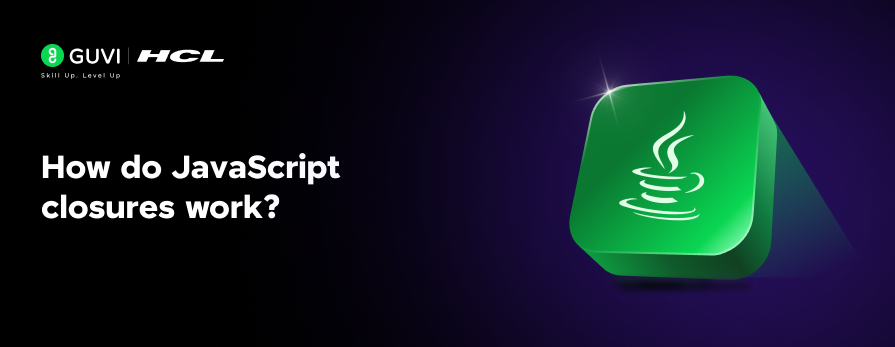
Have you ever come across the term “closure” while learning JavaScript and felt a little lost? Don’t worry; you’re not alone! Closures can seem tricky at first, but once you grasp the concept, you’ll realize they’re everywhere in JavaScript—powering things like callbacks, data privacy, and even module patterns. So, let’s break it down in the simplest way possible and explore some JavaScript closures examples.
Table of contents
- How Do JavaScript Closures Work?
- Why Are Closures Useful?
- Conclusion
- FAQs
- Q1. How does JavaScript closure work?
- Q2. What is the disadvantage of closure in JavaScript?
- Q3. What is the difference between hoisting and closure in JavaScript?
How Do JavaScript Closures Work?
A closure is a function that remembers the scope in which it was created, even after that scope has finished executing. This means a closure can access variables from its parent function, even when that function is no longer active. Understanding JavaScript scope and closures is essential for mastering the language.
Let’s look at an example of a closure function in JavaScript:
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log(`Outer: ${outerVariable}, Inner: ${innerVariable}`);
};
}
const closureExample = outerFunction(“Hello”);
closureExample(“World”);
Output:
Outer: Hello, Inner: World
Here’s what happens step by step:
- outerFunction is called with “Hello”, and it returns innerFunction.
- closureExample now holds a reference to innerFunction.
- When closureExample(“World”) is executed, innerFunction still has access to outerVariable (“Hello”) from outerFunction, even though outerFunction has finished running.
This is what makes closures in JavaScript powerful—they “remember” the variables from their outer scope.
Why Are Closures Useful?
Closures are incredibly useful in JavaScript, and here’s why:
- Data Privacy: Since closures can “remember” variables without exposing them, they help create private variables.
function counter() {
let count = 0;
return function () {
count++;
console.log(count);
};
}
const increment = counter();
increment(); // 1
increment(); // 2
- Callbacks & Event Listeners: Closures are used in asynchronous programming and event handling.
- Function Factories: You can create functions with pre-set values using closures.
function multiplier(factor) {
return function (number) {
return number * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // 10
Want to explore JavaScript in-depth? Do register for GUVI’s JavaScript self-paced course where you will learn concepts such as the working of JavaScript and its many benefits, Variables, Objects, Operators, and Functions, as well as advanced topics like Closures, Hoisting, and Classes to name a few. You will also learn concepts pertaining to the latest update of ES6.
Conclusion
Closures are a fundamental concept in JavaScript that allows functions to remember the scope in which they were created. They help with data encapsulation, function factories, and callbacks, making them an essential tool for writing efficient and maintainable JavaScript code.
FAQs
A closure is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives a function access to its outer scope. In JavaScript, closures are created every time a function is created, at function creation time.
Closures can cause memory frontend development flashcards leaks if they capture variables that are no longer needed. This happens because closures keep references to the variables in their scope, preventing the garbage collector from freeing up memory.
The closure allows the function to access the state in which it was created. Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during the compilation phase, regardless of where they are declared in the code.
Did you enjoy this article?