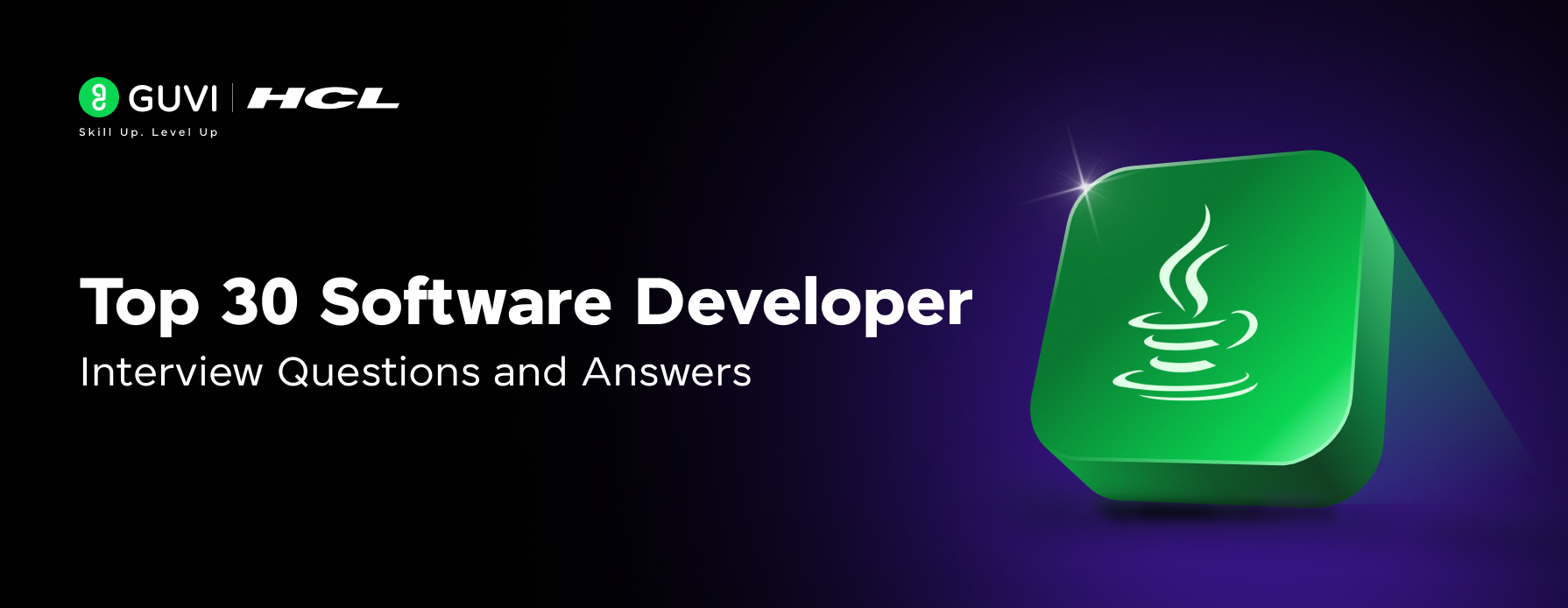
Top 30 Software Developer Interview Questions and Answers
Jul 08, 2025 6 Min Read 4610 Views
(Last Updated)
The world of software development is highly competitive, and preparing for a software developer interview can be a daunting task, especially with the vast array of topics and questions that might come your way.
To help you navigate this process, we’ve compiled a comprehensive list of 30 software developer interview questions and answers, categorized by experience level: Fresher, Intermediate, and Advanced.
Table of contents
- Who is a Software Developer?
- Top 30 Software Developer Interview Questions and Answers
- Freshers
- Intermediates
- Experienced
- Conclusion
- FAQs
- Q1. What are the typical rounds in a software development interview?
- Q2. What topics should I prepare for the technical round?
- Q3. Do I need to know the system design as a fresher?
- Q4. How important is communication during the interview?
Who is a Software Developer?
A software developer is someone who designs, builds digital solutions, such as software and applications that we use on our websites, desktops, and mobile devices. They are problem solvers who figure out how to make things easier, faster, or more useful through technology. They are the people who bring digital ideas to life.
Software developers take responsibility for the product throughout its life cycle from designing, developing, testing, deploying and maintaining. These tasks can be easy and manageable using various software tools.
Now that we have seen who is a software developer is, let’s jump into the top 30 interview questions and answers to become one.
Top 30 Software Developer Interview Questions and Answers
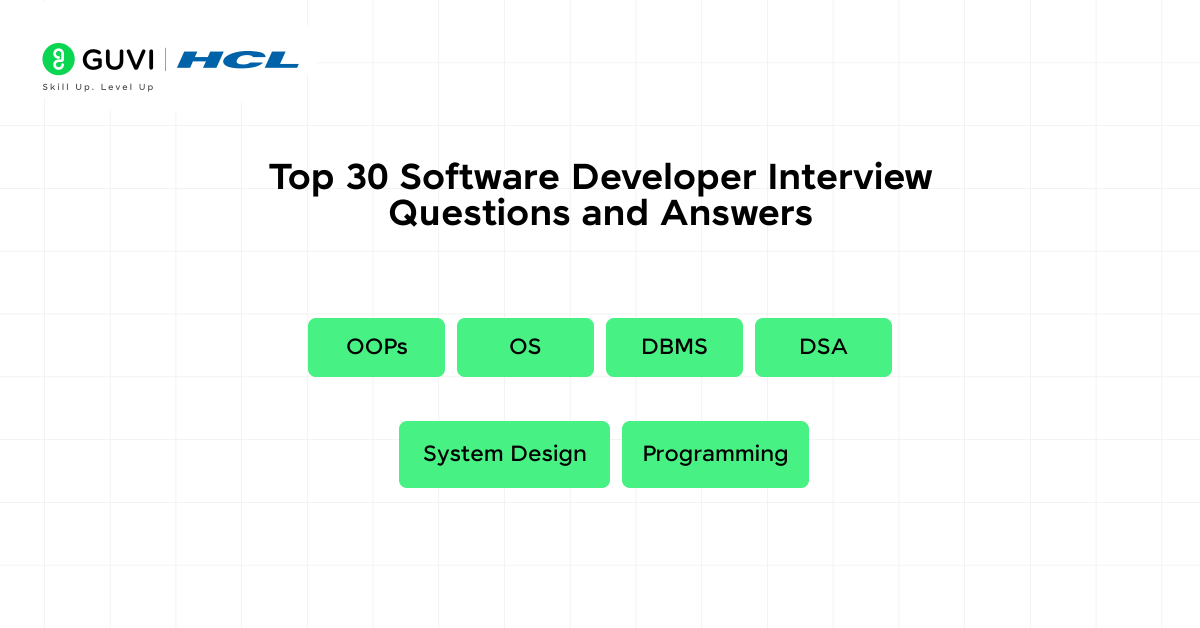
This section covers interview questions for various experience levels, ranging from freshers to experienced. Before jumping into questions, remember that Data Structures and Algorithms (DSA) is a mandatory skill for a software developer interview. Let’s get started!
Freshers
In this section, we will look into some of the software developer interview questions and answers for freshers. This covers concepts such as the basis of Object Oriented Programming (OOP), Linear Data Structures and Algorithms (DSA).
- What is a data structure, and why is it important?
Data structures organize data efficiently, making it easier to access, modify, and store. Common data structures include arrays, lists, stacks, queues, and trees, each designed for specific use cases.
- What is Object Oriented Programming (OOP)?
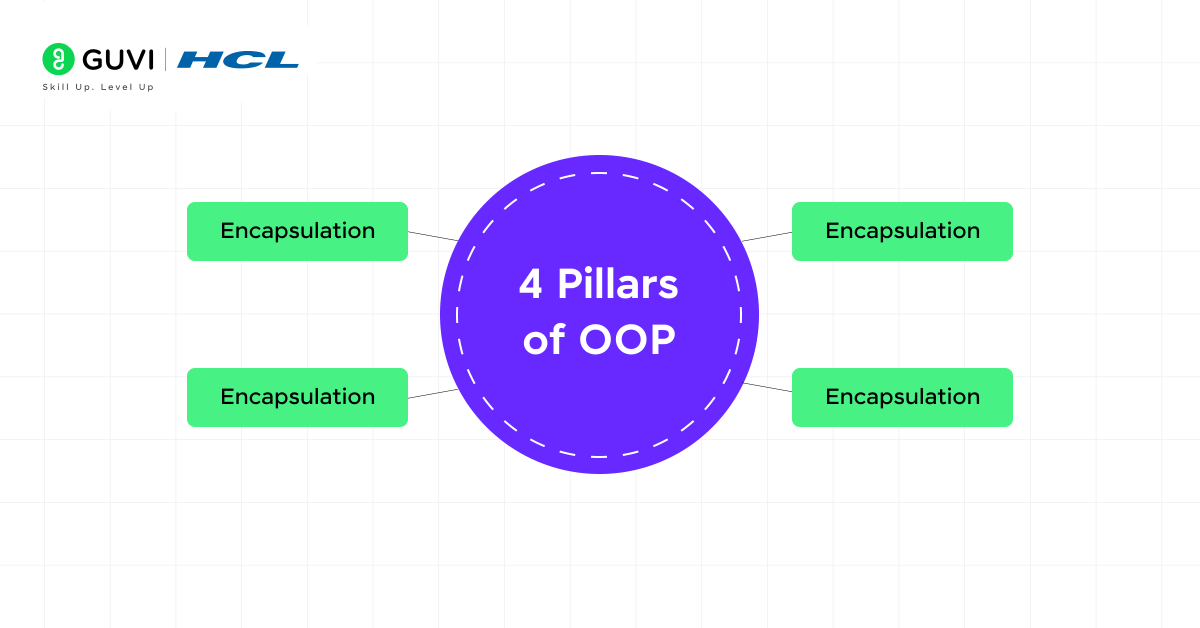
OOP is a programming paradigm that is based on objects, which can hold both data and methods. There are four principles in OOP such as encapsulation, inheritance, polymorphism and abstraction. These principles help write clean and organized code, making it reusable.
- What is an algorithm?
An algorithm is a step-by-step procedure to solve a problem or perform a task. Algorithms are crucial in programming, as they determine the efficiency and speed of your code.
- How does version control work, and why is it important?
Version control manages changes to code, allowing multiple developers to collaborate effectively. Git is a popular tool that records revisions and tracks who made changes.
- Write a function in JavaScript to check if a number is even.
function isEven(num) { return num % 2 === 0; } console.log(isEven(4)); // true console.log(isEven(7)); // false |
- What are linear data structures?
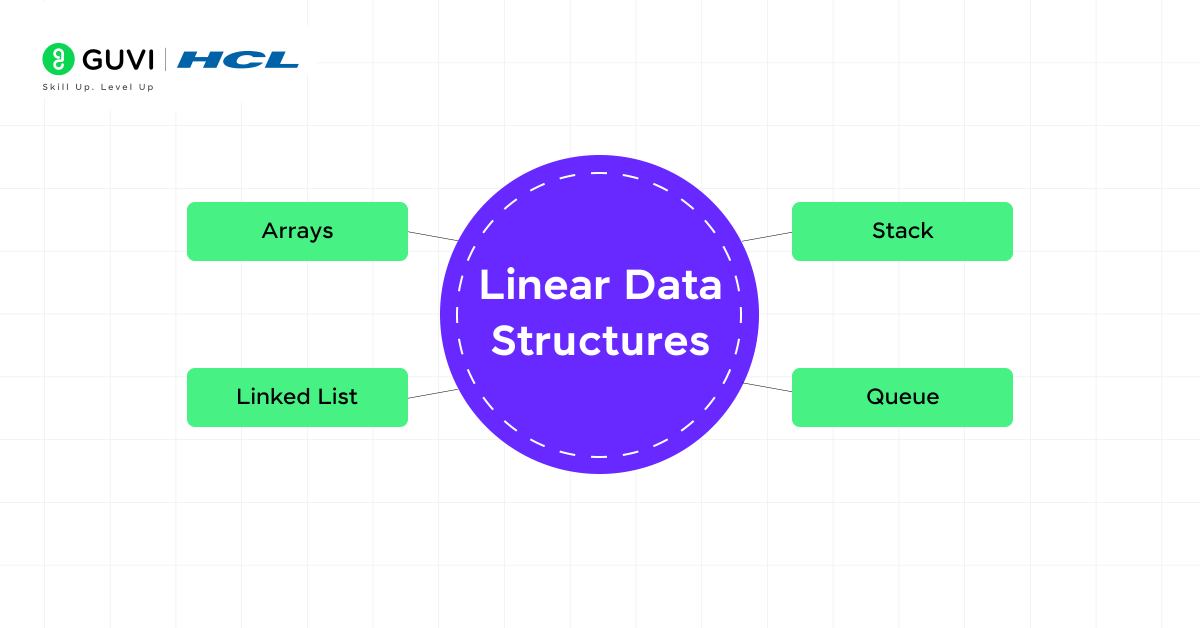
Linear data structures are simple yet powerful data structures that arrange elements in a sequential or linear order. Some of the common linear data structures are arrays, linked lists, stacks, and queues.
- Arrays: It is a fixed-size collection of elements of the same data type in a contiguous memory location.
- Linked List: It is a sequence of nodes where each node points to the next node.
- Stack: It follows the Last In First Out (LIFO) principle, like a pile of plates.
- Queue: It follows the First In First Out (FIFO) principle, like a waiting line.
- Write a function in Python to reverse a string.
def reverse_string(s): return s[::-1] print(reverse_string(“Hello”)) # This function uses Python’s slicing technique to reverse a string. |
- Can you explain what an API is?
An API (Application Programming Interface) is a set of rules that allows different software programs to communicate with each other. For example, a weather app might use a weather API to get real-time data.
- What’s the difference between a compiled and an interpreted language?
A compiled language is translated into machine code before execution (like C or C++), which usually makes it faster. An interpreted language is translated line by line at runtime (like Python or JavaScript), which can be more flexible but a bit slower.
- Describe the differences between a stack and a queue.
A stack operates in a “last in, first out” (LIFO) manner, while a queue follows “first in, first out” (FIFO). For instance, adding books to a stack works from the top, while people joining a queue are served in order.
Mastering any one of the programming languages is a must. If you’re confused about which language to choose, start exploring Guvi’s FREE E-book on Python: A Beginner’s Guide to Coding & Beyond. It is a great start towards your DSA and software developer journey.
Intermediates
This section covers various topics such as Non-linear data structures, Operating System (OS), Database Management System (DBMS) and Programming. Now, let’s get into the intermediate-level software developer interview questions and answers briefly!
- What are non-linear data structures?
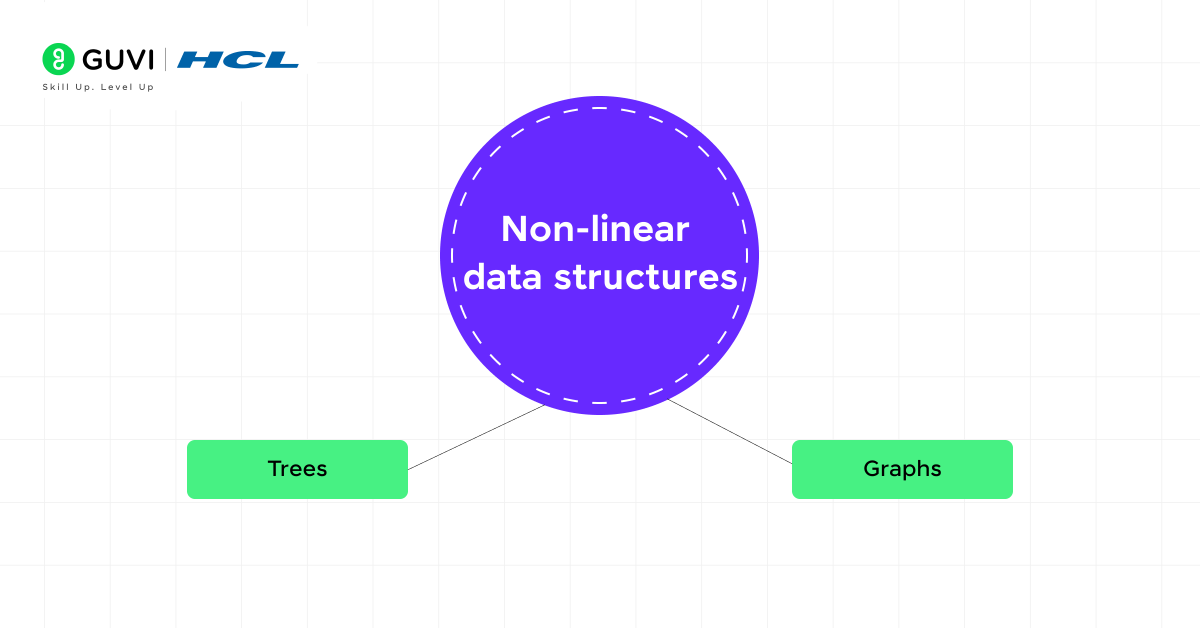
Non-linear data structures are structures where data elements are arranged in a non sequential order. Instead, they are connected in a hierarchical or network-like structure. Common non-linear data structures are trees and graphs.
- Implement a function to sort an array using the Bubble Sort algorithm.
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n – i – 1): if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j] return arr # Example usage print(bubble_sort([64, 34, 25, 12, 22, 11, 90])) # Output: [11, 12, 22, 25, 34, 64, 90] |
- Explain the importance of Big O notation.
Big O notation describes an algorithm’s efficiency based on input size, helping developers predict runtime and optimize performance.
- What is Normalization?
Normalization is the process of organizing a database to minimize redundancy and avoid undesirable characteristics like insertion, update, and deletion anomalies. The goal of normalization is to break down complex data structures into smaller, manageable parts that are easier to maintain and update.
- Implement a function to check if a number is prime.
def is_prime(n): if n <= 1: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True # Example usage print(is_prime(7)) # Output: True |
- What is a deadlock in an operating system?
A deadlock occurs when two or more processes are waiting for each other to release resources, and none of them can proceed. It’s like two people holding one key each, but both need both keys to unlock a door, so they wait forever.
- What is the difference between a process and a thread?
Process | Thread |
It is a program in execution state | It is a lightweight unit of a process |
It runs independently | It depends on the parent process |
It needs inter-process communication | It directly access shared memories |
- What are CRUD Operations? Explain with examples.
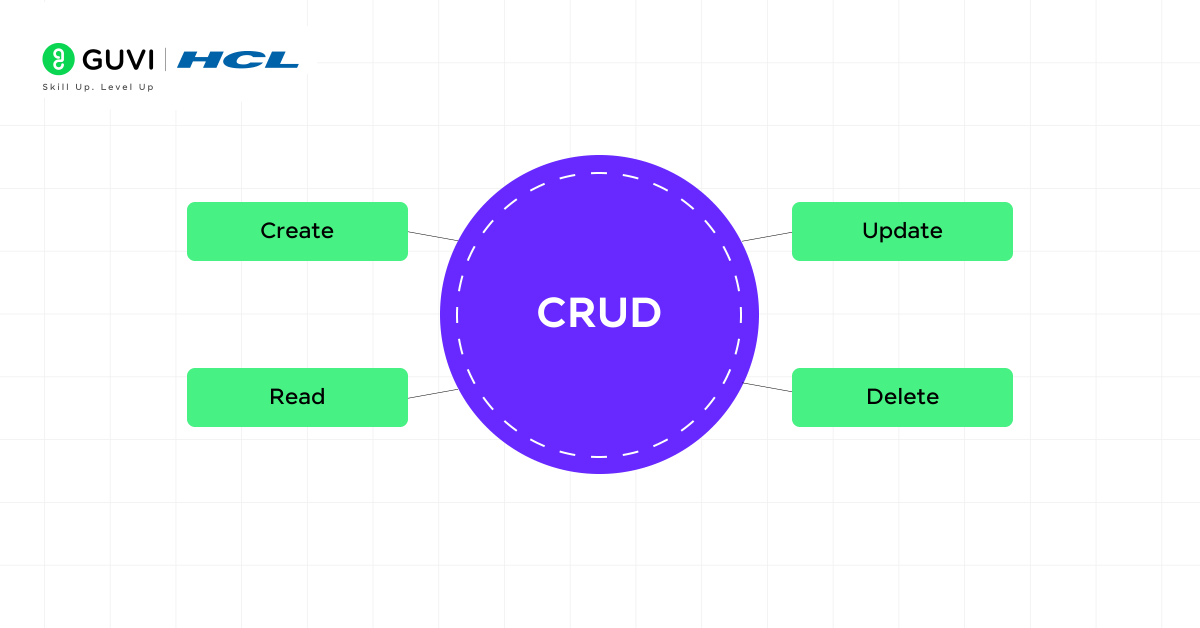
CRUD stands for Create, Read, Update, and Delete, which are the four basic operations for managing data in a database.
- Create: It is used to create a table and insert new records into the table.
- Read: It is used to retrieve records from the table (SELECT * FROM Students;).
- Update: It is used to modify the existing records in the table (UPDATE Students SET Age = 21 WHERE StudentID = 3;).
- Delete: It removes the records from a table (DELETE FROM Students WHERE StudentID = 1;).
- What is RDBMS?
RDBMS stands for Relational Database Management System. It is a subset of the database management system (DBMS), it only stores structured data such as tables. RDBMS defines relationships between tables through the concept of foreign keys. It is based on the relational model of data, where tables represent data and relationships among them. Examples of popular RDBMS include MySQL, PostgreSQL, Oracle, and SQL Server.
- What are the types of joins?
There are five types of joins in SQL. They are:
- INNER JOIN: This join returns only the rows that have matching values (common values) in both tables.
- LEFT JOIN: It is also known as LEFT OUTER JOIN. It returns all the rows from the left table and only returns the matching rows from the right table. If there are no matching rows, then NULL values are returned from the right table.
- RIGHT JOIN: It is also known as RIGHT OUTER JOIN. This join returns all the rows from the right table and only returns the matching rows from the left table. If there are no matching rows, it will return NULL values from the left table.
- FULL JOIN: It is also known as FULL OUTER JOIN. This join returns all rows from both the left and right tables if there is a match between either of the left or right tables. No matching rows will return NULL values.
- SELF JOIN: This will join a table with itself. It is used to compare the rows within the same table.
If you want to become a full stack developer and learn the necessary skills required for it starting from scratch to advance in a single course from India’s top Industry Instructors, consider enrolling in GUVI’s Full Stack Development course that not only teaches you everything about full-stack development from scratch, but also provides you with hands-on project experience and industry-grade certificate!
Experienced
If you are aiming for an experienced role such as SDE-3 or above, then it is mandatory to have system design skills. This section covers concepts such as advanced levels of OS, database security, DSA, and microservices. Let’s get into the software developer interview questions and answers for advanced roles.
- What is multithreading, and why is it used?
Multithreading allows concurrent execution of multiple parts of a program to improve performance, especially in CPU-intensive tasks.
- Explain the difference between optimistic and pessimistic locking in databases.
Optimistic locking assumes conflicts are rare, checking for changes before committing. Pessimistic locking assumes conflicts are frequent, locking records early to prevent them.
def binary_search(arr, target): low, high = 0, len(arr) – 1 while low <= high: mid = (low + high) // 2 if arr[mid] == target: return mid elif arr[mid] < target: low = mid + 1 else: high = mid – 1 return -1 print(binary_search([1, 2, 3, 4, 5], 4)) # Output: 3 |
- What are design patterns? Give an example.
Design patterns are reusable solutions to common programming problems. For example, the Singleton pattern restricts the instantiation of a class to one object, commonly used for database connections.
- What are microservices, and what benefits do they offer?
Microservices divide an application into independent services, each focusing on specific functions, enhancing scalability and flexibility for large applications.
- Write a function to find the longest substring without repeating characters in a given string.
def longest_unique_substring(s): char_map = {} start = max_length = 0 for end in range(len(s)): if s[end] in char_map: start = max(start, char_map[s[end]] + 1) char_map[s[end]] = end max_length = max(max_length, end – start + 1) return max_length # Example usage print(longest_unique_substring(“abcabcbb”)) # Output: 3 (“abc”) |
- Implement a function to perform binary search on a sorted list.
def binary_search(arr, target): left, right = 0, len(arr) – 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: left = mid + 1 else: right = mid – 1 return -1 # Element not found # Example usage print(binary_search([1, 2, 3, 4, 5, 6], 4)) # Output: 3 |
- Write code to implement an LRU (Least Recently Used) cache in Python.
from collections import OrderedDict class LRUCache: def __init__(self, capacity): self.cache = OrderedDict() self.capacity = capacity def get(self, key): if key in self.cache: value = self.cache.pop(key) self.cache[key] = value return value return -1 def put(self, key, value): if key in self.cache: self.cache.pop(key) elif len(self.cache) >= self.capacity: self.cache.popitem(last=False) self.cache[key] = value |
- What is a monolithic application, and how does it differ from microservices?
A monolithic application has tightly integrated components, while microservices split functionality into independent services, making updates easier but adding complexity to deployment.
- Write a function to detect cycles in a linked list.
class ListNode: def __init__(self, x): self.val = x self.next = None def has_cycle(head): slow, fast = head, head while fast and fast.next: slow = slow.next fast = fast.next.next if slow == fast: return True return False # Example usage # Create a linked list with a cycle for testing node1 = ListNode(1) node2 = ListNode(2) node1.next = node2 node2.next = node1 print(has_cycle(node1)) # Output: True |
Conclusion
In conclusion, by preparing these questions across different levels, you can boost your confidence and showcase your knowledge effectively.
Remember, practice makes perfect, so take time to understand the concepts and try coding solutions on your own to reinforce your learning. Good luck with your interview preparation!
FAQs
Most companies follow these rounds:
1. Aptitude/Online Coding Test
2. Technical Interview(s)
3. System Design or Coding Challenge (optional for freshers)
4. HR/Behavioral Round
Data Structures & Algorithms, OOP Concepts, Databases (SQL basics), Operating System & Networking fundamentals, and Basic coding in one programming language (like Java, Python, or C++)
Not in detail. For freshers, companies may ask basic design questions (e.g., how would you design a simple library system or a login page). Understanding fundamentals is enough.
Very important! Explaining your thought process, even if you don’t get the perfect answer, shows your problem-solving skills and how you approach challenges.
Did you enjoy this article?