
Remove an element from a list in Python: 4 Convenient Methods To Look Out For
Jun 10, 2025 4 Min Read 79035 Views
(Last Updated)
A list in Python refers to a data structure in Python that is mutable or changeable. A Python list can contain multiple elements in sequential order. Each value or element inside a list is called an item.
Every element has its unique index number, which is used primarily to access the element.
A list in Python has many methods associated with it, and there are some specific methods used to remove an element from a list in Python.
In this article, we’re trying to decipher some of these methods to remove an element from a list in Python. By the end of this article, you will know which method to pick and when to use them to remove an element from a list in Python.
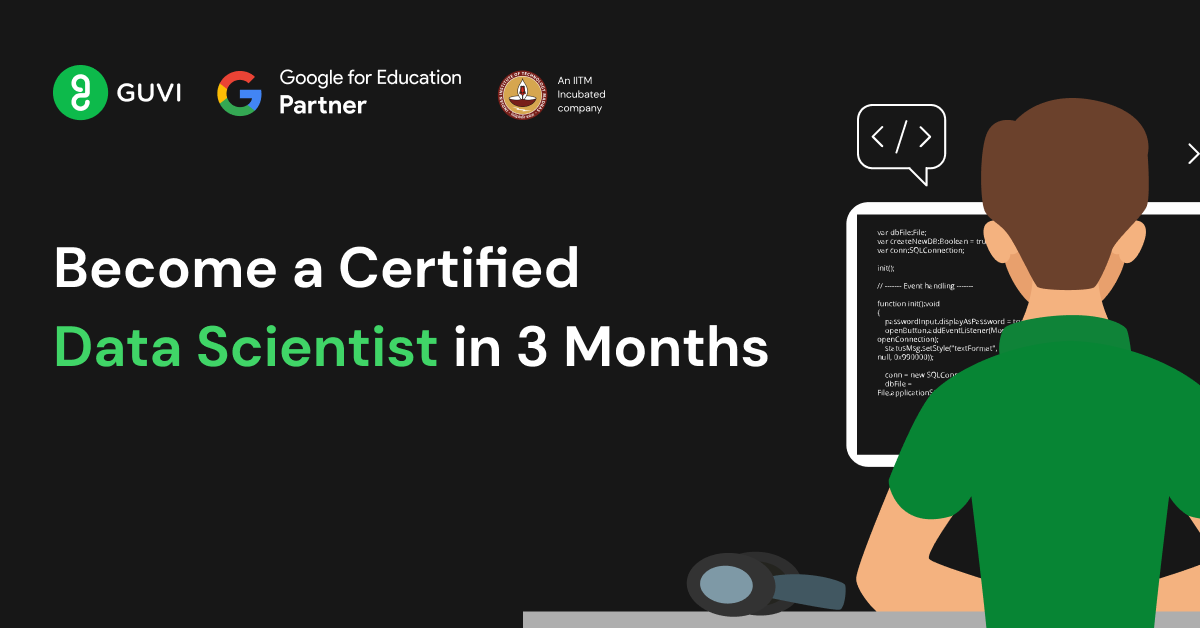
Table of contents
- Why use Python lists?
- List Indexing
- Remove an element from a List
- The Remove ( ) Method
- The Pop( ) Method
- Output
- Output
- Using the Del Operator
- The Clear ( ) method
- Summary
- FAQs
- How do I remove an element from a list by value in Python?
- How do I remove multiple elements from a list in Python?
- What is the difference between remove(), pop(), and del in Python?
- How can I remove elements from a nested list?
Why use Python lists?
Before we address the main topic of learning to remove an element from a list in Python, let us first understand why one should use Python lists.
Every programming language has some built-in data structures, which are most extensively used to access data collection. Python also includes several array-like structures in its standard library, and each has its significant attributes.
Unlike C++ or similar low-level languages, which don’t support dynamic data types, storing varied data types like float, string, or a list inside a list is feasible in Python.
In other programming languages, a list only explicitly consists of strings. Whereas a list in Python stores varied data types in a single collection.
In addition, slicing and indexing are also supported by Python Programming, implying that they can be modified after initialization.
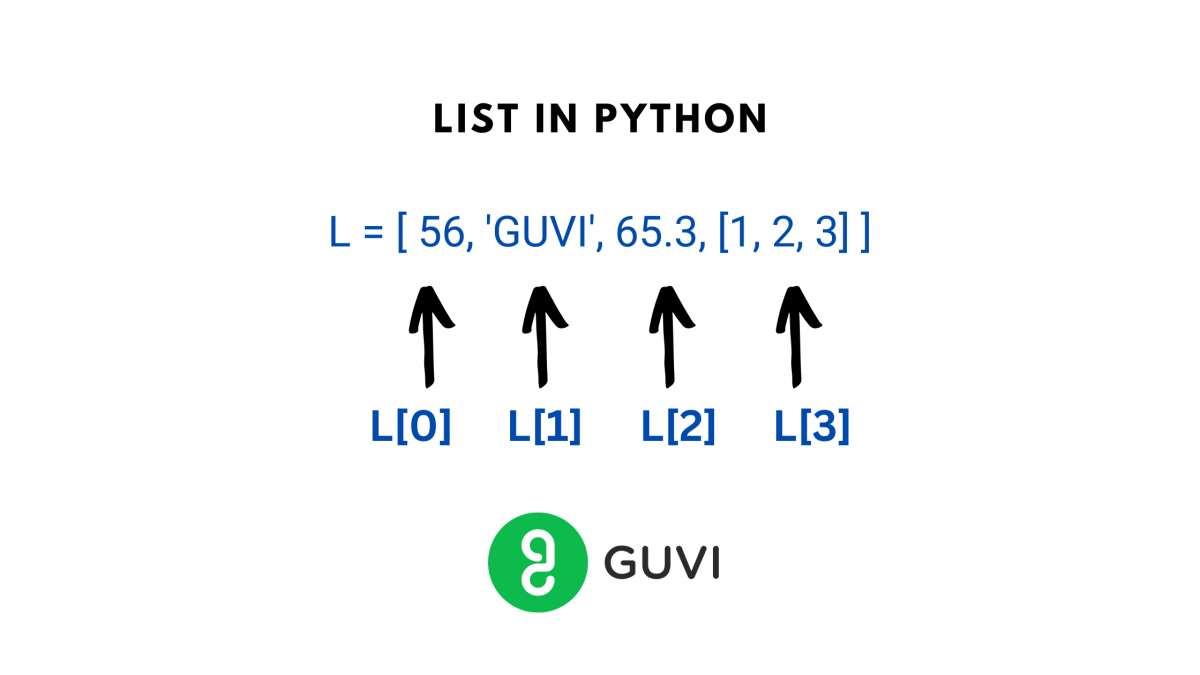
Just like depicted in the picture above, all the elements in the lists are enclosed within square brackets [ ], and every element in the list is separated by a comma (,). A list can also contain another list inside that list, known as a nested collection of lists. To remove an element from a list in Python is as easy as a rewarding process.
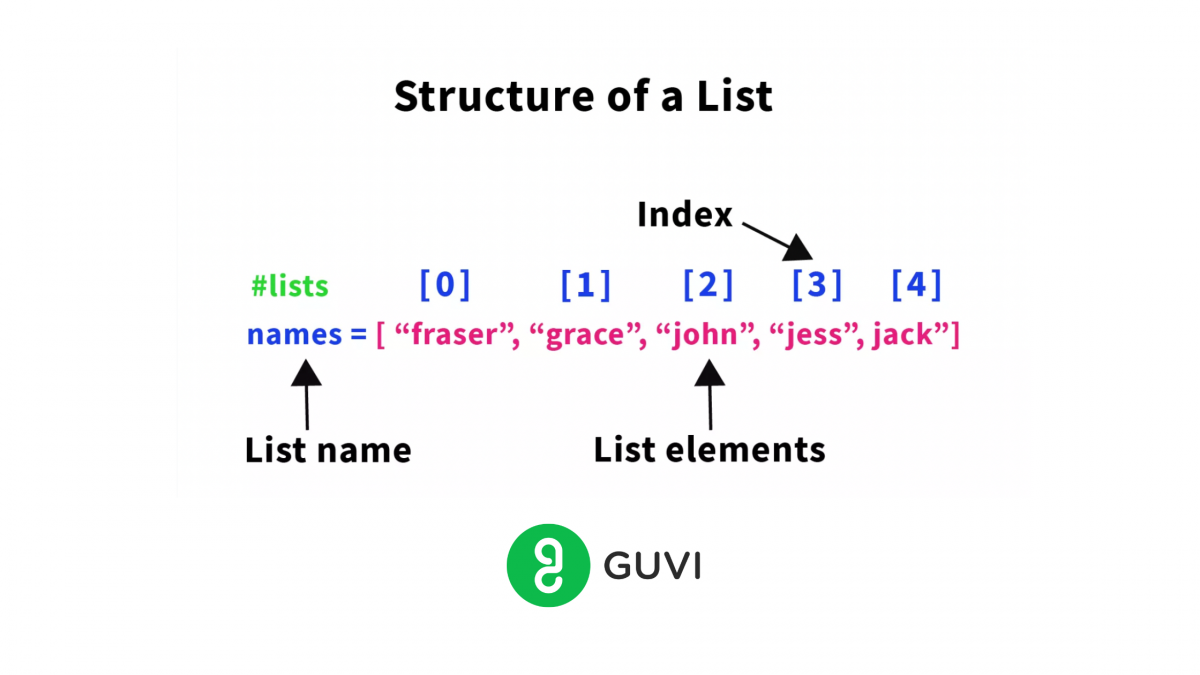
Before diving into the next section of understanding how to remove an element from a list in Python, ensure you’re solid on Python essentials from basics to advanced-level. If you are looking for a detailed Python career program, you can join GUVI’s Python Course with placement assistance. You will be able to master Multiple Exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-paced course, try GUVI’s Python Course.
Also Read: 12 Key Benefits of Learning Python in 2025
List Indexing
In the first image, we have defined a list called “L”. Lists in Python are like variable-length arrays, and we can access all the elements with an Index. The starting index is always set to 0.
For List “L”
At index 0, we have the Integer ‘56’.
At index 1, we have a string named “GUVI”.
At index 2, we have a floating-point number of 65.3.
At index 3, we have a nested collection of lists [ 1, 2, 3 ]
This way, we can store elements of different types in a single list.
Indexing is essential to get a grasp on list methods prevalent in Python so that it provides ease for users to remove an element from a list in Python. Now that you’ve mastered it, let’s see the methods to remove an element from a list in Python.
Explore: Learn Python in 2025: 10 Interesting Reasons to Do So!
Remove an element from a List
Normally, there are four ways in which you can remove an element from a list in Python.
- The Remove( ) Method
- The Pop ( ) Method
- Using the del Operator
- The Clear () Method
Let’s elaborate on them one by one.
1. The Remove ( ) Method
The remove ( ) method is a list method to remove an element from a list in Python. The remove () method removes an item from a list by its value and doesn’t use the index number attribute. Please note that when using the remove method, it will search and only remove the first hit of an item.
This implies that in case if there is more than one instance of an item whose value you have passed as an argument to the method, then only the first occurrence will be removed.
The general syntax of the remove() method looks like:
list_name.remove(value)
ListName conveys the name of the list you have been working on.
Remove() is one of Python’s in-built methods.
Please note that remove() takes one single argument. If you do not provide that you will get a TypeError.
value
is the specific value of the item that you want to remove from list_name
. In case, the specified value does not exist in the list, you’ll get an error named as ValueError.
#original list
Harry_Potter_Cast = ["Harry", "Draco", "Ron", "Hermione"]
#print original list
print(Harry_Potter_Cast)
# remove the value 'Draci' from the list
programming_languages.remove("Draco")
#print updated list
print(Harry_Potter_Cast)
#output
#['Harry', 'Draco', 'Ron', 'Hermione']
#['Harry', 'Ron', 'Hermione']
Top 10 Python Books for beginners? That can help you learn Python in no-time.
2. The Pop( ) Method
The Pop () method is another most commonly used list object method to remove an element from a list in Python and returns the element as output along with the modified list.
Unlike the remove () method that we saw earlier to remove an element from a list in Python, where we need to specify the value of the element, here we can simply specify the index of the item as an argument, and hence it pops out the specified item to be returned at the specified index. In case the particular item to be removed is not found in the list, IndexError is raised.
Also in case, the index is not specified, it removes the last item in the List and returns it.
my_list = ["hello", "world", "welcome", "to", "GUVIBLOGS"]
# removing the last element from the list.
popped = my_list.pop()
print("The poped element is:", popped)
print("Now the list is:",my_list)
Output
The popped element is: GUVI
Now the list is: ['hello', 'world', 'welcome', 'to']
One more instance with specified index.
my_list = ["hello", "world", "welcome", "to", "GUVIBLOGS"]
# removing a specific element using the index value.
popped = my_list.pop(3)
print("The poped element is:", popped)
print("Now the list is:",my_list)
Output
The popped element is: to
Now the list is: ['hello', 'world', 'welcome', 'GUVIBLOGS']
3. Using the Del Operator
The Del Operator is almost similar to pop method with just one subtle difference. Unlike pop method, where the specified index element is returned, in the case of the del operator, the element is simply deleted and not returned.
So essentially, this operator takes the item’s index to be removed as the argument and deletes the item at that index. The del operator also supports removing a range of items from the list as well as remove an element from a list in Python. Similar to pop () method, the del operator raises IndexError when the index or indices specified are out of range.
>>> list_int = list(range(10))
>>> print(list_int)
[ 1, 2, 3, 4, 5, 6, 7, 8, 9] # List of Integer
>>> del list_int[5]
>>> print(list_int)
[ 1, 2, 3, 4, 5, 7, 8, 9] # delete element at index 5
>>> del list_int[-1]
>>> print(list_int)
[ 1, 2, 4, 5, 6, 7, 8] #delete element at index -1 or last index
Let’s see some more instance where del operator is used to remove an element from a list in Python
>>> list_int = list(range(10))
>>> print(list_int)
[ 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> del list_int[2:5]
>>> print(list_int)
[0, 1, 2, 6, 7, 8, 9]
# from index 2 to index 4. Last Index is exclusive
>>> list_int = list(range(10))
>>> del list_int[:3]
>>> print(list_int)
[ 4, 5, 6, 7, 8, 9] # 0th index till 2nd index item deleted.
4. The Clear ( ) method
Just like the name suggests, the clear () method is used to remove all the items in the Python List. This is actually the easiest method to remove an element from a list in Python. Similar to the remove () method, it returns a None value.
my_list = [1,2,3,4,5,6,7,8, GUVI]
# remove all elemets from the list
my_list.clear()
print(my_list)
//Output
[]
Kickstart your Programming journey by enrolling in GUVI’s Python Course, where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python course.
Explore: 18 Famous Websites Built with Python in 2025
Summary
We hope you were able to go through this article and get a fair understanding of the methods and operators used to remove an element from a list in Python. In case of a range of elements to be removed, always use the del operator.
On the other hand, use the above given methods logically & conclusively for minimal machine load. For more such articles on Python, keep following this space.
FAQs
Use the remove()
method. For example, my_list.remove('apple')
will remove the first occurrence of ‘apple’ from my_list
.
You can use list comprehension to create a new list that only includes the elements you want to keep. For example, [x for x in my_list if x not in elements_to_remove].
remove()
deletes by value, pop()
deletes by index and returns the value, and del
can remove elements by index or slices and does not return a value.
You would need to iterate through each sublist and remove elements using any of the methods mentioned.
Did you enjoy this article?