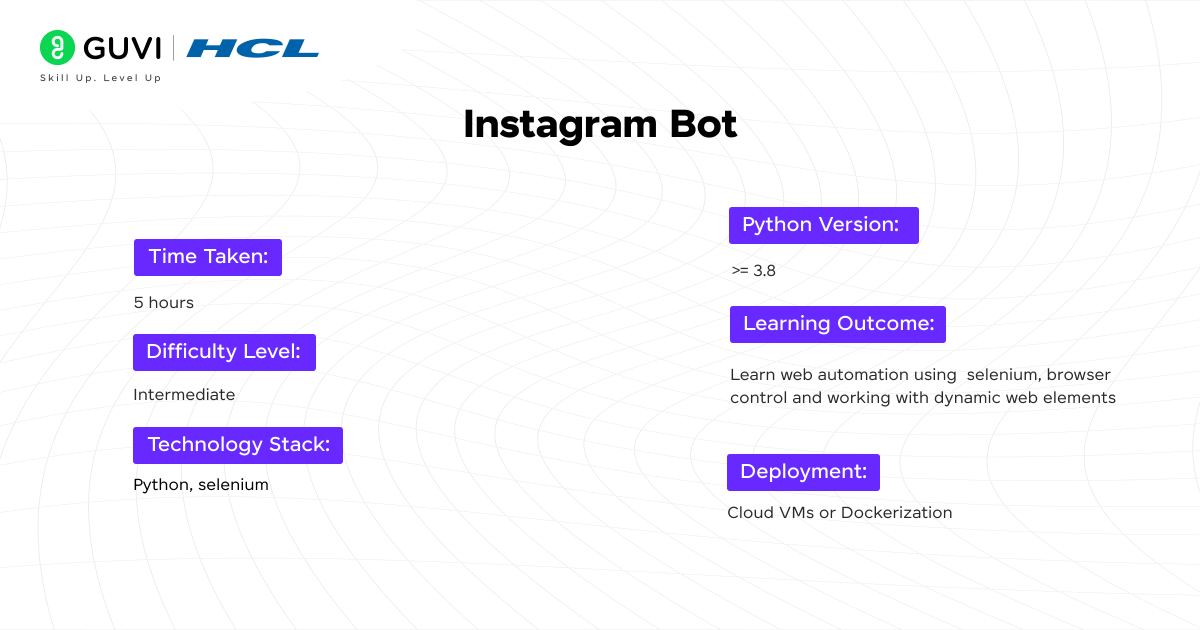
This project automates interactions such as following users, liking posts, and commenting. Using the Selenium library, it simulates real user actions within a browser. The bot can be configured to follow specific hashtags or users, making it a useful tool for growing engagement with your followers.
Time Taken: 5 hours
Difficulty Level: Intermediate
Tech Stack: Python, selenium
Python Version: >= 3.8
Learning Outcome: Learn web automation using Selenium, browser control, and working with dynamic web elements
Deployment: Cloud VMs or Dockerization
Source Code: Instagram Bot
9. Smart Door Lock System
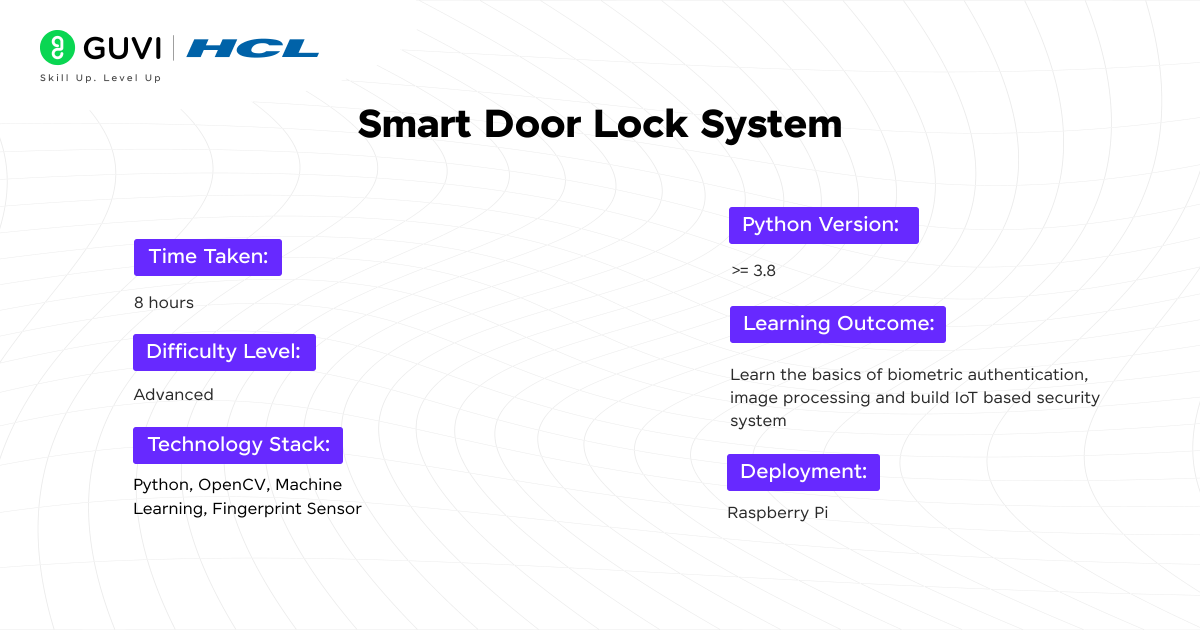
This is an advanced-level smart door lock system that combines fingerprint recognition and camera verification for secure access. The system captures fingerprint data and takes a snapshot of the user’s face to confirm identity.
Time Taken: 8 hours
Difficulty Level: Advanced
Tech Stack: Python, OpenCV, Machine Learning, Fingerprint Sensor
Python Version: >= 3.8
Learning Outcome: Learn the basics of biometric authentication, image processing, and build IoT IoT-based security system
Deployment: Raspberry Pi
Source Code: Smart Door Lock System
10. Temperature & Humidity Logger

It is an environmental sensor like DHT11 or DHT22. It reads real-time data from the sensor and logs it into a file for future analysis. This system can be used for monitoring indoor climates or in applications where environmental tracking is crucial.
Time Taken: 8 hours
Difficulty Level: Advanced
Tech Stack: Python, DHT11/DHT22, Matplotlib
Python Version: >= 3.8
Learning Outcome: Learn to interface sensors with Python, log data and visualize environmental data
Deployment: Raspberry Pi or Arduino with sensors for continuous monitoring
Source Code: Temperature and Humidity Logger
Would you like to master Python as well as Full Stack Development and build an impressive portfolio? Then GUVI’s Full Stack Development Course is the perfect choice for you. Taught by industry experts, this boot camp equips you with everything you need to know, along with extensive placement assistance!
Concluding Thoughts…
Each Python project discussed in this article, from creating basic calculators and games to web scraping and data visualization, serves not just as an exercise in coding but as a stepping stone towards mastering Python.
By engaging with these Python projects, learners cultivate a practical understanding of Python’s syntax and its application across various domains, reinforcing the foundational skills requisite in the vast landscape of programming.
The significance of these Python projects transcends the immediate satisfaction of building something functional; it lies in the incremental development of problem-solving abilities, logical thinking, and creative innovations in programming solutions.
Must Read: 18 Famous Websites Built with Python in 2024
FAQs
To create a simple Python project, start by defining your project goals, setting up a development environment, writing your code using Python syntax, and testing your project for functionality. Learn more with the article above.
Python is versatile and can be used for various projects including web development, data analysis, artificial intelligence, automation, and more.
Beginners can practice Python by solving coding challenges on platforms like LeetCode or HackerRank, building small projects, following online tutorials, and reading Python documentation.
In Python, the double equals sign (==) is used as the equality operator to compare if two values are equal.
Did you enjoy this article?