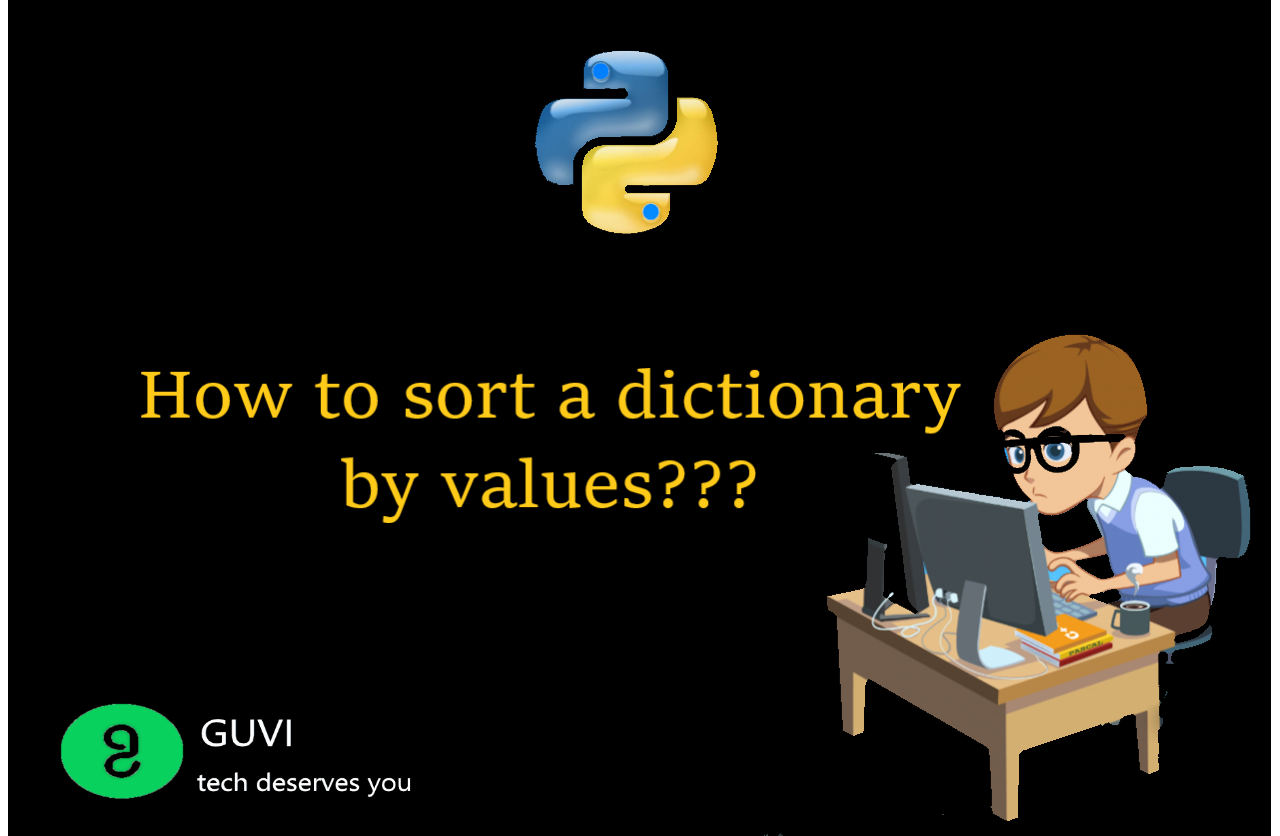
How To Sort A Dictionary By Values In Python?
Mar 26, 2024 3 Min Read 690 Views
(Last Updated)
Sort a dictionary by values! How can we sort elements inside dictionaries using their values in Python? That is a good question while considering Dictionary in Python.
This article will discuss the various ways to sort elements kept inside dictionaries by values. However, before we proceed further, let’s discuss what a dictionary in Python is and what it means to sort a dictionary by value.
Table of contents
- Dictionary in Python
- Creating a dictionary
- Before finding out how to sort a dictionary by values, let's have a look at a few additional functions :
- items() method of a dictionary
- Let’s have a look at an example of using the sorted() function
- Test your understanding of different Python concepts
Dictionary in Python
Dictionary in Python is an important data structure. By using Dictionary, we can store data in the form of key-value pairs. Each key in a dictionary can store at most one value. While declaring a key it must be noted that the keys in the dictionary must be immutable in nature and the supported data types of declaring a key are: integers, strings, and tuples as these are immutable. Also, the dictionary has a unique property that doesn’t allow duplication of keys inside the dictionary.
Note: The older versions of Python beyond 3.7, had dictionaries with unordered data structures. But since Python 3.7 came into existence, dictionaries are ordered data structures. This implies that the elements inside the dictionaries are now stored in an ordered manner.
Before diving into the next section, ensure you’re solid on Python essentials from basics to advanced-level. If you are looking for a detailed Python career program, you can join GUVI’s Python Career Program with placement assistance. You will be able to master the Multiple Exceptions, classes, OOPS concepts, dictionary, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-paced course, try GUVI’s Python Self-Paced course.
Creating a dictionary
You can create a dictionary in Python by enclosing key-value pairs inside curly braces ({}). Each key-value pair is separated with another key-value pair with the help of commas (,).
Syntax:
{key1 : value1, key2 : value2, key3 : value3, key4 : value4,…..}
Let’s have a look at the below-given code snippet which describes the creation of a dictionary in Python.
So, do not use mutable objects as keys inside the dictionary. Instead, we can convert a list into a tuple (immutable type) and then make it a key.
Before finding out how to sort a dictionary by values, let’s have a look at a few additional functions :
items() method of a dictionary
The items() method of a dictionary is used to return a view object that displays a list of tuples containing the key-value pair of the corresponding tuple. Let’s implement a code to understand the working of this method.
Output:
The functions that are declared without a name are called anonymous functions. These anonymous functions are defined using a lambda keyword. That’s the reason why the anonymous functions are also called lambda functions.
Syntax of lambda functions is given as:
lambda arguments: expression
A lambda function can have any number of arguments. However, it has only one expression. It is evaluated and returned after the function operation. Let’s see a sample program to observe the working of the lambda functions.
Output
The sorted function is used to sort an iterable object in a particular order. And then it returns the result in form of a list.
Syntax
sorted(iterable, key, reverse)
The function accepts three parameters that are discussed in detail below:
- iterable: It is an object that can be iterated. Such as a sequence type (like list, tuple, string) or a collection type (like a dictionary, set, frozen set, etc.) or it could be any other iterable object.
- key: It is an optional parameter inside the function. It accepts a function to be passed inside it This becomes the basis over which element in the iterable is compared for sorting. The default value is set to None.
- reverse: It is also an optional parameter and accepts Boolean values in True and False. If the value passed is True, then the sorted list will be displayed in reverse order. Otherwise, the default value is set to False, which displays the result in ascending order.
Let’s have a look at an example of using the sorted() function
The output of sorted() to sort a dictionary by values:
Now that we have an understanding of what keys and values are inside a dictionary. Also, few additional functions such as items(), lambda functions, and sorted() function. Finally, we shall put our focus towards our main goal i.e., sorting the dictionary by its values.
To understand it in a better way, let’s formulate it in a problem statement. Recall in the earlier section, we created a dictionary representing the Test cricket rankings of different countries where the team name was the key and its ranking was the value that key holds. Our objective is to sort the team names based upon rankings (i.e., value) in ascending order.
It should be noted that there aren’t any straightforward built-in functions that we can use for sorting the dictionary by values. Therefore, to achieve our objective we will make use of those additional Python functions that we studied earlier in the tutorial.
Thus, to sort a dictionary by values, we can verify our result by printing the ‘sorted_ranking’ variable our original dictionary will get sorted by the values. While saving and executing the above code, the output will look like this:
Test your understanding of different Python concepts
Question 1. Choose the correct output of the following code.
state = [‘New Delhi’, ‘Assam’, ‘Goa’, ‘Jammu and Kashmir’, ‘Uttar Pradesh’, ‘Rajasthan’, ‘Gujarat’ ]
print(sorted(state[ : : 2]))
- [ ‘New Delhi’, ‘Goa’, ‘Uttar Pradesh’, ‘Gujarat’ ]
- [ ‘Goa’, ‘Gujarat’, ‘New Delhi’, ‘Uttar Pradesh’ ]
- [ ‘Assam’, ‘Goa’, ‘New Delhi ’, ‘Uttar Pradesh’]
- [‘Assam’, ‘Gujarat’, ‘Jammu and Kashmir’, ‘Uttar Pradesh’ ]
Correct Answer: (ii)
[ ‘Goa’, ‘Gujarat’, ‘New Delhi’, ‘Uttar Pradesh’ ]
The question is a combination of two concepts list slicing and then using the sorted() function. Firstly, list slicing will return a sublist in the form of [‘New Delhi’, ‘Goa’, ‘Uttar Pradesh’, ‘Gujarat’] and later it will be sorted to get the correct desired output.
Question 2. For which of the following function it is not mandatory to have a name?
- Anonymous function
- None of these
- Lambda function
- Both (i) and (iii)
Correct Answer: (iv) Both (i) and (iii)
Anonymous functions need not have a name. Therefore, we declare these, using the lambda keyword. Hence they are also known as the lambda function.
Question 3. Which of the following is immutable in nature?
- List
- String
- Tuple
- Both (ii) and (iii)
Correct Answer: (iv) Both (ii) and (iii)
Both tuple and string are of an immutable type. So, once we declare them, mutating them is not possible.
Question 4. To convert a list into a dictionary we can make use of which method?
- tuple()
- dict()
- listtodict()
- None of these
Correct Answer: (ii) dict()
We can make use of the dict() constructor method to convert a list into a dictionary.
Question 5. Which of the following data structure in Python hold data in the form of key-value pairs in Python?
- String
- List
- Dictionary
- Tuple
Correct Answer: (iii) Dictionary
Kickstart your Programming journey by enrolling in GUVI’s Python Career Program where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self Paced course.
Did you enjoy this article?