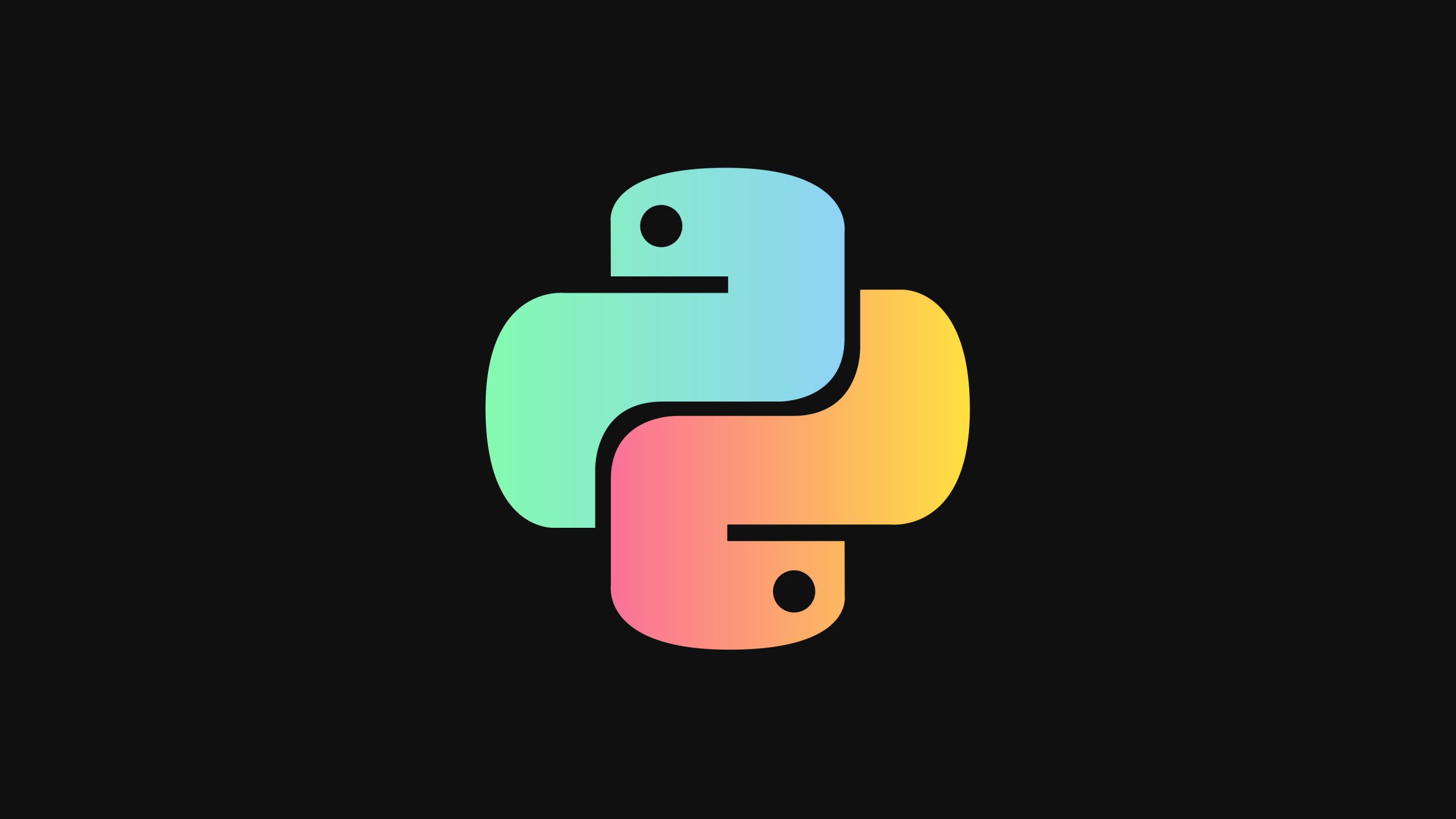
Top 10 Python Terms Every Beginner Should Know
Mar 21, 2024 6 Min Read 1105 Views
(Last Updated)
By the end of 1989, Python’s inventor Guido Van Rossum was investing his time in one of his side projects to keep him occupied during the Christmas break. His goal was to create a new scripting language targeting Unix/C hackers that’d be descendants of ABC.
He named it: Python. Unlike the name suggests, the language’s name isn’t about constricting snake, but a British comedy troupe Monty Python. Little does he know, that it will become one of the most popular programming languages that will drive the biggest tech giants and their products/services: Netflix, Facebook, NASA, and Google to name a few.
Recently Python was ranked #1 on the IEEE spectrum list for “Top Programming Languages 2019.” In this blog, we will try to deconstruct Python learning and will aim to outline all the Top Python Terms that every beginner should know. The idea is to push information in a relevant, short, and focused manner to cover all the vital topics.
Table of contents
- So What is Python and What makes it so versatile?
- Installing Python and PyCharm
- Writing your first “Hello World” - First step towards Python Learning!
- List of Top 10 Python Terms
- Variables
- How to declare and assign value To Variable
- Numeric
- Strings in Python Learning
- List: Collection
- Array
- Conditional Statements
- Loops
- Dictionary
- Lambada Function
- Data Types
- Library
- Object
- Refer free “PYTHON with IIT CERTIFICATION” Course for all Python terminologies and Concepts
- Do you wanna read more constructive blogs on Python?
So What is Python and What makes it so versatile?
According to Guido Van Rossum Python is a high-level, interpreted, dynamically-typed scripting language, and its core design philosophy is all about code readability and syntax that allows coders to express the concepts within a few lines of the codes.
As a result, it takes less time to launch a Python program to market compared to its peers such as Java and C#. Nearly all sorts of applications ranging from UI to analytical tools can be implemented in Python. Another additional key difference is that one does not need to declare any sort of variable type. So it’s easier to implement a Python application.
Although it does not offer advanced statistical features such as R and it’s not suitable for hardware interaction and low-level systems. It is also described as a “batteries included” language for its adaptive standard library. Besides its practical standpoint, there are several books and communities available to support Python Developers.
The way the Python environment runs on machines:
- An associated Python virtual machine is created where the complete package library is installed.
- Normally, the Python code is written in .py file format.
- CPython, the original Python implementation then complies with the code-to-byte code for the Python Virtual Machine.
Also, if you would like to learn Python through a Self-paced course, try GUVI’s Python Self-Paced course with IIT-M certification.
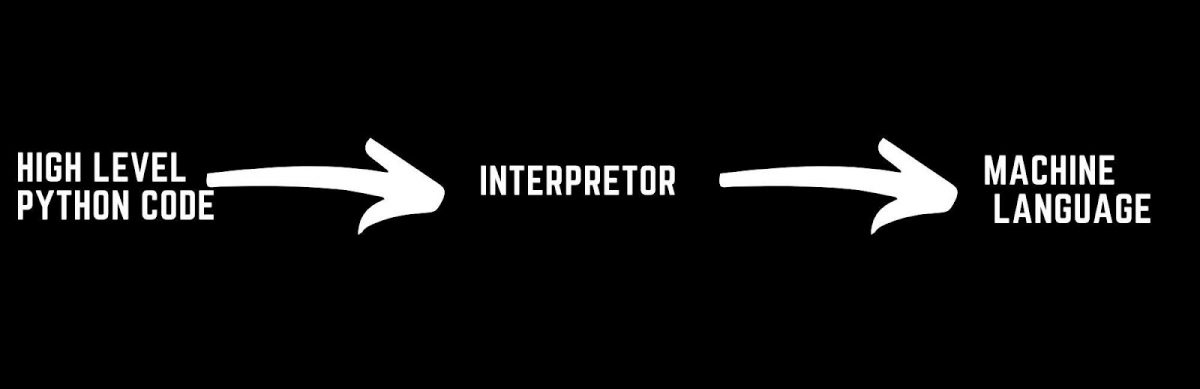
Installing Python and PyCharm
Python is available across all major operating systems: Linux/Unix, Windows, Mac OS X, and others. In Case you are new to it, We will walk you through a step-by-step process on how to install Python on your system. If you aren’t, you can simply scroll to the next section. Although you will now be able to configure the python environment with a commands line interface, It’s more extensive to use the language with an Integrated Development Environment. My recommendation: PyCharm.
- First, go to Python’s official website and download the latest Python release.
- Once done, run the .exe file to install python. Make sure to check “Add Python 3.9 to PATH”.
- To see if the installation is successful, go to your command prompt, type “Python version” and click enter. It will display the python version installed over your system.
- Visit Jetbrains and click the download link below the Community Section for a free version of the IDE.
- Run the setup wizard and install PyCharm. Pick your installation path and create a desktop shortcut for your specified launcher.
- Once done, run the PyCharm Community Edition, and you are good to go.
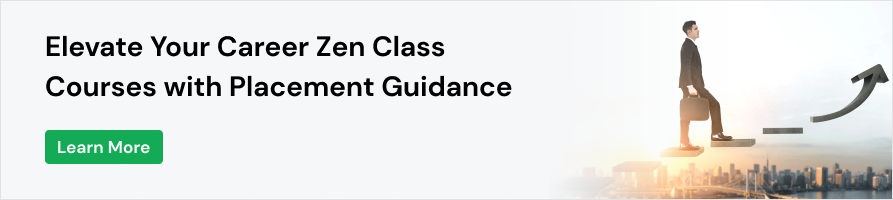
Writing your first “Hello World” – First step towards Python Learning!
To create your first program, open your Pycharm editor and create a new project. Select the destination path where you want to save your first program and name the project from “untitled” to something meaningful such as “First project-Hello World.”
Next, you have to visit the file menu and create a new > Python file. You can type the name of your file as “Hello World” and hit “ok”. Type a single program-
print (“Hello World”)
and run the program with the run menu. You can witness the output of your written program at the bottom of the screen.
In case you don’t have PyCharm installed on your system, you can still run the code from the command prompt. You just need to enter the correct path for the Python file you want to run. The output of the code will be visible below the code. Now let’s summarize the top Python terms that are frequently asked in an Interview.
List of Top 10 Python Terms
1. Variables
Variables store information that can be either used and/or changed in your program. This information can be a text, collection integer, etc. They are used to hold user inputs, local states of your program, etc. Variables have a name so that they can be referenced in the code. The fundamental concept to understand is that everything is an object in Python Learning.
Python supports numbers, sets, lists, strings, tuples, and dictionaries. These are the standard data types. I will explain each of them in detail.
#string
my_university = “Christ University”
#booleans
true_boolean = True
false_boolean = False
#float
University fee = 1700
2. How to declare and assign value To Variable
Assignment sets a value to a variable. To assign a variable a value, We can use the equals sign (=)
FirstVariable = 1
SecondVariable = 2
FirstVariable = "Hello You"
To assign a variable a value, use the equals sign (=)
If you want to assign the same value to more than one variable then you can use the chained assignment:
FirstVariable = SecondVariable = 1
3. Numeric
Integers, float, and decimals are supported.
value = 1 #integer
value = 1.2 #float with a floating point
4. Strings in Python Learning
A string is an array of characters. A string value is enclosed in quotation marks: single, double, or triple quotes. Also, they are immutable, which means the ones assigned can’t be changed and updating them will fail and show a syntax error.
name = 'Shubham'
name = "Shubham"
name = """Shubham"""
5. List: Collection
A list is a collection that can be used to store a list of values (like these integers that you want). So let’s use it:
my_integers = [1, 2, 3, 4, 5]
It is straightforward. We created an array and stored it on my_integer. But maybe you are asking: “How can one get a value from this array?” The list has a concept called index. The first element gets the index 0 (zero). The second gets 1, and so on. You get the idea.
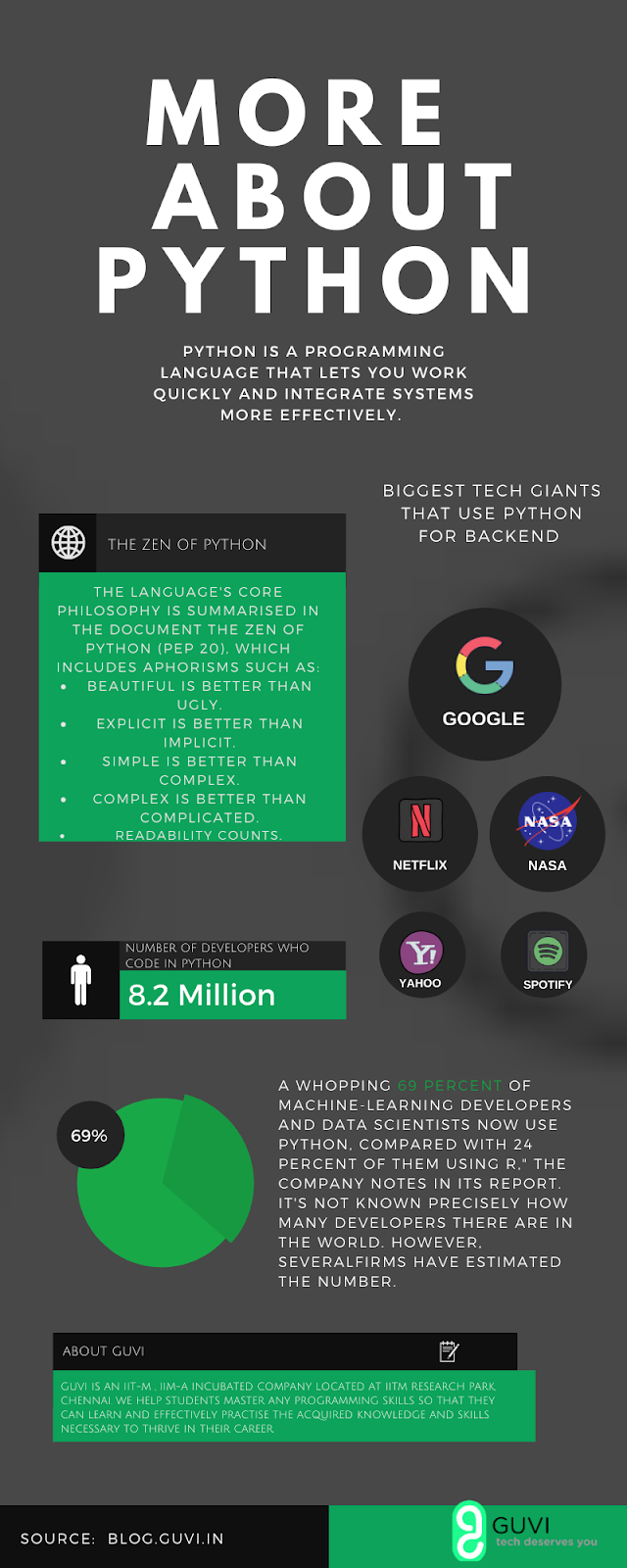
6. Array
To make it clearer, we can represent the array and each element with its index. Illustrated below:
It’s easier to comprehend with a Python Syntax:
my_integers = [5, 7, 1, 3, 4]
print(my_integers[0]) # 5
print(my_integers[1]) # 7
print(my_integers[4]) # 4
But suppose that you don’t want to store integers. You just want to store strings, like a list of your relatives’ names. Which will look something like this:
relatives_names = [
"Mohan",
"Rajesh",
"Yogesh",
"Aishwarya",
"Kartik"
]
print(relatives_names[4]) # Kartik
It works the same way as integers. We just learned how Lists indices work. But you should also know how we can add an element to the List data structure (an item to a list). The most common method to add a new value to a List is appended. Let’s see how it works:
bookshelf = []
bookshelf.append("The Worker")
bookshelf.append("The 8 Hour Work Week")
print(bookshelf[0]) # The Worker
print(bookshelf[1]) # The 8 Hour Work Week
It works the same way as integers. We just learned how Lists indices work. But you should also know how we can add an element to the List data structure (an item to a list). The most common method to add a new value to a List is appended. Let’s see how it works:
The append is super simple. You just need to apply the element (eg. “The Worker”) as the append parameter.
7. Conditional Statements
The word “if” is used to evaluate whether the given statement is true or false, If it’s true, it executes the code written inside the “if” statement. For example:
If true:
print(“Hello If”)
If 2>1:
Print (“2 is greater than 1”)
In case 2 is greater than 1: the “print” code is executed.
Otherwise, the “else” statement is executed, if the “if” statement is false.
if 1 > 2:
print("1 is greater than 2")
else:
print("1 is not greater than 2")
In this case, if 1 is not greater than 2, the code inside the “else” statement will be executed.
In Python, you can also introduce the “elif” statement.
if 1 > 2:
print("1 is greater than 2")
elif 2 > 1:
print("1 is not greater than 2")
else:
print("1 is equal to 2")
8. Loops
In Python Learning, we can iterate in various forms such as “While” “for”, “do while”, and “for while”. Further, we will talk about just two: For and While. Refer to GUVI’s professionally curated Python Course for the rest.
For Looping: To apply “For Loop”; you apply the variable “Num” to the block: and the “for” statement will iterate automatically for you.
for i in range(1, 11):
print(i)
The range initiates with the 1 and goes until the 11th element (10th =10th element)
While loop: Just as the name suggests, While the statement is true; The code inside the code gets executed. Hence, this code will print the number from 1 to 10.
num = 1
while num <= 10:
print(num)
num += 1
For such a statement, it requires a “loop condition” if it stays true, it will continue itself iterating. In the above example, when the num is 11, the loop condition will falsify.
9. Dictionary
Now we are aware that “Lists” are indexed with integer numbers. But what if we don’t want to use integer numbers as indices? Various data structures that we can make use of our string, numbers, or other types of indices.
Let’s learn about the dictionary data structure. Dictionary is a series of key-value pairs. Here’s what it looks like:
dictionary_example = {
"key1": "value1",
"key2": "value2",
"key3": "value3"
}
The key factor here is to index that points over to a value. You can access the “Dictionary value” using the “Key”. As illustrated below:
dictionary_tk = {
"name": "Shubham",
"nickname": "Shiv",
"nationality": "Indian"
“University”: “Christ University
}
print("My name is %s" %(dictionary_tk["name"])) # My name is Shubham
print("But you can call me %s" %(dictionary_tk["nickname"])) # But you can call me Shiv
print("And by the way I'm %s" %(dictionary_tk["nationality"])) # And by the way I'm Indian
print(“I am pursuing my graduation in %s" %(dictionary_tk["University"])) #I am pursuing my graduation in Christ University.
So as you can see, I’ve created a dictionary about a random person. It includes his name, nickname, his nationality, and university. These attributes are known as dictionary keys.
10. Lambada Function
Lambada functions are incredibly useful. They are also known as engines in serverless engineering. If your firm’s building an app that has the necessity to build server-side code deployment, Lambada allows you to achieve the same results without being burdened by hosting, maintenance, and unnecessary codes which are required when building server code in a standard way. But how is this possible?
Python Lambada functions are anonymous functions implying that the function exists without a name. While normal functions are defined as “def” keywords, anonymous functions are defined by the ‘lambada’ keyword. Unlike the ‘def’ function, they are generally used for a short period of time. and one can define and call it immediately at the end of the definition.
The syntax for lambda
functions are given by: lambda arguments: expression Notice, that there can be any number of arguments but can contain only a single expression.
11. Data Types
Every value in python has a data type. Since everything is an object in Python Programming, data types are actually variables and classes, which are instances (objects) of those classes. Python has several data types.
- Float (decimal values)
- Int (numbers without decimals)
- Boolean (True or False)
- String (Combination of 1 or more characters)
- None (After declaring it, You don’t want to give value to the variable you can use None)
- List (Array-like structure but not used to store any datatypes)
- Tuples (Same as lists but we can’t do any changes once it’s created)
- Dict (Key Value Pair)
- Set (Unique set of Pair)
12. Library
Python offers a set of extensive useful libraries that eliminate the need for writing codes from scratch. Perhaps, Python Libraries are one of the reasons, Python is still pretty relevant today. In total there are approximately 137,000 Python libraries present to date, which plays a vital role in developing Machine Learning, Data Science, image and data visualization, data science and data manipulation and the list go on.
In a technical context, a python library is a collection of Pre-combined codes which can be used iteratively to reduce the time to code. They are specifically used for accessing the pre-written codes, which are used frequently. So that instead of writing it down, from the scratch, we can call certain functions which can pave the way. Similar to physical libraries, these are a collection of reusable resources, which means every library has a root source. This is the foundation behind the numerous open-source libraries available in Python.
Top Python Libraries include Scikit-Learn, RAMP, NuPIC, NumPY, TensorFlow, Pipenv, PySpark, PyTorch, MatPlotLib, Dash, SciPy, Seaborn, Keras, Bokeh, and more.
12. Object
An Object is an instance of a Class. A class is like a blueprint while an instance is a copy of the class with actual values. Python is an object-oriented programming language that stresses objects i.e. it mainly emphasizes functions. Objects are basically an encapsulation of data variables and methods acting on that data into a single entity.
Refer free “PYTHON with IIT CERTIFICATION” Course for all Python terminologies and Concepts
Whether you have never programmed before, already know basic syntax, or want to learn about the advanced features of Python Learning, this course is for you! This course will offer you that core, solid understanding of the Python programming language. You will not only be learning Python, but you will be learning industry best practices for Python programming that real employers demand. What’s more- The course is absolutely free, just register to GUVI & start binging our tutorial videos. And we bet, within a couple of hours, you’d be writing your Python code.
Boost your recruitment process with an IIT-Madras certification in Python.
✔️ Master the fundamentals of Python and develop programs to gather, clean, analyze, and visualize data. No prior coding experience is required!
✔️ +70 Hrs of Self-paced, online learning modules in a graded format, designed by top industry experts.
✔️ Lifetime access to recorded sessions and our gamified practice platform-Codekata.
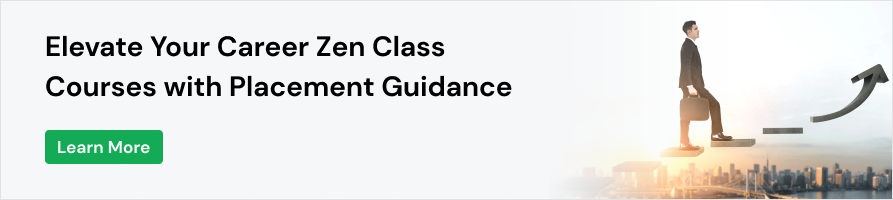
Did you enjoy this article?