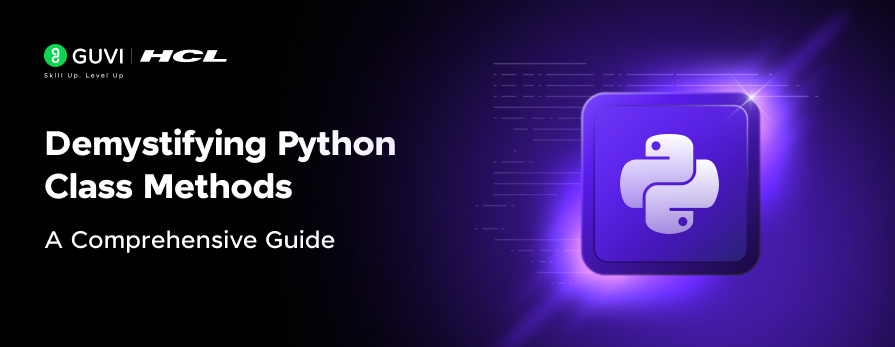
Demystifying Python Class Methods – A Comprehensive Guide
May 09, 2025 3 Min Read 675 Views
(Last Updated)
In the world of Python programming, one of the most fascinating and pivotal concepts is Object-Oriented Programming (OOP). Among the tools offered by OOP class methods stand out as a cornerstone for crafting reusable, readable, and efficient code.
Despite their importance, class methods are often perceived as complex or misunderstood, especially by beginners or those transitioning from procedural programming.
This article aims to demystify the concept of class methods in Python, offering a comprehensive understanding of their utility, functionality, and elegance. So, without further ado, let us get started!
Table of contents
- What are Class Methods?
- Why Use Class Methods?
- Conclusion
What are Class Methods?
At their core, Python class methods are special methods that belong to a class rather than any individual instance of the class. They are defined using the @classmethod decorator and take a unique parameter, typically named cls, that represents the class itself.
Unlike instance methods, which operate on a specific object of the class, class methods operate on the class as a whole, opening doors to a wide range of programming possibilities.
The utility of class methods goes far beyond their definition. They enable us to create alternative constructors, manage class-level data, and implement design patterns such as the Singleton or Factory pattern with remarkable ease.
In scenarios where you need to manipulate or access class-level attributes rather than instance-level attributes, class methods become indispensable tools. They provide an abstraction that encourages code reusability and aligns with the DRY (Don’t Repeat Yourself) principles, a hallmark of clean and maintainable code.
This allows them to access and modify class-level attributes and behaviors. Here’s a simple syntax example:
class MyClass:
class_variable = "Hello, class!"
@classmethod
def class_method(cls):
return f"Class variable value: {cls.class_variable}"
Key Features of Class Methods
- They work on the class rather than instances.
- They are often used as alternative constructors.
- They allow manipulation of class-level attributes.
- They promote code reusability by operating on the class itself.
Why Use Class Methods?
Class methods are not just a syntactic curiosity; they also serve practical purposes that make Python programming more powerful and flexible. Here are some key reasons to use them:
- Alternative Constructors:- Class methods enable developers to create alternative ways to construct objects. This is especially useful when objects need to be initialized from different sources, such as configuration files, databases, or serialized data. Take, for example:-
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
@classmethod
def from_string(cls, book_string):
title, author = book_string.split(" - ")
return cls(title, author)
# main execution function
if __name__ == "__main__":
book = Book.from_string("1984 - George Orwell")
print(book.title) # Outputs: 1984
- Managing Class-Level Data:- When your application requires centralized management of class-level attributes, class methods can act as a bridge between class attributes and external functionality. Take, for example:-
class Counter:
count = 0
@classmethod
def increment(cls):
cls.count += 1
@classmethod
def get_count(cls):
return cls.count
# main execution function
if __name__ == "__main__":
Counter.increment()
print(Counter.get_count())
Difference Between Class Methods and Static Methods
Class methods often get compared to static methods, as both methods belong to the class rather than its instances. Here’s a comparison:
Feature | Class Method | Static Method |
Access to Class | Yes (cls parameter) | No |
Access to Instance | No | No |
Use Case | Class-level functionality | Utility or helper methods |
Static methods are defined using the @staticmethod decorator and do not take any implicit arguments (self or cls). They are best used for tasks that are logically related to the class but do not require access to class-level attributes.
Best Practices for Class Methods
For using the Class Methods efficiently and effectively, kindly adhere to the following rules which are given below:-
- Use clear and concise names
- Restrict class-level modifications
- Document usage
- Complement instance methods
Conclusion
Class methods in Python are a versatile and powerful feature of Object-Oriented Programming. They allow developers to craft more readable, reusable, and efficient code by enabling class-level functionality. From alternative constructors to centralized management of class data, class methods provide tools that simplify programming tasks while encouraging clean coding practices.
Whether you’re building complex systems or working on smaller-scale projects, incorporating class methods into your coding repertoire can elevate your Python programming skills. By understanding their syntax, use cases, and differences from other methods, you will unlock the full potential of this invaluable feature.
So, go ahead, try them out, experiment, and see how they can transform your approach to programming in Python. The journey to mastering class methods begins with understanding, and this guide is here to help.
Did you enjoy this article?