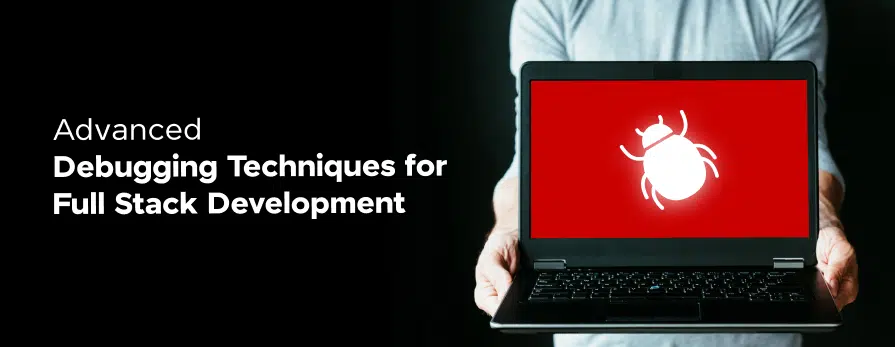
Advanced Debugging Techniques For Full Stack Development
May 08, 2025 6 Min Read 5196 Views
(Last Updated)
Debugging, the vital process of identifying and resolving bugs, errors, or defects in a software system, is an indispensable skill every software developer must master.
It ensures the software’s performance, functionality, and user satisfaction by delving deep into the code to correct any discrepancies that prevent the system from functioning correctly.
Highly technical in nature, effective debugging demands a systematic and disciplined approach, making it crucial for maintaining the quality, reliability, and security of the software.
As you embark on a journey through advanced debugging techniques aimed at unlocking the full potential of stack development, this article will introduce you to a variety of specialized tools and best practices.
This comprehensive guide will discuss everything from the basics to more sophisticated strategies and tools tailor-made for full-stack development, ensuring you have the knowledge and skills required to debug effectively and enhance your software development process.
Table of contents
- Getting into the Debugging Mindset
- Basic Debugging Strategies
- Advanced Debugging Techniques
- Specialized Debugging Tools for Full Stack Development
- Front-end Debugging Tools:
- Back-end Debugging Tools:
- Integrated Development Environments (IDEs):
- Additional Tools:
- Best Practices and Continuous Learning
- 1) Utilize Debugging Tools Effectively
- 2) Adopt a Methodical Approach to Debugging
- 3) Emphasize Continuous Learning and Collaboration
- Concluding Thoughts...
- FAQs
- What are the different debugging techniques?
- What is JTAG debugging?
- What is the difference between JTAG and UART?
- Which is faster UART or USART?
Getting into the Debugging Mindset
Understanding the problem you’re tackling is the cornerstone of effective debugging. Before diving into code changes, it’s essential to:
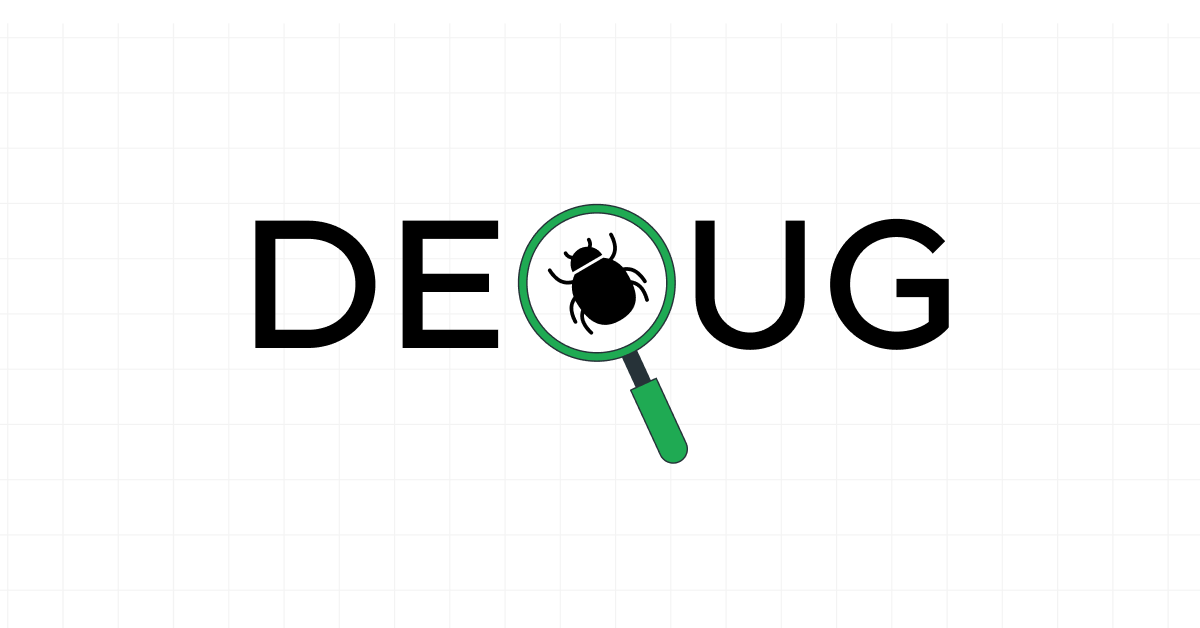
- Reproduce the Issue: Attempt to recreate the bug or error. This step is crucial as it helps to confirm the presence of the problem and provides a clear scenario where the issue manifests.
- Gather Information: Collect as much data as possible about the bug’s behavior. This includes error messages, the conditions under which the bug occurs, and any patterns that emerge.
Debugging is more than just a hunt for errors; it’s a comprehensive process that involves:
- Reproducing the Issue: Ensuring the problem can be consistently observed.
- Understanding the Code: Familiarize yourself with the codebase to better navigate and pinpoint potential fault lines.
- Identifying the Root Cause: Determining the underlying issue that’s causing the bug.
- Fixing the Bug: Implementing a solution to resolve the problem.
- Testing the Fix: Validating that the solution effectively eliminates the bug without introducing new issues.
Also Read: What is Full Stack Development (FSD)? A Complete Guide
The distinction between debugging and testing is significant, each with its unique focus, tools, and objectives. While testing is proactive, seeking to prevent bugs before they occur, debugging is reactive, addressing bugs that have already emerged.
This underscores the importance of a strategic approach to both activities to ensure software quality.
Effective debugging is characterized by a detailed and methodical process:
- Understanding Error Symptoms: Recognizing the signs that indicate a problem.
- Identifying the Cause: Tracing the symptoms back to their source.
- Fixing the Error: Correcting the issue to restore functionality.
Prevention plays a key role in minimizing debugging efforts. By planning and understanding the intended purpose of your code before writing, many potential bugs can be avoided, saving significant time and effort.
When bugs do arise, adopting a mindset of curiosity and openness to exploration can lead to more innovative and effective solutions.
Asking questions, admitting gaps in understanding, and approaching code as a puzzle rather than an adversary are all strategies that foster a productive debugging mindset.
Remember, debugging is not just about fixing problems; it’s an opportunity for learning and growth, allowing developers to deepen their understanding of their code and improve their problem-solving skills.
Also Explore: Full Stack Developer: Discover the Fastest Route to becoming one
Basic Debugging Strategies
In the realm of software development, mastering basic debugging strategies is crucial for efficiently identifying and resolving issues in your code.
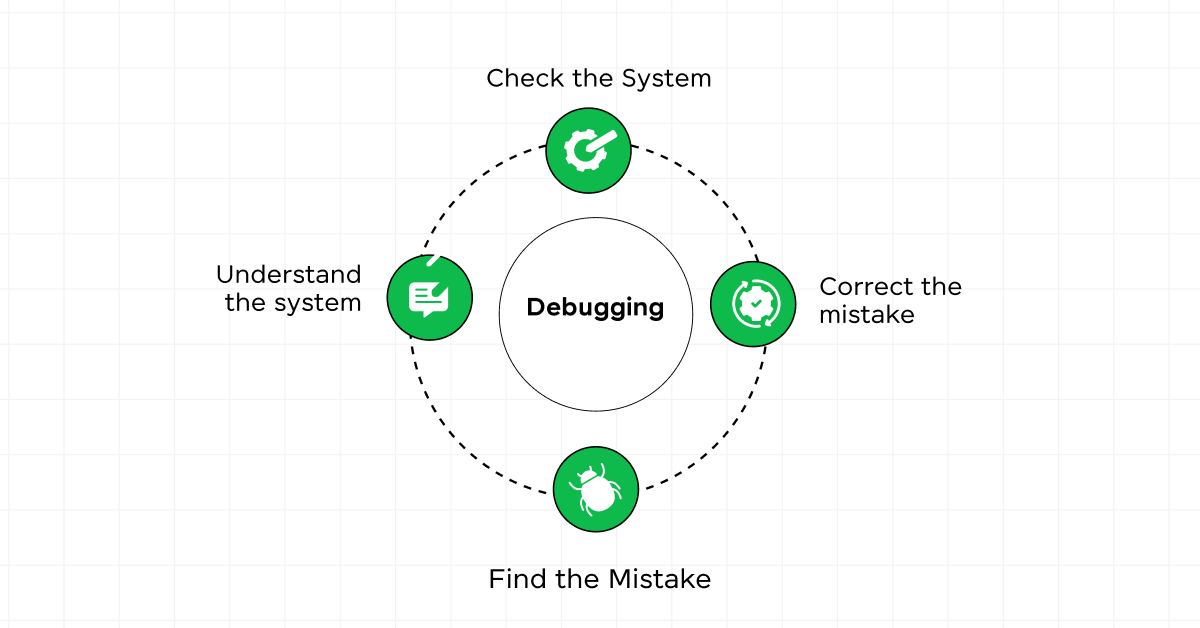
Let’s delve into some foundational techniques that every developer should have in their arsenal:
- Brute Force Method:
- Description: Utilize print statements to display intermediate values, aiding in pinpointing the erroneous statement.
- Tools: Symbolic programs or source code debuggers can systematize this approach.
- Backtracking:
- Description: Derive the source code backward from the error symptom to locate the error. Be mindful, as the number of backward steps can become significantly large.
- Cause Elimination Method:
- Description: Compile a list of potential causes for the error symptom and systematically conduct tests to eliminate each cause.
- Program Slicing:
- Description: Similar to backtracking, this method narrows the search area by utilizing program slices—a set of supply lines that influence the value of a specific variable at a particular statement.
- Backtracing (Backward Debugging):
- Description: Start from the point where the issue first manifested and work backward through the code to understand the root cause.
- Using Debugging Tools:
- Popular Tools: Chrome DevTools, Testsigma, Airbrake, dbForger.
- Advantage: These tools offer a variety of features tailored to different programming languages and environments.
- Breakpoints and Stepping:
- Technique: Set breakpoints at potential problem areas in your code. Utilize steps to move through the code line by line, examining variables and data structures.
- Binary Search:
- Approach: Divide your code into halves and systematically narrow down the bug’s location.
- Rubber Ducking:
- Concept: Explain the problem to an inanimate object (like a rubber duck) to gain clarity and potentially identify the issue yourself.
- Log Analysis:
- Strategy: Implement log analysis statements in strategic code areas to gather valuable debugging information.
- Clustering Bugs:
- Method: Group related bugs to identify common causes or patterns.
- Taking Breaks:
- Importance: Debugging can be mentally taxing; taking breaks helps clear your mind and approach the problem with fresh eyes.
- Taking Notes for Learning:
- Benefit: Documenting the debugging process and solutions discovered serves as a valuable resource for future challenges.
These strategies, ranging from the brute force method to program slicing and the use of sophisticated debugging tools, form the cornerstone of effective debugging.
Find Out Top Full-Stack Developer Skills
By familiarizing yourself with these techniques and incorporating them into your debugging process, you can enhance your ability to diagnose and resolve issues, ultimately improving the quality and reliability of your software.
Advanced Debugging Techniques
Diving deeper into advanced debugging techniques, especially for complex applications involving multiple processes and threads, requires a methodical approach. Ladebug, a powerful tool in this realm, offers features tailored for such scenarios:
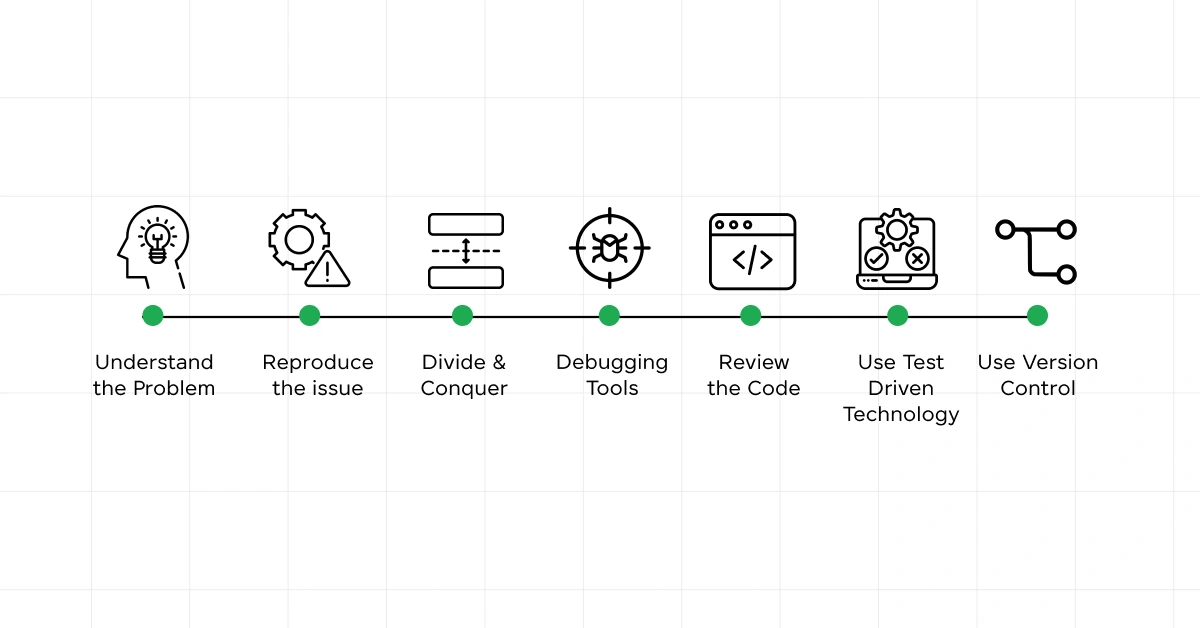
- Process and Thread Management:
- Displaying and Selecting: Utilize the Source View Context Panel to list and manage processes and threads, ensuring you can easily switch context to bring any process or thread under debugger control.
- Attaching and Detaching Processes: Through the Main Window, you can attach the debugger to any process not currently under control, via
Command:Attach to Process
. This opens up a dialog box listing active processes accessible to you, streamlining the process attachment.
- Debugging Multi-threaded and Multiprocess Applications:
- Multithreaded Application Handling: For DECthreads multithreaded applications, Ladebug allows the selection among various threads, changing the current thread context to pinpoint and resolve issues efficiently.
- Multiprocess Application Debugging: Ladebug introduces predefined debugger variables such as
$catchforks
,$catchexecs
, and$stopparentonfork
to facilitate debugging in applications that utilize fork/exec processes, enhancing control over multiprocess debugging scenarios.
Must Explore: Becoming A Top Python Backend Developer: Must-Know Technologies
Beyond the tool-specific strategies, adopting a systematic approach to advanced debugging encompasses several key techniques:
- Replication and Simplification: Always replicate the defect before attempting a fix and consider debugging by subtraction to isolate the problem by progressively removing code segments.
- Binary Search and Rubber Duck Debugging: Employ binary search to divide and conquer large codebases, and articulate your problem-solving process to an inanimate object to uncover logical flaws.
- Logging and Deep Diving: Implement mindful logging for a clear snapshot of the system’s state and understand the broader context around the bug for a more accurate fix.
These advanced techniques, combined with a deep understanding of the debugging tools and the specific needs of your application, can significantly enhance your debugging efficiency.
Whether you’re mastering Ladebug for multiprocess and multithread applications or employing strategic approaches like binary search and rubber duck debugging, the key lies in systematic, consistent applications optimized for your debugging scenarios.
Must Explore About Top 10 Full-Stack Developer Frameworks
Specialized Debugging Tools for Full Stack Development
In the realm of full-stack development, having the right debugging tools at your disposal can significantly streamline the debugging process, making it more efficient and less time-consuming.
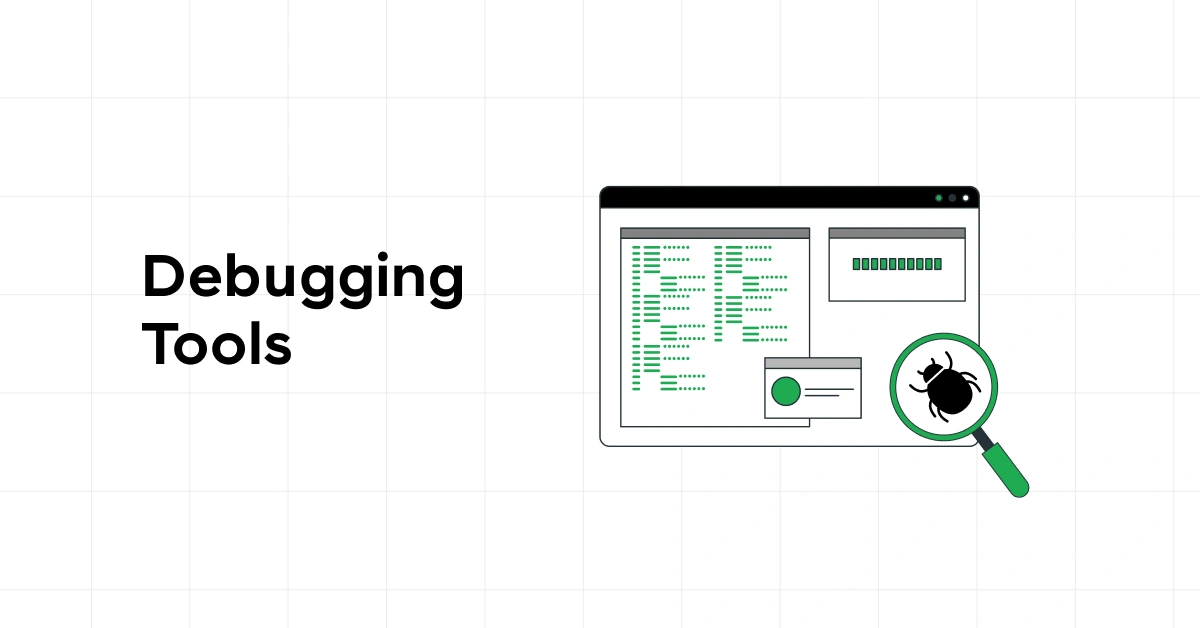
Here’s a breakdown of some specialized debugging tools tailored for different aspects of full-stack development:
Front-end Debugging Tools:
- Chrome DevTools:
- Features: Inspect DOM structure, comprehensive analysis, JavaScript breakpoints.
- Pros: Highly versatile and integrated directly into the Chrome browser.
- Cons: May require a steep learning curve for advanced features.
- Mozilla Firefox DevTools:
- Features: User-friendly interface, powerful JavaScript debugging.
- Pros: Extensive developer community support.
- Cons: Limited cross-browser support.
- Safari Web Inspector:
- Features: In-depth debugging capabilities for iOS and macOS.
- Pros: Native tool for Safari, offering seamless integration.
- Cons: Limited cross-browser support.
Also Read: 10 Best Frontend Development Frameworks
Back-end Debugging Tools:
- Node.js Inspector:
- Features: Setting breakpoints, inspecting variables, CPU and HEAP profiling.
- Pros: Essential for Node.js applications.
- Cons: May require additional setup for remote debugging.
- Eclipse (for Java):
- Features: Step-by-step execution, variable inspection.
- Pros: Comprehensive debugging for Java applications.
- Cons: Can be resource-intensive.
Also Read: Top 10 Backend Web Development Frameworks
Integrated Development Environments (IDEs):
- WebStorm:
- Features: Built-in tools for debugging, testing, and tracing applications.
- Pros: Supports Node.js and client-facing apps.
- Cons: Resource-heavy and potentially complicated UI.
- Visual Studio Code:
- Features: Breakpoints, variable inspection, integrated terminal.
- Pros: Lightweight and highly customizable.
- Cons: Plugins required for certain debugging capabilities.
Explore: 10 BEST Java IDE for Java Programming Language
Additional Tools:
- Debugging Libraries and Frameworks: Enhance debugging experience by troubleshooting specific problems.
- Testing Tools: Automate and evaluate tests, detecting errors and measuring code quality.
- Logging and Monitoring Tools: Collect and analyze data on code behavior and performance.
Each tool and platform offers unique advantages tailored to specific debugging scenarios in full-stack development.
By leveraging these tools, developers can not only expedite the debugging process but also ensure that the software meets the highest standards of quality and performance.
Best Practices and Continuous Learning
In the dynamic world of software development, embracing best practices and dedicating oneself to continuous learning are indispensable for enhancing debugging skills.
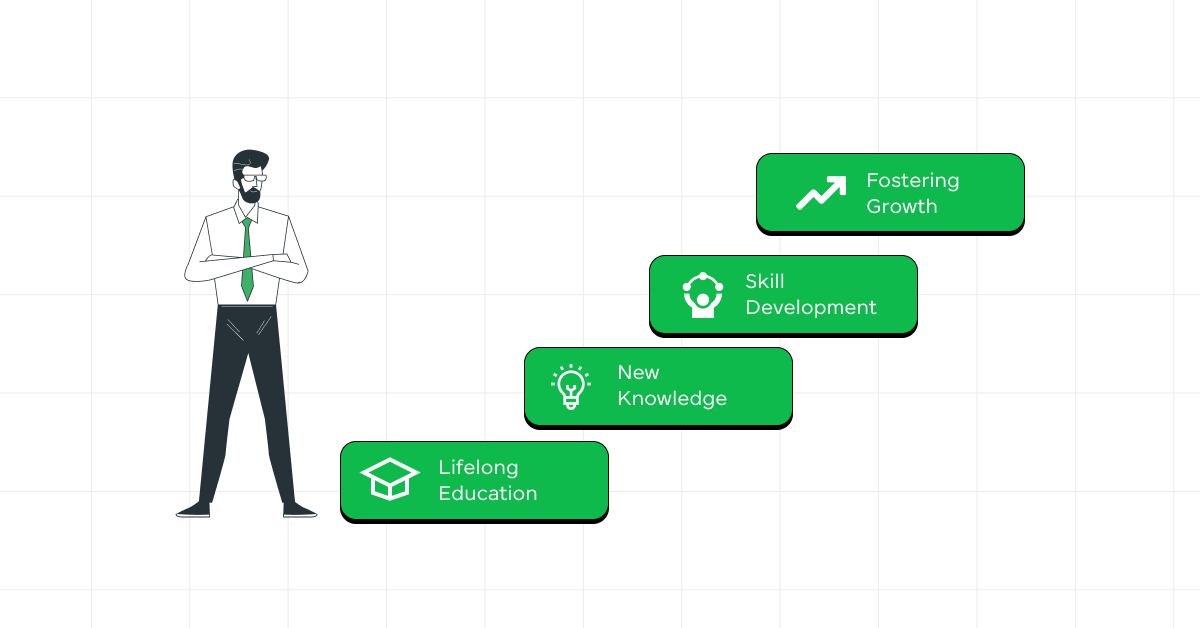
Here are essential strategies and habits that can elevate your debugging expertise:
1) Utilize Debugging Tools Effectively
- Implement Logging: Integrate logging strategically within your code to capture the flow of execution and identify anomalies. Tools like Log4j for Java or Winston for Node.js can be invaluable.
- Leverage Breakpoints and Stepping: Use debugging tools to set breakpoints and step through your code. This allows for a granular examination of code execution and variable states.
- Explore Advanced Tools: Familiarize yourself with advanced debugging tools like reverse debuggers, which can rewind the program state to trace the origin of bugs more intuitively.
Find Out Top 10 Tools Every Full-Stack Developer Should Master
2) Adopt a Methodical Approach to Debugging
- Scientific Method: Approach each debugging session as an experiment. Formulate a hypothesis for the bug’s cause, test this hypothesis, and iterate based on the results.
- Rubber Duck Method: Articulate the problem and your thought process to an inanimate object. This often helps in clarifying your understanding and uncovering overlooked details.
- Consistent Methodology: Whether it’s the scientific method or the rubber duck technique, consistency in your approach ensures a structured path to identifying and solving bugs.
3) Emphasize Continuous Learning and Collaboration
- Stay Updated: Regularly explore new debugging tools, languages, and best practices. Online platforms like GitHub or Stack Overflow offer a wealth of knowledge and updates on the latest trends.
- Collaborate and Seek Feedback: Engage with peers, mentors, and online communities. Sharing debugging experiences and solutions can offer new perspectives and enhance collective knowledge.
- Document and Reflect: Keep a detailed record of bugs encountered, the debugging process, and the resolution. This not only serves as a future reference but also aids in reflecting on and learning from each debugging experience.
By integrating these practices into your routine, you transform debugging from a daunting task into an opportunity for growth and improvement.
Remember, the journey of mastering debugging techniques is continuous, with each challenge offering a chance to refine your skills and contribute to your development as a proficient software engineer.
Must Explore Top Full Stack Development Trends: What to Expect
Concluding Thoughts…
The discussed debugging techniques, from the foundational strategies to more sophisticated techniques like replication, simplification, and the strategic employment of tools like Ladebug and Chrome DevTools, underscore the significance of a systematic approach in enhancing debugging efficiency.
The highly technical nature of debugging demands not only a grasp of these technical strategies and tools but also an attitude of continuous learning and curiosity, which are essential for effective problem resolution and advanced software development.
As developers, the journey of mastering debugging is perpetual, evolving with each new technology, methodology, and challenge.
It presents a unique opportunity to hone one’s technical skills further, ensuring software development not only meets but exceeds the standards of functionality, performance, and user experience.
Also Read: Top 24 Full Stack Developer Interview Questions & Answers
FAQs
Debugging techniques include breakpoint debugging, logging, unit testing, and profiling. Learn more about them in the article above.
JTAG (Joint Test Action Group) debugging is a hardware interface used for testing and debugging embedded systems.
JTAG is a hardware debugging interface, while UART (Universal Asynchronous Receiver-Transmitter) is a serial communication protocol.
USART (Universal Synchronous Asynchronous Receiver-Transmitter) is generally faster than UART because it supports synchronous communication in addition to asynchronous.
Did you enjoy this article?