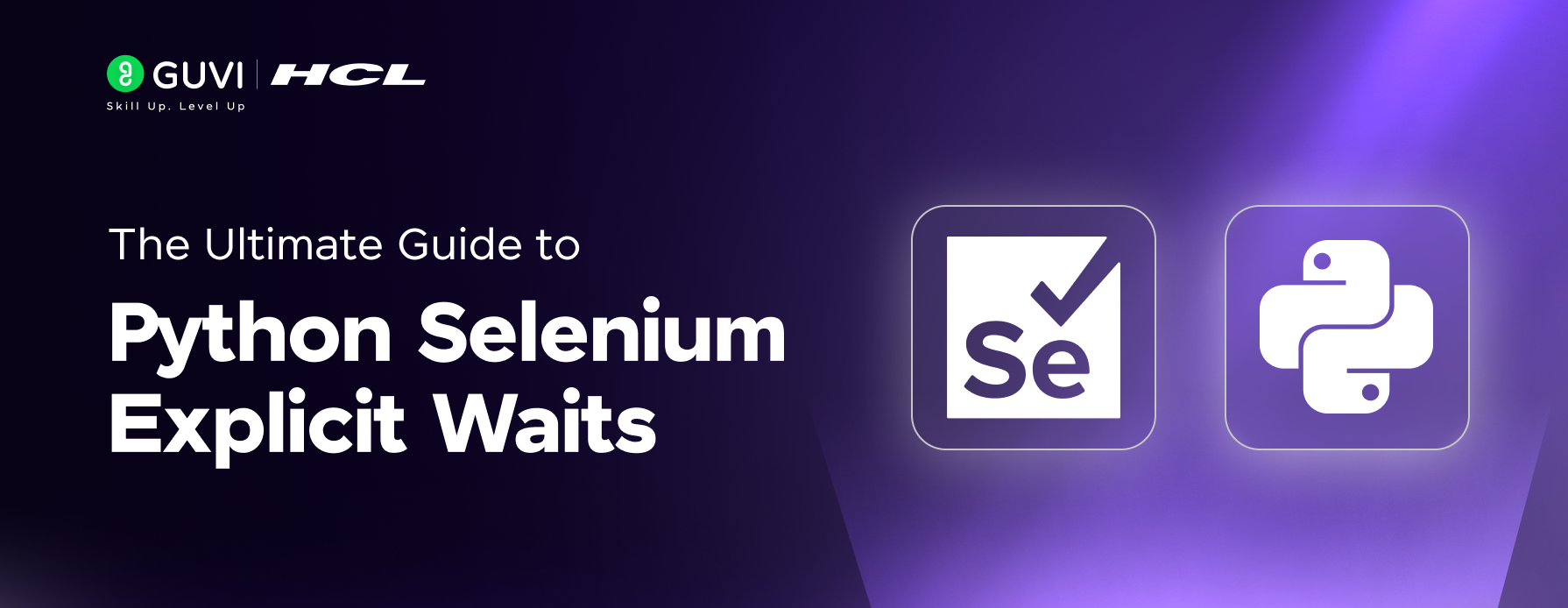
The Ultimate Guide to Python Selenium Explicit Waits
Apr 30, 2025 4 Min Read 411 Views
(Last Updated)
In the realm of web automation with Python Selenium, the ability to interact with dynamic web elements is paramount. While Selenium provides powerful tools for navigating and manipulating web pages, the asynchronous nature of modern web applications often presents a significant challenge; elements may not be immediately available when your script attempts to interact with them. This is where the concept of “waits” comes into play and among them, explicit waits stand out as the most precise and reliable method for ensuring your automation scripts function flawlessly.
Imagine a scenario where your Selenium script clicks a button that triggers an AJAX request. The page updates, and a new element appears, but the time it takes for this element to load is variable. If your script attempts to interact with this element immediately after the click, it will likely fail with a NoSuchElementException or an ElementNotInteractableException. This is because the element has not yet been rendered in the Document Object Model (DOM).
Explicit waits address this issue by instructing Selenium to pause execution until a specific condition is met. This condition is defined using Expected Conditions, a set of predefined functions that check for various states of web elements. By employing explicit waits, you provide Selenium with the necessary patience to handle dynamic content, resulting in more robust and reliable automation scripts.
Table of contents
- Why Explicit Waits are Essential
- Understanding WebDriverWait and Expected Conditions
- Implementing Explicit Wait Using Python Selenium
- Key Components
- Benefits of Explicit Waits
- Conclusion
Why Explicit Waits are Essential
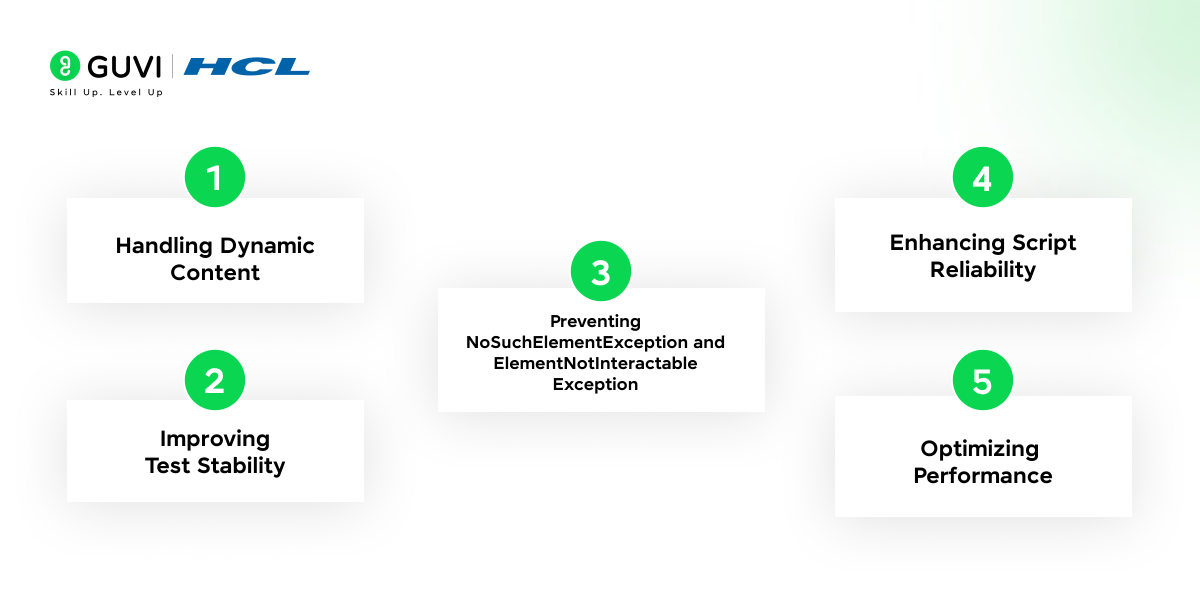
- Handling Dynamic Content : Modern web applications heavily rely on JavaScript and AJAX to dynamically update content. Explicit waits are crucial for handling such dynamic elements, ensuring your script interacts with them only when they are fully loaded and interactable.
- Improving Test Stability: By waiting for specific conditions, explicit waits significantly reduce the likelihood of intermittent test failures caused by timing issues. This leads to more stable and maintainable automation suites.
- Preventing NoSuchElementException and ElementNotInteractableException: These common exceptions arise when Selenium attempts to interact with elements that are not yet present or ready. Explicit waits effectively mitigate these exceptions.
- Enhancing Script Reliability: By introducing controlled delays, explicit waits ensure that your scripts accurately reflect the real-world behavior of users interacting with web applications.
- Optimizing Performance: While implicit waits apply a global delay to all element lookups, explicit waits introduce delays only when necessary, optimizing the overall performance of your automation scripts.
Understanding WebDriverWait and Expected Conditions
The core of explicit waits lies in the WebDriverWait
class and the expected_conditions
module. WebDriverWait
is a class that allows you to specify a maximum waiting time. expected_conditions
provides a set of pre-defined conditions that can be used to check for various states of web elements.
Key Expected Conditions
presence_of_element_located(locator)
Checks if an element is present in the DOM, regardless of its visibility.visibility_of_element_located(locator)
Checks if an element is present in the DOM and visible on the page.element_to_be_clickable(locator)
Checks if an element is present, visible, and enabled.text_to_be_present_in_element(locator, text)
Checks if a specific text is present in an element.presence_of_all_elements_located(locator)
Checks if all the elements matching the locator are present in the DOM.frame_to_be_available_and_switch_to_it(locator)
Checks if a frame is available and switches to it.alert_is_present()
: Checks if an alert is present.invisibility_of_element_located(locator)
Checks if an element is invisible.
Are you interested in starting a career in Python? Kickstart your Python journey using Guvi’s FREE E-book on Python: A Beginner’s Guide to Coding and Beyond. It covers all the necessary concepts you need to know, starting from the installation of Python on your machine.
Implementing Explicit Wait Using Python Selenium
Consider the following code given below and then let us understand the code in detail so that we can able to implement the Explicit Wait successfully using Python Selenium for our automation process:-
# main.py
from selenium import webdriver
from selenium.webdriver.firefox.service import Service
from selenium.webdriver.common.by import By
from webdriver_manager.firefox import GeckoDriverManager
# Python Selenium Wait
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Python Exception
from selenium.common.exceptions import NoSuchElementException
from selenium.common.exceptions import StaleElementReferenceException
class SumanExplicitWait:
driver = webdriver.Firefox(service=Service(GeckoDriverManager().install()))
wait = WebDriverWait(driver, 5)
username_inputBox = 'email' # id
password_inputBox = 'pass' # id
submitButton_locator = 'login' # name
def __init__(self, url):
self.url = url
self.driver.get(self.url)
def presence_of_element_located(self):
try:
username_locator = self.wait.until(EC.presence_of_element_located((By.ID, self.username_inputBox)))
password_locator = self.wait.until(EC.presence_of_element_located((By.ID, self.password_inputBox)))
submit_button = self.wait.until(EC.presence_of_element_located((By.NAME, self.submitButton_locator)))
print('Username Located # ', username_locator)
print('Password box # ', password_locator)
print('Submit Box # ', submit_button)
username_locator.send_keys('suman@guvi.in')
password_locator.send_keys('suman@123')
submit_button.click()
except NoSuchElementException as e:
print(e)
finally:
self.driver.quit()
def title_is(self):
try:
print(self.driver.title == 'Facebook')
except:
print('Web URL Wrong !')
finally:
self.driver.quit()
def url_to_be(self, test_url):
try:
test_url = self.driver.current_url
if (self.driver.current_url == test_url):
return True
except:
return False
finally:
self.driver.quit()
def visibility_of_element_located(self):
try:
username = self.wait.until(EC.visibility_of_element_located((By.ID, self.username_inputBox)))
password = self.wait.until(EC.visibility_of_element_located((By.ID, self.password_inputBox)))
submit = self.wait.until(EC.visibility_of_element_located((By.NAME, self.submitButton_locator)))
if(username and password and submit):
username.send_keys('suman@guvi.in')
password.send_keys('suman@123')
submit.click()
except StaleElementReferenceException:
print("Visibility of Element # ")
finally:
self.driver.close()
if __name__ == "__main__":
url = 'https://www.facebook.com'
explicit_wait = SumanExplicitWait(url)
explicit_wait.presence_of_element_located()
explicit_wait.title_is()
print(explicit_wait.url_to_be('suman'))
explicit_wait.visibility_of_element_located()
Key Components
- Importing Libraries:
- selenium: The core Selenium library for web automation.
- webdriver: Provides the browser driver interface.
- Service and GeckoDriverManager : Manages the Firefox Gecko driver.
- By: Used to locate web elements by various attributes (ID, name, etc.).
- WebDriverWait: Implements explicit waits.
- expected_conditions (EC): Defines conditions to wait for (e.g., element presence, visibility).
- NoSuchElementException and StaleElementReferenceException: Exception handling for element-related errors.
- SumanExplicitWait Class:
- Initializes the Firefox driver and WebDriverWait with a timeout of 5 seconds.
- Defines locators for username, password, and submit button.
__init__(self, url)
: Opens the specified URL in the browser.
presence_of_element_located(self)
Method:
- Uses EC.presence_of_element_located() to wait until the specified elements are present in the DOM.
- Locates username, password, and submit button elements.
- Sends keys to the input fields and clicks the submit button.
- Handles NoSuchElementException in case an element is not found.
- Quits the driver in the finally block.
title_is(self)
Method:
- Check if the page title is “Facebook”.
- Handles potential exceptions and quits the driver.
url_to_be(self, test_url)
Method:
- Verifies if the current URL matches the provided test_url.
- Returns True if the URLs match, False otherwise.
- Quits the driver in the finally block.
visibility_of_element_located(self)
Method:
- Uses EC.visibility_of_element_located() to wait until the specified elements are visible on the page.
- Locates and interacts with the username, password, and submit button elements.
- Handles StaleElementReferenceException if an element becomes stale.
- Closes the driver in the finally block.
if __name__ == "__main__":
Block:
- Sets the target URL.
- Creates an instance of SumanExplicitWait.
- Calls the methods to demonstrate explicit waits.
Want to learn more about Selenium in python? Enroll in Guvi’s wonderful course on Selenium Automation with Python. This course covers everything you need to know about selenium automation using python from beginner to advanced level. You can gain hands on experience with the guided projects along with industry recognized certification.
Benefits of Explicit Waits
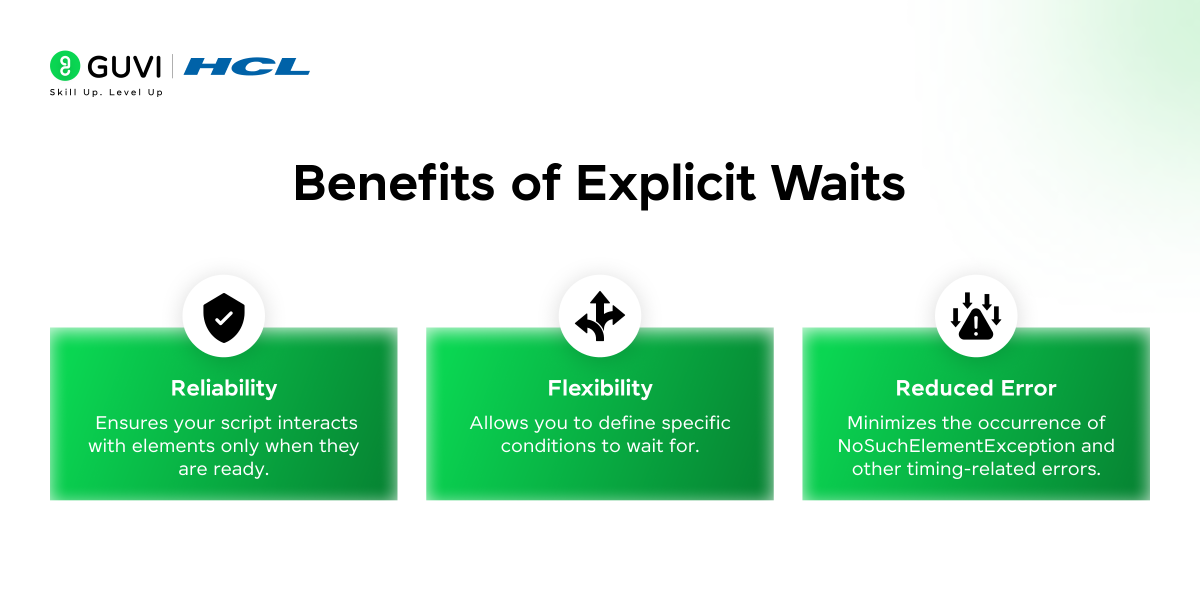
- Reliability: Ensures your script interacts with elements only when they are ready.
- Flexibility: Allows you to define specific conditions to wait for.
- Reduced Errors: Minimizes the occurrence of NoSuchElementException and other timing-related errors.
Conclusion
Explicit waits are essential for robust and reliable Selenium automation. By using WebDriverWait
and expected_conditions
You can create scripts that handle dynamic web pages effectively. The provided code demonstrates how to implement different types of explicit waits, empowering you to build more stable and maintainable automation workflows.
Did you enjoy this article?