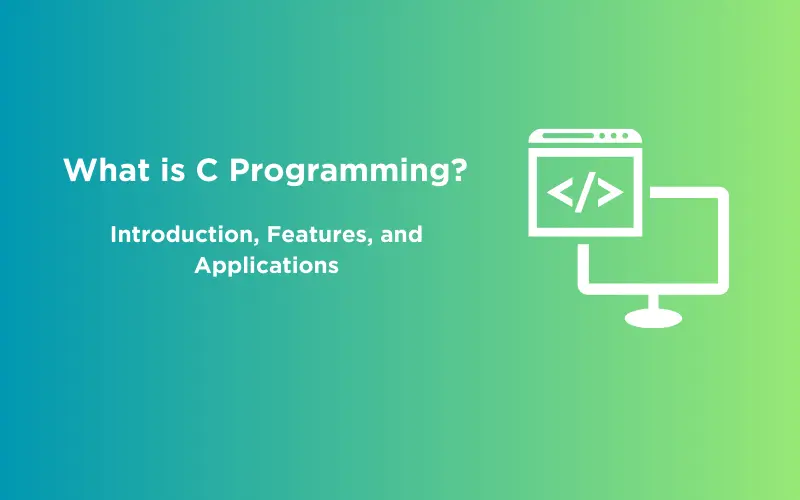
What is C Programming? Introduction, Features, and Applications
Apr 22, 2024 5 Min Read 13478 Views
(Last Updated)
C programming is a versatile and powerful language that has played a significant role in shaping the world of computer programming. It was developed at Bell Laboratories by Dennis Ritchie in the early 1970s. Over the decades, C has evolved and remains one of the most widely used programming languages in the world.
In this article, we will explore the essence of C programming, its fundamental features, basic syntax, various applications, and many more. Whether you are a beginner eager to start your programming journey or an experienced programmer looking to expand your skill set, understanding C is essential. Let’s begin your journey now:
Table of contents
- What is C Programming?
- Features of C Programming
- Portability
- Efficiency
- Flexibility
- Rich Standard Library
- Community Support
- A Simple Hello World Program
- Data Types of C Programming
- Basic Data Types
- Derived Data Types
- Enumeration Data Type
- Operators and Their Types
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Increment and Decrement Operators
- Bitwise Operators
- Ternary Operator (Conditional Operator)
- Functions
- Loops and Their Types
- for loop
- while Loop
- do-while Loop
- Conditional Statements in C Programming
- Application of C Programming
- System Software Development
- Application Software
- Game Development
- Embedded Systems
- Scientific Computing
- Web Development (Backend)
- Compilers and Interpreters
- Conclusion
- FAQs on C Programming
- Is C programming difficult to learn?
- What is the difference between C and C++?
- Is C programming still relevant in today's software development landscape?
- What is the best way to start learning C programming?
What is C Programming?
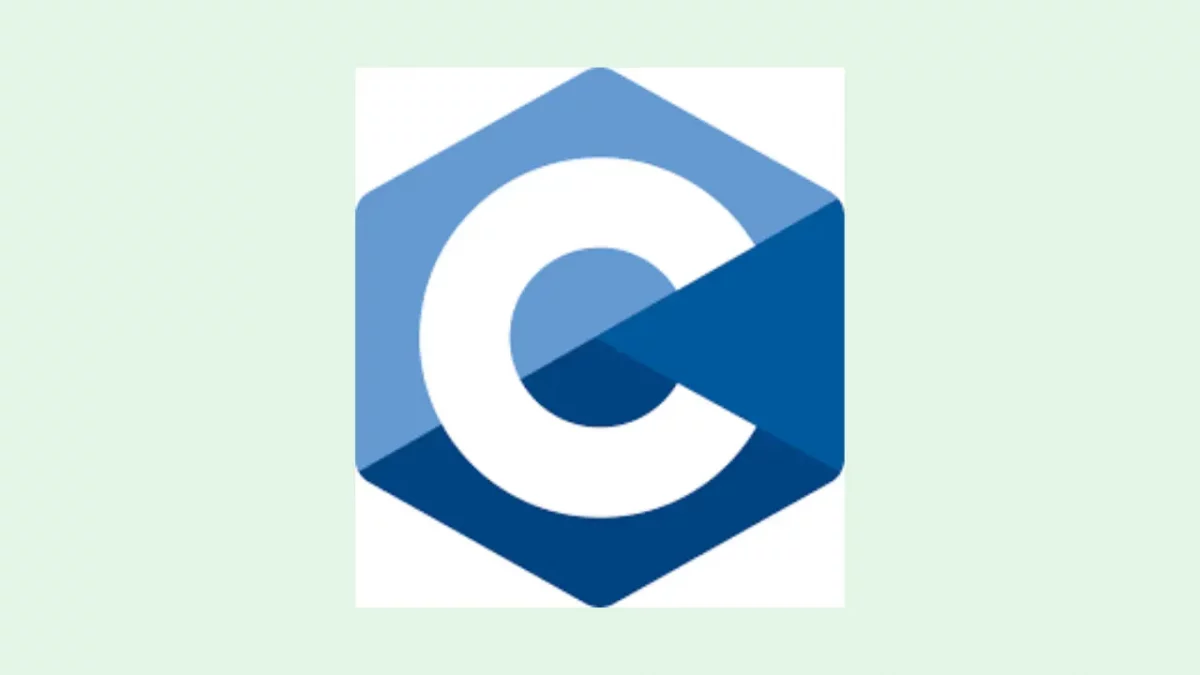
C programming is a high-level programming language that is known for its efficiency, portability, and flexibility. It is often referred to as the “mother of programming language”, a middle-level language because it combines elements of both high-level and low-level languages. C has influenced the development of many other programming languages, including C++, Java, and Python.
At its core, C is a procedural programming language. This means that programs written in C are structured as a series of functions or procedures. Each function can perform specific tasks and can be called from other parts of the program. This modular approach to programming makes C code organized and easy to maintain.
If you’re interested in a C programming course, GUVI has an amazing self-paced course that covers 4 modules of basic to advanced levels.
Features of C Programming
C programming possesses several notable features that have contributed to its enduring popularity:
1. Portability
C programs can run on a wide range of computer systems with minimal modification. This portability is due to the ANSI C standard, which defines the language’s syntax and semantics. As long as a system has a C compiler that adheres to this standard, C code can be compiled and executed without major issues.
2. Efficiency

C is known for its ability to produce highly efficient code. It allows low-level memory manipulation and direct access to hardware, which is crucial in systems programming and embedded systems development. This efficiency makes C an ideal choice for developing operating systems, device drivers, and performance-critical applications.
3. Flexibility
C provides a wide range of data types, operators, and libraries, allowing programmers to have fine-grained control over their code. This flexibility enables developers to write code that closely matches the problem they are trying to solve, making C suitable for a wide variety of applications.
4. Rich Standard Library
C comes with a comprehensive standard library that provides functions for common operations like input/output, string manipulation, and mathematical calculations. These library functions save time and effort for programmers by offering pre-built solutions to common programming tasks.
5. Community Support
C has a vast and active community of developers, which means there are numerous online resources, forums, and libraries available for those learning or working with C. This community support makes it easier to find help and resources when needed.
A Simple Hello World Program
To start your journey in learning C programming language, let’s start with the “Hello, World!” program. This program is often used as a beginner’s introduction to any programming language and serves as a simple way to demonstrate the basic structure of a C program.
#include <stdio.h>
int main()
{
printf("Hello, World!\n");
return 0;
}
In this program:
#include <stdio.h>
: This line includes the standard input/output library, which provides theprintf
function for printing text to the console.int main()
: This is the main function where the program execution begins. Theint
beforemain
indicates that the function returns an integer value.{}
: The curly braces define the scope of themain
function. Inside these braces, we write the code that the function executes.printf("Hello, World!\n");
: This line uses theprintf
function to display the text “Hello, World!” followed by a newline character (\n
) to the console.return 0;
: Finally, thereturn 0;
statement indicates that the program has terminated successfully. The value0
is typically used to indicate success, while non-zero values can indicate errors.
Compile and run this program, and you’ll see the familiar “Hello, World!” message displayed on your screen.
Data Types of C Programming
In C, data types are essential for specifying the kind of data that a variable can hold and the operations that can be performed on it. C provides several basic data types, which can be categorized into the following groups:
1. Basic Data Types
- int: Used for integer values, e.g.,
int x = 10;
- char: Represents a single character, e.g.,
char grade = 'A';
- float: Used for floating-point numbers, e.g.,
float price = 19.99;
- double: Similar to
float
but with higher precision, e.g.,double pi = 3.14159;
2. Derived Data Types
- Array: A collection of elements of the same data type, e.g.,
int numbers[5];
- Pointer: A variable that stores the memory address of another variable, e.g.,
int *ptr;
- Structure: A user-defined composite data type, e.g.,
struct Person { char name[50]; int age; };
3. Enumeration Data Type
- Enum: A user-defined data type consisting of a set of named integer constants, e.g.,
enum Days { Sunday, Monday, Tuesday };
These data types allow C programmers to declare variables, define functions, and manage memory efficiently.
Operators and Their Types

Operators in C are symbols used to perform operations on variables and values. Categorically, operators in C can be divided into the following types:
1. Arithmetic Operators
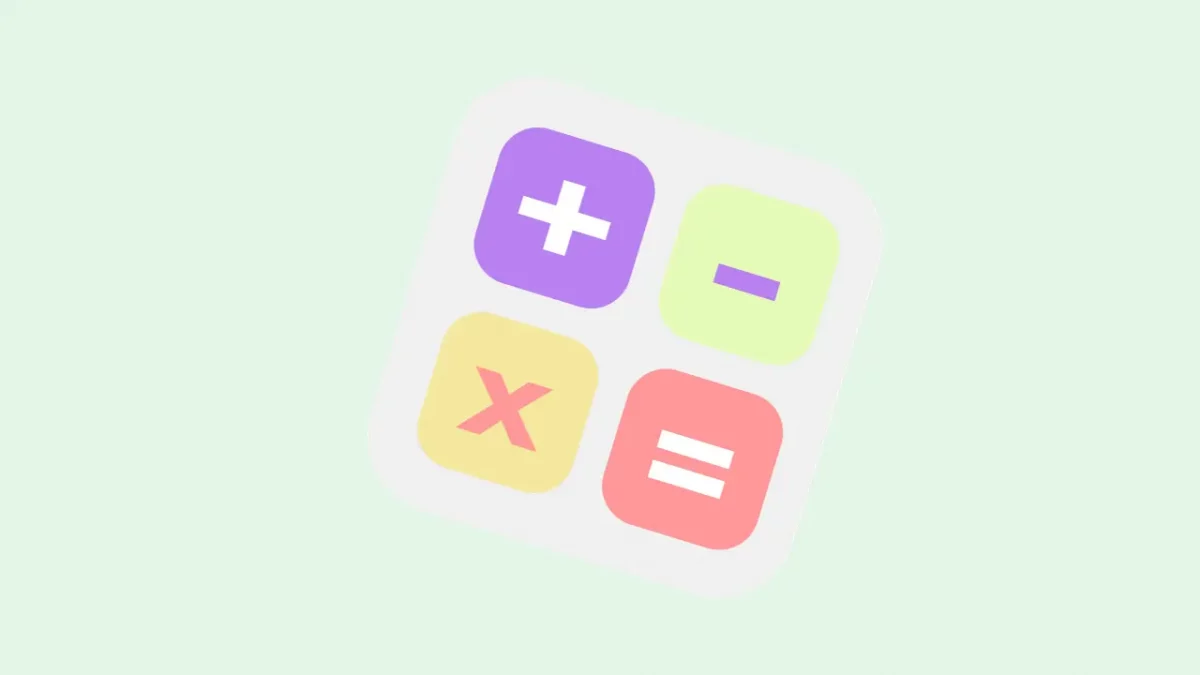
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)
2. Relational Operators
==
: Equal to!=
: Not equal to<
: Less than>
: Greater than<=
: Less than or equal to>=
: Greater than or equal to
3. Logical Operators
&&
: Logical AND||
: Logical OR!
: Logical NOT
4. Assignment Operators
=
: equals+=
: Addition assignment-=
: Subtraction assignment*=
: Multiplication assignment/=
: Division assignment%=
: Modulus assignment
5. Increment and Decrement Operators
++
: Increment by 1--
: Decrement by 1
6. Bitwise Operators
&
: Bitwise AND|
: Bitwise OR^
: Bitwise XOR<<
: Left shift>>
: Right shift
7. Ternary Operator (Conditional Operator)
? :
: Conditional expression
Understanding how to use these operators is crucial for performing various mathematical and logical operations in C programs.
Functions
Functions are blocks of code that perform a specific task. They allow you to break your program into smaller, manageable pieces, making it more organized and easier to maintain. In C, every program must have a main
function, which serves as the entry point to the program.
Loops and Their Types

Loops are used in programming to execute a block of code repeatedly until a certain condition is met. C provides three main types of loops:
1. for loop
The for
loop is used when you know the number of iterations you want to perform. It consists of three parts: initialization, condition, and increment or decrement.
2. while Loop
The while
loop is used when you want to execute a block of code as long as a certain condition is true.
3. do-while Loop
The do-while
loop is similar to the while
loop, but it guarantees that the code block is executed at least once before checking the condition.
Conditional Statements in C Programming
Conditional statements are essential for controlling the flow of your program and making it more dynamic and responsive.
1. if statement: The if
statement is used to execute a block of code if a specified condition is true.
2. if-else statement: The if-else
statement is used to execute one block of code if a condition is true and another block of code if the condition is false.
3. switch statement: The switch
statement is used to select one of many code blocks to be executed.
Application of C Programming
C programming has a wide range of applications across various domains. Here are some notable areas where C is commonly used:
1. System Software Development
C is often used to develop system software, including operating systems (e.g., Unix, Linux, Windows), device drivers, and firmware for embedded systems. Its low-level capabilities make it suitable for tasks that require direct interaction with hardware.
2. Application Software
C is used to build a variety of application software, such as text editors, compilers, and databases. It provides the necessary tools and efficiency to create robust and performance-critical applications.
3. Game Development
Many video games and game engines are developed in C or C++. These languages offer the performance and control needed for real-time rendering and complex game logic.
4. Embedded Systems
C is widely used in the development of embedded systems found in consumer electronics, automotive systems, medical devices, and more. Its ability to work with limited resources makes it a preferred choice in this domain.
5. Scientific Computing
C is used in scientific research and computational modeling due to its efficiency in handling complex calculations. Researchers often use C for simulations, data analysis, and scientific programming.
6. Web Development (Backend)
Although higher-level languages like Python and JavaScript dominate web development, C can be used for developing web server software and optimizing critical components of web applications.
7. Compilers and Interpreters
C is often used to create compilers and interpreters for other programming languages. This bootstrapping process highlights C’s role in building the foundations of other programming ecosystems.
If you’re interested in a C programming course, GUVI has an amazing self-paced course that covers 4 modules of basic to advanced levels.
Conclusion
C programming is a versatile and powerful language with a rich history and enduring popularity. Its efficiency, portability, and flexibility make it suitable for a wide range of applications, from system software to game development and scientific computing.
Understanding C programming is a valuable skill for both beginners and experienced programmers, as it provides a solid foundation for mastering other programming languages and tackling complex software development challenges. In the world of programming, C continues to be a fundamental building block that has stood the test of time.
FAQs on C Programming
1. Is C programming difficult to learn?
Learning C programming can be challenging for beginners, especially if it’s their first programming language. However, it is considered a relatively simple language compared to some modern languages with complex features. With dedication and practice, anyone can learn C programming.
2. What is the difference between C and C++?
C and C++ are closely related programming languages. C++ is an extension of C and includes additional features, such as object-oriented programming (OOP) capabilities. While C is often used for system-level programming and smaller-scale applications, C++ is used for larger software projects, game development, and more.
3. Is C programming still relevant in today’s software development landscape?
Yes, C programming remains highly relevant. It is widely used in systems programming, embedded systems, and other performance-critical applications. Additionally, many modern languages and operating systems are still implemented in C or C++.
4. What is the best way to start learning C programming?
To start learning C programming, you can follow online tutorials, take courses, read books, and practice writing code. Interactive coding platforms and IDEs (Integrated Development Environments) are valuable tools for hands-on learning.
Additionally, joining programming communities and forums can help you get answers to questions and connect with other learners and professionals.
Did you enjoy this article?