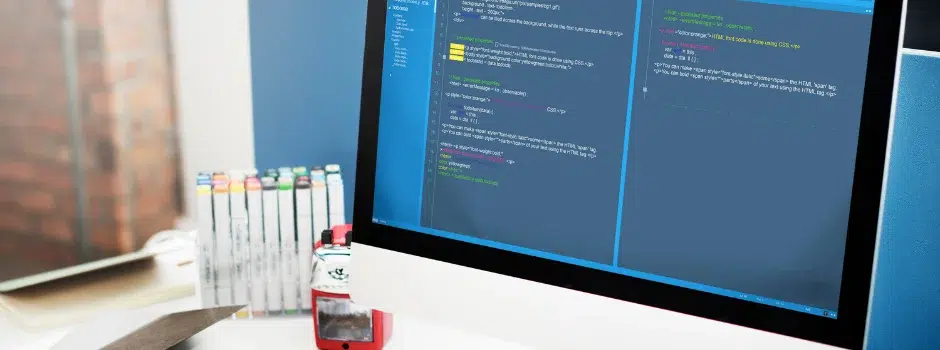
Unearthing Even Gems in a Binary Tree: A Python Adventure
Jan 29, 2025 2 Min Read 621 Views
(Last Updated)
What if your binary tree holds hidden treasures, even gems waiting to be discovered? Imagine finding all the even-numbered nodes, regardless of their position, and storing them in a list for easy access.
This blog takes you on an engaging journey where Python becomes your trusty sidekick to unearth these even gems within a binary tree. Let’s dive into the quest!
Table of contents
- The Quest: Building Our Binary Tree
- Step 1: Define the Node Class
- Step 2: Define the SumanBinaryTree Class
- Recursive Insertion
- Conclusion
- Frequently Asked Questions
- What is a binary tree, and why is it important?
- Why is the largest number set as the root in this example?
- Why should I learn about binary trees?
- How are binary trees different from binary search trees?
The Quest: Building Our Binary Tree
Before we dive into finding the even-numbered nodes, let’s set up our binary tree as specified in the problem statement. The code for the Binary tree is as follows :
"""
Using Python write a python to Create a Binary Tree whose Root Node is 252.
Under Root Node there will be two child nodes 21 and 56.
Now, insert node 49 and 98 inside node 21 and 189 and 1 inside node 56.
Under node 1 insert node 22 and 41 and under node 49 insert node 102 and 103.
Find all the EVEN number nodes and save it inside a list
whether it is present in left side or right side from the binary tree.
"""
class Node:
def __init__(self, val):
self.val = val
self.right = None
self.left = None
class SumanBinaryTree:
def __init__(self):
self.root = None
def insert_node(self, data):
if self.root is None:
self.root = Node(data)
else:
self.__insert__recursive(self.root, data)
def __insert__recursive(self, node, data):
if data < node.val:
if node.left is None:
node.left = Node(data)
else:
self.__insert__recursive(node.left, data)
else:
if node.right is None:
node.right = Node(data)
else:
self.__insert__recursive(node.right, data)
def print_tree(self, node, level=0):
if node is not None:
self.print_tree(node.right, level + 1)
print(' ' * 4 * level + '->', node.val)
self.print_tree(node.left, level + 1)
def find_even_nodes(self, node, even_nodes):
if node is not None:
if node.val % 2 == 0:
even_nodes.append(node.val)
self.find_even_nodes(node.left, even_nodes)
self.find_even_nodes(node.right, even_nodes)
# Create the binary tree
tree = SumanBinaryTree()
tree.insert_node(252)
tree.insert_node(21)
tree.insert_node(56)
tree.insert_node(49)
tree.insert_node(98)
tree.insert_node(189)
tree.insert_node(1)
tree.insert_node(22)
tree.insert_node(41)
tree.insert_node(102)
tree.insert_node(103)
# Find even nodes
even_nodes = []
tree.find_even_nodes(tree.root, even_nodes)
print("Even nodes:", even_nodes)
Step 1: Define the Node Class
The Node class represents a single node in our binary tree.
- __init__ method: The constructor initializes a node with a value (val) and sets the left and right children to None.
Step 2: Define the SumanBinaryTree Class
The SumanBinaryTree class represents the binary tree and contains methods to insert nodes, print the tree, and find even nodes.
- __init__ method: Initializes the root of the tree to None.
- insert_node method: Inserts a new node into the tree. If the tree is empty (root is None), it creates the root node. Otherwise, it calls the private method __insert__recursive to insert the node recursively.
Recursive Insertion
The __insert__recursive method handles the recursive insertion of nodes.
If the data is less than the current node’s value (node.val), it attempts to insert it into the left subtree. If the left child is None, it creates a new node there. Otherwise, it recurses into the left child.
Similarly, if the data is greater than or equal to the current node’s value, it follows the same logic for the right subtree.
Ready to master Python and unlock endless career opportunities in tech? GUVI’s Python Course is designed for learners of all levels, offering a comprehensive curriculum, hands-on projects, and expert mentorship. This course is designed to equip you with the knowledge and tools to excel in fields like AI, Data Science, and Web Development. Take the first step towards transforming your career with Python!
Conclusion
Through this exploration, we’ve built a binary tree, traversed its depths, and uncovered its hidden treasures: the even-numbered nodes. By combining recursive functions and Python‘s versatility, you now have a powerful method to extract these “gems” effortlessly.
Beyond this specific problem, the techniques discussed here can be extended to tackle numerous binary tree challenges, making you a more confident coder. So, keep exploring, keep coding, and let Python guide you in uncovering the secrets hidden within data structures!
Frequently Asked Questions
A binary tree is a hierarchical data structure where each node has at most two children (referred to as left and right). It is widely used for efficient searching, sorting, and hierarchical data representation.
Even gems refer to all the even-numbered nodes present in a binary tree, regardless of their position.
Binary trees are fundamental to many computer science concepts and are widely used in applications like searching, sorting, and hierarchical data storage.
A binary tree allows any structure, while a binary search tree (BST) follows a specific ordering property: left child < root < right child.
Did you enjoy this article?