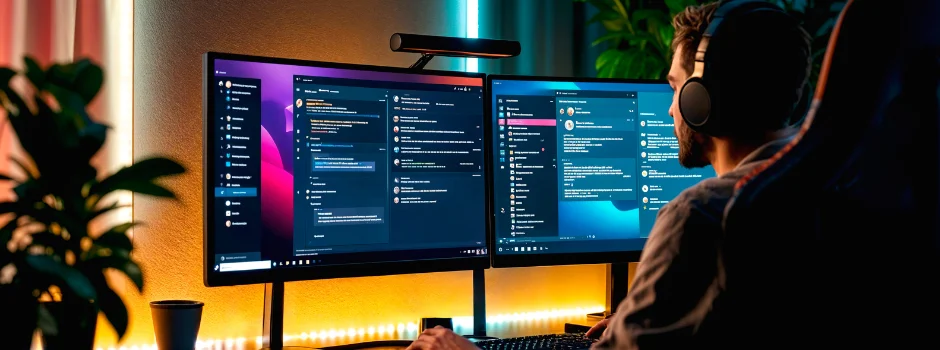
The Ultimate Guide to Python Selenium Fluent Waits
Apr 29, 2025 4 Min Read 355 Views
(Last Updated)
In web automation, Python Selenium is a powerful tool enabling developers to interact with web pages programmatically. However, the dynamic nature of modern web applications presents a significant challenge: elements often load asynchronously, rendering traditional, static waiting strategies unreliable. This is where Selenium’s FluentWait Shines, offering a flexible and robust solution to handle dynamic element loading and enhance the stability of your automated tests.
Table of contents
- The Downfall of Using time.sleep()!
- Fluent Wait: A Symphony of Flexibility:
- The Power of Custom Conditions:
- Imports
- Class Definition: Suman_Fluent_Wait
- How It Works
- Key Features
- Conclusion
The Downfall of Using time.sleep()
!
Before diving into FluentWait
, it is crucial to understand the limitations of simpler waiting methods. time.sleep()
introduces arbitrary delays, making tests slow and inefficient. While implicitly_wait() sets a global timeout, it applies to all element searches, potentially leading to unnecessary delays or premature failures if elements load at varying speeds.
The core issue lies in their static nature. These methods do not account for the dynamic behavior of web applications, whereas elements might appear, disappear, or change state at unpredictable times. Consequently, tests relying on these methods are prone to flakiness, failing intermittently due to timing issues.
Fluent Wait: A Symphony of Flexibility:
FluentWait, which is a part of Selenium’s webdriver. support.wait module addresses these shortcomings by providing a dynamic and customizable waiting mechanism. It allows you to define a condition to be repeatedly checked until it is met, along with a timeout and polling interval.
Key Components of Fluent Wait:
WebDriverWait
: The primary class that orchestrates the waiting process. It takes the WebDriver instance and the maximum timeout as arguments.until()
method: This method accepts a callable (a function or lambda expression) that defines the condition to be checked. This callable should return a truthy value when the condition is met.Polling_frequency
: This parameter specifies the interval (in seconds) between consecutive checks of the condition.Ignored_exceptions
: This parameter allows you to specify exceptions that should be ignored during the waiting process. This is particularly useful for handling situations where elements might not be present initially or might throw temporary exceptions.
Are you interested in starting a career in Python? Kickstart your Python journey using Guvi’s FREE E-book on Python: A Beginner’s Guide to Coding and Beyond. It covers all the necessary concepts you need to know, starting from installing Python on your machine.
The Power of Custom Conditions:
The true strength of FluentWait
lies in its ability to handle custom conditions. You can create complex conditions tailored to your specific application’s behavior. For instance, you might want to wait until an element is visible, clickable, or contains specific text.
Let us look at the code given below and try to understand the power of Fluent Wait.
# main.py
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.firefox.service import Service
from webdriver_manager.firefox import GeckoDriverManager
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import *
class Suman_Fluent_Wait:
# Create a new instance of the Firefox driver
driver = webdriver.Firefox(service=Service(GeckoDriverManager().install()))
# Wait for the username field to be visible for up to 30 seconds, polling every 5 seconds
wait = WebDriverWait(driver, 10, poll_frequency=5, ignored_exceptions=[NoSuchElementException, NoSuchDriverException])
def __init__(self, url, username, password):
self.url = url
self.username = username
self.password = password
# Navigate to OrangeHRM login page
def start(self):
self.driver.maximize_window()
self.driver.get(self.url)
def login(self):
try:
# Find the username field and enter the username
self.wait.until(EC.presence_of_element_located((By.NAME, "username"))).send_keys(self.username)
# Find the password field and enter the password
self.wait.until(EC.presence_of_element_located((By.NAME, "password"))).send_keys(self.password)
# Find the login button and click it
self.wait.until(EC.presence_of_element_located((By.XPATH, "//div[@class='oxd-form-actions orangehrm-login-action']/button"))).click()
except:
print("Error")
finally:
self.driver.quit()
if __name__ == '__main__':
url = "https://opensource-demo.orangehrmlive.com/web/index.php/auth/login"
username = "Admin"
password = "admin123"
suman = Suman_Fluent_Wait(url, username, password)
suman.start()
suman.login()
The code automates logging into the OrangeHRM
demo site (https://opensource-demo.orangehrmlive.com/web/index.php/auth/login) using the Firefox browser. It uses Python Selenium WebDriver with explicit waits to interact with the webpage reliably, entering a username and password, clicking the login button, and then closing the browser.
Imports
The code begins with several imports from the Selenium library and related modules:
- from selenium import webdriver: Provides the WebDriver class to control the browser.
- from selenium.webdriver.common.by import By: Allows specifying how to locate elements (e.g., by name or XPath).
- from selenium.webdriver.firefox.service import Service: Manages the Firefox driver service.
- from webdriver_manager.firefox import GeckoDriverManager: Automatically downloads and sets up the GeckoDriver for Firefox.
- from selenium.webdriver.support.wait import WebDriverWait: Enables explicit waits to pause execution until conditions are met.
- from selenium.webdriver.support import expected_conditions as EC: Provides conditions like “element is present” for waits.
- from selenium.common.exceptions import *: Imports all Selenium exceptions (e.g., NoSuchElementException) for error handling.
These imports set up the tools needed for browser automation.
Class Definition: Suman_Fluent_Wait
The code defines a class Suman_Fluent_Wait
to encapsulate the automation logic.
Class Variables
- driver:
- Initialized as
webdriver.Firefox(service=Service(GeckoDriverManager().install()))
. - Creates a Firefox browser instance using GeckoDriver, which is automatically managed and installed by GeckoDriverManager.
- Initialized as
- wait:
- Initialized as
WebDriverWait(driver, 10, poll_frequency=5, ignored_exceptions=[NoSuchElementException, NoSuchDriverException])
. - A “fluent wait” that:
- Waits up to 10 seconds for a condition to be met.
- Checks every 5 seconds (polling frequency).
- Ignored
NoSuchElementException
(element not found) andNoSuchDriverException
(driver unavailable) During the wait.
- Initialized as
These are defined at the class level, meaning all instances share the same browser and wait object.
Constructor (__init__)
- Parameters: url, username, password.
- Functionality: Stores these parameters as instance variables (self.url, self.username, self.password) for use in other methods.
Method: start
- Purpose: Opens the browser and navigates to the login page.
- Steps:
self.driver.maximize_window()
: Maximizes the browser window.self.driver.get(self.url)
: Loads the provided URL (OrangeHRM login page).
Method: login
- Purpose: Performs the login actions on the webpage.
- Steps:
- Username Field:
self.wait.until(EC.presence_of_element_located((By.NAME, "username"))).send_keys(self.username)
- Waits until the username field (identified by name=”username”) is present, then enters the username.
- Password Field:
self.wait.until(EC.presence_of_element_located((By.NAME, "password"))).send_keys(self.password)
- Waits until the password field (identified by name=”password”) is present, then enters the password.
- Login Button:
self.wait.until(EC.presence_of_element_located((By.XPATH, "//div[@class='oxd-form-actions orangehrm-login-action']/button"))).click()
- Waits until the login button (identified by an XPath) is present, then clicks it.
- Username Field:
- Error Handling:
- Wrapped in a try-except block:
- If any error occurs (e.g., element not found), it prints “Error”.
- Finally block:
self.driver.quit()
: Closes the browser, ensuring resources are released even if an error occurs.
- Wrapped in a try-except block:
Variables:
- url: The OrangeHRM login page URL.
- username: Set to “Admin” (default for the demo site).
- password: Set to “admin123” (default for the demo site).
Execution:
- Creates an instance of Suman_Fluent_Wait with the specified URL, username, and password.
- Calls
start()
to open the browser and navigate to the URL. - Calls
login()
to perform the login actions.
Want to learn more about Selenium in Python? Enroll in Guvi’s wonderful course on Selenium Automation with Python. This course covers everything you need to know about Selenium automation using Python from beginner to advanced level. You can gain hands-on experience with the guided projects along with an industry-recognized certification.
How It Works
- Browser Setup:
- The Firefox browser is launched using
GeckoDriver
, managed byGeckoDriverManager
.
- The Firefox browser is launched using
- Navigation:
- The start method opens the
OrangeHRM
Log in page and maximizes the window.
- The start method opens the
- Login Process:
- The login method uses explicit waits to ensure elements (username field, password field, login button) are loaded before interacting with them.
- It enters “Admin” as the username, “admin123” as the password, and clicks the login button.
- Cleanup:
- The browser closes after the login attempt, whether successful or not, due to the finally block.
Key Features
- Fluent Waits: The use of
WebDriverWait
a 10-second timeout and 5-second polling makes the script robust against delays in page loading. - Error Tolerance: Ignores specific exceptions during waits and includes basic error handling in the login method.
- Modularity: Encapsulates the automation logic in a class, making it reusable with different URLs, usernames, or passwords.
Conclusion
Fluent Wait, implemented via WebDriverWait
In Python, Selenium is an indispensable tool for managing the unpredictability of web applications. Its ability to fine-tune waiting parameters — timeout, polling frequency, and exception handling empowers developers and testers to craft automation scripts that are both robust and efficient. Whether waiting for a button to enable, an element to appear, or a custom state to occur, Fluent Wait ensures interactions happen at the right moment. By incorporating Fluent Wait into your Python Selenium automation testing projects, you can significantly enhance script reliability and performance, thus making it a best practice for tackling dynamic web content. For deeper exploration, the official Selenium documentation offers extensive guidance on waits and advanced automation techniques.
Did you enjoy this article?