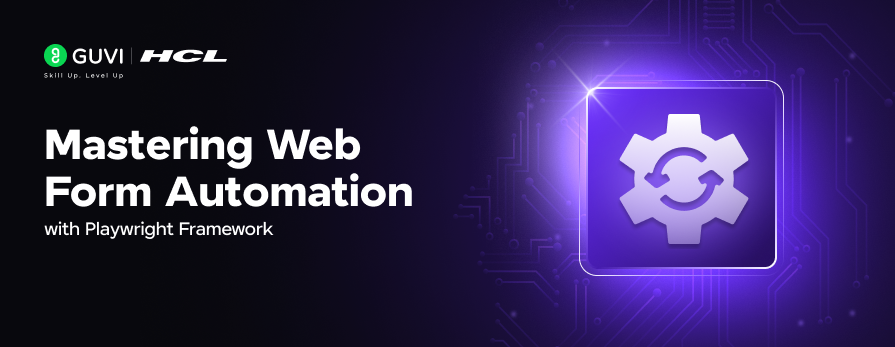
Mastering Web Form Automation with Playwright Framework
Apr 30, 2025 3 Min Read 457 Views
(Last Updated)
In today’s digital landscape, automated testing plays a crucial role in ensuring web applications function seamlessly across different browsers. One of the most efficient automation tools available is Playwright, a powerful framework designed for end-to-end testing.
This guide will walk you through setting up Playwright, structuring test cases, and executing automation scripts to interact with input elements on a web page using Python Playwright.
Table of contents
- What is a playwright?
- Steps to Web Form Automation with Playwright
- Setting Up the Environment
- Project Structure
- Automating Input Elements in Playwright
- Full Playwright Script for Form Automation
- Running the Playwright Script
- Conclusion
What is a playwright?
Playwright is an open-source automation framework developed by Microsoft that supports testing across multiple browsers (Chromium, Firefox, WebKit). It is known for:
- Cross-browser testing
- Headless and headed execution
- Auto-wait mechanism to handle dynamic content
- Powerful selectors for better element handling
Playwright is widely used for web testing due to its fast execution, parallel test capabilities, and support for modern web applications.
Steps to Web Form Automation with Playwright
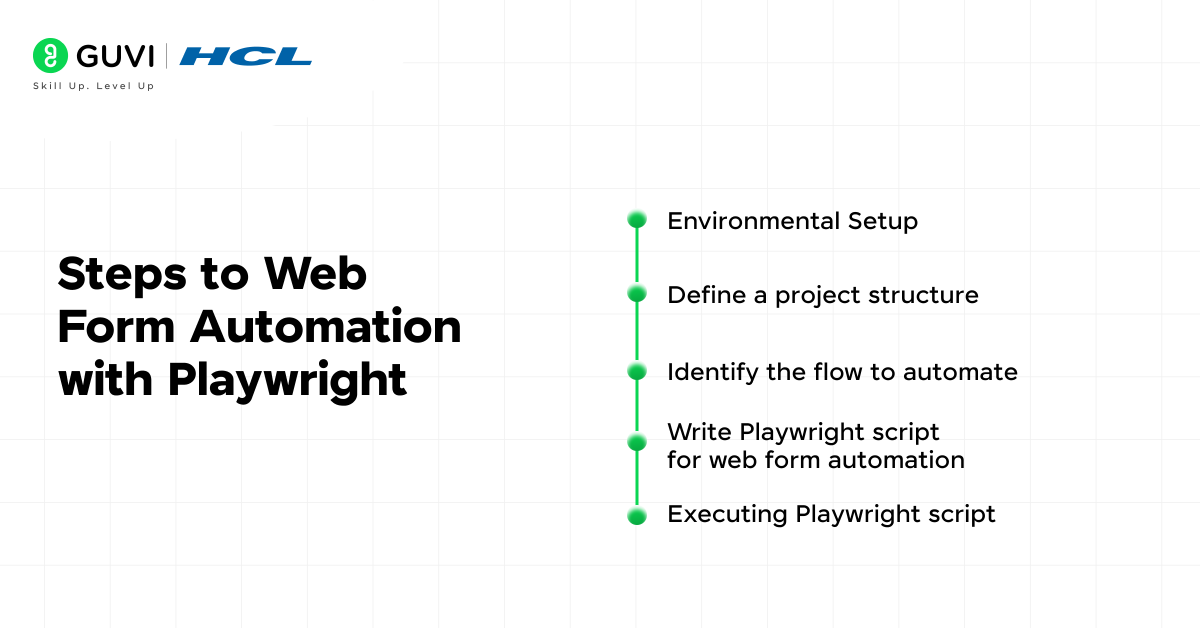
1. Setting Up the Environment
To start automating input elements with Playwright, follow these setup steps:
Step 1: Install Playwright
First, install Playwright and its dependencies using pip:
pip install playwright
Then, install the necessary browsers:
playwright install
Step 2: Install pytest (Optional for Test Case Management)
If you plan to use pytest
for running structured test cases, install it as well:
pip install pytest
Are you interested in starting a career in Python? Kickstart your Python journey using Guvi’s FREE E-book on Python: A Beginner’s Guide to Coding and Beyond. It covers all the necessary concepts you need to know,
2. Project Structure
A well-organized project ensures scalability and maintainability. Here’s an ideal structure for a Playwright test suite:
playwright-tests/
│── tests/
│ ├── test_form.py # Test cases for form interactions
│── utils/
│ ├── browser_setup.py # Playwright setup & teardown functions
│── reports/ # Stores test execution reports
│── pytest.ini # Configuration for pytest (if needed)
│── requirements.txt # Dependencies list
3. Automating Input Elements in Playwright
Let’s consider a sample HTML form hosted at:
🔗 https://suman-dynamic-html-form.netlify.app/
The automation script will perform the following actions:
- Launch a browser
- Fill in personal details
- Select radio buttons and dropdowns
- Click submit and handle alerts
- Close the browser
4. Full Playwright Script for Form Automation
Create a file test_form.py
inside the tests/ directory
and add the following code:
from playwright.sync_api import sync_playwright
from time import sleep
# Define test data
URL = "https://suman-dynamic-html-form.netlify.app/"
FIRST_NAME = "John"
LAST_NAME = "Steward"
ADDRESS = "Bangalore"
PINCODE = "99501"
# Function to automate form filling
def test_fill_form():
with sync_playwright() as p:
# Launch the browser
browser = p.chromium.launch(headless=False) # Set headless=True for faster execution
context = browser.new_context()
page = context.new_page()
# Open the webpage
page.goto(URL)
page.set_viewport_size({"width": 1280, "height": 720})
# Fill input fields
page.fill("input#fname", FIRST_NAME)
page.fill("input#lname", LAST_NAME)
page.fill("input#address", ADDRESS)
page.fill("input#pin", PINCODE)
# Select radio button
page.check("input[value='Male']")
# Select multiple items from dropdown
page.select_option("#food", ["Pizza", "Pasta"])
# Select State and Country
page.select_option("#state", "Karnataka")
page.select_option("#country", "India")
# Click Submit Button
page.click("button:text('Submit')")
# Handle alert
page.on("dialog", lambda dialog: dialog.accept())
# Wait for 5 seconds to observe results
sleep(5)
# Print success message in console
print("SUCCESS, HTML Form Filled !")
# Close the browser
browser.close()
Breakdown of the Playwright Script
- Launching the Browser
browser = p.chromium.launch(headless=False)
context = browser.new_context()
page = context.new_page()
- headless=False allows you to see the automation in action.
- Contexts are used to isolate browser sessions.
- Navigating to the Web Page
page.goto(URL)
page.set_viewport_size({"width": 1280, "height": 720})
- goto() navigates to the test website.
- set_viewport_size() sets the browser window size.
- Filling Input Fields
page.fill("input#fname", FIRST_NAME)
page.fill("input#lname", LAST_NAME)
page.fill("input#address", ADDRESS)
page.fill("input#pin", PINCODE)
- The fill() method enters text into input fields using CSS selectors.
- Selecting Radio Buttons & Dropdowns
page.check("input[value='Male']")
page.select_option("#food", ["Pizza", "Pasta"])
page.select_option("#state", "Karnataka")
page.select_option("#country", "India")
- check() is used for selecting radio buttons and checkboxes.
- select_option() is used for dropdown selections.
- Clicking the Submit Button & Handling Alerts
page.click("button:text('Submit')")
page.on("dialog", lambda dialog: dialog.accept())
- click() is used to submit the form.
- on(“dialog”) listens for alerts and automatically accepts them.
- Closing the Browser
browser.close()
– Ensures that the browser closes after execution.
Are you curious to learn more about automation? Enroll in Guvi’s wonderful course on Selenium Automation with Python. This course covers everything you need to know about Selenium automation using Python from beginner to advanced level. You can gain hands-on experience with the guided projects along with industry-recognized certification.
5. Running the Playwright Script
Execute the script using:
pytest tests/test_form.py
This will launch a browser, fill the form, submit it, handle alerts, and close the browser.
To run tests in headless mode (without opening the browser):
Modify:
browser = p.chromium.launch(headless=True)
Conclusion
This guide provided a structured approach to automating web forms using Python Playwright. We covered:
- Setting up Playwright
- Writing a test script to interact with input elements
- Handling radio buttons, dropdowns, and alerts
- Running and optimizing tests
By leveraging Playwright’s robust API and auto-wait mechanisms, web automation becomes more reliable and efficient. Start integrating Playwright into your testing workflow and streamline web automation effortlessly!
Did you enjoy this article?