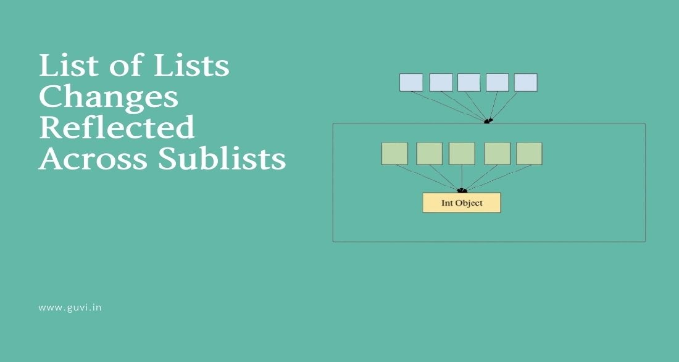
Python | List Of Lists Changes Reflected Across Sublists
Sep 06, 2024 4 Min Read 2608 Views
(Last Updated)
Here it is- the list of list changes across sublists explained with multiple examples.
As a Python user, you might have noticed that you need to perform a specific action a particular number of times. Or you might need to replace the single value of a list of lists. Isn’t it? Yes, you might have to look for an alternative to doing this kind of task.
Well, it is possible by using the range() function of Python. The range() function (in Python 3 and xrange() in Python 2) is one of the in-built functions that is used for creating a sequence of the given numbers.
But sometimes, you have faced the issue of “list of lists changes reflected across sublists.” So, how to solve this kind of issue? Let’s solve it using the range() function and check the other practical methods to solve it.
Table of contents
- What kind of queries concern the list of list changes across subtitles in Python?
- What's executing in the above code?
- Is there any method to solve the issue of the list of lists changes across sublists?
- range()
- deepcopy()
- Attention:
- BONUS POINT:
- Let’s check what you have learned concerning the list of list changes across subtitles!
What kind of queries concern the list of list changes across subtitles in Python?
Sometimes, the user tries to make a list of lists in Python as:
myList = [[0]*4]*3 |
that gives the output as:
[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]] |
But when they want to change any of the inner values like the first element of the first list as:
myList[0][0] = 2 |
that give the output as:
[[2, 0, 0, 0], [2, 0, 0, 0], [2, 0, 0, 0]] |
And it is expected to be as:
[[2, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]] |
So, how to solve this kind of issue in Python. Let’s check it out below.
Before proceeding further, if you want to explore Python through a self-paced course, try GUVI’s Python self-paced course.
What’s executing in the above code?
In the above query, the * cannot make the independent objects as that of the comprehensive list. The reason for it is the multiplication (*) operator.
When a user uses * for multiplication, that is [[0]*4] by 3, it will evaluate the only 1-element list that is [[0]*4]; rather, it needs to evaluate [[0]*4.
Actually, * does not have any idea regarding re-evaluating [[0]*4] and making a copy.
Now, the user can make new references to the current sublist rather than making the new sublists. Anything else might be inconsistent or may need some major redesigning in the primary language designs.
Is there any method to solve the issue of the list of lists changes across sublists?
Yes! There is.
There are three different methods to solve the above query. Users can use any of these methods to replace the first item of a list of lists.
But first, we will describe the method of range() function.
range()
It is used to create the immutable number sequence. It is one of Python’s in-built functions that provides a range of various objects. It includes a series of similar or dissimilar integer numbers.
How to define the range in Python?
Syntax of range() in Python:
range(start, stop[, step])
Here,
=> the start is the lower limit of the sequence. (BY DEFAULT, IT CONSIDERS AS 0). |
=> the stop is the upper limit where the output needs to stop. (THE LAST ELEMENT OF THE LIST IS NEVER INCLUDED IN THE OUTPUT). |
=> the step is kept on adding to the preceding number. (BY DEFAULT, IT CONSIDERS AS 1). |
For example:
To print the 5 elements:
Output:
0
1
2
3
4
Keep in mind: When the Python user passes a single argument to the range, the output is generated the sequence starting from 0 and stopping at -1 of the last value (in the above case 5 elements mean 5-1 = 4. The last element is 4).
In Python, the range() function is used with the for loop to repeat the particular action multiple times. Now, let’s solve the above query.
As the user wants to print the value as [[2, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]], instead the code print the output as [[2, 0, 0, 0], [2, 0, 0, 0], [2, 0, 0, 0]].
Let’s use the range() to get the desired output:
Output
[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
Output
[[2, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
Here, the first range(3) will execute the value [0]*4 three times. But when we provide the write lst[0][0] = 2, it will check and replace the item with indexing 0 in 0 lists with 2.
Finally, this is how a Python user can use the range() function. Now, let’s move to another method.
deepcopy()
The deepcopy() function of Python helps the user to create a copy of the objects. With the help of duplicate copies, users easily make the relevant changes in it.
In the above issue, we can also use the import copy and change the value of any of the items of a list in lists.
Now, let’s understand it with the help of an example
Output
[0, 0, 0, 0]
Output
[[0, 0, 0, 0], [0, 0, 0, 0]]
Output
[[2, 0, 0, 0], [0, 0, 0, 0]]
Whenever there is a need to use the list with the first item 2, call y1. So, this will give the exact value that you need or want.
Attention:
Here, you need to understand the difference between copy() and deepcopy().
As the name copy implies that it makes an exact copy of the original copy. Or we can say that if you make any changes in the copy list, then changes will also occur in the copied list.
Example:
Output:
[[‘AA’, 2, 3], [4, 5, 6], [7, 8, 9]]
[[‘AA’, 2, 3], [4, 5, 6], [7, 8, 9]]
As you can see we have changed the first element of the first row and column, the same output is copied in list 2. This is what we call copy() in python.
On the other hand, deepcopy() is something different. In simple words, we can say that it makes the changes to the specified list only. It does not make the exact copy of the changed list.
Example:
Let’s take the same example of copy() to understand the difference.
Output:
[[‘AA’, 2, 3], [4, 5, 6], [7, 8, 9]]
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
As you can see that we have made the changes in lst1 only and make a deep copy of lst1 in lst2. The lst2 has the previously-stored elements in the list (new changes are not there).
- deepcopy() with for loop
It works the same as the above deepcopy() function. The only difference is that you can generate the list of lists, and then the user can change the value easily.
To perform it, Python user needs to use for loop as:
Output
[0, 0, 0, 0]
Output
[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
Output
[[2, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
So, this is how a Python user can solve the issue of list of lists changes reflected across sublists.
BONUS POINT:
Apart from the above methods towards understanding the list of list changes across subtitles, Python users can implement a direct method to solve the issue of list of lists changes reflected across sublists.
How? Let’s check it!
Output
[[0, 0, 0], [0, 0, 0], [0, 0, 0]]
[[2, 0, 0], [0, 0, 0], [0, 0, 0]]
Do you wish to learn more concepts like the list of list changes across subtitles in Python concepts in depth? Then here is a site you can keep track of – GUVI Blogs on Python. Let’s sum up this!
You can understand that there are different methods to solve the issue of list of lists changes reflected across sublists. Python users can use any of these methods as per convenience.
But I can say that the range() function is the best approach to solve this issue. Range() helps in replacing or changing the value of the list with n number of items in the list of lists. So, that was all that we have on the list of list changes across subtitles in Python.
Let’s check what you have learned concerning the list of list changes across subtitles!
- What is the output of the following code?
x = range(2, 5)
for i in x:
print(i)
- 2
3
4
- [2 3 4]
- Error
- None
Correct Answer: (A), the above code will only print the sequential numbers, not a list. |
2. What is the output of the following code?
list1 = [“xyz”, “abc”, “30”, “20”, “pqr”]
print(list1)
- [xyz, abc, 30, 20, pqr]
- Error
- [‘xyz’, ‘abc’, ‘30’, ‘20’, ‘pqr’]
- None
Correct Answer: (C), the above code will print the list of items with ‘ ‘ inverted commas. |
3. What is the output of the following code?
lis1 = [ 1, 2, 3, 4 ]
lis2 = lis1.copy()
print(lis2)
- Error
- None
- [‘1’, ‘2’, ‘3’, ‘4’]
- [1, 2, 3, 4]
Correct Answer: (D), the above code will print the copied list of items without ‘ ‘ inverted commas. |
4. What is the output of the code?
import copy
x = [0] * 2
print(x)
x1 = [x, copy.deepcopy(x)]
print(x1)
x1[0][0] = 1
print(x1)
- Error
- None
- [[0, 0], [0, 0]]
- [0, 0]
[[0, 0], [0, 0]]
[[1, 0], [0, 0]]
Correct Answer: (D), the above code will print the value by replacing the value of the first item of the first row and column. |
5. Can we change the value of the first element of all the list of lists?
- May be change
- Yes, we can
- May not be change
- No, we can’t
Correct Answer: (B), We can easily change the value of the first element of all the lists using the * operator. |
If you would like to explore Python through a Self-paced course, try GUVI’s Python course with IIT Certification.
Did you enjoy this article?