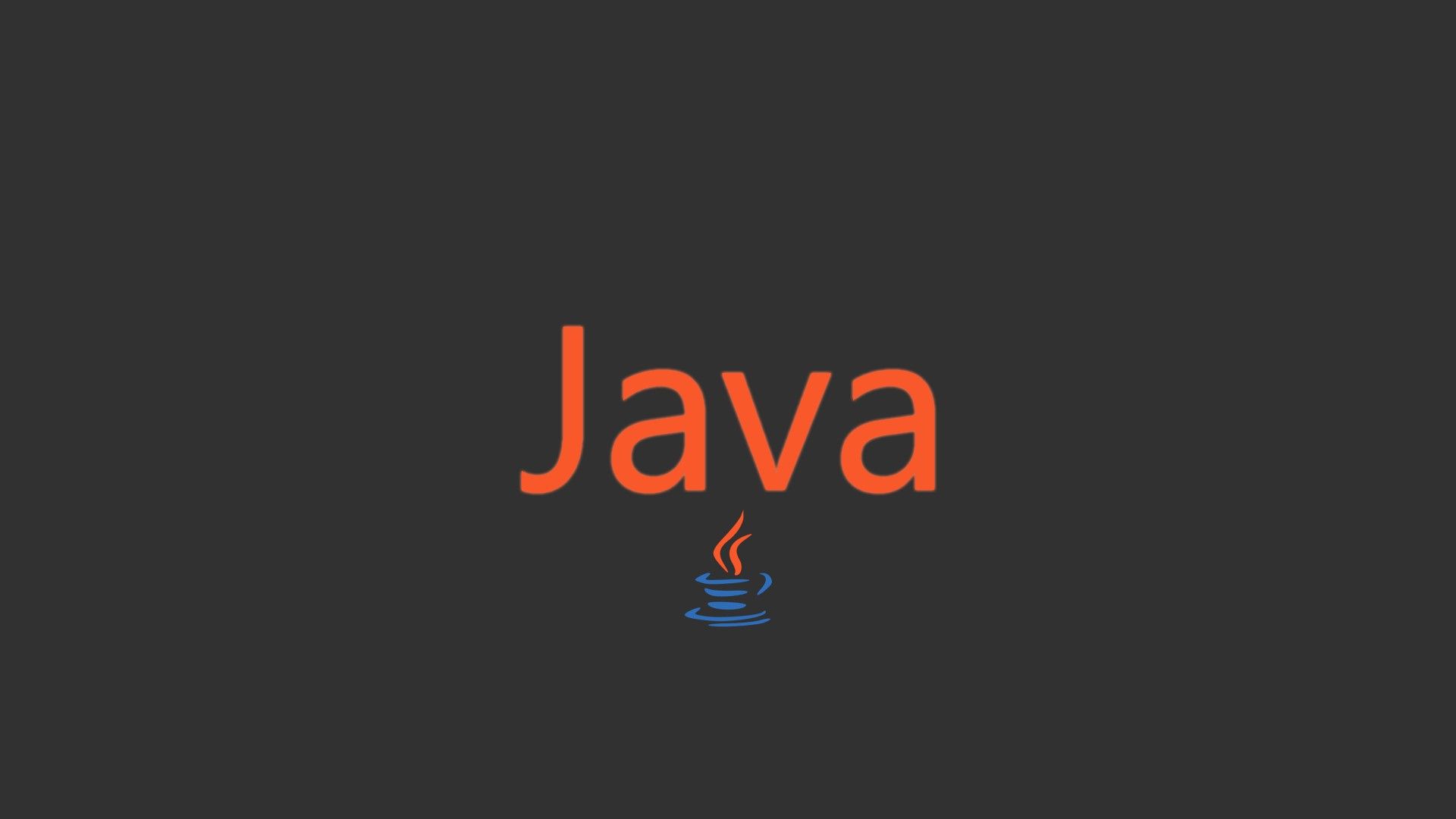
Data Types in JAVA: Primitive VS Non-Primitive Data Types
Jul 10, 2025 5 Min Read 10712 Views
(Last Updated)
In case you’re going to use the JAVA programming language in your career, the first and foremost thing you need to know is how to declare a variable. Variables are nothing but reserved memory locations to store values.
The fundamental question arises as to what kind of data the variable stores. So the data types in programming specify the various sizes and values that can be stored in the variable. Basically, there are two types of data types in Java.
- Primitive Data types – include boolean, char, byte, short, int, float, long & double.
- Non-primitive Data Types – include Interfaces, Classes, and Arrays.
There can be a lot of confusion about the computation of a program if the variables’ data types are not known. In this tutorial blog, we are going to deconstruct both primitive and non-primitive data types in Java and go over each type.
Table of contents
- Primitive Data Types
- INT
- Float
- Syntax
- Double
- Boolean
- char
- Short
- Long
- Byte
- Non-Primitive Data Types in Java
- Classes
- Interface
- Strings
- Array
- Summing Up
- FAQs
- How many primitive data types are there in Java?
- What is a nonprimitive data type in Java?
- How to check data type in Java?
- Is string a primitive data type in Java?
- How to find data types in Java?
Primitive Data Types
The eight primitive data types in Java are int, boolean, short, long, char, double, float, and byte. Unlike non-primitive data types, these aren’t considered real objects and represent raw values.
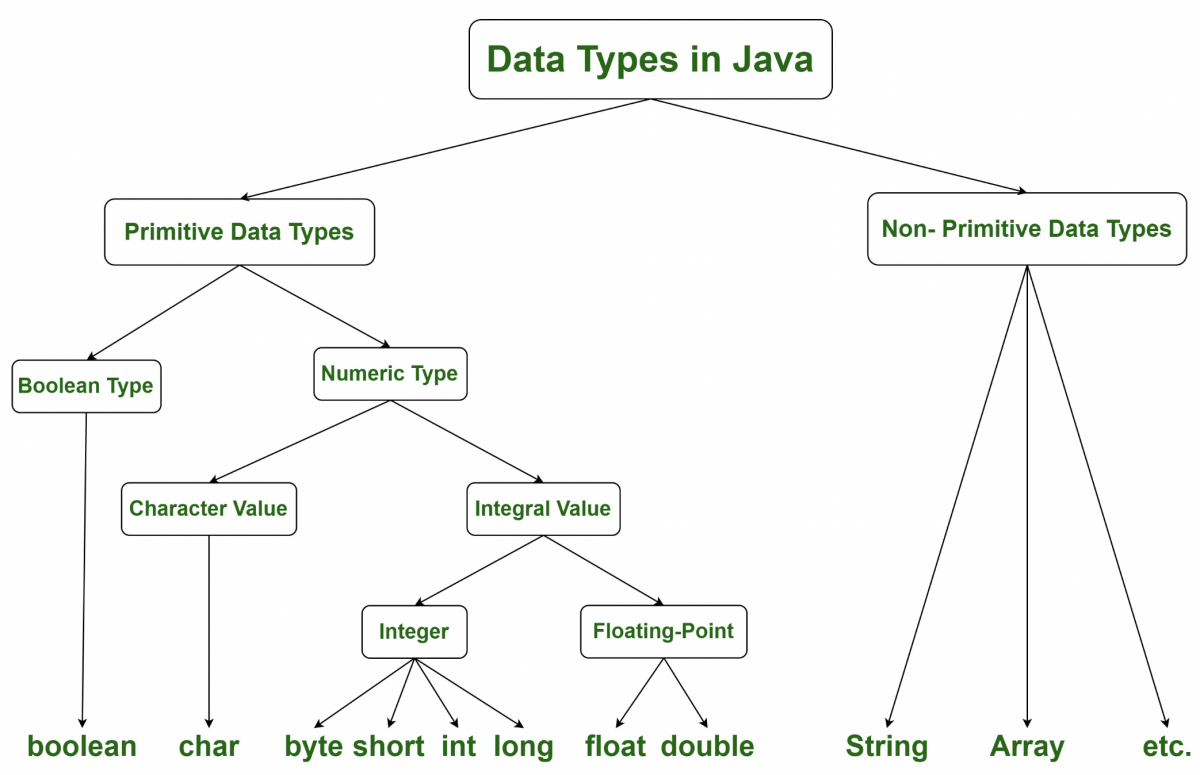
They are predefined and available within the Java programming language. Primitive data types do not interact with other primitive data types. Without primitive data types, it will be impossible to frame programs; they are also considered building blocks for the non-primitive data types.
They are stored directly on the stack. Now, as per the memory management in Java, we’ll take a larger look at default values, storage size, and examples of how to use each type.
Before proceeding further, make sure you have a strong grasp of essential concepts in Java Full Stack Development, including front-end frameworks, back-end technologies, and database management. If you’re looking for a professional future in Java Full Stack Development, consider joining GUVI’s Java Full Stack Development Career Program. With placement assistance included, you’ll master the Java stack and build real-world projects to enhance your skills.
Also, if you’re looking for a self-paced course in Java in your language, you can go for GUVI’s Java Course with Certification to help you master Java from the basics to an advanced level.
INT
The first primitive data type we’re going to uncover in the series is int. Int is short for integer, and the int type holds a wide range of non-fractional number values. To be specific, JAVA stores it using 32-bit memory. The default value of any int value is O.
In JAVA SE 8 & later, one can use the int data type to represent an unsigned 32-bit integer, which has a value in the range [0, 232-1]. You can use the integer class to use the int data type as an unsigned integer.
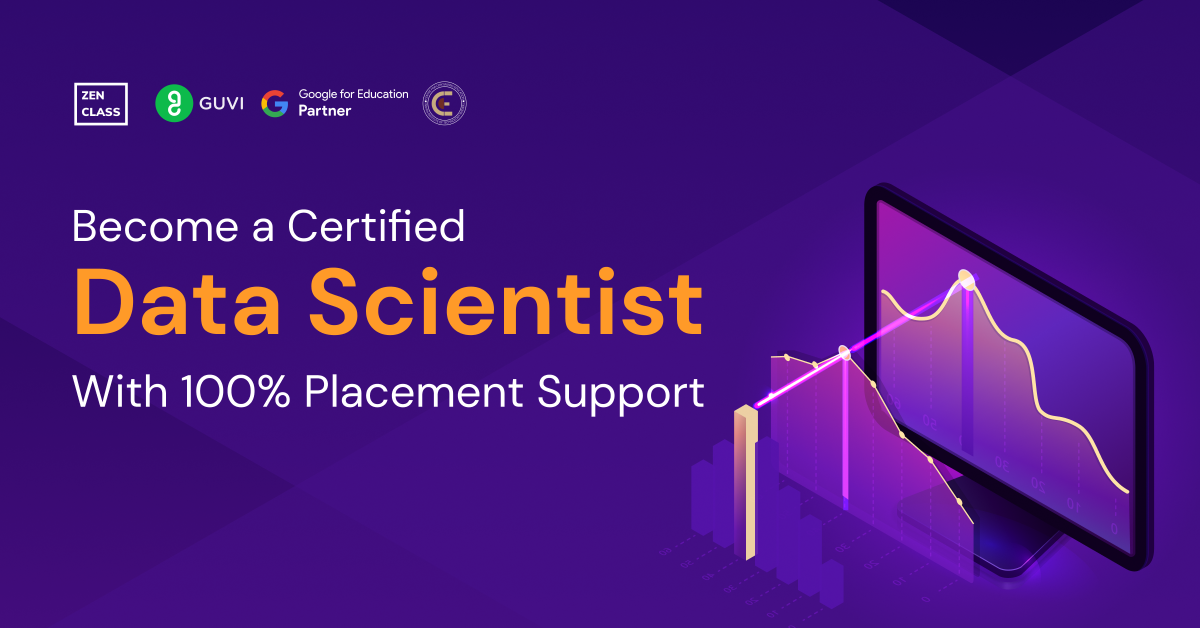
Syntax
int x = 424_562;
int y;
If a variable is defined in a method, you must assign a value before you can assign it in a function. You can perform all the arithmetic operations on ints, just be aware of the fact that decimal values will be minced off when performing these on integers.
Float
For basic fractional numbers, we use float data type. This is a single-precision decimal number, which implies that if we get past six decimal points, the number will become less precise and more of an estimate.
The Float data type is a single-precision 32-bit IEEE 754 floating point. Use a float (instead of double) if you need to save memory in large arrays of floating-point numbers.
More or less, in most cases, we won’t care about the precision loss. But if our case requires scientific precision, we need to use specific data types designed for this work. This type is stored in 32 bits of memory just like int.
However, because of the floating decimal point, its range is much different. It can represent both positive and negative numbers. & the default value is o.o instead of o.
Syntax
float f = 4.156f;
flaot f;
Double
Just like the name suggests, the double data type is a double-precision 64-bit floating point. This implies that it can represent a much larger range of possible numbers than float. Although It suffers from the same limitation limit as float does. The default value is also 0.0 similar to float & to declare the literal as a double, we also add the letter “D” at the end.
dobule d = 5.384094576304495056574846D;
dobule d;
Boolean
If you’re into programming, you would have guessed it. Boolean is the simplest primitive data type for every programming language; in our case JAVA. It can only contain two values: True or False. It stores its value in a single bit. However, JAVA for convenience pads the value and stores it in a single byte.
Here’s how to declare boolean
boolean b = flase;
boolean b;
Boolean data types are the cornerstones of controlling our program flow
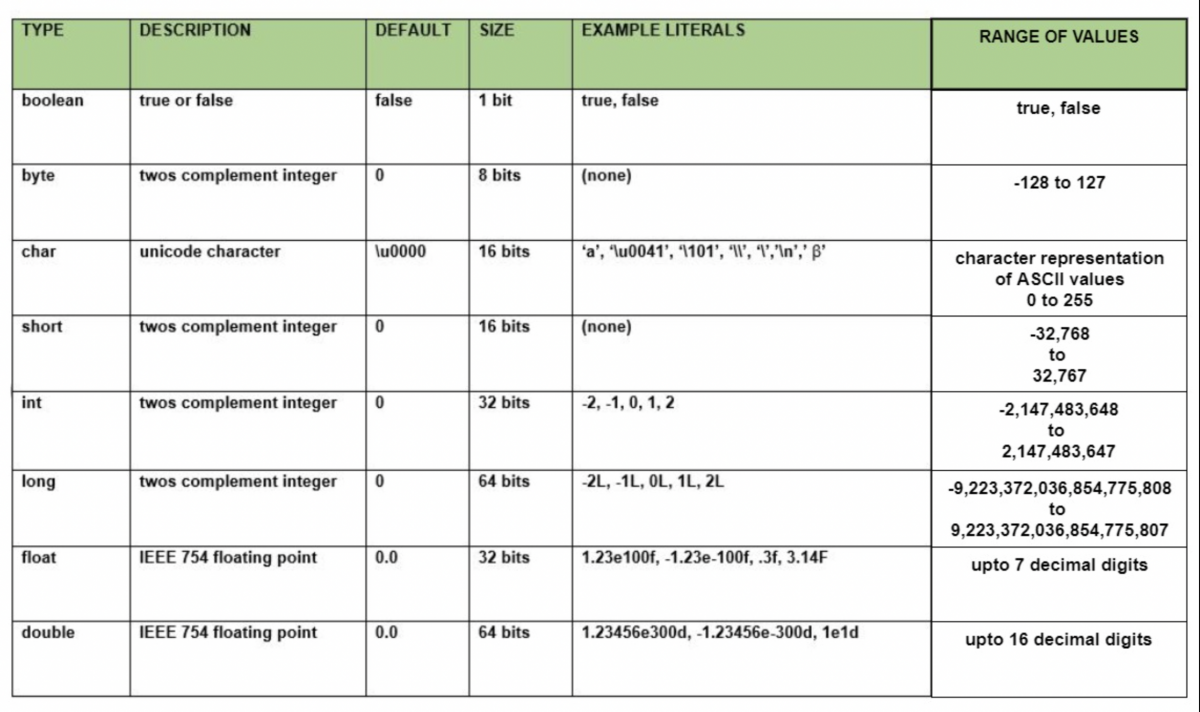
char
char is short for the character. it is a 16-bit integer representing a Unicode-encoded character. Its range is from 0 to 65,535. In Unicode, this represents ‘\u0000′ to ‘\uffff’.
For a list of all possible Unicode values, check out websites such as Unicode Table.
Syntax to declare char
char c = 'a';
char c = 65;
char c;
Short
The next primitive data type in Java is short. In case you want to save memory and the byte is too small, we can also use halfway between byte and int short. At 16 bits of memory, it’s half the size of an int and twice the size of a byte. Its range of possible values is -32,768(-215) to 32,767(215 – 1).
Syntax
short s = 30_030;
short s;
Similar to other data types in Java, the default value is 0. We can also use other standard arithmetic on short as well.
Long
Long is the last integer data type in Java. Long is a big brother of int as it stores 64 bits of memory. Compared to int, it can store a significantly larger set of possible values.
The possible values of a long are between -9,223,372,036,854,775,808 (-263) to 9,223,372,036,854,775,807 (263 – 1).
It is useful for certain occasions when int data type is not able to accommodate the desired value.
Syntax
long l = 1_455_568_890;
long l;
Byte
byte is similar to int data type, except it only takes an 8-bit memory, that’s why it’s referred to as a byte.
Because the memory size is so small, bytes can only hold values from -128 (-27) to 127 (27 – 1). The default value of the byte data type is 0.
Here’s how we can create a byte:
byte b = 69;
byte empty;
Non-Primitive Data Types in Java
Non-primitive data types in Java are also known as reference types because they refer to objects. These are the datatypes that have instances like objects.
Unlike primitive data types in Java which are predefined, the non-primitive data types are created by the programmer and are not defined by the Java language. (except for string) Primitive data types are building blocks for any program, however non-primitive data types in Java are used to call methods to perform certain operations, which primitive data types can’t.
The other differences found between primitive and non-primitive data types include:
- A primitive type starts with a lowercase letter, while a non-primitive type starts with an uppercase letter.
- A primitive type has always a value, while non-primitive types can be
null
. - The size of a primitive type depends on the data type, while non-primitive types are all the same size.
They are primarily class, objects, strings, interfaces, or arrays. Let’s deconstruct them one by one.
Classes
A class is a user-defined blueprint or prototype from which objects are created. It basically represents the set of methods or properties that are common to all objects of one type. Everything in Java is associated with objects and classes.
In layman’s terms, if a car is an object, The car has attributes, such as weight and color, and methods, such as drive and brake. A Class is like an object constructor or a “blueprint” for creating objects.
To create a class with public access
public class Main {
int x = 5;
}
To take it up a notch further
public class GUVI {
int a,
b,
c;
Static void teach() {
System.out.println(“Hi I am teaching at GUVI”);
}
void learn() {
System.out.println(“Hi I am learning at GUVI”);.
}
}
NOTE
- A class can have public or default access.
- The class name’s should always begin with the initial letter. (capitalized for convention)
- The class body is always surrounded by {} braces.
Python vs. Java? Which programming language to master in 2025?
Interface
Similar to class, an interface can have methods and variables, but with one prime difference, i.e. the methods are abstract by nature. which implies that they have no “body.”
If any class is implemented as an interface, then the programmer must declare all the details to every function of the interface, if not, then we must declare the class as abstract. So basically an interface specifies what a class must do and not how. it’s the blueprint or prototype of the class.
interface DataFlair {
void teach();
void learn();
}
class DataFlairCls implements Zenclass {
public void teach() {
System.out.println("I teach at Zen Class");
}
public void learn() {
System.out.println("I learn at ZEN Class");
}
public static void main(String[] args) throws IOException {
Zenclass Cls ob = new ZenclassCls();
ob.teach();
ob.learn();
}
}
Strings
Strings are usually known as an array of characters. The only difference between an array and a string is that a string is designed to hold a sequence of characters in a single variable, while an array is a collection of separate char-type elements.
Unlike other programming languages such as C or C++, strings in Java do not end with a null character. Also, Java features many methods of manipulating strings such as length, substring, and many more.
How to declare strings in JAVA
// Declare String without using new operator
String s = "GUVIZENCLASS";
// Declare String using new operator
String s1 = new String("GUVIZENCLASS");
Array
The indexed arrays are special memory locations that are used to store a collection of homogenous (liked-type variables) data. All the variables in the array are indexed, always starting from zero.
Another fascinating thing about arrays is that they can be used as a static field, a method perimeter, and a local variable. However, the direct superclass of an array type is known as an object. Unlike C & C+=, the length of the array can be measured using member length.
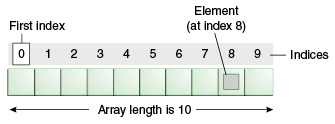
Syntax
class Testarray{
public static void main(String args[]){
int a[]=new int[5];
a[0]=10;
a[1]=20;
a[2]=70;
a[3]=40;
a[4]=50;
//traversing array
for(int i=0;i<a.length;i++)
System.out.println(a[i]);
}}
Summing Up
To add a conclusion, these are major data types in JAVA. Without classifying these data types in Java, it will be impossible to deconstruct various varieties of inputs and variables. Hence a solid foundational knowledge is eminent for fluid coding in Java Programming Language.
Kickstart your tech Java career now and get placed with a handsome salary package with GUVI’s Java Full Stack Development Career Program, providing placement assistance. Master essential technologies including Java, Maven, Eclipse, HTML, CSS, MongoDB, and more while working on practical real-world projects to enhance your expertise.
You can also accelerate your career with an awesome course on JAVA offered by GUVI, which has 11 hours of recorded sessions and is subdivided into modules for better understanding. You must go and register now for this course and explore Java.
FAQs
There are basically 8 primitive data types in Java. The above list has mentioned all of them. They are char, int, and double. long, short, boolean, float, and byte.
Non-primitive data types are the ones that have an instance-like object. Unlike primitive data types in Java which are predefined, the non-primitive data types are created by the programmer and are not defined by the Java language.
Sometimes, we do require to check the data type of a variable to perform logical operations. To check data type in Java, use getclass() and getSimpleName() method to get class and names respectively.
No, it’s not. As it’s not predefined, a user has to program and declare it in code.
As mentioned in question no. 3, we can check the type of a variable in Java by calling getClass().getSimpleName() method via the variable.
Did you enjoy this article?