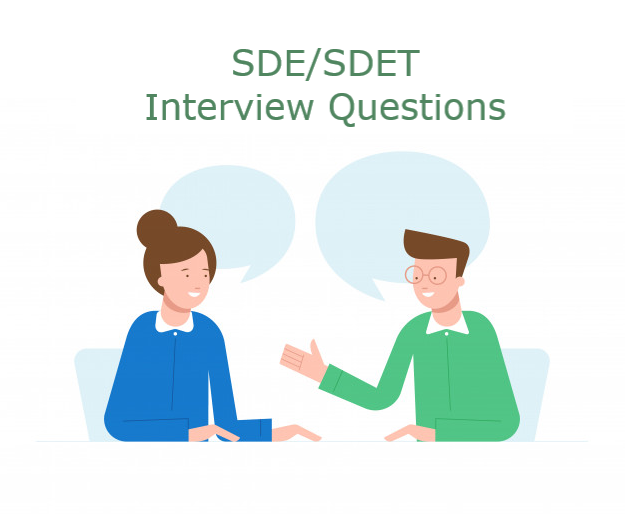
100+ SDE & SDET Interview Questions With Answers
Oct 02, 2024 3 Min Read 5544 Views
(Last Updated)
SDE/SDET Interview questions that will ramp up your interview preparation
Myself, Balaji Boggaram Ramanarayan – I bag experience in taking/preparing for interviews in several big companies and always felt sharing a few of them such that it might be useful for you in interview preparation.
Note: These questions might help you ramp up your speed in the path of preparing for SDE /SDET interviews in most techie companies.
SDE – Software Development Engineer
SDET – Software Development Engineer in Test
Table of contents
- The must-know SDE/SDET Interview Questions
- SDE/SDET Interview Questions- you should be able to answer
- 30+ Software Developer (SDE) / Software Developer In Test (SDET) Questions for you
- Find moreSoftware Developer (SDE) / Software Developer In Test (SDET) Questions
The must-know SDE/SDET Interview Questions
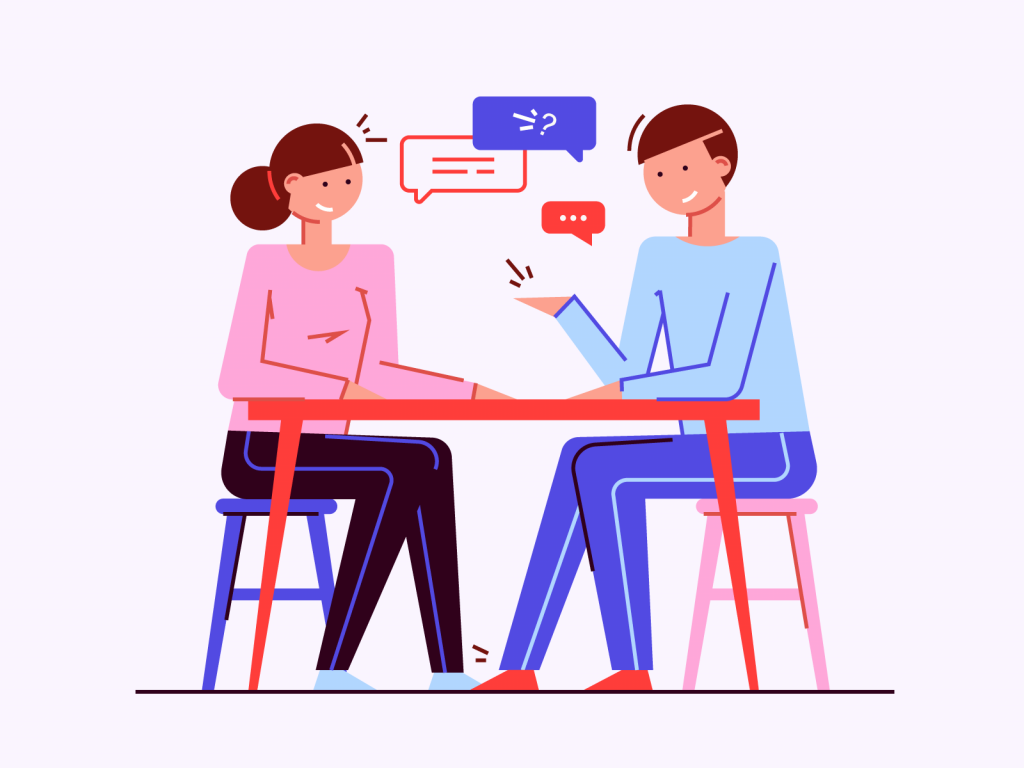
- Heap – Max Heaps and Min Heaps
- Conversions: Decimal, Binary, Hex, Octal (All other possible combinations)
- 90 Degrees Matrix conversion
- Quick Sort + Applications
- Merge Sort + Applications
- Remove duplicates in a String — In place
- Reverse a string – Inplace
- Decide if 2 strings are anagrams or not?
- Binary Search
- Reverse SLL without using any extra nodes
- Maximum Subarray [Kadane Algorithm]
- Find an element that is repeated more than n/2 times in a given set/array. [Moores Voting algorithm]
- Find an element in rotated Binary sorted array
- Implement power function without pow() function
- Verify if the given linked list is circular/cyclic or Acyclic. Follow up can be to indicate the starting point of the cycle
- Implement Blocking queue
- Find a pair in an array that will sum up to a particular number
- Reverse a double linked list
- Reverse pairs in SLL. i.e I/P : a->b->c->d->e->f O/p : b->a->d->c->f->e
- Segregate even and odd nodes in a given linked list
- Addition of 2 linked lists to separate one. (also learn inplace)
- Convert SLL into DLL (XOR based linked lists)
- Circular shift an array of integer input array by ‘k’ number of elements
- Search for a given pattern in the text [Rabin Karp Algorithm]
- atio() and itoa() implementations (ASCII to integer and Integer to ASCII)
- Binary Semaphores
- Generate subsets of a given set of integers
- Generate all permutations of a given string
- Level order Traversal
- The inorder successor of a given node in BST (Binary Search Tree)
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with placement assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
SDE/SDET Interview Questions- you should be able to answer
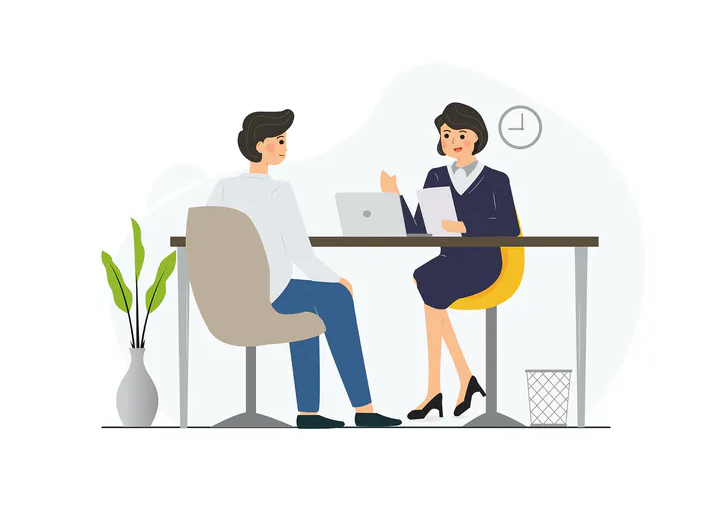
- Find ‘k’ largest /smallest elements in a given array (Hint: can use heaps)
- Heap Sort + applications
- Find minimum length unsorted subarray on which storing them makes a complete sorted array
- Search if a given pattern exists in input text using Suffix Arrays
- Re-arrange a string so that all same characters are ‘d’ distant apart
- Knights tour problem
- Rat in Maze [Back tracking]
- Find out if two rectangles overlap or not
- Where are the closest pair of points in the given plane
- Find all subset of elements in the given set whose sum equals to a given target
- Compute x^y such that it can work for floats and negative values
- Find median of given two input sorted arrays
- Find the total no of zeros in a given array of 1’s followed by 0’s
- If there is any sub-array find the ones which sum up to zero
- Count number of inversions in a given array
- Find a minimum element in a rotated sorted array
- Which is the fixed point in the given array
- Find the maximum subarray sum [D&C]
- Count # of occurrences of a number in the sorted array
- Find the max and min element in a given array with a minimal number of comparisons
30+ Software Developer (SDE) / Software Developer In Test (SDET) Questions for you
- Check if a number is multiple of 3 or not?
- One line function to check if a number is the power of 2 or not?
- Function to multiple a number by 7.
- Which is the function to multiply two numbers without using * product operator
- Function to write Fibonacci series in an iterative manner
- Generate all prime numbers less than or equal to n [Sieve of Erastho..]
- Given a number, Find the next biggest palindrome number
- Implement logic for a fair coin from a biased coin
- Check if a number is divisible by 7 without mod operator
- Find all the possible words from a phone keypad
- Lexicographic sort of permutation of all words
- Shuffle a given array/deck of cards [Fisher Yates Algo]
- Reservoir Sampling Algorithm
- Select ‘k’ random elements from ‘n’ elements
- Given a number ‘n’. generate a pascal triangle out of it.
- Write an exponential precision function [Taylor series]
- Generate all prime factors of a given number
- Generate all possible combinations of ‘r’ elements in a given array of size ‘n’ [Probability Distribution Function]
- Length of Longest common subsequence of a given sequence of numbers
- Find minimum cost path in a given cost matrix
- Total # of solutions in a coin change problem
- Find binomial co-efficient
- Knap-sack standard problem
- Egg-Drop standard problem
- Length of longest palindrome sequence
- Palindrome Partitioning
- Maximum Length of chained increasing pair
- Find the middle of the given linked list
- Check if a given Single linked list is a palindrome or not
- Insert/Delete/Search in max heap
- Implement sizeof() operator
- Find successor of given BST
- Locate all triangle triples in the given array
- Find the lowest common ancestor of a given node
- Return a single element by knocking out all other elements by ‘k’ [Josephus]
Find more Software Developer (SDE) / Software Developer In Test (SDET) Questions
- Given a sorted skewed binary tree, Create a BST out of it.
- Given an array with integers, Output all the elements which were repeated exactly twice
- Maximum depth/height/diameter of a given tree
- Serialize and Deserialize a given binary tree
- Find a single repeated or non-repeated number in the conditional list. (XOR)
- Implement “diff” in Linux
- Program to count # of set bits in a given integer.
- Convert BST to a doubly-linked list
- Convert integer to String without.toString()
- GCD of two numerals
- Given an array in post-order traversal, check whether the given array is in BST or not
- Reverse the words in a given English sentence
- Get the median of a stream of large numbers
- Print all the paths of a given Binary Tree from root to leaf
- Modify array such that arr[i] == arr[arr[i]] Inplace
- Find the missing number in a billion number list
- Bitwise addition
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with placement assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Did you enjoy this article?